Python Regular Expressions: A Practical Guide
- Get link
- X
- Other Apps
Python Regular Expressions: A Practical Guide
Regular expressions (regex) are an incredibly powerful tool for manipulating text. Python, with its emphasis on readability and efficiency, offers excellent support for regular expressions through the re
module. In this practical guide, we'll explore how to use regular expressions in Python to match, search, and manipulate strings.
Introduction
The article "Python Regular Expressions: A Practical Guide" aims to provide a comprehensive and practical approach to using regular expressions in Python. Whether you are a beginner or an intermediate Python programmer, this guide will help you understand the fundamental concepts of regular expressions and how to effectively apply them in real-world scenarios.
In this article, we will cover the following practical examples of using regular expressions in Python:
- Exact Match: Understanding how to search for exact matches within a given text.
- Match Ranges: Exploring the use of character ranges to match specific patterns.
- Character Classes: Learning about predefined character classes like digits, word characters, and whitespace.
- Quantifiers: Understanding how to specify the quantity of characters or groups in a pattern.
By delving into these examples, you will gain a solid understanding of the basic components of regular expressions and their applications in Python. So, let's dive into the world of Python regular expressions and explore their practical usage in the upcoming sections.
Understanding Regular Expressions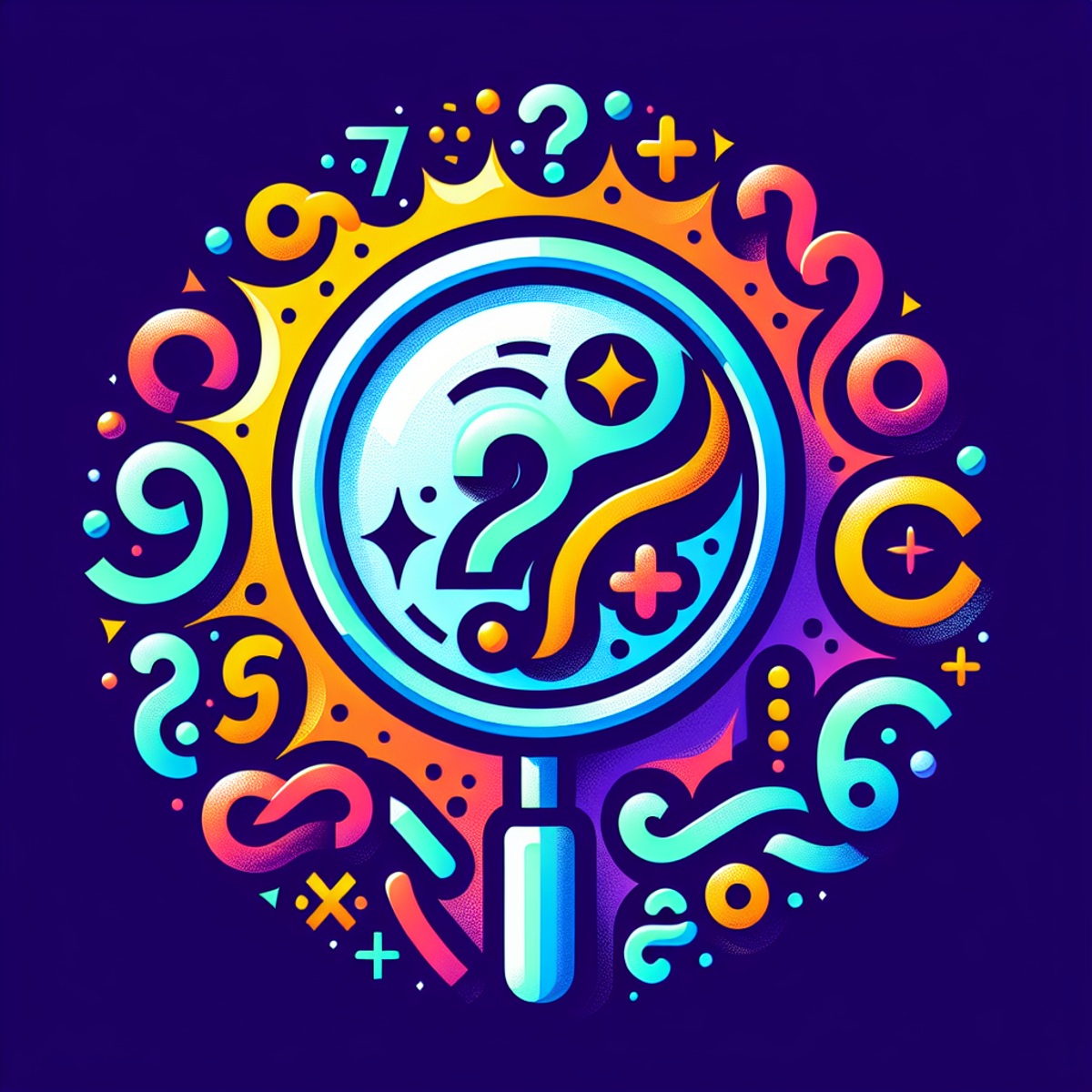
Regular expressions (regex) are a powerful tool for manipulating and searching for text patterns. In Python, the re
module provides support for working with regular expressions. Understanding the basic components of regex is essential for harnessing its full potential.
At its core, a regular expression is a sequence of characters that forms a search pattern. It can be used to check if a string contains the specified search pattern.
Basic Components of Regex
1. Metacharacters
Metacharacters are the building blocks of regular expressions and have special meanings within patterns. Some essential metacharacters include:
.
(dot): Matches any character except a newline.^
(caret): Matches the start of a string.$
(dollar): Matches the end of a string.|
(pipe): Acts as an OR operator between two patterns.\
(backslash): Escapes metacharacters to match them as literals.
