Effective Error Handling in Python: Mastering Resilience in Your Code
Effective Error Handling in Python: Mastering Resilience in Your Code
Introduction
Effective error handling is a crucial aspect of software development, especially in Python. It involves gracefully handling unexpected situations to ensure the resilience of applications. When errors occur, whether due to user input, network issues, or bugs in the code, proper error handling can prevent crashes and provide meaningful feedback to users.
In this article, we will explore the importance of error handling in software development and how it can enhance the overall quality and reliability of your code. We will delve into various strategies for mastering exception handling in Python, including best practices and specific techniques for clean and robust code. Additionally, we will discuss the significance of exception handling in data pipelines and provide practical tips for implementing effective exception handling strategies.
The content of this article will cover the following areas:
- Understanding Python exceptions and how they work
- Identifying common scenarios that can lead to exceptions
- Implementing effective exception handling strategies in your code:
- Creating custom exception classes
- Logging and error reporting
- Raising and catching exceptions at appropriate levels of abstraction
We will also explore techniques for testing, debugging, and monitoring your exception handling code to ensure its effectiveness. Furthermore, we will discuss the role of exception handling in data pipelines and provide insights into handling exceptions in these critical systems.
By the end of this article, you will have a solid understanding of effective error handling techniques in Python and be equipped with the knowledge to master resilience in your code. Let's dive in!
Understanding Python Exceptions
Python exceptions are unexpected events that occur during the execution of a program, disrupting the normal flow of the application. When an exception occurs, the program stops and raises an error unless it is handled properly.
Exceptions can result from various conditions, such as:
- Invalid input
- Division by zero
- Attempting to access a non-existent file
Understanding Python exceptions is crucial for writing robust and resilient code. By identifying potential exception scenarios and implementing effective exception handling strategies, developers can ensure the stability and reliability of their applications.
Python handles errors by raising exceptions. An exception is an event that occurs during the execution of a program and disrupts its normal flow. Understanding these exceptions is the first step towards effective error handling.
Common Built-in Exceptions
- SyntaxError: Occurs when Python encounters incorrect syntax.
- IndexError: Raised when accessing an index that is not in a sequence.
- KeyError: Similar to IndexError, but for dictionaries.
- TypeError: When an operation is applied to an object of an inappropriate type.
- ValueError: When a function receives an argument with the right type but an inappropriate value.
- FileNotFoundError: Raised when a file or directory is requested but doesn’t exist.
Understanding Exception Handling in Python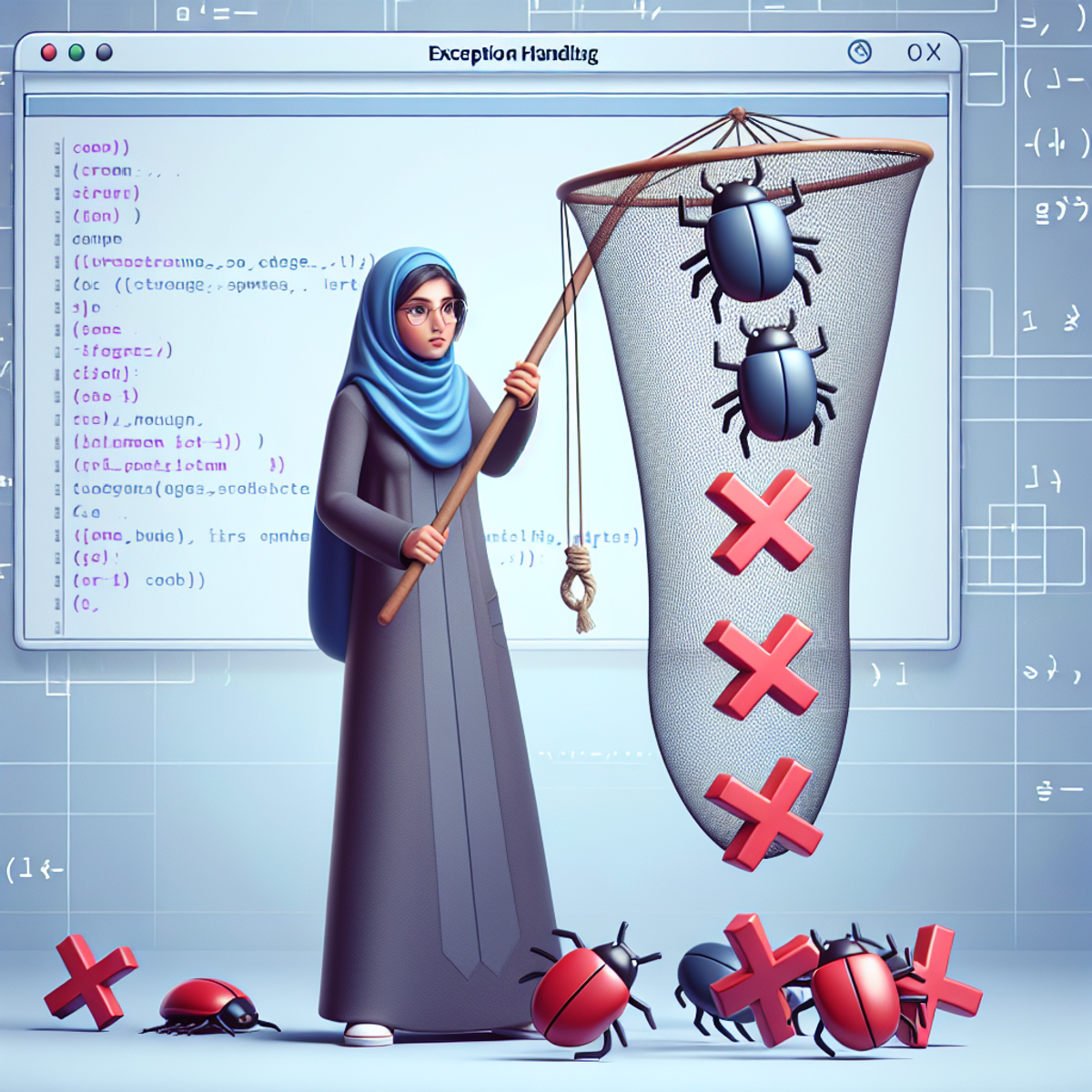
Exception handling is a crucial aspect of software development, allowing programmers to gracefully handle unexpected situations and ensure the resilience of their applications. In Python, exception handling provides a mechanism to detect, respond to, and recover from errors or exceptional conditions that may occur during the execution of a program.
