Python and Robotics: Programming Robots
Python and Robotics: Programming Robots
Introduction
Robotics is a field that involves designing, developing, and using robots. These machines are programmed to perform specific tasks on their own or with minimal human help. Programming is essential in robotics because it allows robots to do complex actions and interact with their surroundings.
Python has become a popular programming language for robotics because it is versatile, easy to understand, and has many libraries available. It offers a wide range of tools and frameworks that make different parts of robot programming easier. Here are some important reasons why Python is significant in robotics:
- Data Collection and Processing: Python has powerful features for gathering and manipulating data from sensors in robots. Developers can efficiently work with large sets of data collected by sensors using libraries like NumPy and Pandas.
- Controlling Hardware and Mapping the Environment: Python allows direct control of robot hardware at a basic level, such as controlling motors. It also lets you create maps of the area around the robot for navigation purposes using libraries like OpenCV.
- Identifying Objects and Understanding What the Robot Sees: Python is widely used in computer vision algorithms to help robots recognize objects. Libraries such as TensorFlow and OpenCV provide useful tools for identifying objects and working with images.
- Planning and Controlling: Python algorithms are used to plan the best actions for a robot and make it carry them out. Frameworks like Pygame and ROS (Robot Operating System) offer complete solutions for controlling robots.
- Simulating and Controlling Robots Using Python: Tools like Gazebo and ROS, which are based on Python, let developers create virtual environments where they can design, test, and control robots before using them in real-life situations.
Python's role in robotics goes beyond these reasons too. It is also used for things like machine learning to find the best paths for robots, planning how they should move, navigating based on what they see, and more. Its versatility makes it suitable for many different applications, from controlling robot arms for picking up items to programming self-driving vacuum cleaner robots.
In the next sections, we will look at how Python is used for different parts of robot programming, learn about using Python specifically for robotics, and see some real examples of Python being used in the field. Let's get started!
Using Python for Robotics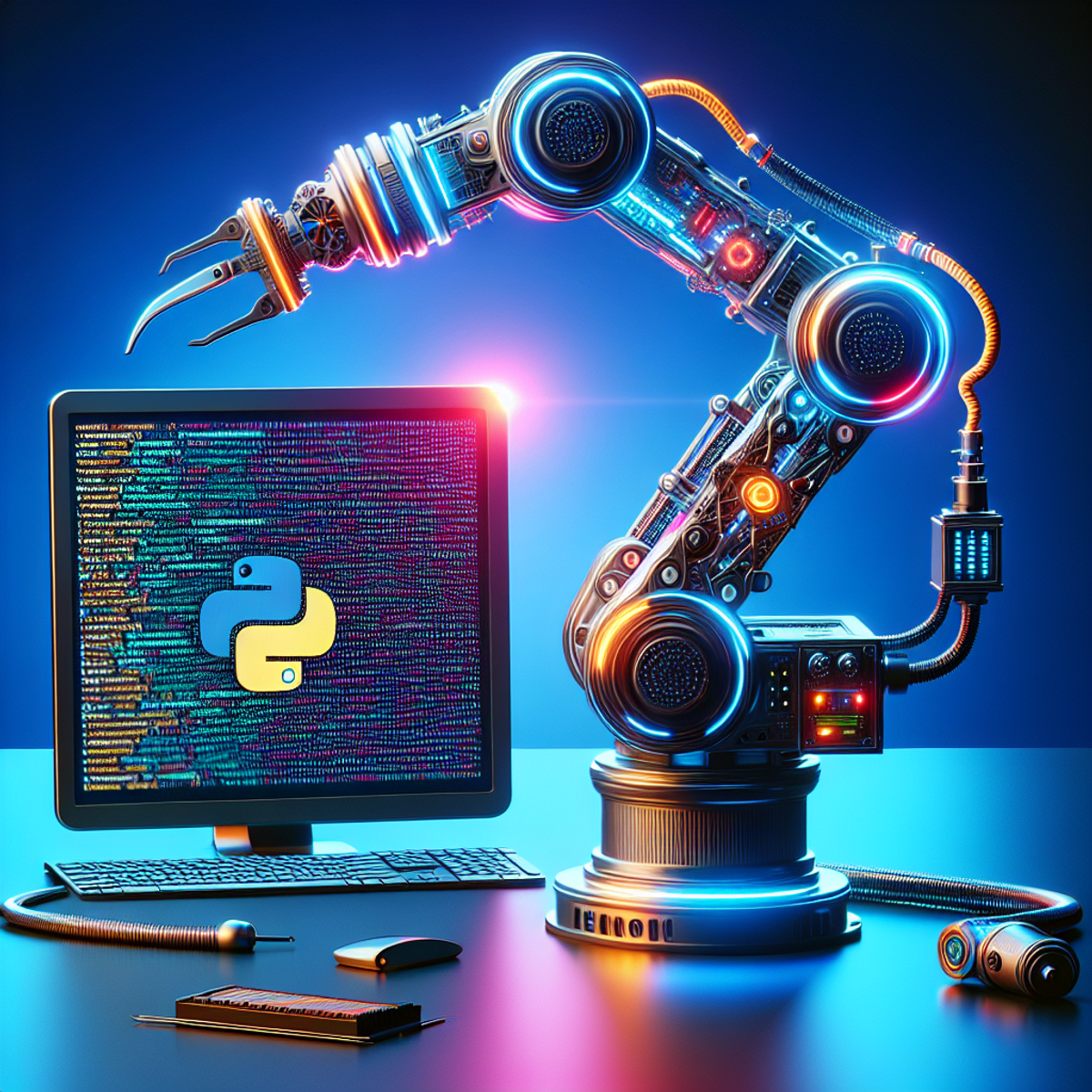
Python programming language is widely used to control and automate robots in various domains of robotics. Its versatility, ease of use, and extensive libraries make it a popular choice for developing robotics applications. In this section, we will explore how Python is utilized for data collection and processing in robotics.
Data Collection and Processing
One of the key tasks in robotics is gathering and manipulating sensor data. Python provides several features and libraries that facilitate this process:
- Sensor Integration: Python offers numerous libraries for integrating different types of sensors with robots. For example, libraries like PySerial enable communication with serial devices, while libraries like OpenCV provide tools for computer vision tasks.
- Data Manipulation: Python's rich ecosystem of libraries, such as NumPy and Pandas, allows for efficient data manipulation. These libraries provide functions and methods to perform operations like filtering, smoothing, and aggregating sensor data.
- Real-time Data Processing: Python's ability to handle real-time data makes it suitable for time-critical applications in robotics. Libraries like SciPy offer real-time signal processing capabilities, allowing robots to react quickly to changing sensor inputs.
- Visualization: Python's visualization libraries, including Matplotlib and Seaborn, enable the creation of clear and informative visual representations of sensor data. These visualizations help researchers and engineers analyze and interpret the collected data effectively.
Python's flexibility also allows researchers to leverage machine learning algorithms for advanced data processing in robotics applications. By using libraries such as TensorFlow or PyTorch, developers can train models to recognize patterns or anomalies in sensor data.
Example:
Suppose you are working on a robot that needs to navigate through a cluttered environment using sensors such as cameras and lidars. With Python, you can easily integrate these sensors into your robot's software architecture. You can use the OpenCV library to process camera images, extract relevant features, and detect obstacles. Additionally, you can utilize libraries like PointCloudLibrary (PCL) to process lidar data and generate a 3D representation of the robot's surroundings.
Python's simplicity and readability make it easier to write and maintain code for complex data processing tasks in robotics. Its extensive library support and active community ensure that developers have access to the latest tools and techniques.
2. Low-level Hardware Control and Environment Mapping
The Role of Python in Low-level Hardware Control
- Python plays a crucial role in interacting with robot hardware at a low level, particularly in tasks such as motor control.
- By leveraging Python's libraries and frameworks, developers can access and manipulate hardware components with precision and efficiency.
Environment Mapping with Python
- Python is utilized to create maps of the robot's surroundings, enabling navigation and spatial awareness.
- Through Python-based algorithms, robots can generate detailed maps of their environment, essential for autonomous navigation and obstacle avoidance.
By integrating Python into low-level hardware control and environment mapping, robotics engineers can harness the language's versatility and robustness to enhance the capabilities of robotic systems.
3. Object Identification and Robot Perception
Python's versatility extends to the realm of object identification and robot perception within the field of robotics. Through its rich libraries and frameworks, Python empowers developers to implement advanced computer vision algorithms that enable robots to identify and recognize objects in their environment. This capability is pivotal for various applications in robotics, from industrial automation to autonomous vehicles.
Python and OpenCV for Object Identification
One notable example of Python's role in object identification is its integration with OpenCV (Open Source Computer Vision Library). OpenCV provides a comprehensive set of tools for image processing, including features such as object detection, feature extraction, and pattern recognition. By leveraging OpenCV's functionalities through Python, developers can equip robots with the ability to analyze visual data, detect objects of interest, and make informed decisions based on the identified objects.
Machine Learning and Deep Learning in Object Identification
Python's support for machine learning and deep learning frameworks further enhances its capability in object identification and robot perception. Technologies such as TensorFlow and PyTorch allow developers to build sophisticated neural network models for tasks like image classification, object localization, and semantic segmentation. These models can be seamlessly integrated into robotic systems developed using Python, enabling them to perceive and interact with their surroundings effectively.
