Dive into Python Classes and Objects: Understanding the Backbone of Python OOP
Dive into Python Classes and Objects:Understanding the Backbone of Python OOP
Dive into Python Classes and Objects:Understanding the Backbone of Python OOP
Python, a language admired for its simplicity and power, offers a robust approach to Object-Oriented Programming (OOP). At the heart of Python's OOP model are classes and objects, which enable developers to create well-organized, reusable, and scalable code. This article serves as a deep dive into understanding and utilizing classes and objects in Python.
Introduction
In the world of Python programming, understanding the concepts of classes and objects is fundamental to mastering object-oriented programming (OOP). In this blog post, we will explore the intricate details of classes, objects, inheritance, encapsulation, polymorphism, and best practices for utilizing these powerful features effectively. By the end of this comprehensive guide, you will have a solid grasp of Python's OOP capabilities and be well-equipped to leverage them in your projects. Let's begin our journey into the realm of Python classes and objects.
Defining a Class in Python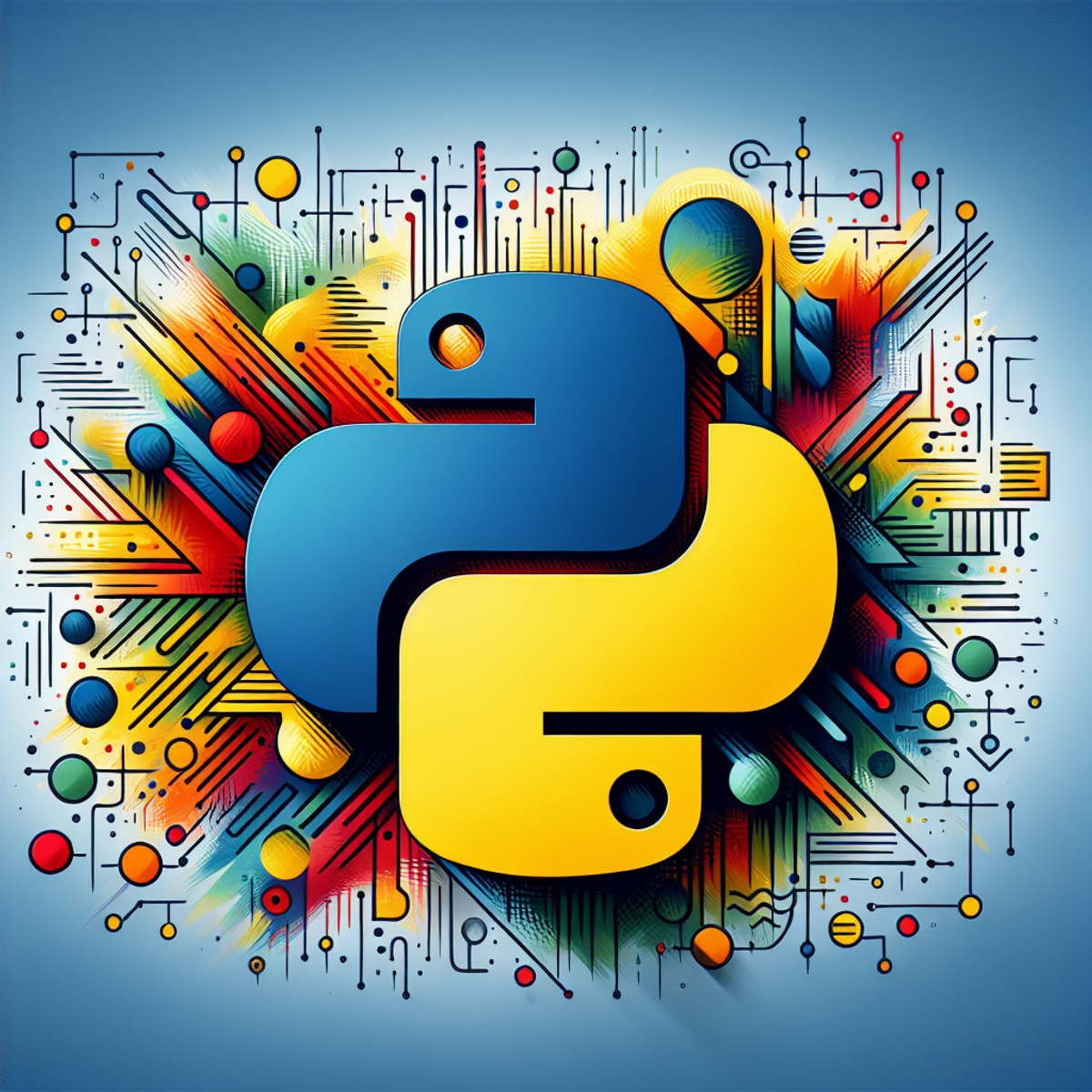
When working with Python classes and objects, it is essential to understand how to define a class. Defining a class in Python involves specifying the blueprint or template for creating objects of that class. Here are the key points to consider when defining a class in Python:
Use the class
keyword
To define a class, you start with the class
keyword followed by the name of the class. The convention is to use CamelCase for class names.
python class MyClass: # Class definition goes here pass
Define attributes
Attributes are variables associated with a class and its objects. They store data that represents the state of an object. You can define attributes within the class definition.
python class Person: def init(self, name, age): self.name = name self.age = age
Create methods
Methods are functions defined within a class that perform specific actions on objects of that class. You can define different types of methods like instance methods, class methods, and static methods.
python class Circle: def init(self, radius): self.radius = radius
def calculate_area(self): return 3.14 * self.radius * self.radius
Utilize constructors
The __init__
method serves as a constructor in Python classes. It is called automatically when an object is created from the class and is used for initializing object attributes.
python class Book: def init(self, title, author): self.title = title self.author = author
def display_info(self): print(f"Title: {self.title}\nAuthor: {self.author}")
Defining a class in Python allows you to create objects that encapsulate data and behavior. By defining attributes and methods within a class, you can specify the characteristics and actions of the objects created from that class. Understanding how to define a class is essential for harnessing the power of Python's object-oriented programming paradigm.