2. Character Classes
Character classes allow matching specific sets of characters. They are enclosed in square brackets [ ]
and can include ranges or individual characters. For example:
[a-z]
: Matches any lowercase letter.[A-Za-z]
: Matches any uppercase or lowercase letter.
3. Quantifiers
Quantifiers control the number of times a character or group can appear in a pattern. Some commonly used quantifiers include:
*
: Matches zero or more occurrences.+
: Matches one or more occurrences.?
: Matches zero or one occurrence.{m}
: Matches exactly m occurrences.{m, n}
: Matches between m and n occurrences.
4. Anchors
Anchors assert whether a match occurs at the beginning or end of a line. The main anchor metacharacters are:
^
: Asserts the position at the start of a line.$
: Asserts the position at the end of a line.
5. Escaped Characters
Some characters have special meanings in regex and require escaping to be matched literally, such as \
, *
, .
etc. For a quick reference on escaped characters in different programming languages, refer to this Microsoft documentation.
Understanding these basic components is crucial for constructing effective regular expressions in Python. These components provide a foundation for defining patterns that can be used for searching, extracting, and replacing text within strings.
By mastering these fundamental elements, you will gain the ability to create precise and powerful regular expressions to manipulate text data effectively. If you are interested in exploring more about regex in different programming languages, resources like this Mozilla Developer Network guide or this DataCamp tutorial on regex in R can be helpful as well.".
The re Module in Python
Python’s re module provides a suite of functions to work with regular expressions.
import re
Some of the key functions are:
- re.match(): Checks if the regex matches at the beginning of the string.
- re.search(): Searches the entire string for a match.
- re.findall(): Returns all non-overlapping matches of the regex in the string.
- re.sub(): Replaces occurrences of the regex with another string.
- Literals : Ordinary characters that match themselves (e.g., 'a', '1').
- Metacharacters : Characters with a special meaning (e.g., '^', '$', '*', '+', '?').
- Character classes : A set of characters enclosed in square brackets (e.g., '[abc]', '[0-9]').
- Quantifiers : Specify how many times the preceding element should occur (e.g., '*', '+', '?').