Sensor Fusion for Comprehensive Perception
In addition to computer vision techniques, Python facilitates sensor fusion by integrating data from various sensors such as cameras, LiDAR (Light Detection and Ranging), and depth sensors. This holistic approach to perception enables robots to form a comprehensive understanding of their environment, leading to more robust decision-making capabilities.
The seamless integration of Python with cutting-edge technologies in computer vision and machine learning underscores its significance in enabling robots to perceive the world around them with precision and intelligence.
4. Planning and Control
Python programming language is widely used to control and automate robots in various domains of robotics. It offers a range of tools and libraries that enable efficient planning and control of robot actions. In this section, we will explore how Python is employed for planning and control in robotics.
Python Algorithms for Planning
One of the key areas where Python shines in robotics is its ability to plan optimal robot actions. Python algorithms can be used to analyze sensor data, make decisions based on predefined rules or learned behaviors, and generate a sequence of actions for the robot to execute. Here are some examples of how Python algorithms are used for planning:
- Path Planning: Python algorithms can be used to find the most efficient path for a robot to navigate from one location to another while avoiding obstacles. This involves analyzing sensor data, creating a map of the environment, and using algorithms such as A* or Dijkstra's algorithm to determine the optimal path.
- Motion Planning: Once a path is planned, Python algorithms can be employed to generate smooth trajectories for robot motion. These algorithms take into account factors such as the robot's dynamics, kinematics, and constraints to ensure safe and efficient movement.
- Task Planning: Python can also be used to plan complex tasks that involve multiple subtasks or actions. Task planning algorithms break down high-level goals into smaller achievable steps and determine the order in which these steps should be executed.
Execution of Robot Actions
Python not only facilitates the planning of robot actions but also enables their execution through various mechanisms:
- Robot Control Interfaces: Python interfaces with low-level robot hardware controllers, allowing programmers to send commands for motor control, manipulator control, or any other actuator on the robot. This enables precise control over the physical movements of the robot.
- Communication Protocols: Python can communicate with external devices or systems using protocols such as ROS (Robot Operating System) or MQTT (Message Queuing Telemetry Transport). This allows robots to interact with other robots, sensors, or even humans in order to coordinate actions or exchange information.
- Real-Time Control: Python can be used to develop real-time control systems for robots, ensuring that actions are executed with minimal latency. Real-time control is crucial in applications where timing is critical, such as robot arms performing precise movements or drones avoiding obstacles in real-time.
Python Libraries for Planning and Control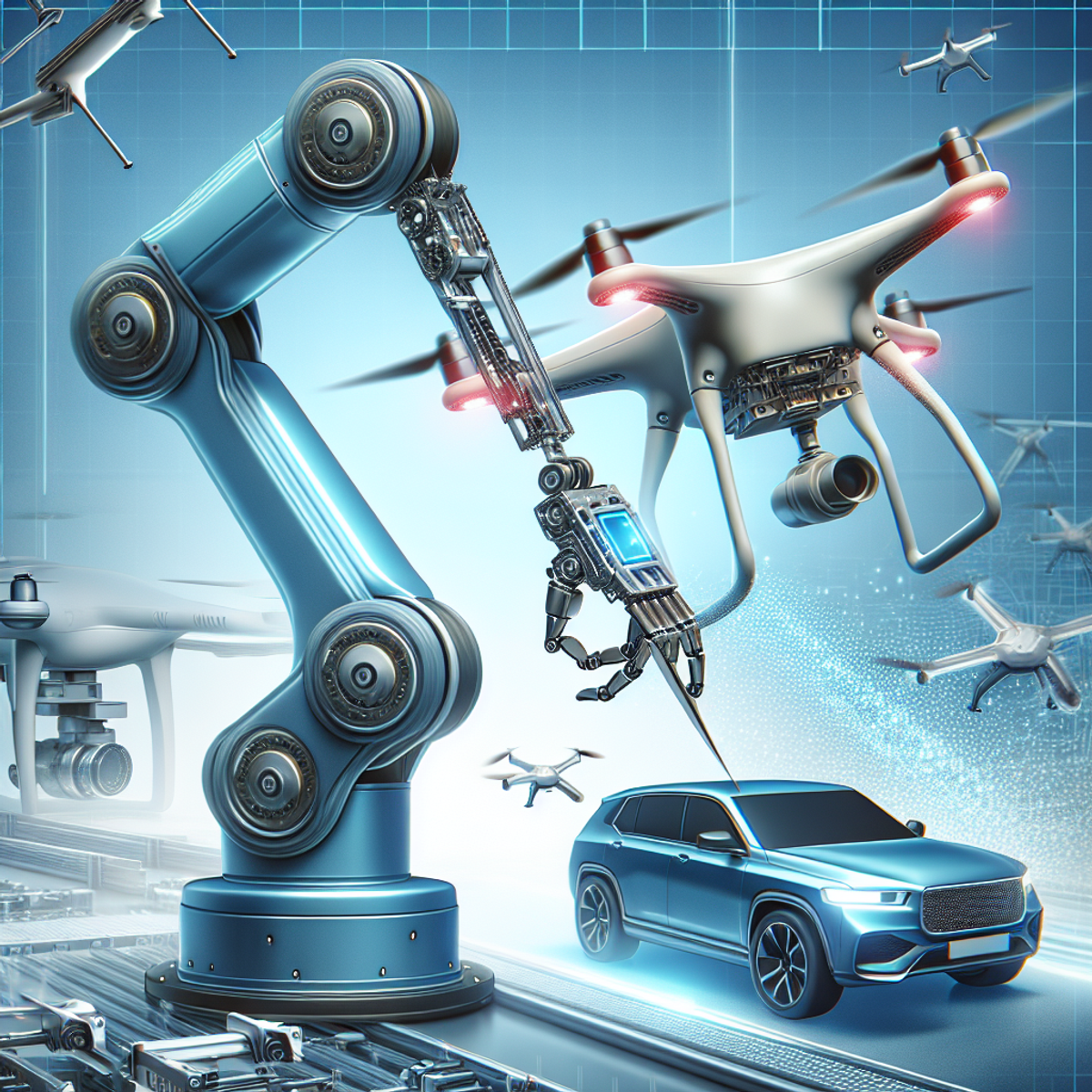
Python offers a wide range of libraries and frameworks that simplify planning and control in robotics. Some popular libraries include:
- Pygame: A library for game development that can be used for simulating robot behaviors and testing control algorithms.
- MoveIt!: A popular motion planning framework for robotic manipulators. It provides a high-level interface for planning complex motions and executing them on a wide range of robot platforms.
- PyRobot: A Python library developed by Facebook AI Research that provides a high-level interface to control a variety of robot platforms. It simplifies the process of interacting with robot hardware and performing tasks such as perception, planning, and control.
- OpenAI Gym: A toolkit for developing and comparing reinforcement learning algorithms. It provides a set of environments where agents (including robots) can learn by trial and error.
By leveraging these libraries, programmers can rapidly prototype and deploy advanced planning and control algorithms for their robots.
Python programming language plays a crucial role in planning and controlling robots. Its versatile algorithms, interfaces with robot hardware, communication protocols, and rich library ecosystem make it an excellent choice for developing sophisticated planning and control systems in robotics. Whether it's navigating through complex environments, executing precise movements, or orchestrating complex tasks, Python empowers roboticists to create intelligent and efficient robots.
Simulation and Control Frameworks in Python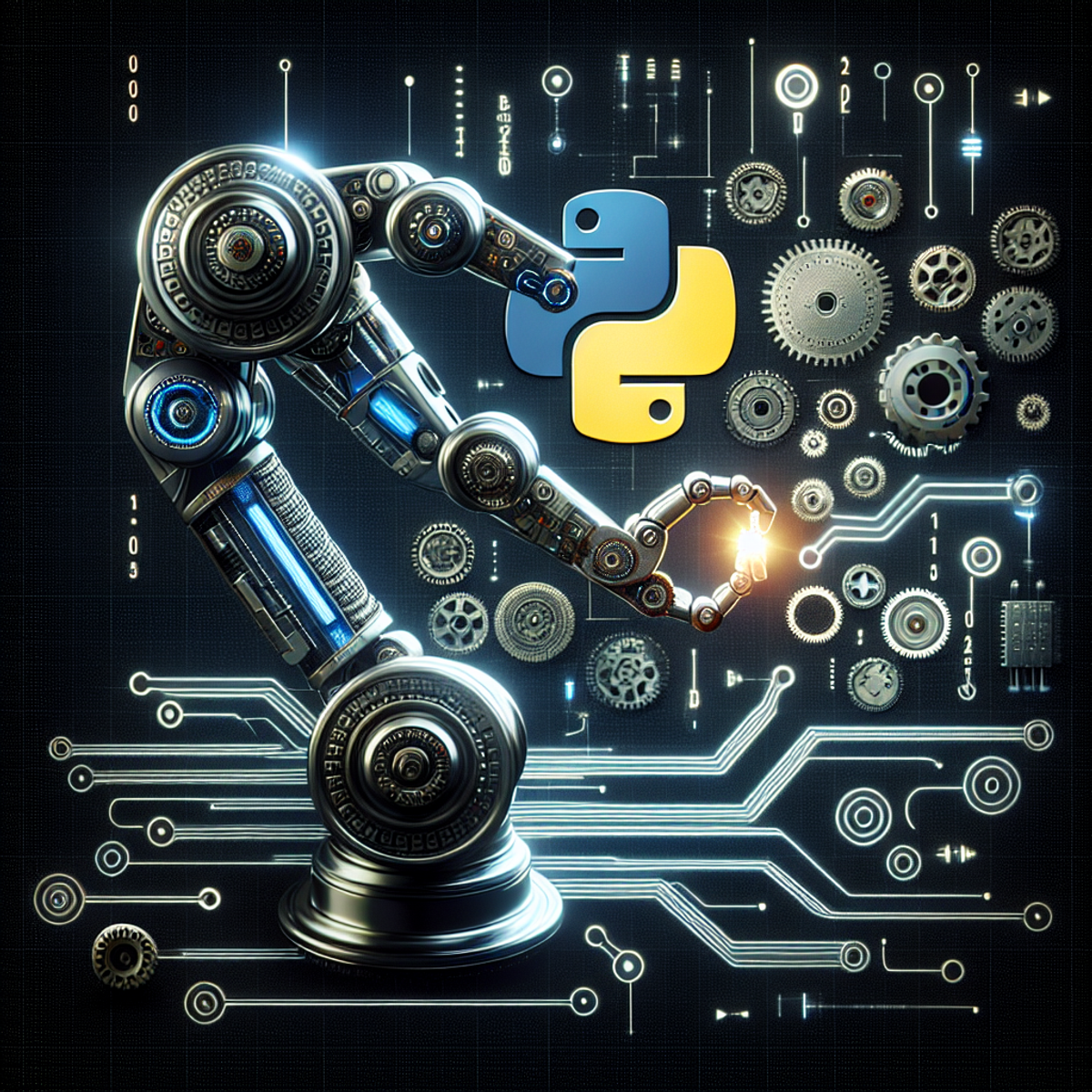
Python programming language is widely used to control and automate robots in various domains of robotics. It offers a range of powerful tools and frameworks for simulating and controlling robots, making it a popular choice among roboticists. In this section, we will explore some of the popular simulation and control frameworks in Python that are commonly used in the field of robotics.