Definition and Purpose of Exception Handling
Exception handling is the process of dealing with runtime errors or exceptional conditions in a way that prevents the program from crashing. It allows developers to anticipate potential errors and provide alternative courses of action when those errors occur. The primary purpose of exception handling is to maintain the stability and reliability of an application by handling errors in a controlled manner.
How Exception Handling Works in Python
In Python, exception handling revolves around the concept of raising and catching exceptions. When an error occurs during program execution, an exception is raised, indicating that something unexpected has happened. Python provides a variety of built-in exceptions for common error scenarios, such as TypeError
, ValueError
, and FileNotFoundError
. Additionally, developers can create custom exceptions to handle specific situations unique to their applications.
The key elements involved in exception handling in Python are:
- Try-Except Blocks: The try-except block is a fundamental construct used for exception handling in Python. It allows developers to specify the code that might raise an exception within the try block. If an exception occurs within the try block, it is caught and handled by one or more except blocks that follow.
- Exception Classes: Exceptions are instances of classes derived from the base
Exception
class. Each exception class represents a specific type of error or exceptional condition. By catching different types of exceptions separately, developers can handle them differently based on their specific requirements. - Handling Exceptions: When an exception occurs within the try block, Python searches for a matching except block that can handle that particular type of exception. If a match is found, the code within the corresponding except block is executed. This allows developers to define the desired response or recovery mechanism for specific types of exceptions.
By effectively utilizing try-except blocks and handling exceptions appropriately, developers can ensure that their programs gracefully handle errors and continue executing without crashing.
Exception handling plays a fundamental role in Python programming, enabling developers to create robust and reliable applications. In the next section, we will explore common scenarios that can lead to exceptions and how to identify them.
Common Scenarios for Exceptions and How to Identify Them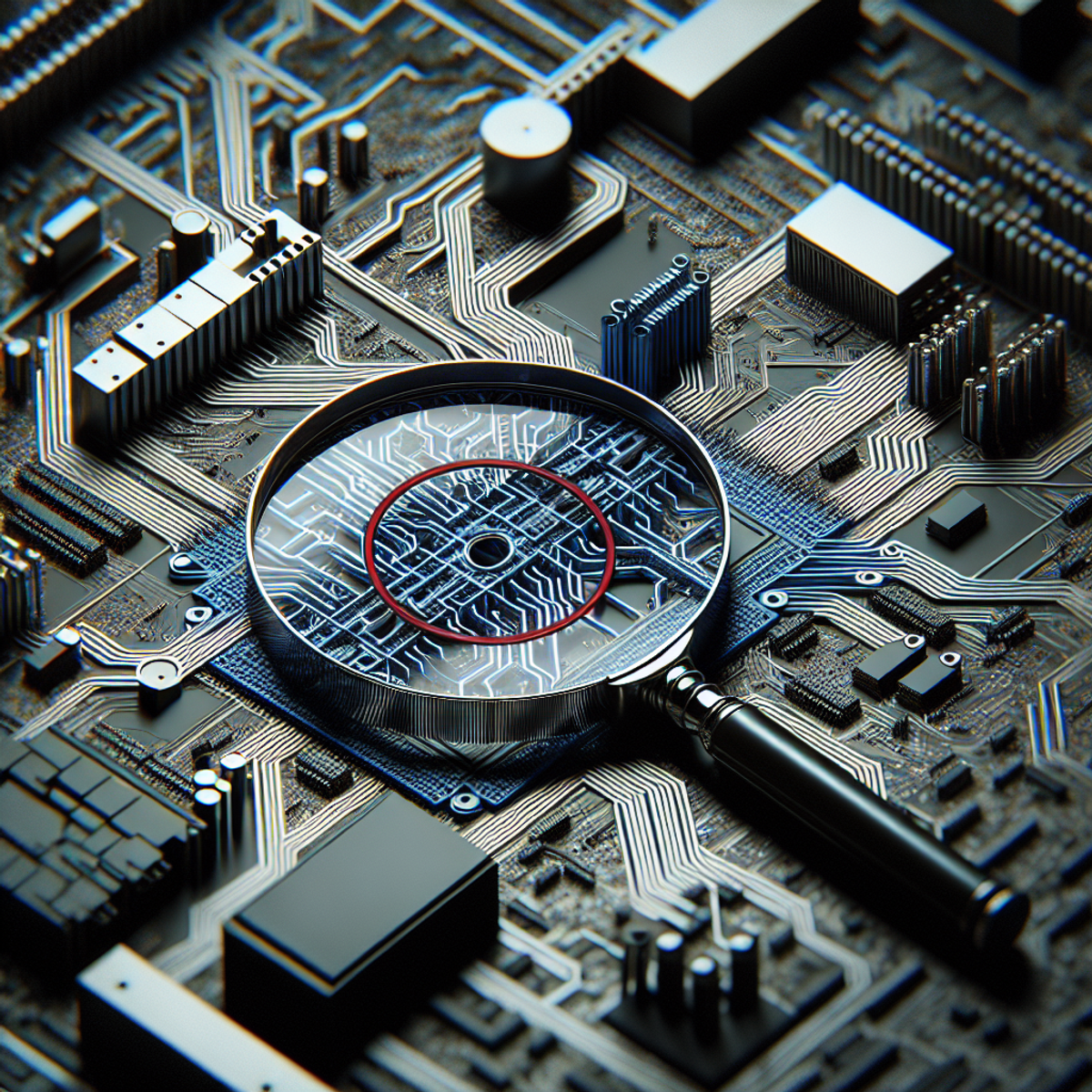
When writing Python code, it's crucial to anticipate potential exception scenarios to ensure robust error handling. By identifying common scenarios that can lead to exceptions, you can proactively implement effective exception handling strategies. Here are some typical situations where exceptions may occur:
1. File Operations:
- Attempting to read from or write to a file that does not exist.
- Insufficient permissions for file operations.
2. Network Operations:
- Connecting to a server that is unavailable or experiencing connectivity issues.
- Timeouts while waiting for a response from a remote server.
3. Input Validation:
- Invalid user input format, such as entering text when expecting numerical input.
- Out-of-range input values causing unexpected behavior.
4. Database Interactions:
- Querying non-existent tables or columns in a database.
- Violating constraints, such as unique key violations.
- Properly handling database exceptions is crucial for data integrity.
5. Resource Management:
- Running out of memory or other system resources during intensive operations.
- Failure to acquire locks or other synchronization primitives.
6. External Dependencies:
- Integration with third-party APIs encountering unexpected responses or errors.
- Incompatibility with specific versions of external libraries or services.
By recognizing these potential scenarios for exceptions, you can proactively design your code to handle these situations gracefully. Identifying these common pitfalls enables you to implement targeted exception handling logic to mitigate the impact of such occurrences. This proactive approach contributes to the overall resilience and reliability of your Python applications.
