Understanding Python's Lambda Functions
Understanding Python's Lambda Functions
Introduction
Lambda functions, also known as anonymous functions, are a powerful feature of Python that allows for concise coding solutions. They play a significant role in simplifying the syntax and structure of code, making it more expressive and easier to read.
In this article, we will delve into the world of lambda functions in Python, exploring their syntax, differences from regular functions, practical applications, and limitations. By the end of this article, you will have a comprehensive understanding of how to effectively use lambda functions in your Python projects. Let's get started by examining the syntax of a lambda function. A lambda function is defined using the keyword `lambda`, followed by a list of parameters, a colon, and an expression. The general format is:
```python
lambda parameters: expression
```
Unlike regular functions, lambdas can only contain a single expression, which is evaluated and returned as the result. This concise syntax makes them ideal for simple, one-line operations.
Now that we have an understanding of the basic structure of lambda functions, let's explore their differences from regular functions.
Understanding Lambda Functions in Python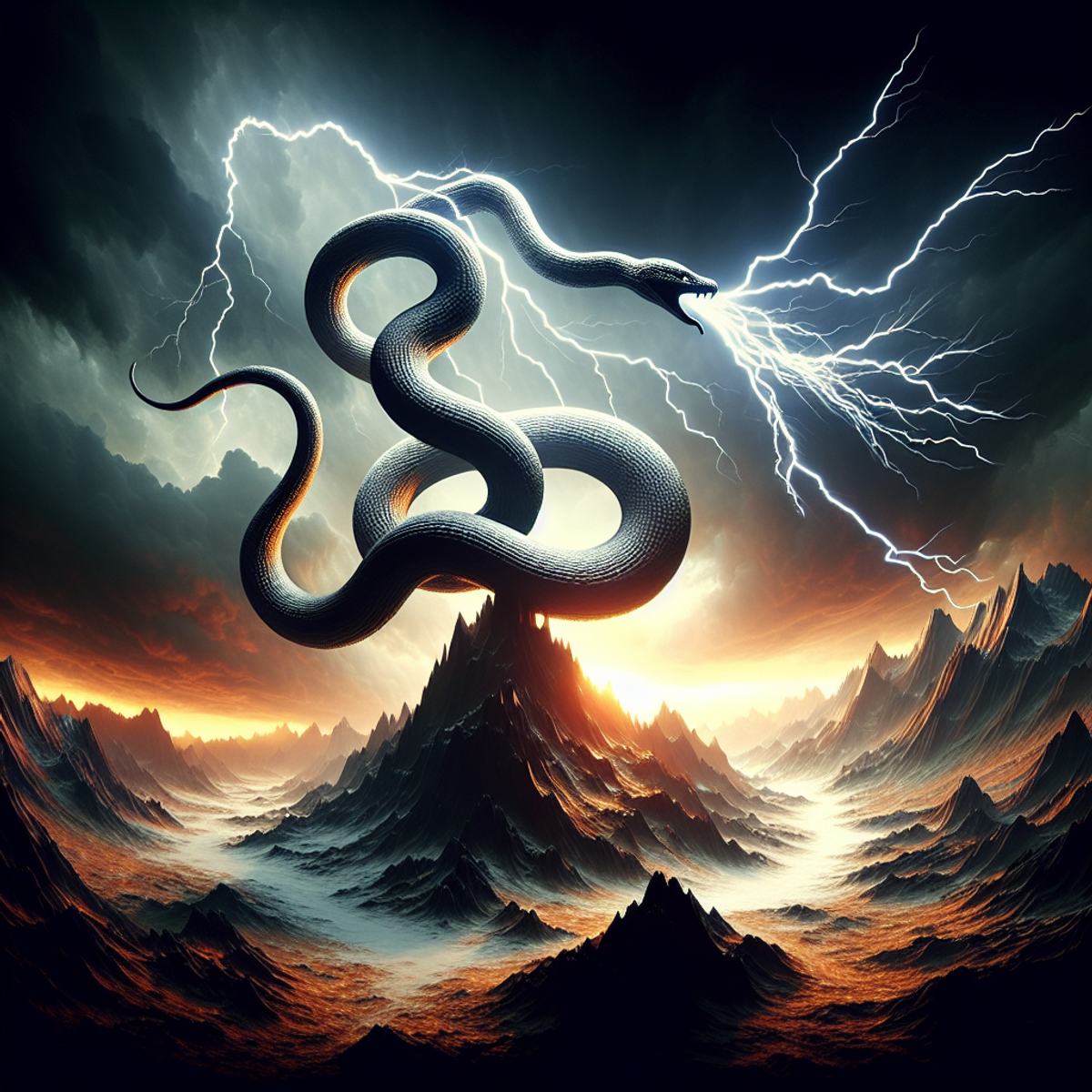
Lambda functions in Python are a powerful feature that allows you to create small, anonymous functions on the fly. These functions are commonly used when you need a quick and concise solution without the need for defining a named function.