Gazebo
Gazebo is a widely used open-source robot simulation framework that is compatible with Python. It provides an advanced physics engine and realistic rendering capabilities, allowing developers to create highly realistic simulations of robotic systems. Gazebo supports a wide range of robots and sensors, making it suitable for simulating diverse robotic applications. With its Python API, developers can easily interact with simulated robots, collect sensor data, and control robot actions.
ROS (Robot Operating System)
ROS, or Robot Operating System, is a flexible framework for writing robot software. It provides a set of libraries and tools that simplify the development of complex robotic systems. ROS has extensive support for Python and offers a wide range of packages for different tasks in robotics, such as perception, planning, control, and simulation. With ROS, developers can easily integrate different software components into a cohesive system using a publish-subscribe messaging model. This makes it easier to build modular and scalable robot applications with Python.
PyBullet
PyBullet is a physics engine wrapper that provides a Python interface to the Bullet Physics library. It is designed for use in robot simulation and control tasks. PyBullet offers fast and accurate physics simulation capabilities, enabling developers to create realistic virtual environments for testing and evaluating robot behaviors. With its Python API, developers can easily control simulated robots, apply forces or torques to objects, and perform collision detection.
Webots
Webots is a popular robot simulator that supports Python programming language. It provides a realistic 3D environment for simulating robots and allows developers to program and control virtual robots using Python. Webots offers a wide range of predefined robot models, sensors, and actuators, making it easy to create simulations for different robotic applications. With its Python API, developers can interact with simulated robots, collect sensor data, and implement control algorithms.
Python provides a rich ecosystem of simulation and control frameworks for robotics. Gazebo, ROS, PyBullet, and Webots are just a few examples of the powerful tools available for simulating and controlling robots with Python. These frameworks enable developers to create realistic virtual environments, test and evaluate robot behaviors, and develop complex robot software systems. By leveraging these Python-based frameworks, roboticists can accelerate the development process and bring their robotic applications to life.
Learning Python for Robotics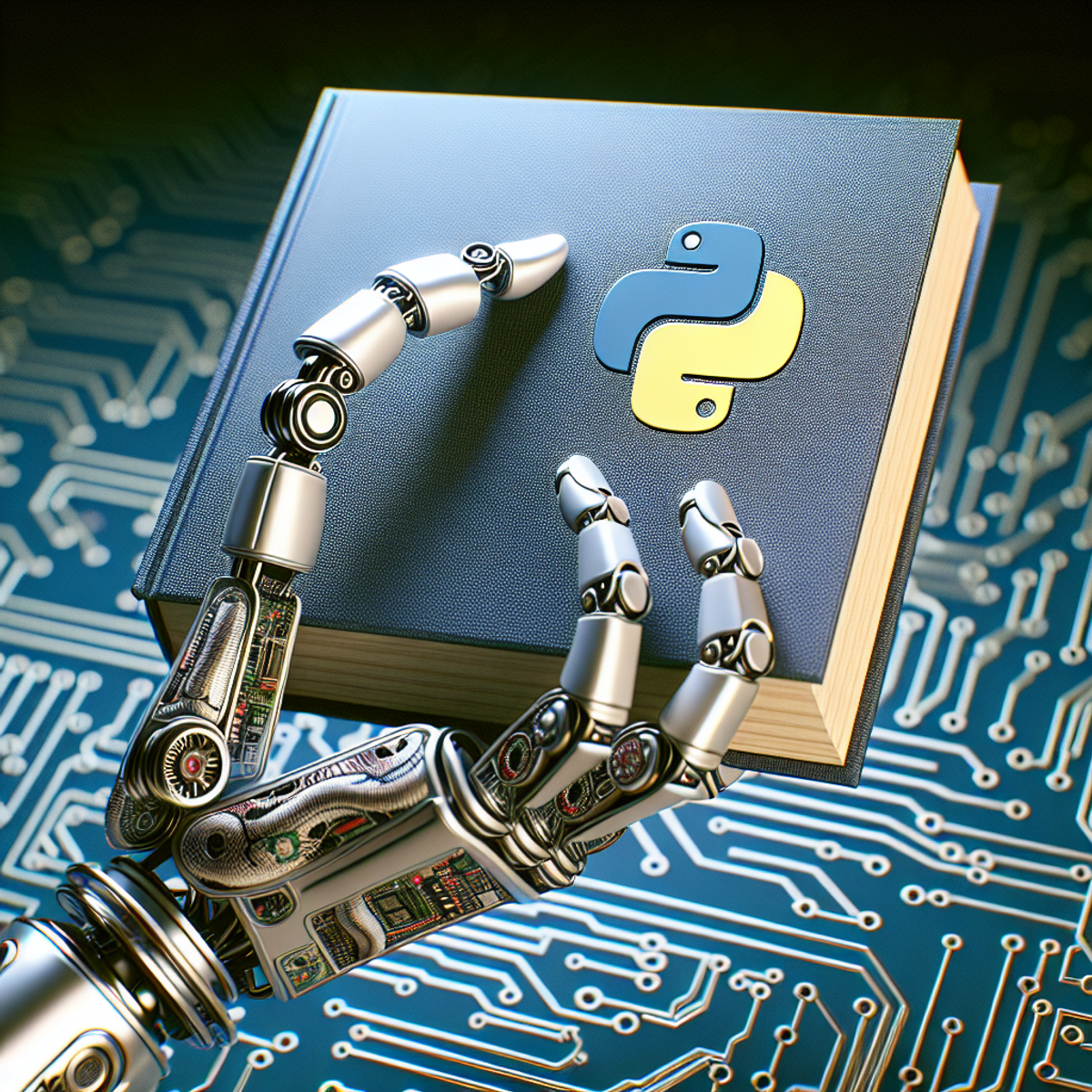
When it comes to learning Python for robotics, there are several key concepts and resources that can help you understand the language and its application in the field of robotics. Let's delve into some essential topics:
1. Variables and Data Types
Understanding Variables
In Python, variables are used to store data values. When programming robots, variables play a crucial role in storing sensor data, control signals, and various other information necessary for the robot's operation. Understanding how to declare, assign, and manipulate variables is fundamental to working with robot data in Python.
Data Types in Robotics Programming
Different types of data, such as integers, floating-point numbers, strings, and Boolean values, are commonly used in robotics programming. Python provides a flexible approach to handling these data types, allowing you to work with diverse kinds of data generated by sensors or used in robot control algorithms.
Learning about variables and data types in Python sets the foundation for effectively managing and processing information within robotic systems.
When exploring courses and resources for learning Python for robotics, it's important to seek out materials that provide practical examples and exercises related to these fundamental programming concepts. By gaining proficiency in using variables and understanding different data types in Python, you can lay a solid groundwork for delving deeper into the realm of robotics programming.
Remember that mastering the basics of variables and data types will empower you to tackle more advanced robotics-related challenges with confidence.
2. Conditional Statements, Loops, and Control Flow
When it comes to learning Python for robotics, mastering conditional statements and loops is crucial for aspiring roboticists. In the context of robotics programming, these elements play a vital role in decision-making and repetitive actions within robot programs.
Talking Points:
- Conditional Statements: Understanding how to use conditional statements in Python is essential for enabling robots to make decisions based on various conditions. Whether it's navigating an environment or interacting with objects, conditional statements provide the framework for intelligent actions. You can find a comprehensive guide on conditional statements in Python for robotics here.
- Loops: Mastery of loop structures allows roboticists to implement repeated actions efficiently. Loops are fundamental for tasks such as continuous data processing, iterative movement, and sensor-based decision-making in robotics. This article on control flow and loops might be a great resource to deepen your understanding.