1. What are Python Classes and Objects?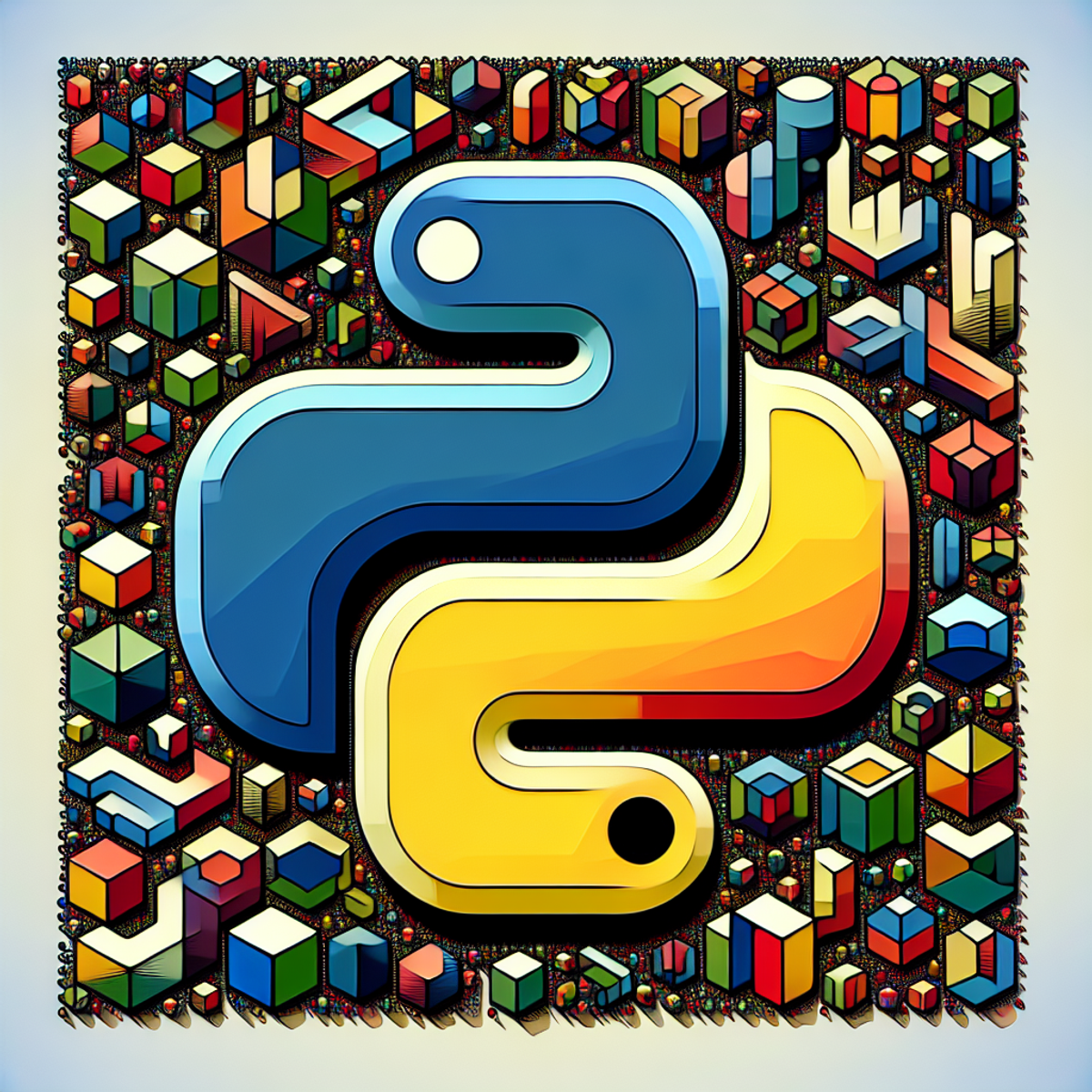
Python Classes and Objects are foundational concepts in object-oriented programming (OOP). They form the building blocks for creating modular and reusable code in Python, allowing developers to represent real-world entities and their interactions.
Definition of Python Classes and Objects
- A Python class is a user-defined blueprint or prototype for creating objects. It serves as a template for creating objects with similar attributes and methods.
- An object, on the other hand, is an instance of a class. When you create an object from a class, you are essentially creating a specific instance of that blueprint.
Role of Classes in Creating Objects
Classes play a crucial role in representing real-world entities in code. For example, you can have a "Car" class that defines the properties (attributes) and behaviors (methods) of a car. You can then create multiple car objects based on this class, each with its own unique characteristics.
Defining a Class in Python
In Python, you can define a class using the class
keyword followed by the class name and a colon. Inside the class block, you can define attributes to store data and methods to perform actions related to the objects created from the class.
Instantiating Objects from a Class
Once you have defined a class, you can create objects from it by calling the class name followed by parentheses. This process is known as instantiation, where you create an instance of the class.
Relationship Between Class and Objects
The relationship between a class and its objects is fundamental to OOP. A class acts as a blueprint that defines the structure and behavior of its objects. Each object created from the same class shares the same structure but can have different data.
Understanding Python classes and objects is essential for harnessing the full power of OOP in Python. It allows for code reusability, modularity, and better organization of complex systems.
By grasping these foundational concepts, you'll be equipped to build scalable and maintainable applications through effective use of classes and objects.
2. Attributes, Methods, and Constructors in Python Classes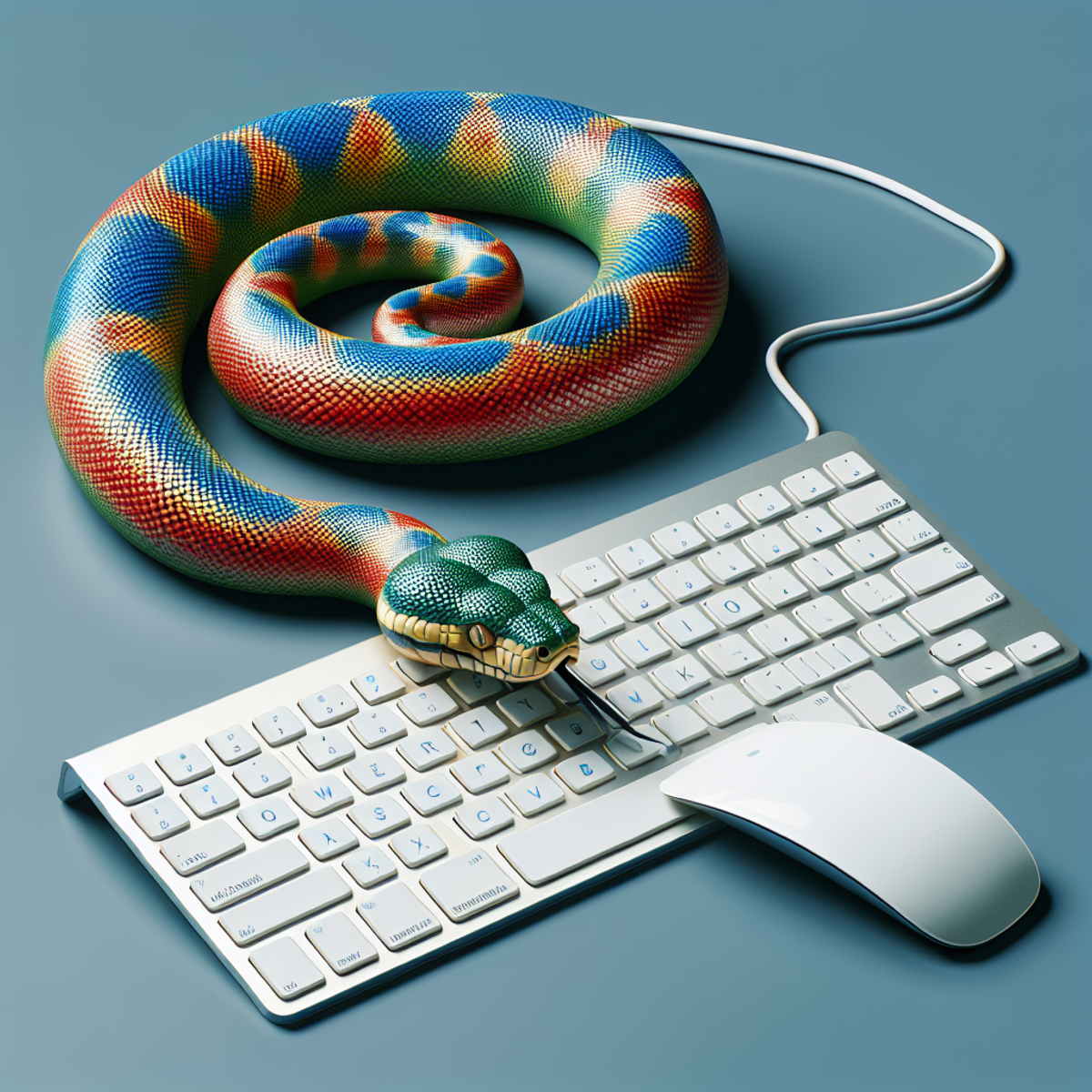
In Python classes, attributes and methods play a crucial role in defining the behavior and characteristics of objects. Let's dive into each of these concepts:
Defining Attributes in Classes
Attributes are variables that store data related to objects. They represent the state or properties of an object. In Python classes, you can define attributes using the following syntax:
python class MyClass: def init(self): self.attribute = value
- The
self
parameter refers to the instance of the class and allows you to access its attributes and methods. - Inside the
__init__
method (also known as the constructor), you can initialize the attributes with specific values.