Lambda functions are created using the lambda
keyword, followed by a list of arguments, a colon, and an expression. The result of the expression is automatically returned by the lambda function. Here's the basic syntax:
python lambda arguments: expression
Lambda functions can take any number of arguments, but they can only have one expression. This means that they are best suited for simple tasks that can be done in a single line.
Benefits of Lambda Functions
Lambda functions offer several benefits over regular named functions:
- Conciseness: Lambda functions allow you to write short and compact code by eliminating the need for defining separate named functions.
- Readability: Lambda functions can make your code more readable by keeping the logic close to where it is used, especially for simple operations.
- Flexibility: Since lambda functions are anonymous, they can be defined and used at runtime without cluttering your code with unnecessary function definitions.
These benefits have been discussed extensively in articles like this one and this Reddit thread, which delve into the advantages of lambda functions in Python from different perspectives. The conciseness of lambda functions can make code more readable, especially when performing simple operations. This feature, combined with their flexibility, allows for more efficient and streamlined coding practices. However, it's important to note that lambda functions should be used judiciously and primarily for small, single-line tasks. Using them for complex operations can lead to code that is difficult to understand and maintain. It's always a good idea to strike a balance between conciseness and clarity in your code.
Limitations of Lambda Functions
However, it's important to note that lambda functions also have some limitations compared to regular named functions:
- Single-line expressions: Lambda functions are limited to single-line expressions, which means they cannot contain multiple statements or complex logic.
- No statements or type annotations: Lambda functions can only contain expressions and cannot include statements like
return
oryield
. They also do not support type annotations.
These limitations have been discussed in detail in articles like this one, which provide a comprehensive understanding of the constraints posed by lambda functions.