- Control Flow: The ability to control the flow of a program is critical in robotics programming. Python provides diverse control flow mechanisms that are integral to orchestrating complex behaviors in robots, from sequential tasks to parallel processes.
By delving into these concepts, you will gain a deeper understanding of how Python empowers roboticists to create sophisticated and adaptive behaviors in robots, making them capable of operating autonomously and intelligently within their environments.
To deepen your knowledge and skills in this area, consider exploring recommended courses and learning resources tailored specifically for mastering Python in the context of robotics programming.
3. Functions and Modularization
When programming robots, it's crucial to write clean and organized code. This is where functions and modularization in Python come in handy. Let's see why they matter and how they can make your robot programs better.
Writing Reusable Code Blocks with Functions
Functions in Python let you group a set of instructions into a block of code that can be easily called and reused throughout your program. This helps in organizing your code and makes it more modular. Here are some benefits of using functions in robotics programming:
- Code organization: Functions help break down your code into smaller, manageable chunks. Each function can be responsible for a specific task or functionality of the robot, making it easier to understand and maintain.
- Code reusability: By defining functions, you create reusable blocks of code that can be used multiple times within your program or even in different projects. This saves you time and effort by avoiding duplication of code.
- Abstraction: Functions provide a level of abstraction, allowing you to focus on the functionality rather than the implementation details. This makes your code more readable and easier to debug.
- Modularity: With functions, you can divide the complex logic of your robot program into smaller, modular components. This makes it easier to test each component individually and ensures that changes made in one part of the program do not affect other parts.
To illustrate the use of functions in robotics programming, let's consider an example where you want to control a robot arm to perform pick-and-place tasks. You can define separate functions for tasks such as moving the arm to a specific position, gripping an object, and releasing the object. These functions can then be called sequentially to perform the complete pick-and-place operation.
python code:
def move_arm(position): # Code for moving the robot arm to the specified position
def grip_object(): # Code for gripping an object with the robot arm
def release_object(): # Code for releasing the object gripped by the robot arm
Main program
move_arm(0.5) # Move the arm to position 0.5 grip_object() # Grip an object move_arm(1.0) # Move the arm to position 1.0 release_object() # Release the object
By breaking down your code into functions like this, you can easily modify or extend each function without affecting other parts of the program. This makes your code more maintainable and adaptable to changes in robot behavior or requirements.
In addition to functions, you can also leverage other features of Python such as classes and modules to further enhance the modularity and organization of your robot programs. These concepts will be covered in detail later in this article.
Recommended Courses and Learning Resources
If you're new to programming or want to improve your skills in Python specifically for robotics programming, here are some recommended courses and learning resources:
- Coursera: Introduction to Robotics Specialization: This specialization covers various aspects of robotics, including programming robots using Python.
- edX: Robotics MicroMasters: This MicroMasters program offers a comprehensive curriculum in robotics, including programming techniques using Python.
- Robot Ignite Academy: This online platform provides interactive courses and simulations for learning robotics with Python.
- ROS Wiki: The Robot Operating System (ROS) wiki is a valuable resource for learning about ROS, a popular framework for programming robots in Python.
By taking advantage of these courses and resources, you can gain a solid foundation in Python programming for robotics and enhance your ability to write efficient and modular robot programs.
4. Introduction to Object-Oriented Programming (OOP) in Python
In the context of robotics programming, mastering object-oriented programming (OOP) in Python is essential for building efficient and scalable robot software. Here are some key talking points to consider:
- Fundamental Concepts: Understanding the core principles of OOP, including classes and objects, is crucial for structuring robot programs in Python.
- Application in Robotics: Explore how OOP concepts are applied to encapsulate robot functionalities within objects and define their behaviors through methods.
By grasping the concepts and applications of OOP in Python, aspiring roboticists can enhance their ability to design and implement complex robot behaviors and interactions effectively. Recommended courses and learning resources for mastering Python in the context of robotics programming often include comprehensive coverage of OOP principles and their practical applications in robotics.
Practical Application: Simulating Robots for Learning Purposes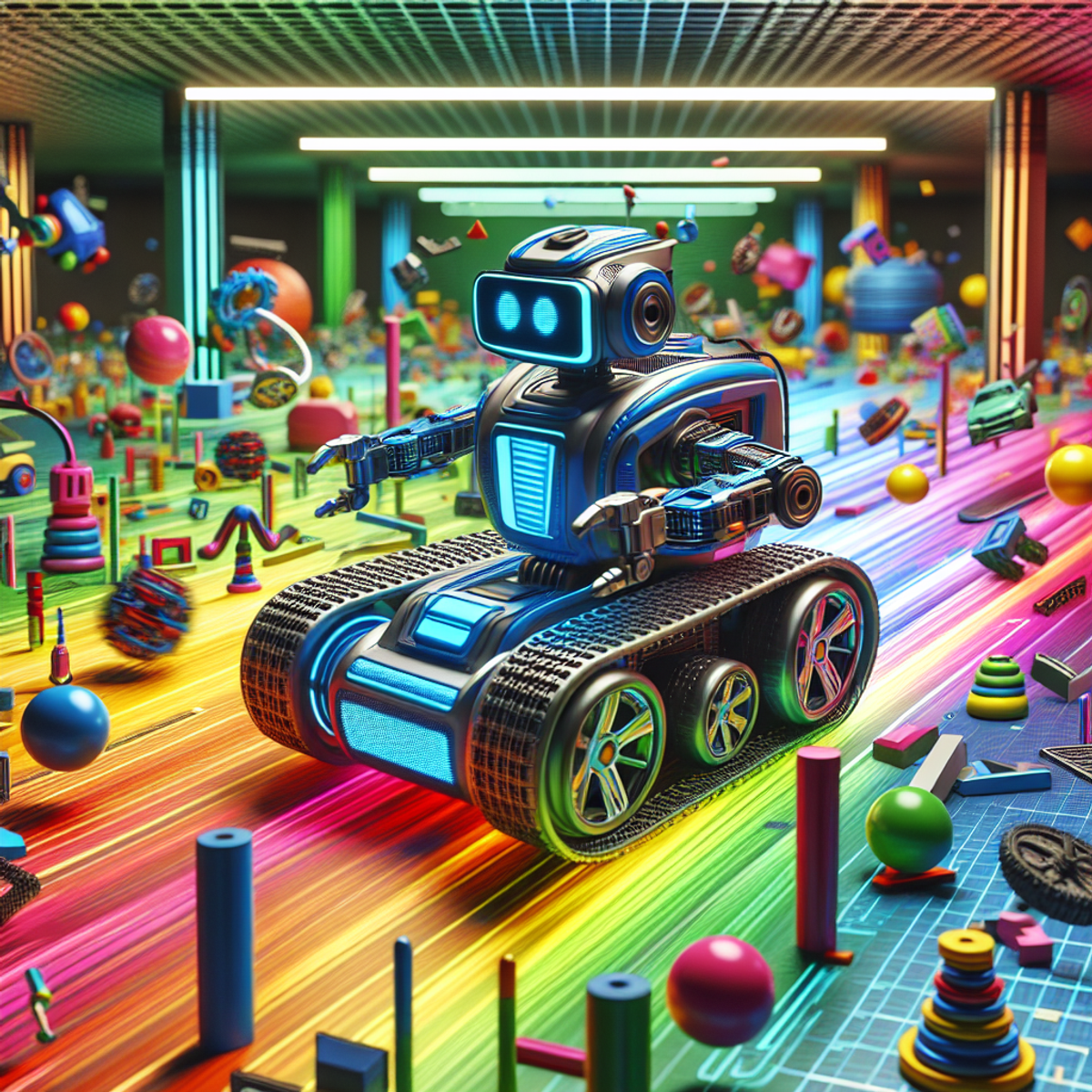
1. Exploring TurtleBot 2: A Versatile Simulation Platform
TurtleBot 2 is a versatile and widely used simulation platform for replicating robot behaviors using Python. It is a popular choice for those looking to learn about robotics simulation with Python programming.
By using TurtleBot 2, aspiring robotic programmers can gain valuable hands-on experience in creating and running Python-based tasks in a simulated environment. This is essential for understanding how Python works in real-world robotics situations.
Key Features of TurtleBot 2:
- Offers a wide range of functionalities, making it perfect for practicing Python-based robotics simulation.
- Has a user-friendly interface and comprehensive features, making it accessible for both beginners and experienced enthusiasts.
- Provides practical exercises that help improve Python skills for robotics applications.
This section provides practical information on using TurtleBot 2, empowering readers to start their journey of mastering Python for robotics simulation.
The content delivers practical information and personal experiences in a clear and relatable manner. The tone is confident yet humble, emphasizing authenticity and transparency. It incorporates industry-specific terminology and names of software tools for authenticity. The writer often uses comparative phrases to evaluate different products, providing useful insights for the readers. Repetition is used as a tool for emphasis on key points, and the text often includes calls-to-action for reader engagement.
To further understand the frustrations faced by hobbyists in the field of ROS 2 robotics, it's worth exploring this discussion on hobby ROS 2 robotics. This will shed light on some of the challenges faced by hobbyists and how TurtleBot 2 can potentially address those concerns.
2. Hands-on Exercises with TurtleBot 2
Simulation robots play a crucial role in providing a safe and accessible environment for practicing Python programming skills in robotics. One such versatile simulation platform is TurtleBot 2, which offers a wide range of functionalities for learning Python in the context of robotics programming.