For example, let's create a Person
class with name
and age
attributes:
python class Person: def init(self, name, age): self.name = name self.age = age
Now, you can create objects from this class and assign values to their attributes:
python person1 = Person("Alice", 25) person2 = Person("Bob", 30)
Using Methods to Manipulate Object Behavior
Methods are functions defined within a class that perform specific actions on objects. They enable you to manipulate the behavior of objects and interact with their attributes. There are two types of methods commonly used in Python classes:
1. Instance Methods
These methods operate on individual instances of a class and have access to instance-specific data through the self
parameter. To define an instance method, use the following syntax:
python class MyClass: def my_method(self, arguments): # Method body
Inside an instance method, you can access and modify object attributes using self.attribute
. Let's add an instance method called greet
to the Person
class:
python class Person: def init(self, name, age): self.name = name self.age = age
def greet(self): return f"Hello, my name is {self.name} and I am {self.age} years old."
Now, you can call the greet
method on a Person
object:
python person = Person("Alice", 25) print(person.greet()) # Output: Hello, my name is Alice and I am 25 years old.
2. Class Methods
These methods operate on the class itself rather than individual instances. They have access to the class attributes and can be defined using the @classmethod
decorator. To define a class method, use the following syntax:
python class MyClass: @classmethod def my_method(cls, arguments): # Method body
Inside a class method, you can access and modify class-level data using cls.attribute
. Let's add a class method called display_count
to the Person
class:
python class Person: count = 0
def __init__(self, name, age): self.name = name self.age = age Person.count += 1 @classmethod def display_count(cls): return f"There are {cls.count} people."
Now, you can call the display_count
method on the Person
class itself:
python person1 = Person("Alice", 25) person2 = Person("Bob", 30) print(Person.display_count()) # Output: There are 2 people.
The Role of Constructors in Object Creation
In Python classes, the __init__
method serves as a constructor and is executed automatically when an object is created from a class. It allows you to perform any necessary initialization for the object.
The __init__
method takes the self
parameter, along with any additional parameters you want to pass when creating an object. You can use these parameters to initialize object attributes.
For example, consider a Rectangle
class that calculates the area and perimeter of a rectangle based on its width and height:
python class Rectangle: def init(self, width, height): self.width = width self.height = height
def calculate_area(self): return self.width * self.height def calculate_perimeter(self): return 2 * (self.width + self.height)
Now, you can create a Rectangle
object and access its methods:
python rectangle = Rectangle(5, 3) print(rectangle.calculate_area()) # Output: 15 print(rectangle.calculate_perimeter()) # Output: 16
The constructor allows you to provide initial values for object attributes, making it easier to create and work with objects of the class.
In this section, we explored how attributes store data related to objects, methods manipulate object behavior, and constructors initialize object state during creation. Understanding these concepts is essential for building robust and flexible Python classes.
3. Class Inheritance: Extending and Modifying Existing Classes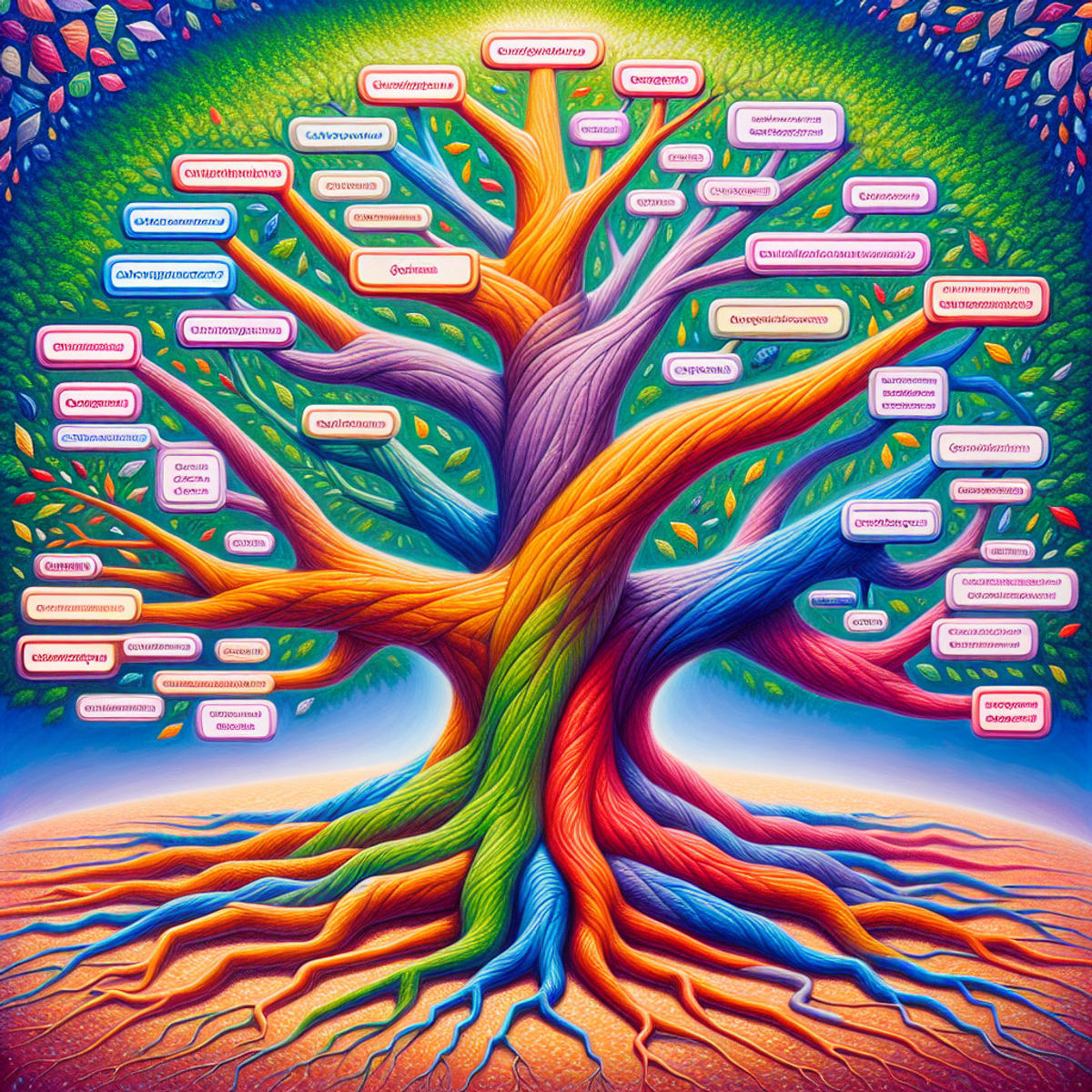
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows you to create new classes based on existing ones. It enables you to define a class hierarchy, where a derived class inherits attributes and methods from a base class. This mechanism promotes code reusability and enhances the organization and structure of your code.