Despite these limitations, lambda functions are still a powerful tool in Python for writing concise and expressive code. They excel in scenarios where you need a small function for a short period of time, such as condition checking, list comprehension, and sorting. Additional resources like this GeeksforGeeks article can further enhance your understanding of lambda functions and their various applications. They are particularly useful in functional programming paradigms, where functions are treated as first-class citizens and can be passed around as arguments or returned as values. By leveraging lambda functions, you can write more compact and readable code that focuses on the logic at hand rather than boilerplate code.
Understanding Lambda Functions in Python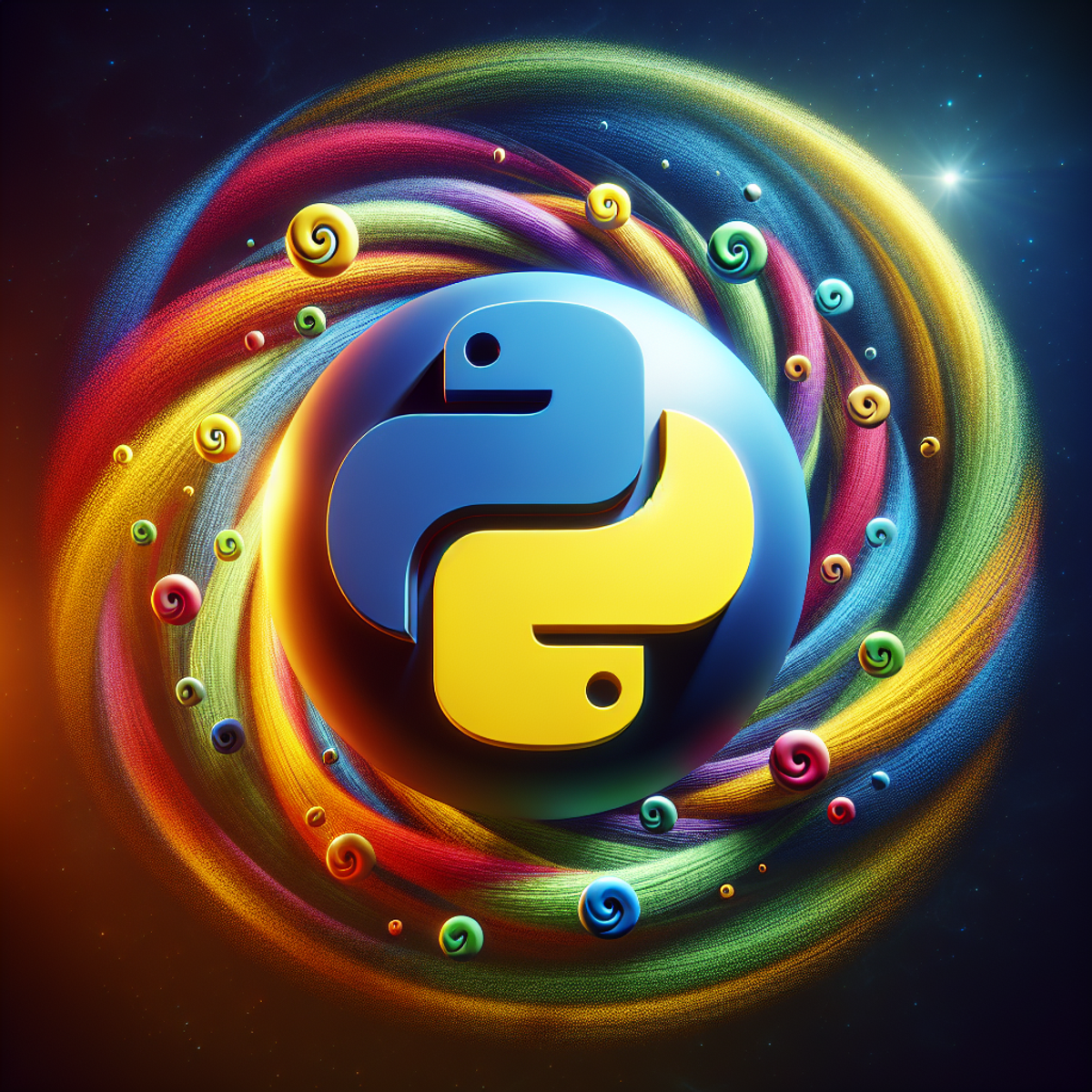
Lambda functions in Python, also known as anonymous functions, serve as powerful tools for concise and expressive coding. Let's dive into the essential aspects of understanding lambda functions to harness their potential effectively.
Definition and Purpose
Lambda functions are anonymous functions in Python, meaning they are defined without a name. They are primarily used for short periods within other functions, where creating a full-fledged function would be cumbersome or unnecessary. Their purpose lies in providing a more streamlined approach to defining and invoking functions, especially when the function's lifespan is short-lived.
Working as Anonymous Functions
The concept of anonymity is at the core of lambda functions. Unlike named functions, lambda functions do not require a formal definition using the def
keyword. This allows for on-the-fly creation and usage of simple functions without cluttering the code with extensive function definitions.
Syntax and Structure
The syntax for creating lambda functions involves the lambda
keyword followed by parameters (if any) and a single expression. The general structure is as follows: python lambda arguments: expression
For example, a lambda function that doubles its input can be represented as: python double = lambda x: x * 2
Creating and Using Lambda Functions
Creating a lambda function involves assigning it to a variable, similar to regular function definition. Once created, lambda functions can be used directly or passed as arguments to other functions. For instance: python result = (lambda x: x * 2)(5)
This line creates an anonymous function that doubles its input and immediately applies it to the value 5
, resulting in 10
.
Lambda Calculus
Lambda calculus is a formal system in mathematical logic and computer science that heavily influenced the development of lambda functions in programming languages. It serves as the theoretical foundation for functional programming constructs, including anonymous functions like those found in Python.
By understanding these fundamental aspects of lambda functions in Python, you can use their concise nature to improve your coding efficiency and keep your code clear.