Understanding the Basics of Regular Expressions in Python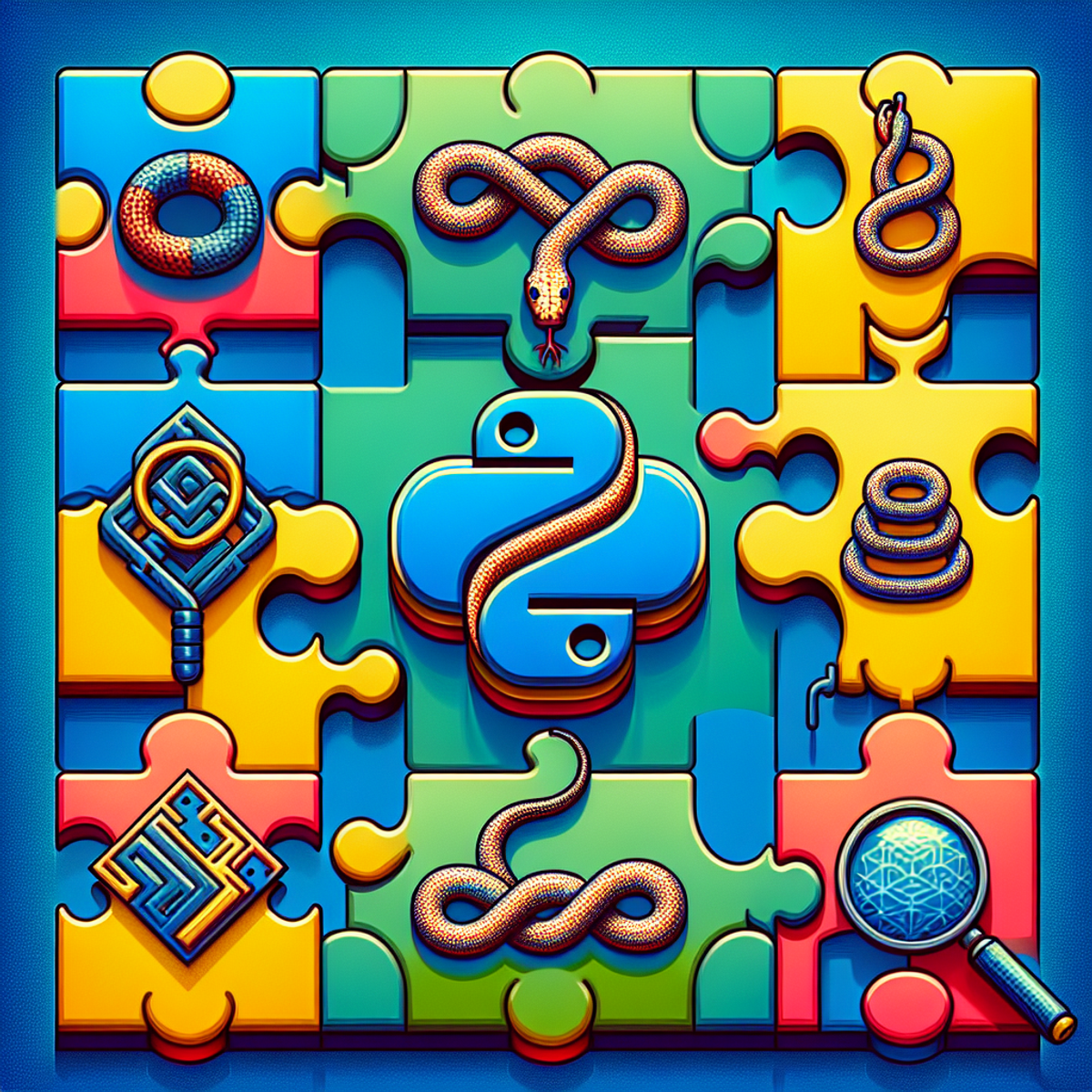
Regular expressions are a powerful tool for pattern matching and text manipulation in Python. They allow you to search for specific patterns of characters within a larger string, making it easier to extract information or validate input. In this section, we will explore the basics of regular expressions in Python, including their syntax and structure.