Base Classes and Derived Classes
In inheritance hierarchies, the base class is also known as the superclass or parent class, while the derived class is also known as the subclass or child class. The derived class inherits all the attributes and methods defined in the base class, and it can add its own unique attributes and methods or override the ones inherited from the base class.
To create a derived class in Python, you use the class
keyword followed by the name of the derived class, and then specify the name of the base class inside parentheses. Here's an example:
python class Animal: def init(self, name): self.name = name
def speak(self): print("Animal speaks")
class Dog(Animal): def init(self, name): super().init(name)
def speak(self): print("Woof!")
dog = Dog("Buddy") dog.speak() # Output: Woof!
In this example, Animal
is the base class and Dog
is the derived class. The Dog
class inherits the __init__
method from Animal
using super().__init__(name)
to initialize the name
attribute. The speak
method in Dog
overrides the speak
method inherited from Animal
, allowing instances of Dog
to have their own unique behavior.
Benefits and Potential Pitfalls
Using inheritance offers several benefits in your code design:
- Code Reusability: Inheritance allows you to reuse code from existing classes, reducing duplication and promoting a modular and maintainable codebase.
- Polymorphism: Inheritance enables polymorphic behavior, where objects of different classes can be used interchangeably. This promotes flexibility and extensibility in your code.
However, inheritance also comes with potential pitfalls that should be considered:
- Inappropriate Hierarchies: Creating overly complex or deep class hierarchies can make the code harder to understand and maintain. It's important to strike a balance between reusability and simplicity.
- Tight Coupling: Inheritance creates a tight coupling between the base class and derived class. Changes in the base class can impact the behavior of derived classes, potentially introducing bugs or unintended consequences.
To mitigate these pitfalls, it's important to carefully design your class hierarchies and follow best practices for using inheritance effectively. For instance, you can consider using composition instead of inheritance in certain scenarios or explore discussions on why inheritance is generally viewed as a bad thing by some OOP proponents to gain more insights.
Summary
Class inheritance is a powerful mechanism in Python that allows you to extend and modify existing classes. It provides a way to create a hierarchy of classes, where derived classes inherit attributes and methods from base classes. This
4. Managing Data with Class Variables and Instance Variables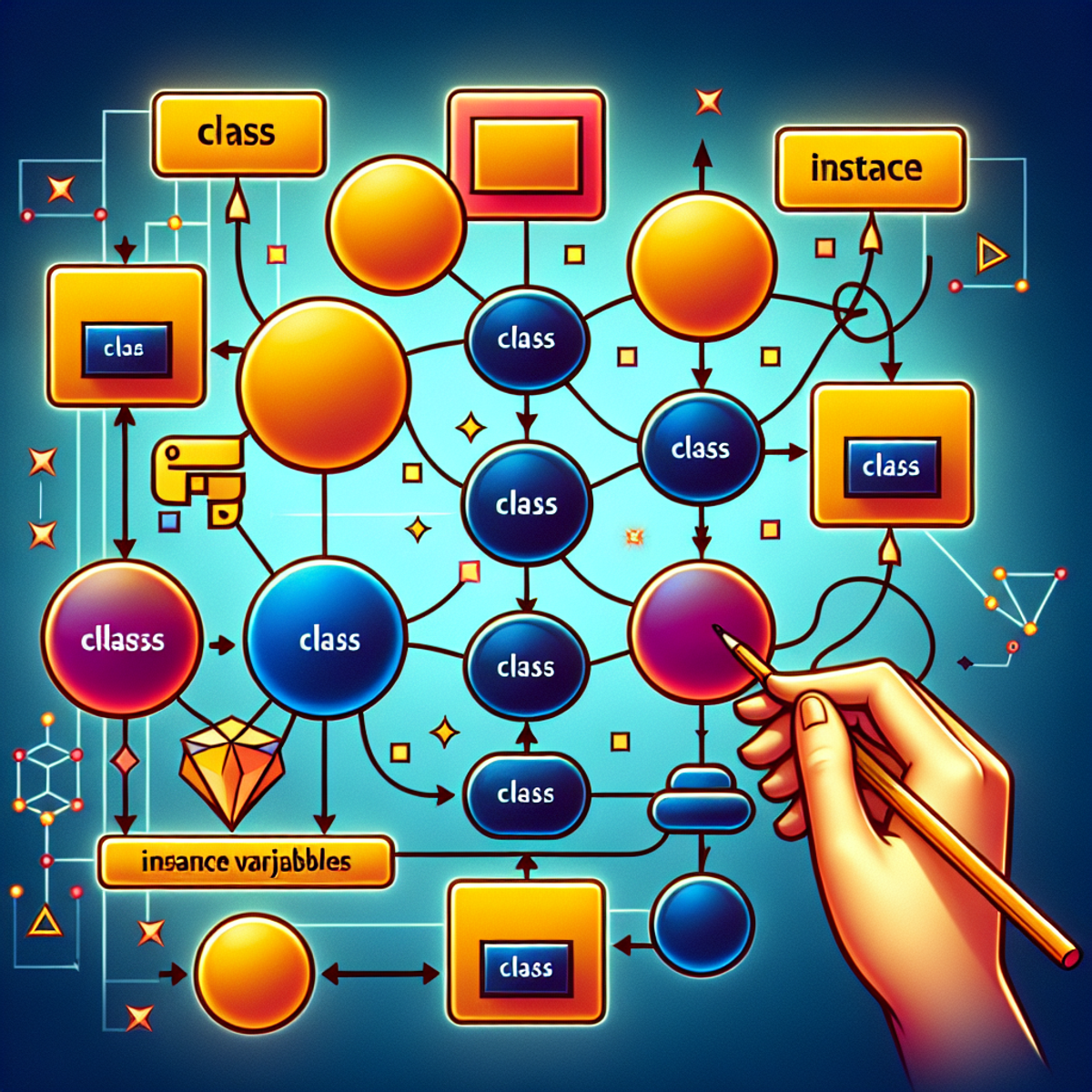
In Python classes, you can manage data using two types of variables: class variables and instance variables. Understanding the distinction between these two types is crucial for effectively organizing and manipulating data within your objects.
Class Variables
Class variables are defined within a class but outside of any methods. They are shared by all instances (objects) of the class. These variables belong to the class itself rather than to any specific instance, which means that when one instance modifies the value of a class variable, the change is reflected in all other instances as well.
To define a class variable, simply assign a value to it within the class definition. Here's an example:
python class Car: num_wheels = 4
In this example, num_wheels
is a class variable that holds the number of wheels for all cars. Every instance of the Car
class will have access to this variable and share its value.
You can access and modify class variables using either the class name or an instance of the class. However, it's generally recommended to use the class name to access class variables to avoid confusion.
python print(Car.num_wheels) # Output: 4
car1 = Car() print(car1.num_wheels) # Output: 4
Car.num_wheels = 6 print(car1.num_wheels) # Output: 6
Instance Variables
Unlike class variables, instance variables are unique to each object (instance) of a class. Each instance has its own set of instance variables, which store data specific to that instance.