Lambda Functions vs Regular Functions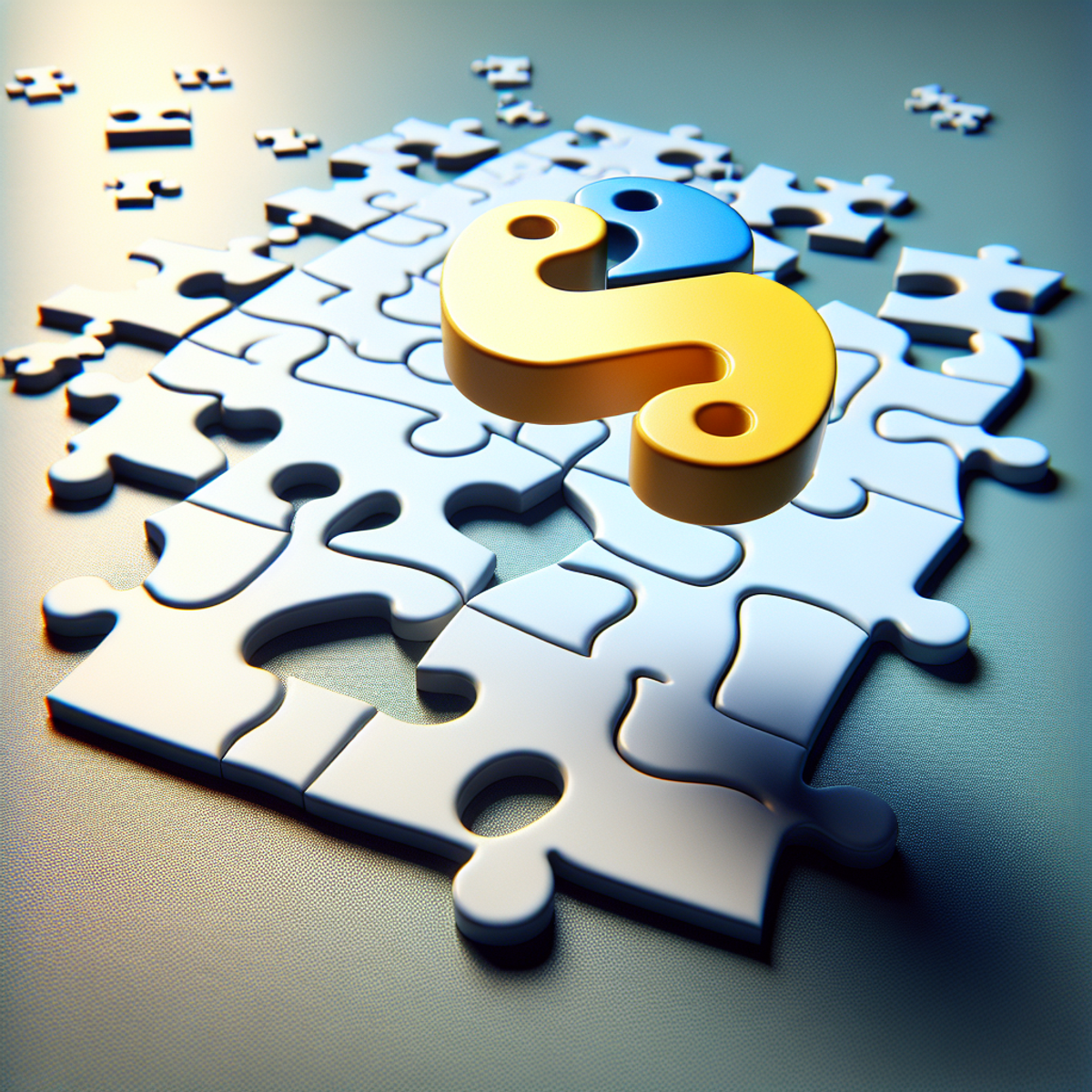
Comparison between Lambda Functions and Regular Functions
When comparing lambda functions (anonymous functions) and regular named functions in Python, there are several key differences to consider:
- Syntax:
- Lambda Functions: Defined using the
lambda
keyword followed by parameters and a single expression. - Regular Functions: Defined using the
def
keyword, a name, parameters, and a block of code. - Anonymous vs Named:
- Lambda Functions: Anonymous and do not have a name associated with them.
- Regular Functions: Have a specific name associated with them.
- Complexity:
- Lambda Functions: Suited for simple tasks and short expressions.
- Regular Functions: Can handle more complex logic with multiple statements and greater flexibility.
Advantages and Disadvantages of Using Lambda Functions over Regular Functions
Advantages:
- Conciseness: Lambda functions are often more concise than regular functions, allowing for quick implementation of simple tasks.
- Readability: For certain operations, lambda functions can make the code easier to read and understand by keeping the focus on the operation being performed.
Disadvantages:
- Limited Functionality: Due to their single-line nature, lambda functions are restricted in terms of complexity and cannot contain multiple expressions or statements. As mentioned in this Stack Overflow post, it is recommended to use
def
instead of assigning lambda expressions. - Lack of Naming: The lack of a specific name for lambda functions can make the code less readable when used for more complex operations.