Definition and Importance
Regular expressions, also known as regex, are sequences of characters that define a search pattern. They consist of a combination of literal characters and special symbols called metacharacters, which have a specific meaning in the context of regular expressions. By using regex, you can perform advanced search operations that go beyond simple string matching.
Regular expressions are widely used in various applications such as data validation, text processing, web scraping, and data extraction. They provide a flexible and efficient way to handle complex pattern matching tasks. Being able to use regular expressions effectively is an essential skill for any Python developer.
Exact Match
One of the simplest regular expressions is an exact match. It matches only the specific sequence of characters you specify. For example, if you want to find all occurrences of the word "python" in a text, you can use the regular expression python
. The re
module in Python provides functions like search()
and findall()
that allow you to search for patterns within strings using regular expressions.
Character Set
A character set allows you to match any single character from a specified set of characters. It is defined by enclosing the desired characters within square brackets [ ]
. For example, the regular expression [aeiou]
matches any vowel character. You can also use ranges within character sets, such as [a-z]
to match any lowercase letter or [0-9]
to match any digit.
Match Ranges
In addition to character sets, regular expressions provide a shorthand notation called match ranges. Match ranges allow you to match a specific range of characters without explicitly listing each character. For example, \d
matches any digit character from 0 to 9, \w
matches any alphanumeric character, and \s
matches any whitespace character.
Character Classes
Character classes are predefined sets of characters that have a specific meaning within regular expressions. They provide a convenient way to match common patterns. Some commonly used character classes include:
\d
: Matches any digit character.\D
: Matches any non-digit character.\w
: Matches any alphanumeric character.\W
: Matches any non-alphanumeric character.\s
: Matches any whitespace character.\S
: Matches any non-whitespace character.
Quantifiers
Quantifiers are metacharacters that specify the number of times a preceding element should occur in order for a match to be found. They allow you to match patterns of varying lengths. Some commonly used quantifiers include:
*
: Matches zero or more occurrences of the preceding element.+