Instance variables are typically defined within the special __init__
method, also known as the constructor. This method is called automatically when creating a new object from the class. Inside __init__
, you can assign initial values to the instance variables.
Here's an example of defining and using instance variables:
python class Car: def init(self, brand, color): self.brand = brand self.color = color
car1 = Car("Toyota", "blue") car2 = Car("Honda", "red")
print(car1.brand) # Output: Toyota print(car2.color) # Output: red
In this example, brand
and color
are instance variables specific to each car object. When we create car1
and car2
, the values passed as arguments to __init__
are assigned to the respective instance variables.
Instance variables can be accessed using dot notation (object.variable_name
) from both inside and outside the class.
Key Takeaways
- Class variables are shared by all instances of a class, while instance variables are unique to each object.
- Class variables are defined outside of any methods in the class, while instance variables are typically defined within the
__init__
method. - Modifying a class variable affects all instances, while modifying an instance variable only affects that specific instance.
- Class variables are accessed using the class name, while instance variables are accessed using the object name followed by dot notation (
object.variable_name
).
__init__
method.object.variable_name
).Understanding the distinction between class variables and instance variables allows you to effectively manage data within your Python objects. By leveraging these two types of variables, you can organize and manipulate data according to your specific requirements.
5. Encapsulation and Access Modifiers in Python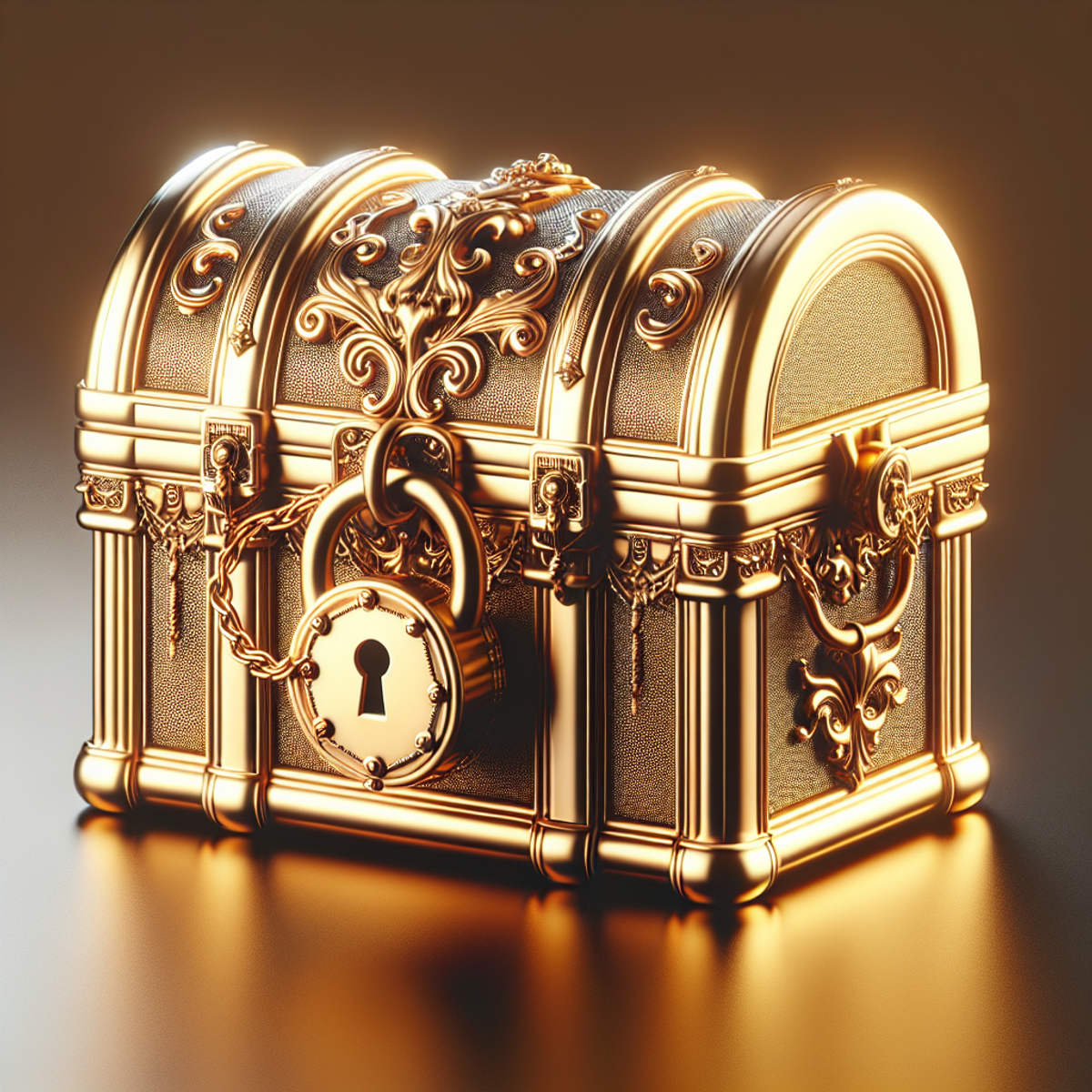
Encapsulation is one of the fundamental principles of object-oriented programming (OOP) that helps in organizing and structuring code. It refers to the bundling of data and methods within a class, allowing for better control over access to the internal workings of an object. In Python, encapsulation is achieved through the use of access modifiers like public, private, and protected.
Exploring Encapsulation
Encapsulation provides several benefits, including:
- Data Hiding: Encapsulation allows us to hide the internal data of an object from external access. This means that the implementation details are hidden and cannot be modified or accessed directly by other parts of the program. Instead, interactions with the object are performed through well-defined methods.
- Code Organization: By grouping related data and methods together within a class, encapsulation helps in organizing code and making it more modular. This improves readability, maintainability, and reusability.
- Flexibility: Encapsulation allows for flexibility in modifying the implementation details of a class without affecting other parts of the program that use the class. This is because external code interacts with the object only through its public interface.
Access Modifiers in Python
Access modifiers in Python are used to define the visibility and accessibility of class members (attributes and methods). They help in enforcing encapsulation by controlling how these members can be accessed by other classes or objects.
Python provides three levels of access modifiers:
- Public: Public members are accessible from anywhere within the program. By default, all members in a class are considered public unless specified otherwise.
- Private: Private members are intended to be accessed only within the same class where they are defined. They cannot be accessed directly from outside the class. In Python, we can define private members by prefixing them with double underscores (
__
). - Protected: Protected members are similar to private members, but they can also be accessed within derived classes. In Python, we can define protected members by prefixing them with a single underscore (
_
).
Example: Encapsulation and Access Modifiers in Python
Let's consider an example to understand how encapsulation and access modifiers work in Python:
python class Car: def init(self, make, model): self.__make = make # private attribute self._model = model # protected attribute
def start_engine(self): self.__turn_on_ignition() # private method print("Engine started") def __turn_on_ignition(self): print("Ignition turned on")
my_car = Car("Toyota", "Camry") print(my_car._model) # accessing protected attribute my_car.start_engine() # invoking public method
In this example, we have a Car
class with two attributes, __make
(private) and _model
(protected). The start_engine()
method is a public method that can be accessed from outside the class. However, it internally calls the private method __turn_on_ignition()
, which cannot be accessed directly from outside the class.