Key Factors to Consider when Deciding Whether to Use Lambda Functions or Regular Functions
When deciding whether to use lambda functions or regular functions for a particular task, consider the following factors:
- Complexity: If the task requires complex logic with multiple statements, a regular named function may be more appropriate. As explained in this GeeksforGeeks article, regular functions offer greater flexibility and can handle more complex tasks.
- Readability: Evaluate whether using a lambda function enhances or detracts from the readability of the code in a given context.
- Reusability: Regular named functions can be reused in multiple parts of the codebase, whereas lambda functions are typically designed for single-use scenarios.
In summary, while lambda functions offer conciseness and can improve code readability for simple operations, they come with limitations in terms of complexity and reusability. Regular named functions provide greater flexibility for handling complex tasks and are reusable across different parts of a codebase. It is essential to consider these trade-offs when choosing between lambda functions and regular functions for specific programming tasks.
Higher-Order Functions and Lambda Functions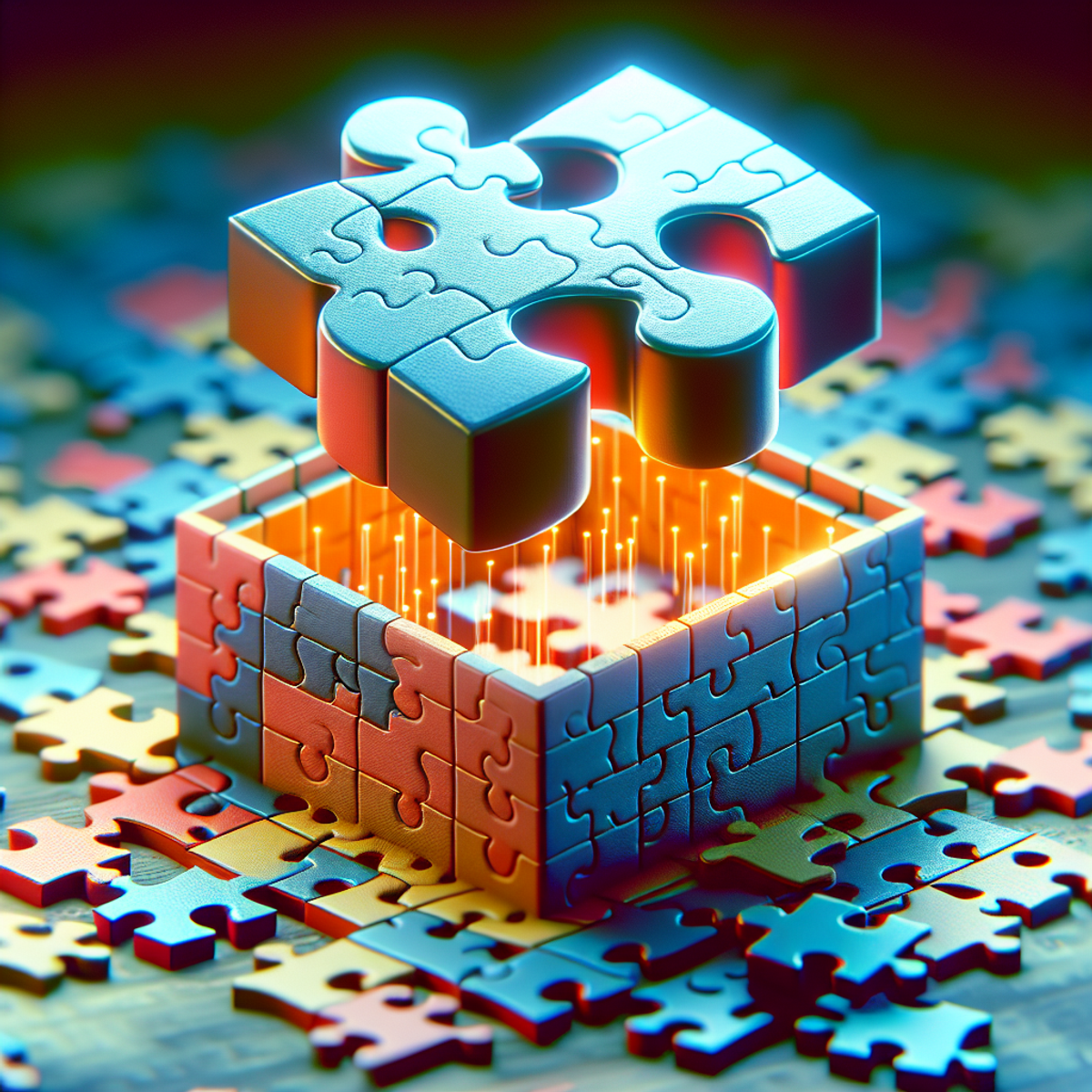
Higher-order functions in Python are essential in functional programming. They treat functions as first-class citizens, allowing them to be passed as arguments or returned as results. This flexibility opens up the use of lambda functions in various situations.