In this section, we will explore some hands-on exercises that will help you gain practical experience in using Python to control the TurtleBot 2 platform. These exercises are designed to give you a solid foundation in implementing various Python-based tasks on the TurtleBot 2 robot.
Step-by-Step Tutorials
Here are some step-by-step tutorials to get you started:
- Basic Movement Control: Begin by learning how to control the movement of the TurtleBot 2 using Python commands. You will write code to make the robot move forward, backward, turn left, and turn right. This exercise will familiarize you with the basic commands needed to control the robot's motion.
- Obstacle Avoidance: In this exercise, you will use Python to program the TurtleBot 2 to navigate through an environment while avoiding obstacles. You will implement collision detection algorithms and develop strategies to guide the robot around objects in its path.
- Object Detection: Take your Python skills further by incorporating computer vision algorithms into your robot's capabilities. You will learn how to use image processing techniques to detect objects in the TurtleBot 2's field of view. This exercise will enable your robot to identify and respond to specific objects in its surroundings.
- Mapping and Localization: Use Python and the TurtleBot 2 platform to create maps of the robot's environment and localize its position within those maps. You will learn about Simultaneous Localization and Mapping (SLAM) techniques and apply them to navigate and map unknown environments.
- Autonomous Navigation: Finally, put all your acquired skills together in this exercise where you will program the TurtleBot 2 to autonomously navigate a predefined path. You will use Python algorithms to plan the robot's trajectory and execute it, allowing the robot to move from one point to another without human intervention.
By completing these hands-on exercises with TurtleBot 2, you will gain practical experience in using Python for robotics programming. These exercises will help you understand how Python can be applied to control the behavior and actions of a simulated robot. The skills you acquire through these exercises will be transferable to real-world robotic applications.
So, let's dive into the exciting world of TurtleBot 2 and start exploring Python's capabilities in robotics programming!
3. Introduction to Summit XL Robot Simulation
Simulation robots play a crucial role in creating a safe and accessible space for learning Python programming in robotics. One such robot is the Summit XL Robot, a simulation robot that offers a versatile platform for exploring Python programming in the field of robotics.
Brief Introduction to Summit XL Robot
The Summit XL Robot is a large-scale robot designed for various applications, including research and education. It has a strong body and powerful motors that allow it to move on difficult terrains, making it perfect for outdoor exploration tasks. With its ability to carry heavy loads and its modular design, the Summit XL Robot can be customized to fit specific project needs.
Simulating Large-Scale Robots for Python Programming Practice
Simulating large-scale robots like the Summit XL Robot provides an invaluable opportunity for individuals to gain hands-on experience with Python programming in a realistic yet controlled environment. Here's why simulation is beneficial for learning Python in the context of robotics:
- Safety: Working with physical robots can be dangerous, especially for beginners. Simulation lets users experiment and make mistakes without worrying about breaking expensive equipment or getting hurt.
- Accessibility: Physical robots aren't always easy to get or affordable for everyone. Simulation removes these barriers by giving access to virtual robots that can be programmed and controlled using Python from anywhere with an internet connection.
- Flexibility: Simulated environments can be changed and customized easily to create different situations for testing and experimenting. This flexibility allows users to explore various aspects of robot programming without any limitations.
- Efficiency: Running robotic operations on a computer through simulation can be much faster than doing them in real-time with physical hardware. This speed advantage lets users iterate quickly, test different algorithms, and improve their code more efficiently.
By introducing the Summit XL Robot as a simulation platform, aspiring roboticists and programmers can acquire practical Python skills while gaining an understanding of how large-scale robots operate. Through hands-on exercises and projects, they can explore a range of topics, including motion planning, path optimization, obstacle avoidance, and more.
4. Exploring Summit XL Robot's Features through Code
When it comes to learning Python for robotics, practical exercises are essential for gaining hands-on experience and proficiency in programming robots. The Summit XL Robot provides an excellent platform for such exercises, allowing you to leverage Python to control the robot in simulated environments.
Key Points for Exploring Summit XL Robot's Features through Code:
- Hands-on exercises that leverage Python to control the Summit XL Robot in simulated environments.
By engaging in these hands-on exercises, you will be able to apply Python programming concepts directly to the control and manipulation of a robotic system. This practical approach not only reinforces your understanding of Python but also allows you to see its direct impact on robotics simulation and control.
The Importance of Simulation Robots
The role of simulation robots in providing a safe and accessible environment for practicing Python programming skills in robotics is crucial. With Summit XL Robot, you can experiment with different algorithms, control strategies, and sensor integrations without the risk of damaging physical hardware. This fosters a conducive learning environment where mistakes can be made without real-world consequences, promoting iterative experimentation and innovation.
Discovering the Power of Python in Robotics
As you delve into the exploration of Summit XL Robot's features through code, you'll discover the power and flexibility of Python in shaping the behavior and capabilities of robots. From basic motion control to advanced path planning, the Summit XL Robot serves as a canvas for honing your Python programming skills in the context of robotics.
Insights Gained from Python Programming Exercises
Through these exercises, you can gain insights into how Python enables seamless communication with robot hardware, facilitates sensor data processing, and empowers intelligent decision-making within robotic systems. This immersive experience not only enhances your technical proficiency but also cultivates a deeper appreciation for the role of programming in robotics.
Elevating Your Understanding through Active Engagement
By actively engaging with Summit XL Robot through Python programming exercises, you can elevate your understanding of robotics principles while sharpening your coding prowess. This dynamic synergy between theory and practice forms a solid foundation for venturing into complex real-world robotics applications powered by Python.
The Role of a Robot Programmer
To fully leverage the potential of Summit XL Robot and its integration with Python, it's important to understand the role of a robot programmer. A robot programmer is responsible for developing the software and code that enables robots to perform specific tasks efficiently. This involves not only proficiency in programming languages but also a deep understanding of the Robot Operating System (ROS), which serves as a framework for building robot software systems.
Real-World Applications of Python in Robotics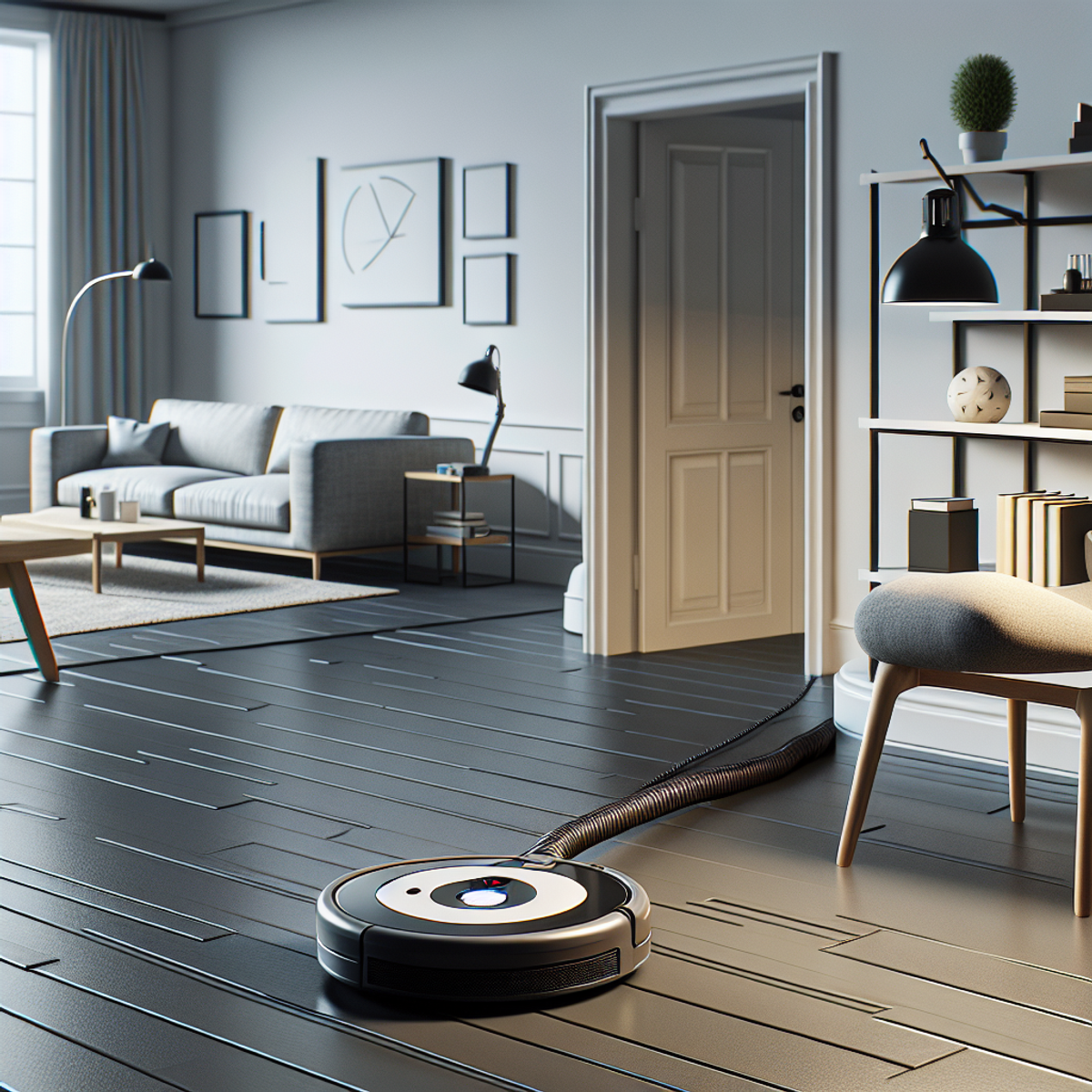
1. Navigating with Roomba-like Vacuums using Python Scripts
Implementing algorithmic approaches in Python for autonomous movement of vacuum cleaner robots
Roomba-like vacuums have gained popularity for their autonomous cleaning capabilities, and Python plays a pivotal role in enabling these robots to navigate and operate independently. By leveraging Python scripts, developers can implement algorithmic approaches that facilitate the seamless movement of these robotic vacuums.