Understanding the specific areas in your code that are susceptible to exceptions allows you to tailor your error handling mechanisms accordingly, ensuring that your applications can respond effectively to unforeseen circumstances. Recognizing these common scenarios sets the stage for implementing effective exception handling strategies, which will be further explored in the subsequent sections of this article.
Implementing Effective Exception Handling Strategies in Your Code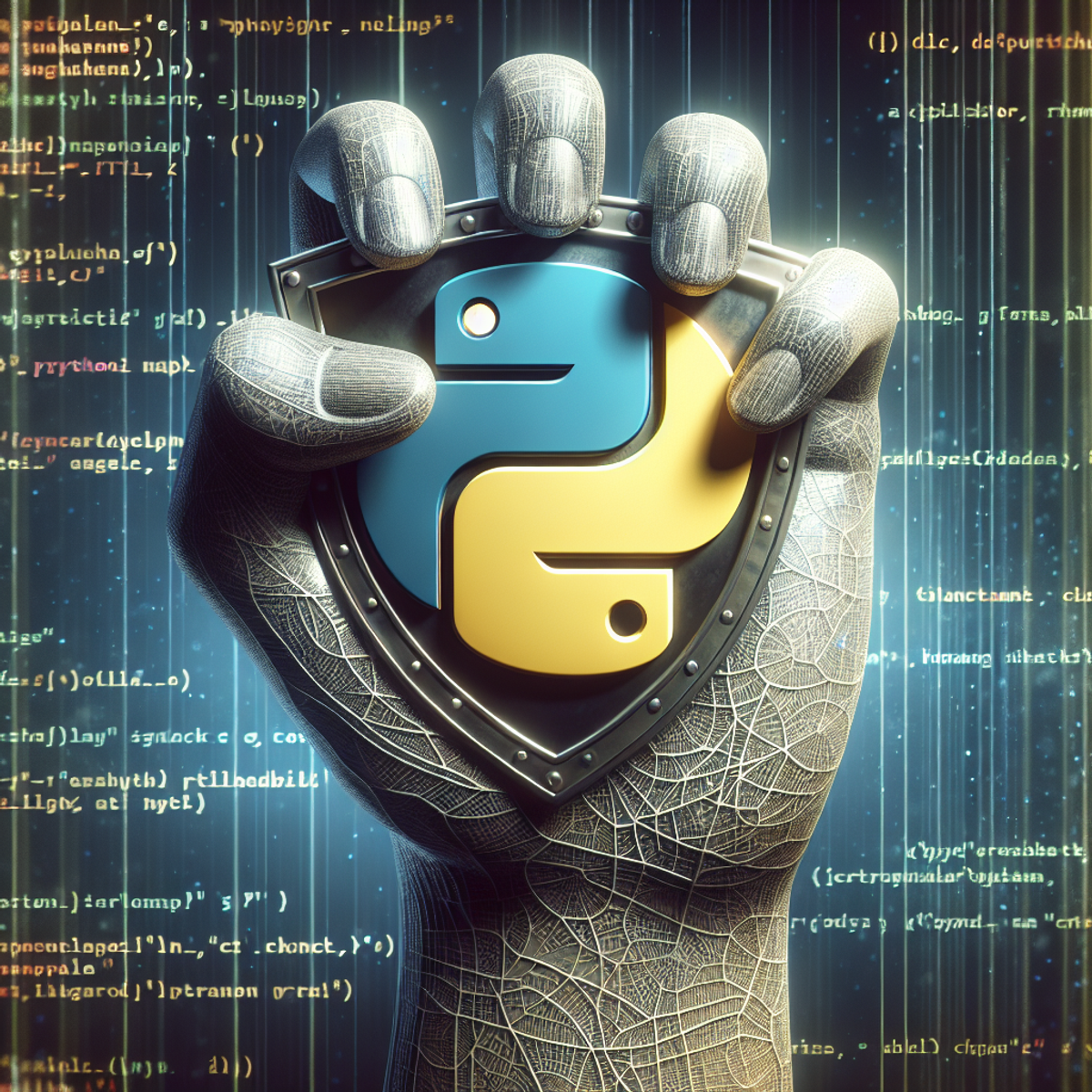
When it comes to mastering resilience in your Python code, implementing effective exception handling strategies is crucial. By incorporating clean code techniques, robust code techniques, and leveraging the power of custom exception classes, you can ensure that your applications gracefully handle unexpected situations. These techniques collectively enhance the precision and flexibility of your error handling mechanisms.
1. Creating Custom Exception Classes
One of the most powerful techniques for effective exception handling in Python is the creation of custom exception classes. This approach offers several advantages:
- Improved Readability: Custom exceptions allow you to convey specific information about the error, making it easier to understand the cause of the exception.
- Granular Error Handling: By defining custom exception classes for different error scenarios, you can provide tailored responses based on the type of exception.
- Centralized Maintenance: With custom exception classes, you can centralize error messages and handling logic, promoting code reusability and maintainability.
To further enhance your exception handling capabilities, consider exploring additional Python exceptions tricks. These tricks provide valuable insights into better error handling practices and can be seamlessly incorporated into your existing strategies.
Let's consider an example implementation of a custom exception class in Python:
python class CustomError(Exception): def init(self, message): self.message = message super().init(self.message)
Raise the custom exception
def validate_input(input_data): if not input_data: raise CustomError("Input data cannot be empty")
In this example, we define a custom exception class CustomError
that inherits from the base Exception
class. We initialize it with a custom error message and utilize it within a function to handle specific input validation scenarios.
By leveraging these techniques in your Python code, you can significantly enhance the precision and flexibility of your error handling mechanisms.
2. Logging and Error Reporting
Logging is an important part of effective error handling in Python. It helps you track how your program is running, find problems, and understand how your code behaves in different situations. Here are some key things to know about logging and error reporting:
1. Why Logging Matters
Logging is a way to keep a record of what's happening when your code runs. It's useful for:
- Finding errors: When something goes wrong, you can look at the log to see what happened right before the error occurred.
- Understanding unexpected behavior: If your code isn't doing what you expect, logging can show you what it's actually doing.
- Tracking important events: You can use logging to keep track of specific actions or milestones in your program.
2. Different Types of Log Messages
In Python, you can use different log levels to categorize your messages based on their importance or severity. The main log levels are:
- DEBUG: Detailed information for debugging purposes
- INFO: General information about what's happening
- WARNING: Indication that something unexpected happened or may be going wrong
- ERROR: An error occurred, but it didn't stop the program from running
- CRITICAL: A serious error occurred that forced the program to stop
By using these different log levels, you can control how much information gets recorded and easily filter out less important messages when needed.