Understanding Higher-Order Functions
Higher-order functions are functions that work with other functions by either taking them as arguments or returning them as results. In Python, this concept enables the creation of more abstract and versatile code that can be applied in different scenarios.
The map(), filter(), and reduce() Functions
map(): The map()
function applies a specified function to each item in an iterable (such as a list) and returns a new iterator with the transformed results.
filter(): The filter()
function creates a new iterator containing only the elements from an iterable that satisfy a certain condition specified by a function.
reduce(): The reduce()
function, which was previously built-in to Python 2 but is now part of the functools
module in Python 3, performs a rolling computation on sequential pairs of values in an iterable, ultimately reducing them to a single value.
Using Lambda Functions with Higher-Order Functions
Lambda functions are often used alongside higher-order functions because their concise syntax makes them convenient for passing as arguments. This article on Python Functional Programming provides further insights into how these concepts can be leveraged together to write expressive and efficient code while maintaining readability and clarity.
Here are some examples illustrating their usage:
Example 1: Using map() with lambda
python numbers = [1, 2, 3, 4] doubled = list(map(lambda x: x * 2, numbers)) print(doubled) # Output: [2, 4, 6, 8]
Example 2: Using filter() with lambda
python numbers = [1, 2, 3, 4] evens = list(filter(lambda x: x % 2 == 0, numbers)) print(evens) # Output: [2, 4]
Example 3: Using reduce() with lambda (Python 2)
python from functools import reduce
numbers = [1, 2, 3, 4] result = reduce(lambda x, y: x + y, numbers) print(result) # Output: 10
These examples demonstrate how lambda functions seamlessly integrate with higher-order functions to provide concise solutions for data transformation and processing tasks.
Limitations of Lambda Functions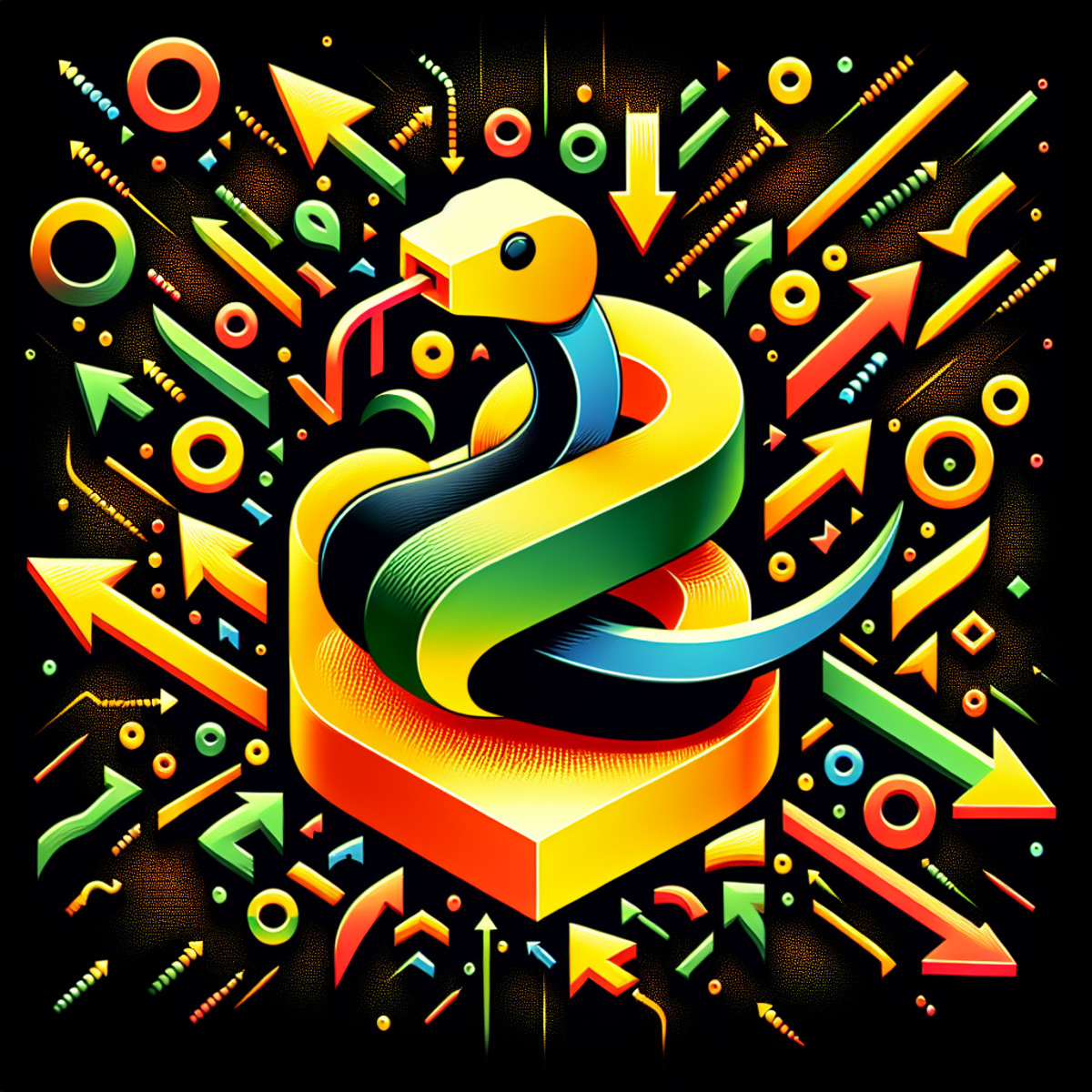
Lambda functions in Python are powerful tools for writing concise and expressive code. However, they also have certain limitations and restrictions that you should be aware of when using them. Here, we will explore the limitations of lambda functions in Python and discuss alternative approaches when these limitations become too restrictive for a task.
Limitation 1: Single-line execution
Lambda functions are limited to a single line of code. This means that you cannot use multiple statements or include complex logic within a lambda function. For example, if you need to perform multiple operations or include control flow statements like loops or if-else conditions, using a lambda function may not be feasible.
Limitation 2: No statements or type annotations