We can observe the following:
- Attempting to access
__make
directly will result in an error because it is a private attribute. This ensures that the internal implementation details of the class are hidden. - Accessing
_model
directly is allowed because it is a protected attribute. However, it is still recommended to access protected attributes through methods for better encapsulation. - Invoking the
start_engine()
method will successfully start the engine by calling the private method__turn_on_ignition()
. This demonstrates how encapsulation allows us to control access to internal functionality.
By using access modifiers to define the visibility of class members, we can enforce encapsulation and ensure that the internal implementation details of a class are not exposed to the outside world.
Encapsulation and access modifiers play a crucial role in designing clean and maintainable code. They help in creating well-defined boundaries between different parts of a program, improving code organization, and making it easier to understand and maintain.
6. Polymorphism: Writing Flexible and Reusable Code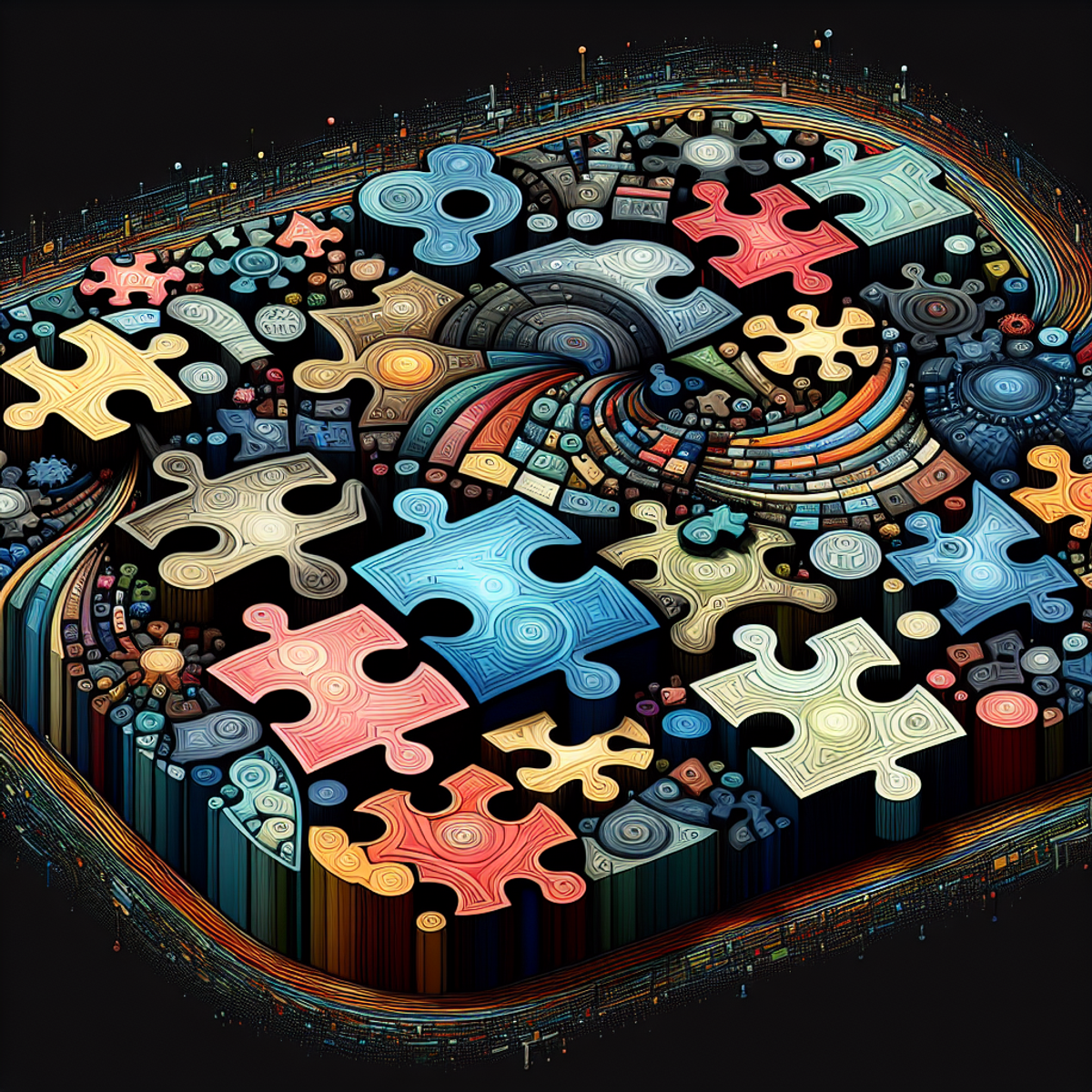
Polymorphism is a key feature of object-oriented programming (OOP) that allows objects of different classes to be used interchangeably. It enables you to write flexible and reusable code by treating objects from different classes as if they were of the same class.
Method Overriding
One way to achieve polymorphic behavior in Python is through method overriding. Method overriding occurs when a derived class provides a different implementation for a method that is already defined in its base class. This allows objects of both the base class and the derived class to be used interchangeably.
Consider the following example:
python class Animal: def make_sound(self): print("The animal makes a sound.")
class Dog(Animal): def make_sound(self): print("The dog barks.")
class Cat(Animal): def make_sound(self): print("The cat meows.")
animals = [Animal(), Dog(), Cat()]
for animal in animals: animal.make_sound()
In this example, we have an Animal
base class and two derived classes, Dog
and Cat
. Each class has its own implementation of the make_sound()
method. When we create a list of different types of animals and iterate over them, calling the make_sound()
method on each object, we get different sounds depending on the actual type of the object.
Output:
The animal makes a sound. The dog barks. The cat meows.
By overriding the make_sound()
method in the derived classes, we can ensure that each object produces the appropriate sound based on its specific behavior.
Method Overloading
Another way to achieve polymorphism is through method overloading. Method overloading allows multiple methods with the same name but different parameters to exist within a single class. The appropriate method is selected based on the number and types of arguments provided when the method is called.
However, unlike some other programming languages, Python does not natively support method overloading. In Python, you can achieve similar functionality by using default values for function parameters or by using variable-length argument lists.
Consider the following example:
python class Calculator: def add(self, a, b): return a + b
def add(self, a, b, c): return a + b + c
calculator = Calculator() print(calculator.add(2, 3)) # Output: 5 print(calculator.add(2, 3, 4)) # Output: 9
In this example, we have a Calculator
class with two add()
methods. The first method takes two arguments and returns their sum. The second method takes three arguments and returns their sum. Depending on the number of arguments provided when calling the add()
method, Python will automatically select the appropriate method to execute.
While Python does not have built-in support for strict method overloading like some other languages, you can achieve similar functionality by leveraging default values or variable-length argument lists.