: Matches one or more occurrences of the preceding element.?
: Matches zero or one occurrence of the preceding element.
Understanding the Basics of Regular Expressions in Python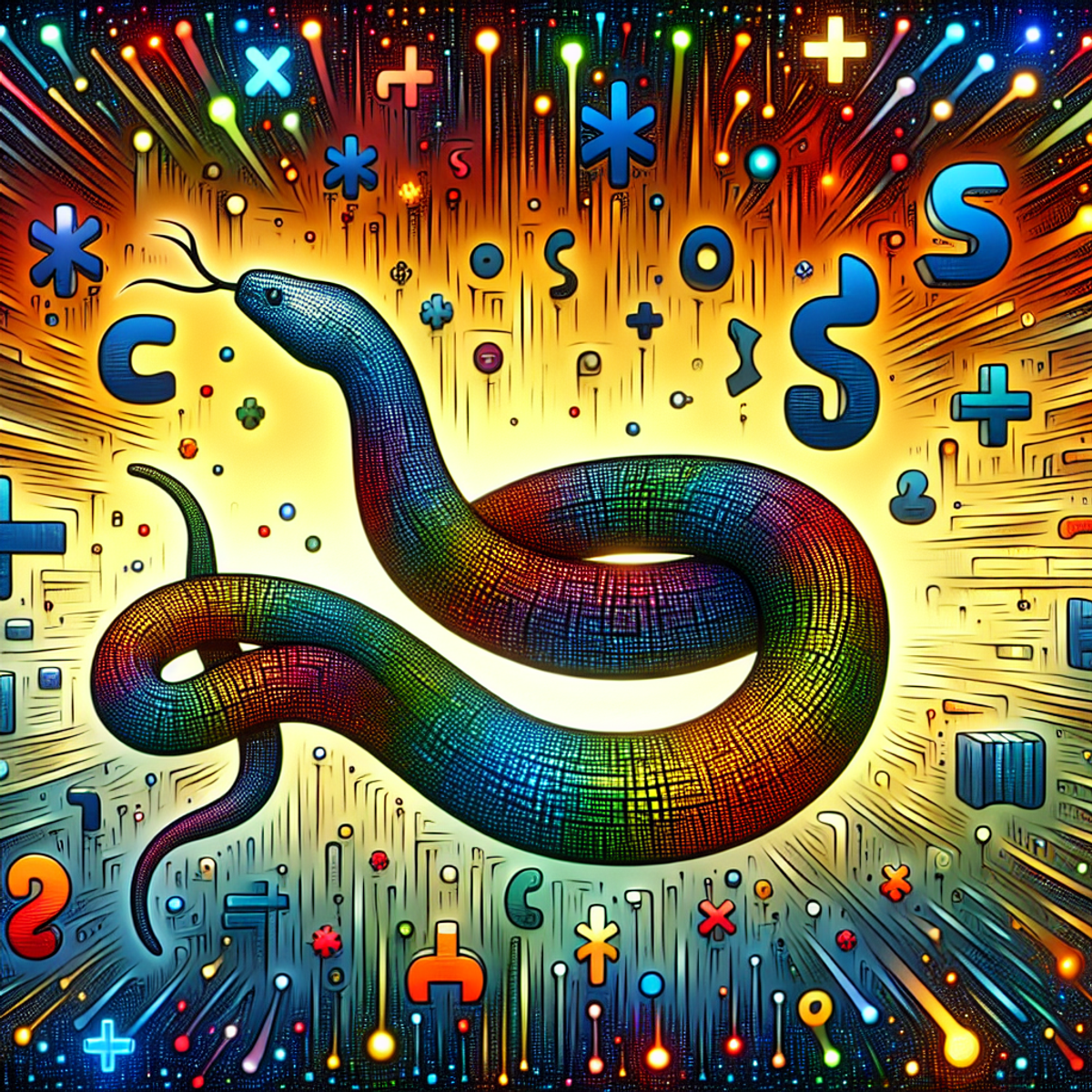
Regular expressions (regex) are a powerful tool for pattern matching and text manipulation. In Python, the re
module provides support for working with regular expressions. Understanding the basics of regex is essential for effectively utilizing its advanced features.
Basic Components of Regex
When working with regular expressions in Python, it's important to grasp the fundamental building blocks that form the basis of regex patterns. These components include:
1. Exact Match
An exact match specifies a pattern that must occur exactly as written in the input string.
python import re pattern = r"cat" text = "The cat and the hat" result = re.search(pattern, text) print(result.group(0)) # Output: 'cat'
2. Match Ranges
Regex allows you to define ranges of characters using square brackets.
python pattern = r"[a-zA-Z]" text = "Sample123" result = re.search(pattern, text) print(result.group(0)) # Output: 'S'
3. Character Classes
Character classes allow you to match specific sets of characters, such as digits or whitespace.
python pattern = r"\d" # Matches any digit text = "Sample123" result = re.search(pattern, text) print(result.group(0)) # Output: '1'
4. Quantifiers
Quantifiers specify how many instances of a character or group should be matched.
python pattern = r"a{2,3}" # Matches 'a' repeated 2 to 3 times text = "caaandy" result = re.search(pattern, text) print(result.group(0)) # Output: 'aa'
pattern = r"a{2,}" # Matches 'a' repeated at least 2 times result = re.search(pattern, text) print(result.group(0)) # Output: 'aaa'
Understanding these basic components lays a strong foundation for delving into more advanced features of Python regular expressions. To further enhance your understanding, you can explore additional resources like:
- A comprehensive guide to regular expressions
- Character classes in other programming languages
- Regular expression language quick reference
- Regex glossary
Applying Python Regular Expressions in Real-World Scenarios
Regular expressions are a powerful tool that can be applied to a wide range of real-world scenarios. By understanding how to use regular expressions effectively in Python, you can streamline your tasks and improve your productivity. In this section, we will explore the significance of practical examples in mastering regular expressions and briefly mention two common applications: log parsing and bulk file renaming.