Lambda functions can only contain expressions, not statements. This means that you cannot include assignments, print statements, or any other statement that does not result in a value. Additionally, lambda functions do not support type annotations, which can be useful for documenting and enforcing the types of function arguments and return values.
Alternative Approaches
When you encounter these limitations while working with lambda functions, consider using the following alternative approaches:
- Named functions: When you need to perform more complex operations or include multiple lines of code, using named functions is often a better choice than lambda functions. Named functions allow you to write more expressive and readable code by giving meaningful names to your functions and breaking down complex logic into smaller, reusable units.
- Helper functions: If you find yourself needing more than a single line of code within a lambda function, consider creating helper functions instead. These helper functions can encapsulate the additional logic that cannot be expressed within a lambda function and provide more flexibility in terms of code organization and readability.
- Class-based solutions: In some cases, using classes instead of lambda functions can offer more flexibility and readability. By defining methods within a class, you can incorporate complex logic and state management, making your code more modular and maintainable. Classes also allow you to use type annotations effectively, providing clearer documentation and better error handling.
- Functional programming libraries: Python has various functional programming libraries, such as realpython and the official Python documentation, that provide higher-level abstractions for common tasks. These libraries often include predefined functions that can handle complex operations more efficiently than lambda functions alone. Leveraging these libraries can lead to cleaner and more concise code.
By selecting the appropriate approach based on the complexity and requirements of your task, you can ensure that your code remains concise, readable, and maintainable.
Practical Applications of Lambda Functions in Python
Lambda functions in Python have practical applications in various scenarios, providing a concise and expressive way to handle tasks such as condition checking, list comprehension, and working with built-in functions like filter()
, map()
, and reduce()
.
Condition Checking
Lambda functions are commonly used for condition checking, providing a quick and efficient way to evaluate conditions and return results based on the conditions met. List Comprehension
Another common use case for lambda functions is in list comprehension. Lambda
- For example, you can use a lambda function to check if a number is even:
python is_even = lambda x: x % 2 == 0 print(is_even(4)) # Output: True print(is_even(3)) # Output: False
List Comprehension
Lambda functions are often utilized in list comprehensions for performing operations on each element of a list and returning the results as a new list.