3. Monitoring Errors in Real-time
It's not enough to rely solely on logs after something goes wrong; it's better to catch and address errors as soon as possible. This is where error reporting tools come in handy. By integrating these tools with your Python applications, you can:
- Receive notifications when errors happen
- See detailed information about each error, including the stack trace (which shows the sequence of function calls leading to the error)
- Track the frequency and impact of different types of errors over time
Some popular error reporting tools for Python include Sentry, Rollbar, and New Relic. These tools can help you stay on top of any issues in your code and ensure that your applications are running smoothly.
By using logging effectively and integrating error reporting tools into your workflow, you can get a better understanding of how your Python applications behave in real-world scenarios. This knowledge will empower you to fix problems quickly and build more robust software.
3. Raising and Catching Exceptions at Appropriate Levels of Abstraction
Raising and catching exceptions at the appropriate levels of abstraction is crucial for writing clean and robust code in Python. By following best practices and using specific techniques for Python, you can ensure that your code handles exceptions effectively. Here are some key points to consider:
Writing Modular and Reusable Code
One of the fundamental principles of clean code is writing functions and classes that are focused on specific tasks. By breaking down your code into smaller, modular components, it becomes easier to handle exceptions at the appropriate level. Each function or class should have a well-defined responsibility, making it easier to identify where exceptions should be raised or caught.
Using Context Managers for Resource Management
In Python, context managers provide a convenient way to manage resources such as files or network connections. By using the with
statement, you can ensure that resources are properly released, even in the presence of exceptions. Context managers automatically handle cleanup operations using the __enter__
and __exit__
methods. This not only helps in writing cleaner code but also ensures that resources are properly managed without relying on manual error handling.
Handling Multiple Exceptions with a Single Except Block
Python allows you to handle multiple exceptions using a single except
block. This can help reduce code duplication and improve readability. Instead of having separate blocks for each exception type, you can list multiple exception types within a single except
block. For example:
python try: # Code that may raise different exceptions except (ExceptionType1, ExceptionType2) as e: # Exception handling logic
By catching multiple exception types together, you can provide a unified error-handling approach and perform common actions for those exceptions.
By considering these techniques, you can write code that is more resilient to unexpected errors and easier to maintain. Remember to raise exceptions at the appropriate levels of abstraction, handle resources properly using context managers, and use the flexibility of Python's exception handling to handle multiple exception types efficiently.
Testing, Debugging, and Monitoring Your Exception Handling Code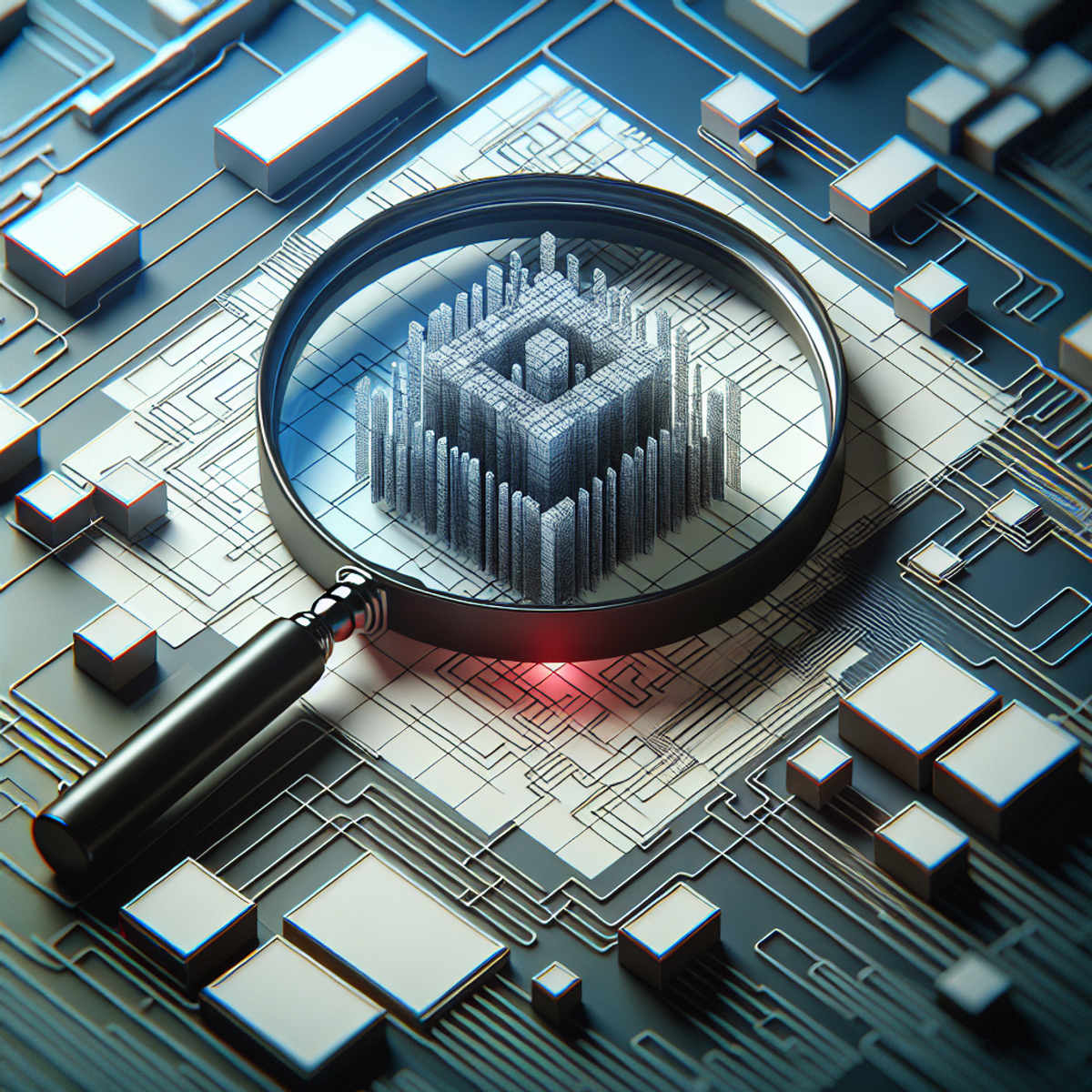
Testing exception handling logic is crucial to ensure the robustness and reliability of your code. Writing test cases for expected exceptions and simulating error scenarios can help identify potential issues and verify the effectiveness of your exception handling strategies. Additionally, configuring logging in Python applications enables you to monitor and track errors in real-time, providing valuable insights for debugging and troubleshooting.