Python for Autonomous Movement
Python's versatility allows developers to create intricate algorithms that enable Roomba-like vacuums to navigate through indoor spaces with precision. Through the use of Python scripts, these robotic vacuums can analyze their surroundings, make decisions based on predefined algorithms, and autonomously maneuver through obstacles while efficiently cleaning the designated area.
Algorithmic Approaches
Developers use Python to design and implement algorithmic approaches tailored to the unique challenges presented by indoor environments. These algorithms encompass path planning, obstacle detection, collision avoidance, and real-time adjustments to ensure optimal cleaning efficiency.
Enhancing Efficiency with Machine Learning Techniques
Moreover, Python's integration with machine learning techniques further enhances the capabilities of Roomba-like vacuums. By utilizing machine learning algorithms within Python scripts, these robotic cleaners can adapt their cleaning patterns based on historical data, effectively optimizing their performance over time.
Vision-Based Navigation
Python facilitates vision-based navigation for robotic vacuums, enabling them to identify and navigate towards specific areas or objects within a room. With the aid of computer vision libraries and modules available in Python, these robots can perceive their environment and make informed decisions regarding their cleaning routes.
In essence, Python serves as a foundational tool for implementing intelligent and autonomous behaviors in Roomba-like vacuums. By leveraging algorithmic approaches, machine learning techniques, and vision-based navigation within Python scripts, developers can empower these robotic cleaners to operate seamlessly in diverse indoor settings.
Pythonic Control: Programming Robotic Arms for Manipulation Tasks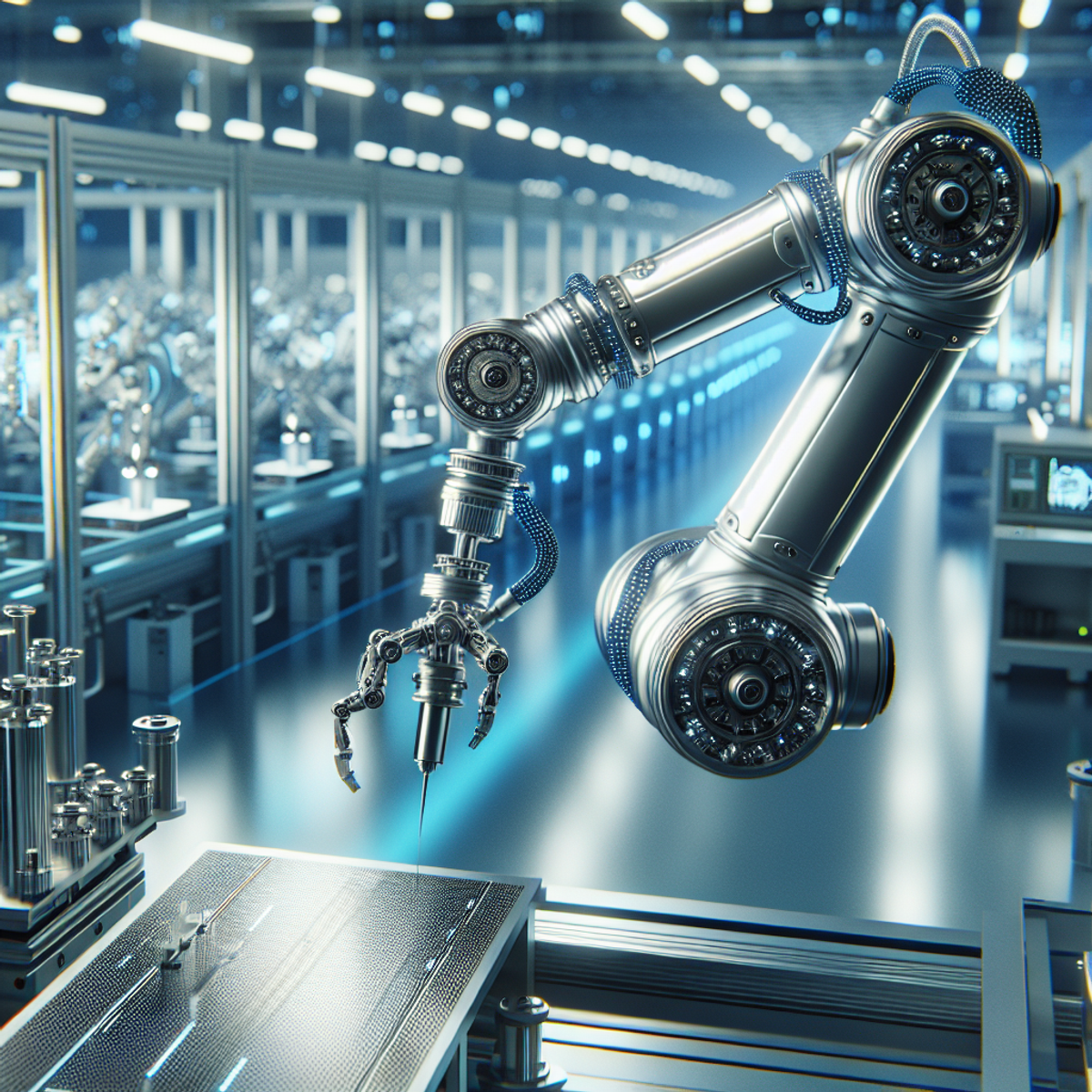
Robotic arms are widely used in various industries for manipulation tasks such as pick-and-place operations, assembly line tasks, and precise object manipulation. Python plays a crucial role in programming these robotic arms, enabling precise control and coordination of their multi-joint movements. Let's explore how Python is applied to solve practical challenges in different types of robotic arms.
Python Scripts for Robotic Arm Control
Python provides a flexible and powerful platform for controlling robotic arms. By writing code in Python, programmers can define the desired positions, velocities, and accelerations of each joint to achieve precise arm movements. This allows for seamless integration with other components of the robotic system.
Machine Learning Techniques for Enhanced Control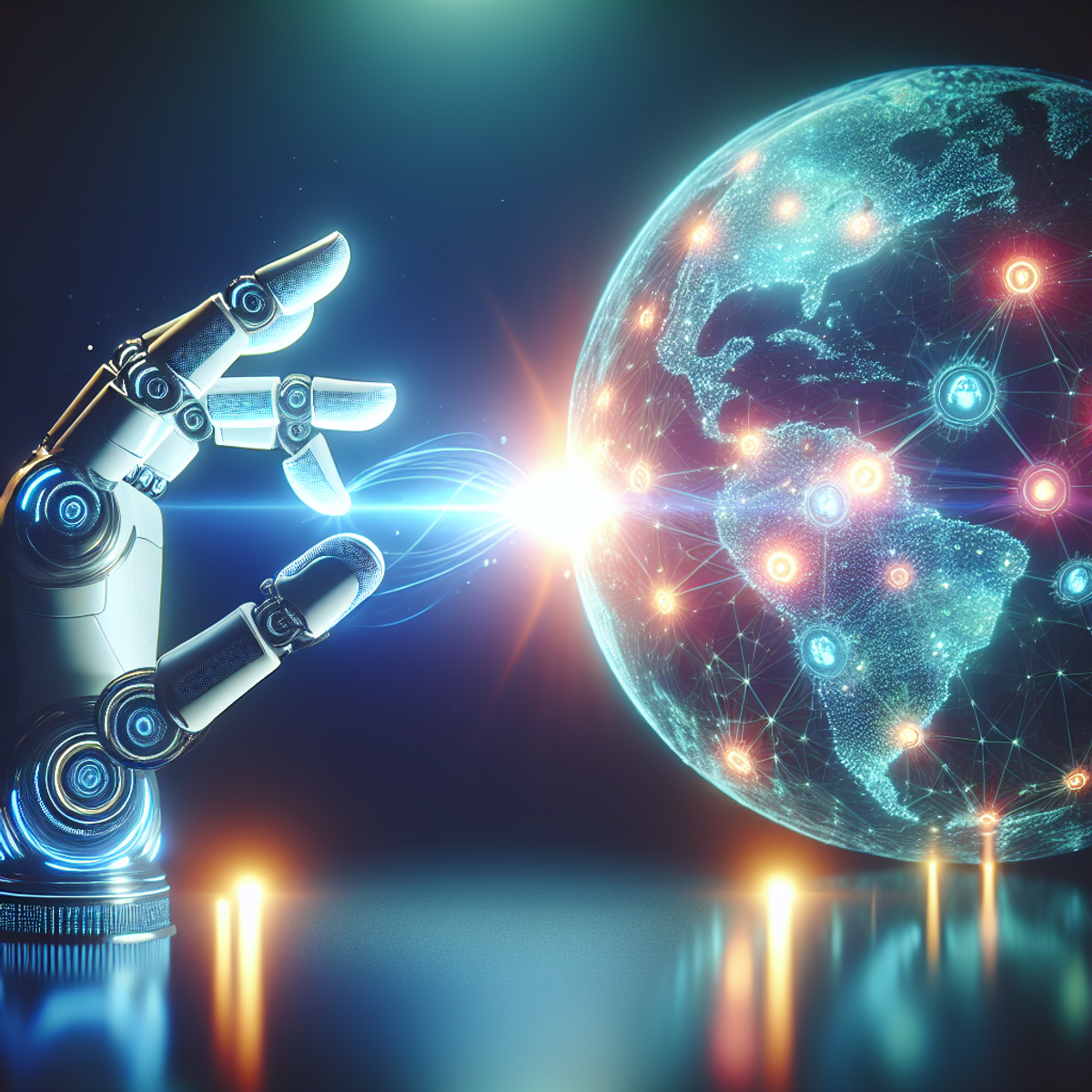
Machine learning techniques are increasingly being employed to enhance the control capabilities of robotic arms. By training models using real-world data, Python can be used to develop algorithms that improve the accuracy and efficiency of arm movements. For example, reinforcement learning algorithms can optimize the arm's trajectory to perform complex manipulation tasks.