- Here's an example of using a lambda function within a list comprehension to square each element of a list:
python numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(lambda x: x ** 2, numbers)) print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Built-in Functions (filter(), map(), reduce())
Lambda functions are frequently used with built-in higher-order functions such as filter()
, map()
, and reduce()
to apply operations on iterables or sequences.
- For instance, you can use a lambda function with filter() to filter out even numbers from a list:
python numbers = [1, 2, 3, 4, 5] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4]
In summary, lambda functions provide an elegant and succinct way to handle condition checking, list comprehension, and work seamlessly with built-in functions like filter()
, map()
, and reduce()
in Python. Their versatility makes them valuable tools for writing clear and efficient code. Lambda functions are a powerful tool in Python that can be used in various scenarios. They are particularly useful for condition checking, as they allow you to define simple yet concise expressions. Additionally, lambda functions can be employed in list comprehensions to perform operations on each element of a list and generate a new list. Moreover, they seamlessly integrate with built-in functions such as filter(), map(), and reduce(), providing a compact and efficient approach to working with iterables or sequences. The versatility and flexibility of lambda functions make them an invaluable asset for any Python programmer seeking to write clean and efficient code.
Conclusion
Lambda functions in Python are powerful tools for writing concise and expressive code. They provide a way to define small, anonymous functions that can be used in various contexts. Throughout this article, we have explored the syntax, differences, practical applications, and limitations of lambda functions in Python. Let's summarize the key points:
- Lambda functions are small, anonymous functions that can take any number of arguments but can only have one expression. They are created using the
lambda
keyword. - Lambda functions can be used wherever function objects are required and are commonly used with higher-order functions like
map()
,filter()
, andreduce()
. - Compared to regular named functions, lambda functions offer the advantage of conciseness and can be defined inline without the need for separate function definitions.
- Lambda functions excel in scenarios where a short-lived function is needed, such as condition checking, list comprehension, sorting, and working with built-in functions.
- However, lambda functions have limitations. They are restricted to single-line expressions and cannot contain multiple statements or have type annotations.
- When the limitations of lambda functions become too restrictive for a task, alternative approaches such as using named functions should be considered.
In conclusion, lambda functions in Python provide a flexible and concise way to write code. Their simplicity makes them an ideal choice for situations where a small function is needed for a specific purpose. By using lambda functions in conjunction with higher-order functions like map()
, filter()
, and reduce()
, you can achieve more expressive and elegant solutions.
Remember that while lambda functions have their advantages, it's important to consider the trade-offs. If your code becomes complex or requires multiple statements, it may be better to use regular named functions for clarity and maintainability.
By understanding how lambda functions work and when to use them, you can leverage their power to create efficient and readable code. So go ahead, experiment with lambda functions in your Python projects, and discover the benefits they can bring to your programming journey.
Happy coding with lambda functions!
FAQs (Frequently Asked Questions)
What is the purpose of lambda functions in Python?
Lambda functions in Python serve the purpose of creating anonymous functions that can be used for concise coding solutions, especially when a function is needed for a short period and will not be reused.
How are lambda functions different from regular named functions in Python?
Lambda functions differ from regular named functions in Python by being anonymous, single-line expressions that can only contain one expression. Regular functions, on the other hand, have a name, multiple lines of code, and can have type annotations.
What are some practical applications of lambda functions in Python?
Lambda functions in Python are commonly used for condition checking, list comprehension, and with built-in higher-order functions like filter(), map(), and reduce(). They provide a concise way to write code for these types of tasks.
What are the limitations of lambda functions in Python?
The limitations of lambda functions in Python include the requirement for single-line execution, inability to contain multiple statements or have type annotations. When these limitations become too restrictive for a task, alternative approaches such as using named functions should be considered.
How do lambda functions work with higher-order functions like map(), filter(), and reduce() in Python?
Lambda functions are commonly used with higher-order functions like map(), filter(), and reduce() in Python to provide the function logic as an argument. This allows for concise and expressive code when working with lists or iterables.
What is the key takeaway regarding lambda functions in Python?
The key takeaway regarding lambda functions in Python is their significance in providing concise coding solutions. This article explores their syntax, differences from regular functions, practical applications, limitations, and provides examples to help understand their usage better.
Comments
Post a Comment