Importance of Testing Exception Handling Logic
- Unit testing exception scenarios allows you to validate the behavior of your code under various error conditions.
- It helps uncover potential vulnerabilities and ensures that exceptions are handled appropriately without causing unexpected failures.
Writing Test Cases for Expected Exceptions
- Define specific test cases to cover different types of exceptions that your code may encounter.
- Verify that the correct exceptions are raised and handled as intended in response to different error conditions.
Mocking External Dependencies for Controlled Testing
- Mocking external dependencies such as APIs or databases allows you to create controlled testing environments.
- By simulating different failure scenarios, you can evaluate how your code responds to exceptions without impacting actual resources.
Configuring Logging in Python Applications
- Utilize the logging module in Python to record detailed information about errors and exceptions during application execution.
- Configure log levels to categorize the severity of logged messages, enabling effective filtering and analysis of error data.
By incorporating comprehensive testing, logging, and monitoring practices into your exception handling strategy, you can proactively identify and address potential issues, ensuring the resilience and stability of your Python applications.
The Role of Exception Handling in Data Pipelines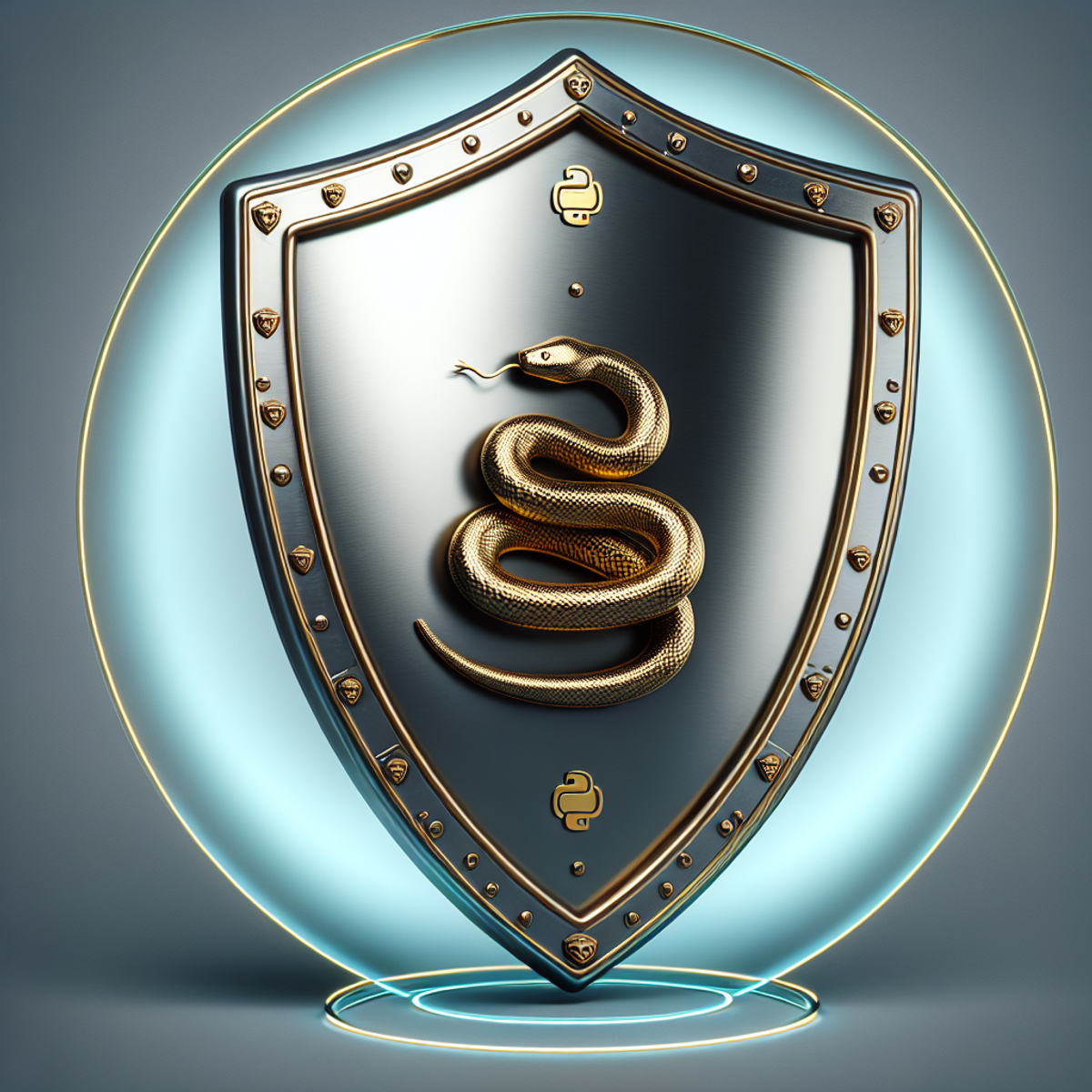
Exception handling is crucial in ensuring the smooth operation of data pipelines. These pipelines are responsible for handling the movement and processing of data from one system to another. Given the complexity and importance of data pipelines, it's essential to understand why exception handling matters in this context.
1. Significance of Exception Handling in Data Processing
Exception handling is vital in data pipelines because any unexpected error or failure during the processing of large volumes of data can have far-reaching consequences. Properly handled exceptions ensure that:
- Data integrity is maintained
- The pipeline can continue processing without disrupting the overall workflow
By implementing effective exception handling strategies, developers can minimize disruptions to the data flow and maintain the reliability and consistency of the entire pipeline.
2. Challenges in Handling Exceptions in Data Pipelines
Data pipelines often face specific challenges when it comes to handling exceptions:
- Dealing with diverse data formats
- Managing complex transformation logic
- Integrating with various external systems
In addition to these challenges, it's crucial for data pipelines to be able to handle intermittent network issues or system failures while maintaining fault tolerance and resilience. Exception handling must address these challenges by providing mechanisms for:
- Graceful error recovery
- Seamless continuation of data processing operations
By addressing these challenges and emphasizing the significance of exception handling in data pipelines, developers can build resilient and reliable data processing systems that are capable of effectively managing unexpected scenarios while maintaining the integrity of the underlying data flow.