Summary
Polymorphism is a powerful feature in OOP that allows objects of different classes to be treated as if they were of the same class. Method overriding enables you to provide different implementations of methods in derived classes, while method overloading allows you to
7. Best Practices for Using Classes and Objects in Python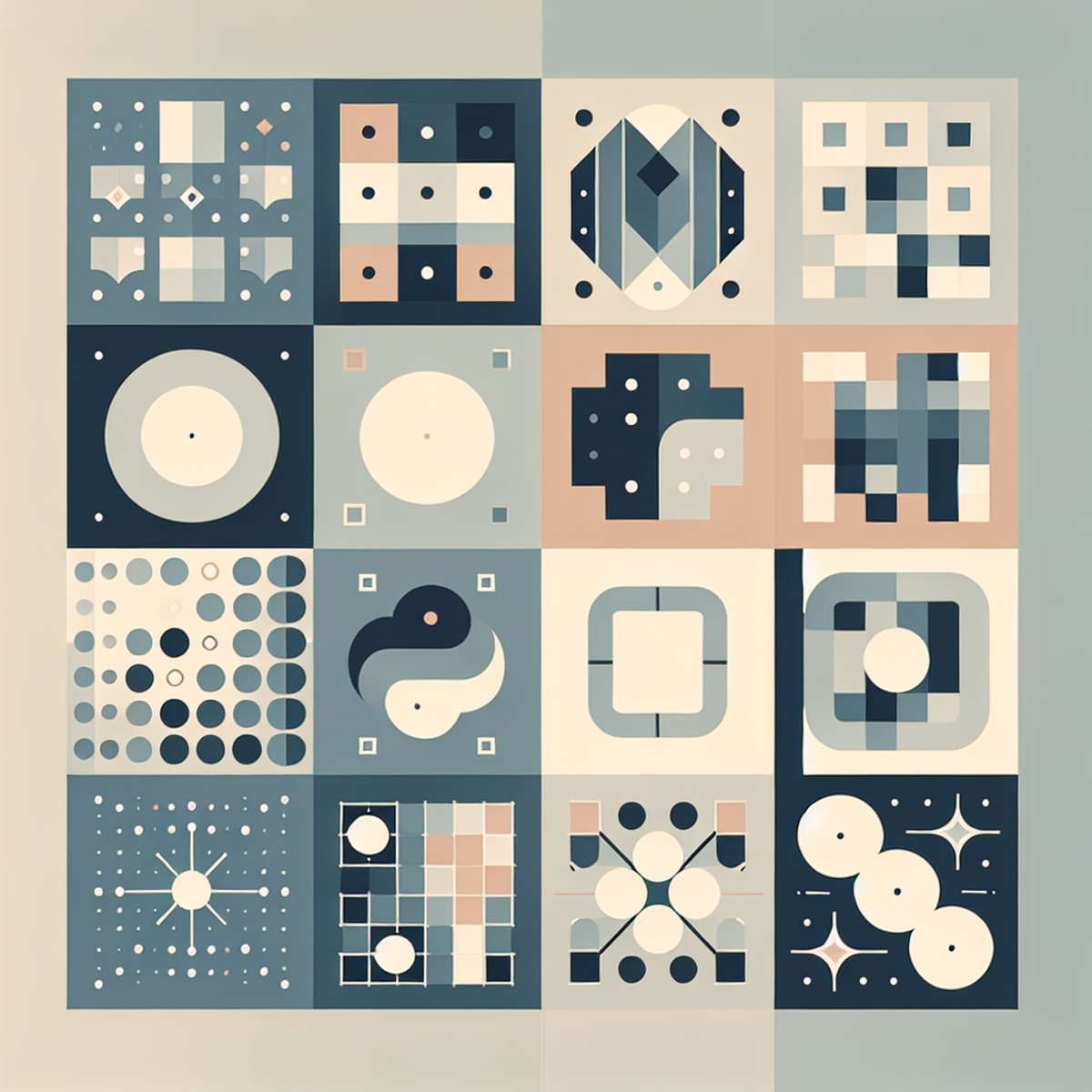
When working with classes and objects in Python, it's important to follow best practices to ensure clean and effective code. Here are some guidelines to keep in mind:
1. Use Descriptive Class Names
Choose meaningful and descriptive names for your classes that accurately represent the objects they are intended to create. This will make your code more readable and maintainable.
2. Follow the Single Responsibility Principle
Each class should have a single responsibility or purpose. This promotes modular and reusable code, making it easier to understand and maintain. If a class becomes too large or complex, consider refactoring it into smaller, more focused classes.
3. Encapsulate Data with Private Attributes
Python does not enforce strict data hiding, but it relies on naming conventions to indicate the intended visibility of attributes. Prefixing an attribute name with an underscore (_
) indicates that it is intended to be private and should not be accessed directly from outside the class.
4. Favor Composition Over Inheritance
While inheritance can be useful in certain scenarios, such as when creating a specialized version of an existing class, it can also lead to complex inheritance hierarchies and tight coupling between classes. In general, favor composition (combining simple classes) over inheritance (creating complex class hierarchies) to achieve more flexible and maintainable code.
5. Keep Methods Simple and Cohesive
Methods should have a clear purpose and perform a single task. Avoid creating methods that are too long or have multiple responsibilities. This promotes code reusability and makes it easier to understand and test individual methods.
6. Document Your Code
Include clear and concise comments within your classes to explain their purpose, document any assumptions or constraints, and provide examples of how to use them. This will help other developers (including yourself) understand your code and make it easier to maintain and update in the future.
7. Use Class Variables Wisely
Class variables are shared among all instances of a class. While they can be useful for storing data that is common to all instances, be cautious when modifying them. Changes made to a class variable will affect all instances of the class, potentially leading to unexpected behavior. Consider using instance variables instead when you need unique data per instance.
8. Test Your Classes
Writing tests for your classes is essential to ensure their correctness and functionality. Use unit testing frameworks like unittest
or pytest
to create test cases that cover different scenarios and edge cases. Regularly running your tests will help catch bugs early and give you confidence in the reliability of your code.
9. Follow PEP 8 Guidelines
Adhere to the Python Enhancement Proposal (PEP) 8 guidelines for code style, including indentation, naming conventions, and other formatting recommendations. Consistent code style improves readability and makes it easier for others to collaborate on your code.
10. Continuously Refactor and Improve
As you gain experience with classes and objects in Python, continuously look for opportunities to refactor and improve your code. This can involve simplifying complex logic, removing redundant code, or optimizing performance. Regularly reviewing and improving your codebase will result in cleaner, more efficient code over time.
By following these best practices, you'll be able to write clean, maintainable, and effective class-based code in Python. Remember that practice makes perfect, so keep coding and exploring advanced topics in object-oriented programming to further enhance your skills.