Significance of Practical Examples in Mastering Regular Expressions
Practical examples play a crucial role in mastering regular expressions. They allow you to apply the concepts you have learned to real-world situations, helping you gain a deeper understanding of how regular expressions work and how to use them effectively.
By working through practical examples, you will become more comfortable with the syntax and logic of regular expressions. You will learn to identify patterns and match specific strings or characters within text, which is a valuable skill for data processing, text manipulation, and pattern matching tasks.
If you're looking for some real-life examples with detailed explanations, this practical regex guide can be a great resource.
Log Parsing
Log files are commonly used to record information about system events or user activities. Analyzing log files can provide valuable insights into system performance, security breaches, or user behavior. Regular expressions are a powerful tool for parsing log files and extracting relevant information.
For example, let's say you have a log file that contains entries like this:
2021-06-15 13:45:21 User 'john' logged in from IP address 192.168.0.1 2021-06-15 14:20:10 User 'alice' logged out
Using regular expressions, you can easily extract information such as timestamps, usernames, login/logout events, and IP addresses from these log entries. Here's an example of how you can use regular expressions in Python to extract usernames:
python import re
log_entry = "2021-06-15 13:45:21 User 'john' logged in from IP address 192.168.0.1" username_pattern = r"User '(\w+)'"
match = re.search(username_pattern, log_entry) if match: username = match.group(1) print(username) # Output: john
By using regular expressions, you can easily extract the desired information from log files and perform further analysis or processing.
Bulk File Renaming
Another practical application of regular expressions is bulk file renaming. If you have a large number of files that need to be renamed according to a specific pattern or criteria, regular expressions can greatly simplify the process.
For example, let's say you have a directory containing image files with names like "image001.jpg", "image002.jpg", and so on. You want to rename these files to have a more descriptive name, such as "photo_001.jpg", "photo_002.jpg", and so on.
Using regular expressions in Python, you can easily achieve this:
python import os import re
directory = "/path/to/images/" pattern = r"image(\d+).jpg"
for filename in os.listdir(directory): match = re.search(pattern, filename) if match: new_filename = f"photo_{match.group(1)}.jpg" os.rename(os.path.join(directory,
Practical Examples
1. Validating Patterns
Suppose you want to validate email addresses:
email_pattern = r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$"
email = "example@domain.com"
if re.match(email_pattern, email):
print("Valid email address.")
else:
This regex checks for a pattern that resembles a standard email format.
2.Finding Patterns Imagine you need to extract all hastags from a tweet :
tweet = "Loving the #Python and #Regex tutorial on #OpenAI!"
hashtags = re.findall(r"#(\w+)", tweet)
print(hashtags)
Output : ['Python', 'Regex', 'OpenAI']
The re.findall()
function extracts all words that follow a hash symbol.
3. Replacing Strings
For instance, anonymizing phone numbers in a text:
text = "Call me at 415-555-1234 or 415-555-9999"
anonymized_text = re.sub(r"\d{3}-\d{3}-\d{4}", "XXX-XXX-XXXX", text)
print(anonymized_text)
Output : Call me at XXX-XXX-XXXX or XXX-XXX-XXXX
The re.sub()
function replaces all occurrences of the phone number pattern with 'XXX-XXX-XXXX'.
4. Splitting Strings
Splitting a string based on any of multiple delimiters:
text = "apples, oranges; bananas,grapes"
fruits = re.split(r",|;", text)
print(fruits)
Output : ['apples', ' oranges', ' bananas', 'grapes']
The re.split()
function uses a regex pattern as a delimiter.