Techniques for Effective Exception Handling in Data Pipelines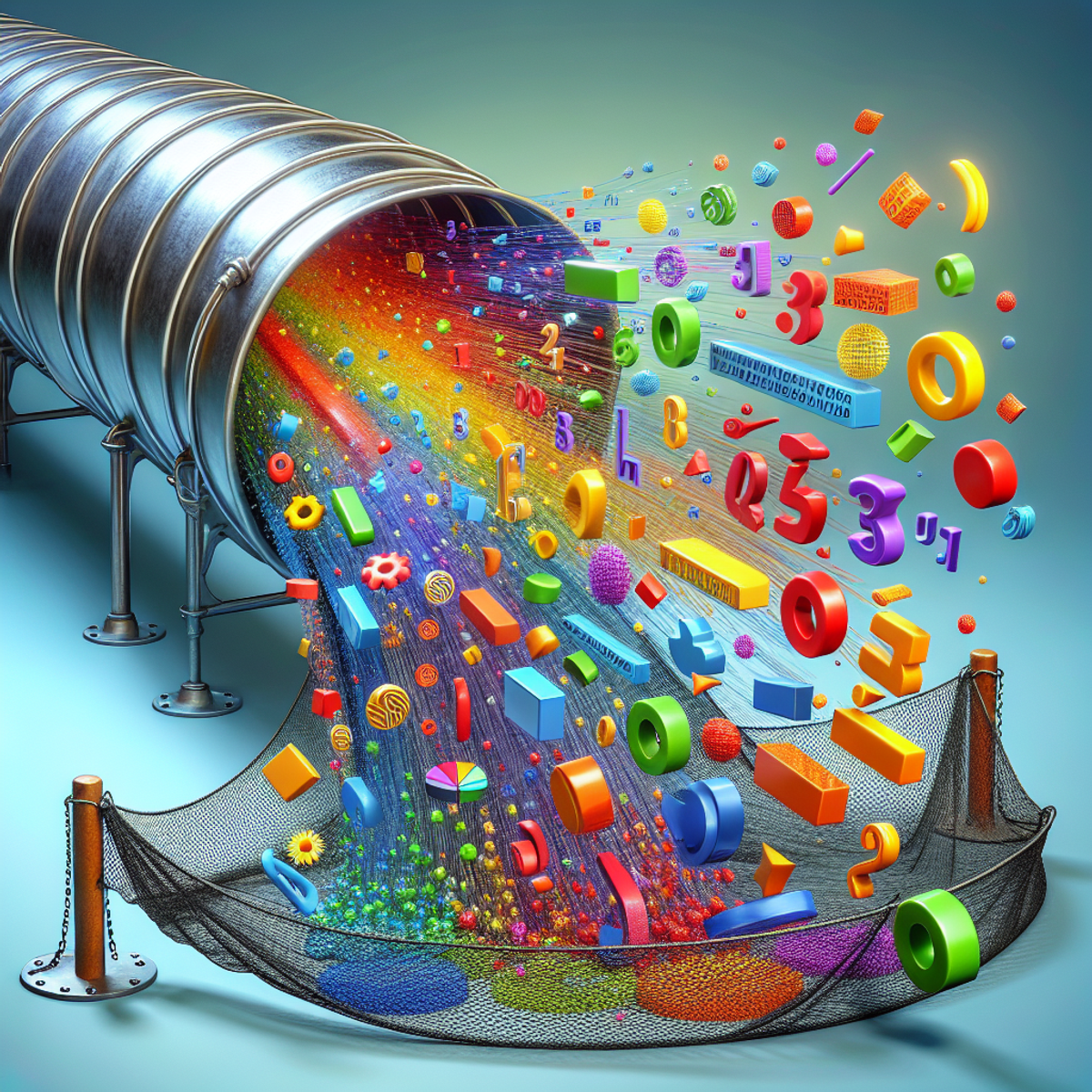
When it comes to data pipeline development, it's crucial to implement effective exception handling strategies to ensure the integrity of data and the smooth functioning of the pipeline. Here are some key techniques for mastering resilience in data pipelines:
1. Ensuring Data Integrity During Exception Recovery Processes
During exception recovery processes, it's essential to prioritize data integrity. When an exception occurs, the processing of data may be interrupted, leading to potential inconsistencies or incomplete operations. To address this, consider implementing strategies such as transactional processing or checkpoints. These mechanisms enable the pipeline to resume from a consistent state after recovering from an exception, thus preserving the integrity of the data being processed.
2. Implementing Retry Mechanisms for Transient Failures
Transient failures, which are temporary and often caused by network issues or resource unavailability, can significantly impact the reliability of a data pipeline. By incorporating retry mechanisms into the exception handling strategy, you can automatically retry failed operations after a short delay, thereby increasing the likelihood of successful execution without manual intervention. This approach helps mitigate the impact of transient failures and enhances the robustness of the data pipeline.
3. Understanding Common Built-in Exceptions
In Python, there are several built-in exceptions that are commonly encountered in data pipeline development. Understanding these exceptions and their implications is fundamental for effective exception handling. Some common built-in exceptions include:
- IOError: Raised when an input/output operation fails.
- ValueError: Occurs when a function receives an argument with the correct type but an inappropriate value.
- KeyError: Raised when a dictionary key is not found.
- TimeoutError: Indicates that a specific operation did not complete within the allocated time.
By familiarizing yourself with these exceptions and their respective contexts, you can tailor your exception handling strategies to address specific scenarios encountered in data pipeline development.
By leveraging these techniques and emphasizing proactive measures for managing exceptions in data pipelines, developers can enhance the reliability and resilience of their pipeline infrastructure.