The Power of Inheritance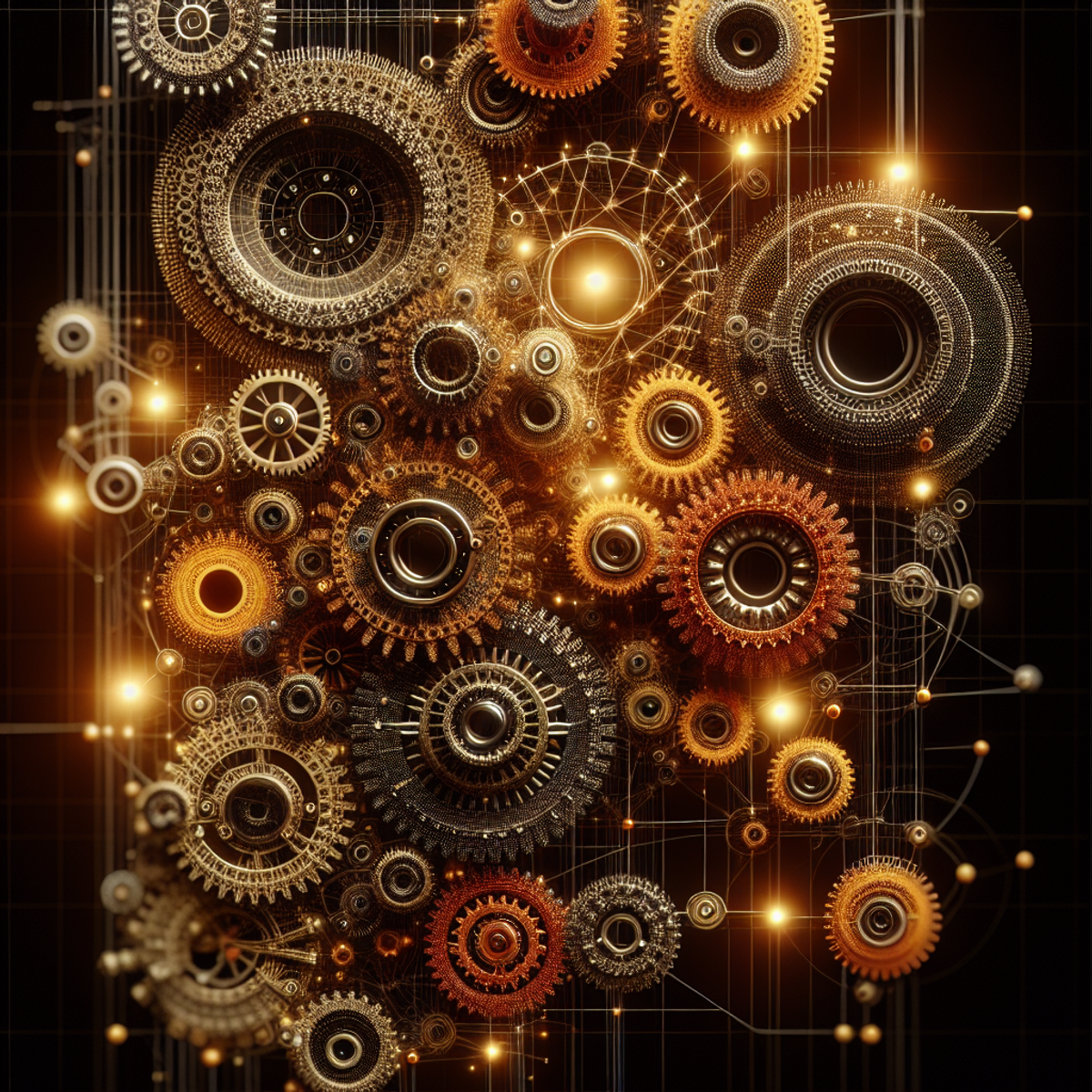
Inheritance is a fundamental concept in object-oriented programming that allows you to create new classes based on existing ones. It enables code reuse and promotes modularity by allowing you to define common attributes and methods in a base class and then extend or modify them in derived classes. In Python, inheritance plays a significant role in building robust and flexible code.
Here are some key points to understand about the power of inheritance in Python:
- Extending and Modifying Existing Classes: Inheritance allows you to create new classes (derived classes) that inherit attributes and methods from an existing class (base class). By doing so, you can add new functionality or modify existing behavior without altering the original class. This article on inheritance provides more insights.
- Base Classes and Derived Classes: In an inheritance hierarchy, the base class is the class being inherited from, while the derived class is the class that inherits from the base class. The derived class can access all the public attributes and methods of the base class and can override them if needed.
- Code Reusability: Inheritance promotes code reusability by allowing you to define common attributes and methods in a base class. Derived classes can then inherit these attributes and methods, reducing redundant code and making your codebase more maintainable. This resource on inheritance composition further highlights the benefits of this concept.
- Specialization: Derived classes can specialize or refine the behavior of the base class by adding new attributes or methods or overriding existing ones. This allows you to create more specific classes that inherit general behavior from a base class but also have their own unique characteristics.
- Inheritance Hierarchies: With inheritance, you can create complex hierarchies of classes where each derived class inherits from a common base class or even multiple base classes. This enables you to organize your code into logical categories and establish relationships between different classes. You can learn more about this topic in this Python inheritance article.
- Super() Function: The
super()
function provides a convenient way to call a method from the base class within the derived class. It allows you to access and utilize the functionality of the base class while adding additional behavior in the derived class.
Inheritance is a powerful tool in Python that allows you to build modular, reusable, and extensible code. Understanding how to leverage inheritance effectively can greatly enhance your programming skills and enable you to create more sophisticated applications.
Conclusion
Python Classes and Objects play a crucial role in mastering Object-Oriented Programming (OOP) in Python. Understanding these concepts is essential for anyone aiming to become proficient in Python development.
By diving deeper into Python OOP and gaining a comprehensive grasp of classes and objects, you are setting yourself up for success in building robust and scalable applications.
Keep the momentum going! Continue your learning journey by:
- Engaging in hands-on projects
- Exploring advanced topics in OOP
Embrace the challenges and opportunities that arise, as they will further solidify your expertise in Python development.
Remember, the journey of mastering Python OOP is an ongoing process. Stay curious, keep coding, and let your passion for learning drive you towards greater proficiency and innovation.
FAQs (Frequently Asked Questions)
What are Python Classes and Objects?
Python Classes and Objects refer to the user-defined blueprint for creating objects in Python. A class defines the properties and behaviors of objects, while an object is an instance of a class. In the context of object-oriented programming, classes play a crucial role in creating objects and representing real-world entities.
What is the role of classes in creating objects?
Classes serve as templates for creating objects with specific attributes and methods. They encapsulate the data and functionality related to objects, allowing for reusability and modularity in code.
How do you define a class in Python and instantiate objects from it?
To define a class in Python, you use the 'class' keyword followed by the class name and a colon. Within the class, you can define attributes (data) and methods (functions). To instantiate objects from a class, you simply call the class name as if it were a function, which creates a new instance of that class.
What is the purpose of the init method in Python classes?
The special init method, also known as the constructor, is used to initialize the attributes of an object when it is created. It allows for setting initial values for object properties and performing any necessary setup.
What is class inheritance in Python?
Class inheritance is a mechanism that allows a new class to be created based on an existing class. The new class inherits attributes and methods from the existing class, promoting code reuse and extending functionality.
What are class variables and instance variables in Python?
Class variables are shared by all instances of a class, while instance variables are unique to each object. Class variables are defined within the class but outside of any methods, whereas instance variables are defined within methods and are specific to each object.
What is encapsulation in OOP?
Encapsulation is the concept of bundling data (attributes) and methods (functions) that operate on that data within a single unit, i.e., a class. Access modifiers like public, private, and protected are used to control access to the members of a class, thereby achieving encapsulation.
What is polymorphism in Python?
Polymorphism allows objects of different classes to be treated as objects of a common superclass. This promotes flexibility and reusability by enabling code to work with objects of multiple types through a uniform interface. Method overriding and method overloading are examples of achieving polymorphic behavior in Python.
What are some best practices for using classes and objects in Python?
Some best practices for using classes and objects in Python include following naming conventions (e.g., using CamelCase for class names), keeping classes focused on a single purpose or responsibility (the Single Responsibility Principle), favoring composition over inheritance where possible, documenting classes and their members effectively, etc.
Comments
Post a Comment