Tips for Using Regex in Python
- Raw Strings: Use raw strings (prefixed with
r
) to avoid confusion with Python’s string escape sequences. - Regex Compilation: For repeated use, compile the regex pattern using
re.compile()
for better performance. - Readability: Complex regex can be hard to read. Commenting and spacing in longer patterns can improve readability.
Conclusion
Python regular expressions are a powerful tool for pattern matching and data manipulation. With a solid understanding of regular expression syntax and functionality, you can unlock a world of possibilities in your coding projects. Here are some final thoughts to keep in mind as you continue your journey in learning Python regular expressions:
- Practice Makes Perfect: Regular expressions can be complex, so don't be discouraged if you don't grasp all the concepts immediately. Like any skill, it takes practice to become proficient. The more you work with regular expressions, the more comfortable and confident you will become.
- Start Simple: When starting out with regular expressions, begin with simple patterns and gradually build up to more complex ones. This approach will help you understand the basics before diving into advanced features. Don't hesitate to experiment and test your patterns with different inputs.
- Learn from Examples: One of the most effective ways to learn regular expressions is by studying practical examples. Look for code snippets or tutorials that demonstrate how regular expressions are used in real-world scenarios. Analyze the patterns, understand the logic behind them, and adapt them to suit your own needs.
- Be Mindful of Performance: While regular expressions are powerful, they can also be resource-intensive. Keep performance in mind when working with large datasets or writing regex patterns that need to be executed frequently. Consider optimizing your patterns or exploring alternative approaches if necessary.
Now that you have a solid foundation in Python regular expressions, it's time to put your knowledge into action! Here are some suggestions for hands-on practice and further exploration:
- Challenge Yourself: Set yourself coding challenges that involve using regular expressions to solve specific problems. For example, try extracting email addresses from a text file or validating phone numbers based on a specific format. These exercises will help reinforce your understanding and improve your regex skills.
- Contribute to Open Source Projects: Many open-source projects rely on regular expressions for tasks like text parsing or data validation. Contributing to these projects is a great way to gain practical experience and collaborate with other developers. You can also learn from existing code and see how regular expressions are used in real-world applications.
- Stay Updated: Regular expressions, like any technology, evolve over time. Stay up-to-date with the latest developments, new features, and best practices in the world of regular expressions. Follow relevant blogs, participate in online communities, and explore resources that focus on regex in Python.
- Explore Other Languages: While this article focused on Python regular expressions, it's worth noting that regular expressions exist in many programming languages. If you find yourself working with other languages, take the opportunity to explore their regex capabilities as well. The concepts you've learned in Python can be applied to other languages with some minor syntax adjustments.
In conclusion, learning Python regular expressions opens up a world of possibilities for pattern matching and data manipulation. With practice and exploration, you can become proficient in constructing complex patterns and leveraging the advanced features of Python's regex engine. Remember to start simple, learn from examples, be mindful of performance, and most importantly, keep coding!
FAQs (Frequently Asked Questions)
What is the purpose of this article?
The purpose of this article is to provide a practical guide to Python Regular Expressions, with a brief mention of the practical examples covered.
What are the basic components of Regex?
The basic components of Regex include understanding the basics of regular expressions in Python, such as syntax and structure, definition and importance, exact match, character set, match ranges, character classes, and quantifiers.
Why is it important to understand advanced features for effective regex patterns?
Understanding advanced features is important for effective regex patterns as it allows for the utilization of capture groups, logical OR, reference capture groups, and naming capture groups.
How can Python regular expressions be applied in real-world scenarios?
Python regular expressions can be applied in real-world scenarios through practical examples and applications. Practical examples play a significant role in mastering regular expressions and can be used for log parsing and bulk file renaming.
What are the final thoughts on learning Python regular expressions?
The final thoughts on learning Python regular expressions include encouragement for hands-on practice and further exploration to solidify understanding and application of the concepts discussed.
- Get link
- X
- Other Apps
Comments
Post a Comment