The try-except
Block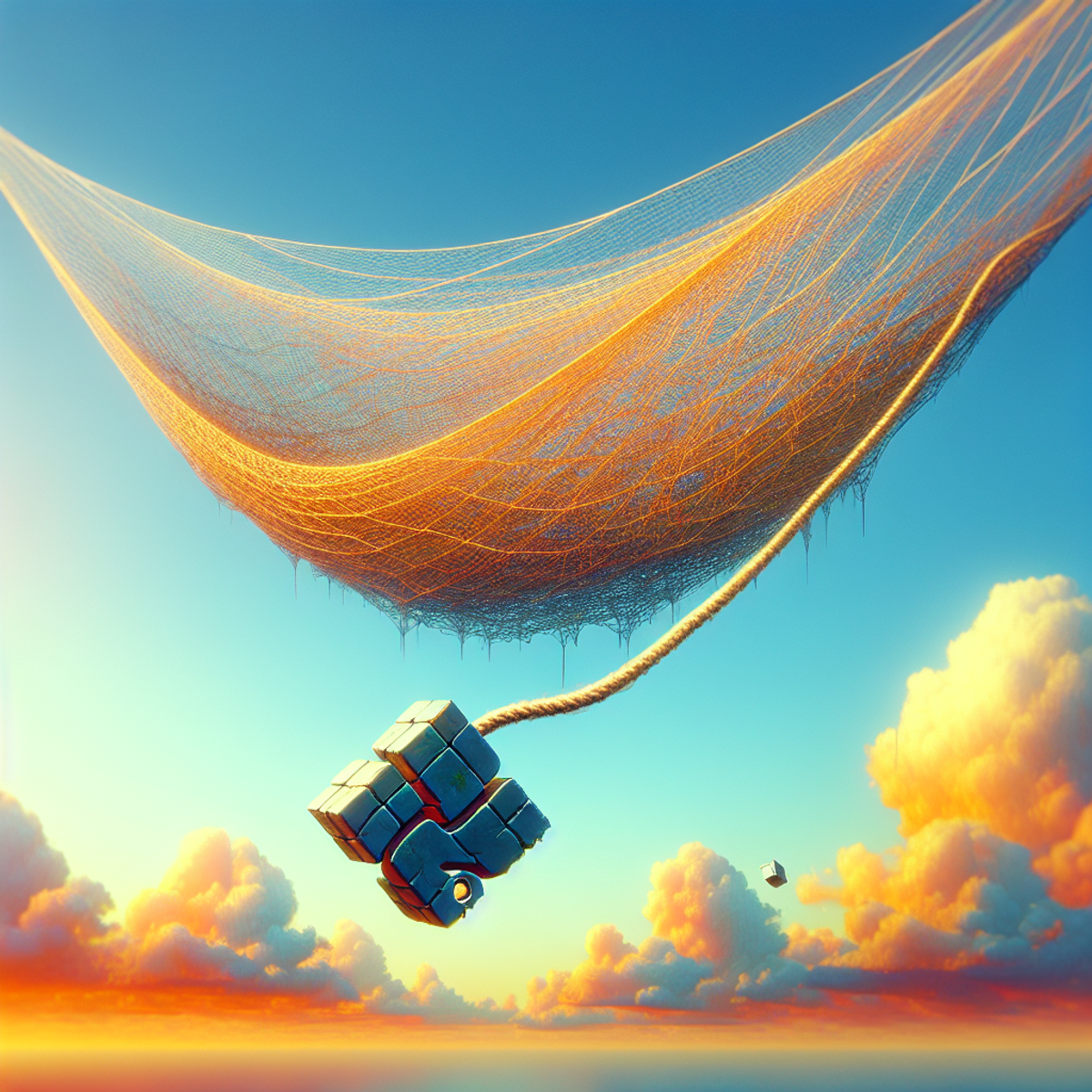
The try-except
block is a fundamental construct in Python that allows you to handle exceptions gracefully. It provides a mechanism to catch and handle specific types of exceptions, preventing your code from crashing when unexpected errors occur.
The structure of a try-except
block is as follows:
python try: # Code that may raise an exception except ExceptionType: # Code to handle the exception
Here's how the try-except
block works:
- The code within the
try
block is executed. - If an exception occurs within the
try
block, Python looks for a matchingexcept
block. - If a matching exception type is found, the code within the corresponding
except
block is executed. - After the execution of the
except
block, program execution continues normally.
You can have multiple except
blocks to handle different types of exceptions. This allows you to provide specific error handling for each type of exception that may occur.
python try: # Code that may raise an exception except ExceptionType1: # Code to handle ExceptionType1 except ExceptionType2: # Code to handle ExceptionType2
If no matching exception type is found, the exception propagates up the call stack until it is either caught by an outer try-except
block or causes the program to terminate with an unhandled exception error.
The use of the try-except
block helps you anticipate potential errors and control how your program responds to them. By handling exceptions effectively, you can prevent your code from crashing and provide meaningful error messages or alternative paths of execution.
While it's useful for handling exceptions, excessive use of broad exception handlers (e.g., using just except:
) should be avoided as it can make debugging more difficult. Instead, it's recommended to catch specific exception types to handle them appropriately.
To gain a deeper understanding of how exceptions work in Python, you can refer to the official documentation. Additionally, this in-depth article on Python exceptions provides valuable insights into their usage. If you encounter situations where unhandled exceptions are causing issues, you can explore techniques like logging uncaught exceptions to aid in debugging.
The primary mechanism for handling exceptions in Python is the