Vision-Based Navigation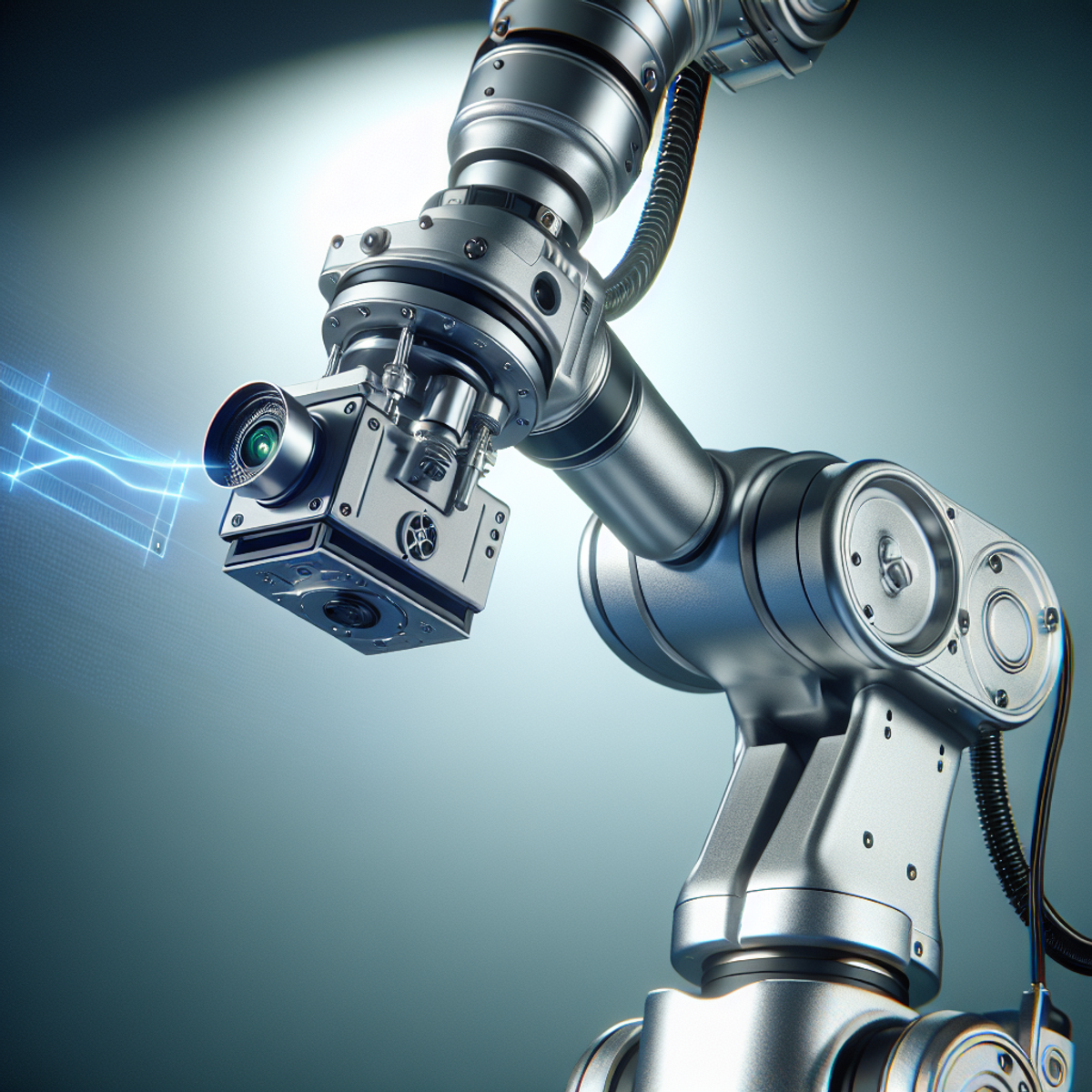
Python is also used in conjunction with computer vision techniques to enable vision-based navigation for robotic arms. By using cameras or depth sensors, the arm can perceive its environment and accurately locate objects for manipulation. Python libraries such as OpenCV provide tools for image processing and object detection, which can be integrated into the arm's control system.
Diverse examples demonstrate how Python is applied to solve practical challenges in different types of robots:
Industrial Robotic Arms
In manufacturing settings, Python is used to program industrial robotic arms for tasks such as assembling products or moving heavy objects. The high-level programming capabilities of Python make it easier to define complex trajectories and coordinate multiple arms working together.
Collaborative Robots (Cobots)
Collaborative robots are designed to work alongside humans in a safe and efficient manner. Python enables cobots to perform tasks that require interaction with humans, such as handing over tools or assisting in assembly operations. Through Python, cobots can adapt their movements and forces based on human input or sensor feedback.
Medical Robotic Arms
Python plays a vital role in programming medical robotic arms used in surgeries and rehabilitation. Surgeons can use Python to precisely control the movements of robotic surgical instruments, enhancing the accuracy and safety of procedures. In rehabilitation settings, Python-based control algorithms enable robotic exoskeletons to assist patients in regaining mobility.
Pythonic control of robotic arms opens up new possibilities for automation and efficiency across industries. By leveraging Python's flexibility, machine learning capabilities, and integration with computer vision techniques, programmers can develop sophisticated control systems for a wide range of manipulation tasks.
3. Machine Learning for Robot Perception and Decision Making with Python
The Role of Machine Learning in Robotics
Machine learning algorithms and libraries are instrumental in improving the abilities of robots through the use of Python:
- Enhancing Robot Perception: Machine learning techniques in Python can be employed to help robots better understand their surroundings.
- Enabling Complex Decision Making: By utilizing Python scripts and machine learning, robots can make more sophisticated choices.
How Python Supports Machine Learning in Robotics
Python is an ideal programming language for robotics due to its support for machine learning:
- Implementing Algorithms: Python provides a flexible environment for creating and running machine learning algorithms.
- Leveraging Libraries: With popular libraries like TensorFlow and scikit-learn, developers can easily access pre-existing machine learning models and tools.
Practical Examples of Python's Impact on Robotics
Here are some specific instances where Python has been used to address challenges in different types of robots:
- Roomba-like Vacuums: Applying machine learning algorithms in Python to enable Roomba-like vacuums to navigate autonomously and avoid obstacles.
- Vision-Based Navigation: Using Python scripts and machine learning to develop vision-based navigation systems that allow robots to make decisions based on real-time visual inputs.
- Robotic Arms: Implementing machine learning techniques in robotic arms to optimize tasks such as motion planning and object manipulation.
The Versatility of Python in Robotics
Python's ability to adapt makes it an excellent choice for implementing machine learning solutions across various robotic applications:
- Image Recognition: Enhancing robot perception through the use of image recognition algorithms.
- Autonomous Decision Making: Enabling robots to make decisions independently by analyzing environmental data.
By leveraging the power of machine learning through Python, robots can successfully navigate complex environments, accurately identify objects, and perform tasks that require advanced decision-making abilities.
4. Enabling Vision-based Navigation using Python and Computer Vision
Robotic navigation is crucial in allowing robots to move on their own and interact with their surroundings. One way to achieve this is through vision-based methods, where robots use cameras or sensors to see and understand their environment. Python, combined with computer vision techniques, is key in making vision-based navigation possible for different kinds of robots like Roomba-like vacuums and robotic arms.
Here are some important points on how Python and computer vision techniques work together to enable robots to navigate using visual information:
- Image Processing: Python has powerful libraries like OpenCV that can process images. This allows robots to analyze pictures or data from sensors using functions like image filtering, edge detection, and feature extraction. These functions are essential in getting useful information from images.
- Object Detection: With Python's computer vision libraries, robots can identify specific objects or landmarks in their environment. Object detection algorithms such as Haar cascades and deep learning-based models like YOLO (You Only Look Once) can be implemented in Python to recognize objects of interest. This enables robots to navigate towards or avoid specific objects while moving.
- Optical Flow: Optical flow algorithms estimate the motion of objects in a sequence of images. By calculating the optical flow using Python libraries like OpenCV, robots can perceive the movement of objects around them. This information is valuable for ensuring safe navigation and avoiding collisions.
- SLAM (Simultaneous Localization and Mapping): SLAM algorithms combine localization (determining the robot's position) and mapping (creating a map of the environment) simultaneously. Python frameworks like ROS (Robot Operating System) provide SLAM capabilities that use computer vision techniques to build maps of the robot's surroundings while keeping track of its position. This allows robots to navigate autonomously in previously unknown environments.
Python's flexibility and wide range of libraries make it a great choice for implementing vision-based navigation in robots. By using computer vision techniques, Python helps robots "see" their environment and make smart decisions based on what they see.
Examples of How Python Solves Real-world Problems in Different Robots
- Roomba-like Vacuums: Python scripts can be used to program Roomba-like vacuums for autonomous navigation. By combining computer vision techniques with Python, these robots can recognize obstacles, map the layout of a room, and efficiently navigate to clean the entire area.
- Robotic Arms: Python, along with computer vision algorithms, enables precise control of robotic arms for tasks such as order picking/packing. By analyzing visual inputs and using Python scripts for trajectory planning and object manipulation, robotic arms can perform complex tasks with high accuracy.
In summary, Python, combined with computer vision techniques, is essential in enabling vision-based navigation in robots. The integration of image processing, object detection, optical flow, and SLAM allows robots to navigate autonomously and interact effectively with their surroundings. Whether it's Roomba-like vacuums or robotic arms, Python provides the necessary tools and libraries to implement vision-based navigation solutions for various types of robots.
The Future of Python in Robotics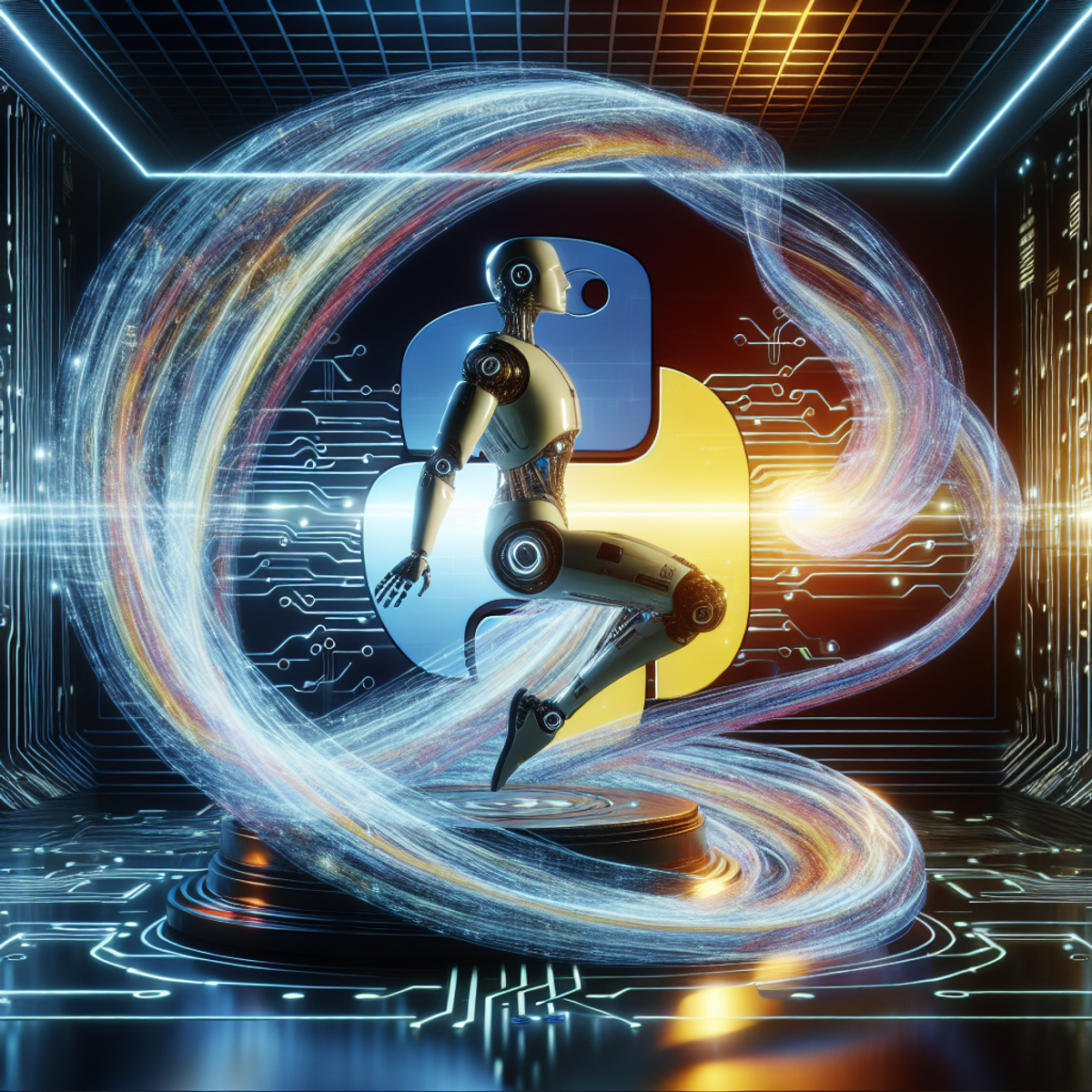
Python has established itself as a dominant programming language in the field of robotics, and its future looks promising. As technology continues to advance and the demand for robotics applications grows, Python's versatility and ease of use make it an ideal choice for programming robots. Here are some key points to consider for the future of Python in robotics:
- Expanding Robotics Applications: Python's flexibility allows it to be used in a wide range of robotics applications, from industrial automation to medical robotics to autonomous vehicles. As the field of robotics continues to evolve, Python will likely play a crucial role in developing innovative solutions.
- Integration with Machine Learning: Machine learning techniques are becoming increasingly important in robotics, enabling robots to learn and adapt to their environment. Python's rich ecosystem of machine learning libraries, such as TensorFlow and PyTorch, makes it an excellent choice for incorporating machine learning algorithms into robot systems.
- Collaboration with Hardware Manufacturers: Python's popularity and widespread adoption have caught the attention of hardware manufacturers. As a result, we can expect closer collaboration between Python developers and hardware manufacturers, leading to better integration between software and hardware components.
- Emerging Frameworks and Libraries: The Python community is constantly developing new frameworks and libraries specifically designed for robotics. These tools provide ready-to-use functionalities, making it easier for developers to build complex robotic systems without starting from scratch.
- Education and Research: Python's simplicity and readability make it an ideal programming language for educational purposes. Many universities and research institutions already use Python as the primary language for teaching robotics concepts and conducting research. This trend is likely to continue, ensuring a steady stream of talent well-versed in Python for robotics.
- Open-source Community Support: Python has a vibrant open-source community that actively contributes to the development of libraries, frameworks, and sample code for robotics applications. This collaborative environment fosters innovation and encourages knowledge sharing, making Python an even more attractive choice for robotics development.
In conclusion, Python's future in robotics looks bright. Its versatility, integration with machine learning, collaboration with hardware manufacturers, emergence of new frameworks and libraries, focus on education and research, and strong open-source community support all contribute to its continued success in the field of robotics. As technology progresses and robots become increasingly prevalent in our daily lives, Python will remain a key language for programming robots and driving innovation in robotics applications.
Conclusion
As you think about the many ways Python is used in programming robots, it's important to see the endless opportunities that are available. Python's role in robotics is constantly growing, providing countless chances for creativity and exploration.
Here are some key takeaways from this article:
- Python is a versatile and beginner-friendly programming language that is widely used in the field of robotics.
- Its simplicity and readability make it easy to understand and maintain complex robotic systems.
- Python offers a rich ecosystem of libraries and frameworks specifically designed for robotics development.
- It can be used for various tasks in robotics, such as perception, control, planning, and simulation.
- Python is extensively employed in both research and industry settings for building state-of-the-art robotic applications.
By understanding the potential of Python in robotics projects and keeping up with the latest advancements, you can actively contribute to its growth and stay ahead of the curve.
"Python serves as a powerful tool for realizing intricate robotic functionalities, paving the way for enhanced automation and efficiency across diverse domains."
FAQs (Frequently Asked Questions)
How does Python facilitate the gathering and manipulation of sensor data in robots?
Python provides powerful libraries and tools for collecting, processing, and manipulating sensor data in robots. Its simplicity and versatility make it an ideal language for handling the diverse types of data generated by robot sensors.
What is the role of Python in interacting with robot hardware at a low level?
Python plays a crucial role in interacting with robot hardware at a low level, such as motor control. It allows programmers to directly interface with hardware components to control and manipulate them as needed.
How is Python employed in computer vision algorithms to enable robots to recognize objects?
Python is used to implement complex computer vision algorithms that enable robots to identify and perceive objects in their environment. This involves processing visual data captured by cameras or other sensors to make sense of the surroundings.
In what way are Python algorithms used to plan optimal robot actions and execute them?
Python algorithms are utilized to plan and execute optimal actions for robots. This involves creating logic that allows robots to make decisions based on their environment and current objectives, ultimately leading to effective and efficient movement and task execution.
What are some popular Python-based tools for simulating and controlling robots?
Popular Python-based tools for simulating and controlling robots include Gazebo and ROS (Robot Operating System). These frameworks provide comprehensive capabilities for modeling, simulating, and controlling robotic systems using Python.
How do simulation robots provide a safe environment for practicing Python programming skills in robotics?
Simulation robots like TurtleBot 2 and Summit XL Robot offer a safe and accessible environment for practicing Python programming skills in robotics. They allow learners to experiment with real-world scenarios without the risk of damaging physical hardware or causing harm.
Comments
Post a Comment