try-except:block
try:
# Code that might cause an exception
result = 10 / 0
except ZeroDivisionError: # Code that runs if the exception occurs
print("You can't divide by zero!")
Best Practices with
try-except
- Catch Specific Exceptions: It's a good practice to catch specific exceptions rather than using a blanket
- except:clause. This prevents masking other bugs.
- Access Exception Details: Use as e to access details of the exception object.
- Least Privilege Principle: Wrap the smallest amount of code possible. Don’t include code that’s unlikely to raise an exception.
The else
Clause in Exception Handling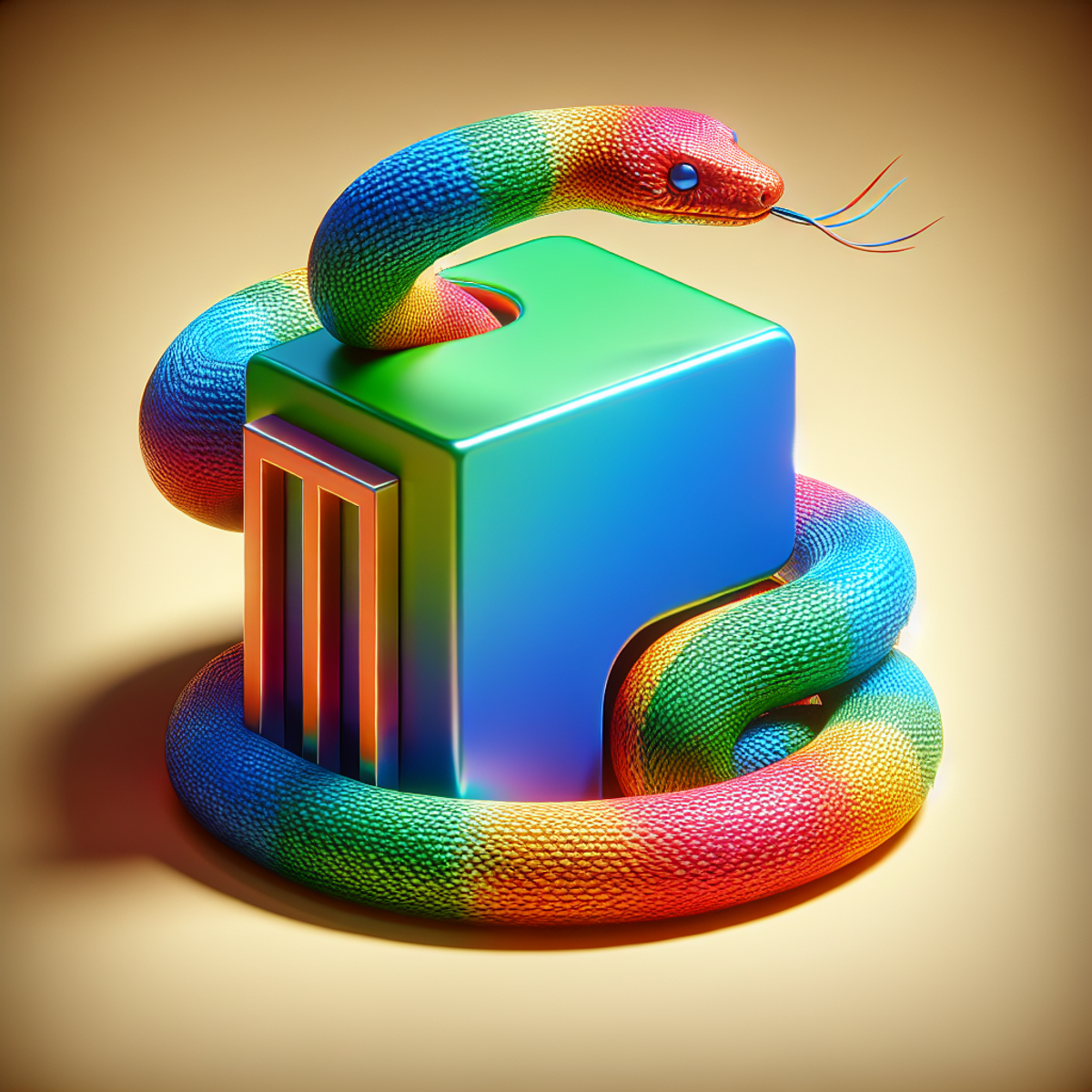
In Python, the else
clause is used in conjunction with the try
and except
blocks to define a piece of code that should be executed if no exceptions are raised within the try block. Here's a breakdown of its significance:
- The
else
clause provides a way to specify code that should run only if no exceptions were raised in the preceding try block. This can be helpful for separating the normal execution path from the exception handling logic. - It is important to note that the
else
block will not be executed if an exception occurs and is not handled by an except block or if a return, continue, or break statement is encountered within the try block.
The finally
Clause in Exception Handling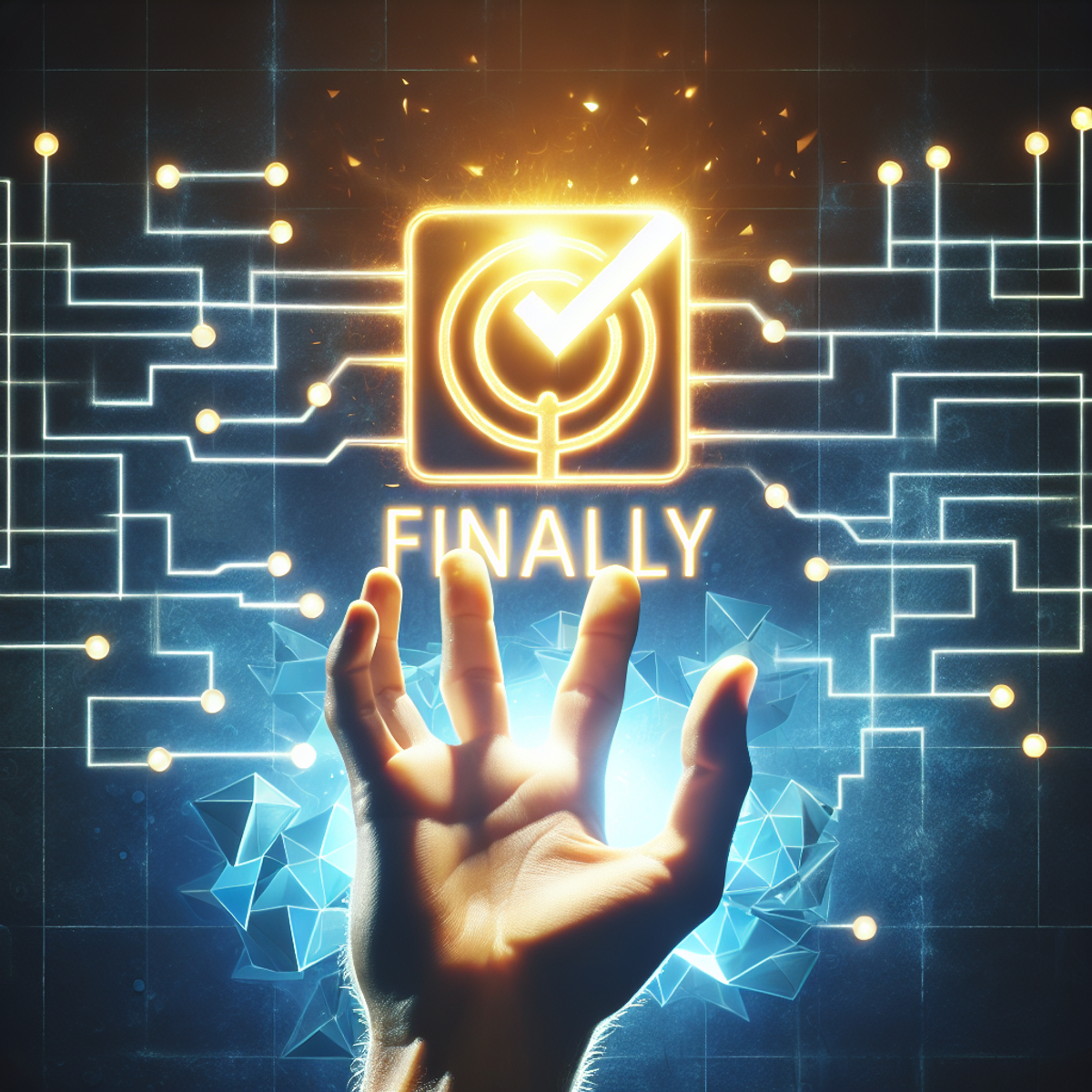
On the other hand, the finally
clause allows you to define a block of code that will always be executed after the try block, regardless of whether an exception was raised or not. Here's what you need to know about it:
- The
finally
block is commonly used for releasing external resources such as files or network connections, ensuring that these resources are properly closed regardless of whether an exception occurred. - It is also useful for performing clean-up actions like resetting variables or closing database connections to maintain the integrity of your program's state.
By understanding and effectively utilizing the else
and finally
clauses in Python's exception handling mechanism, you can enhance the robustness and reliability of your code. These clauses offer additional control over how your program responds to exceptions and manages critical resources, contributing to overall code resilience.
Logging Errors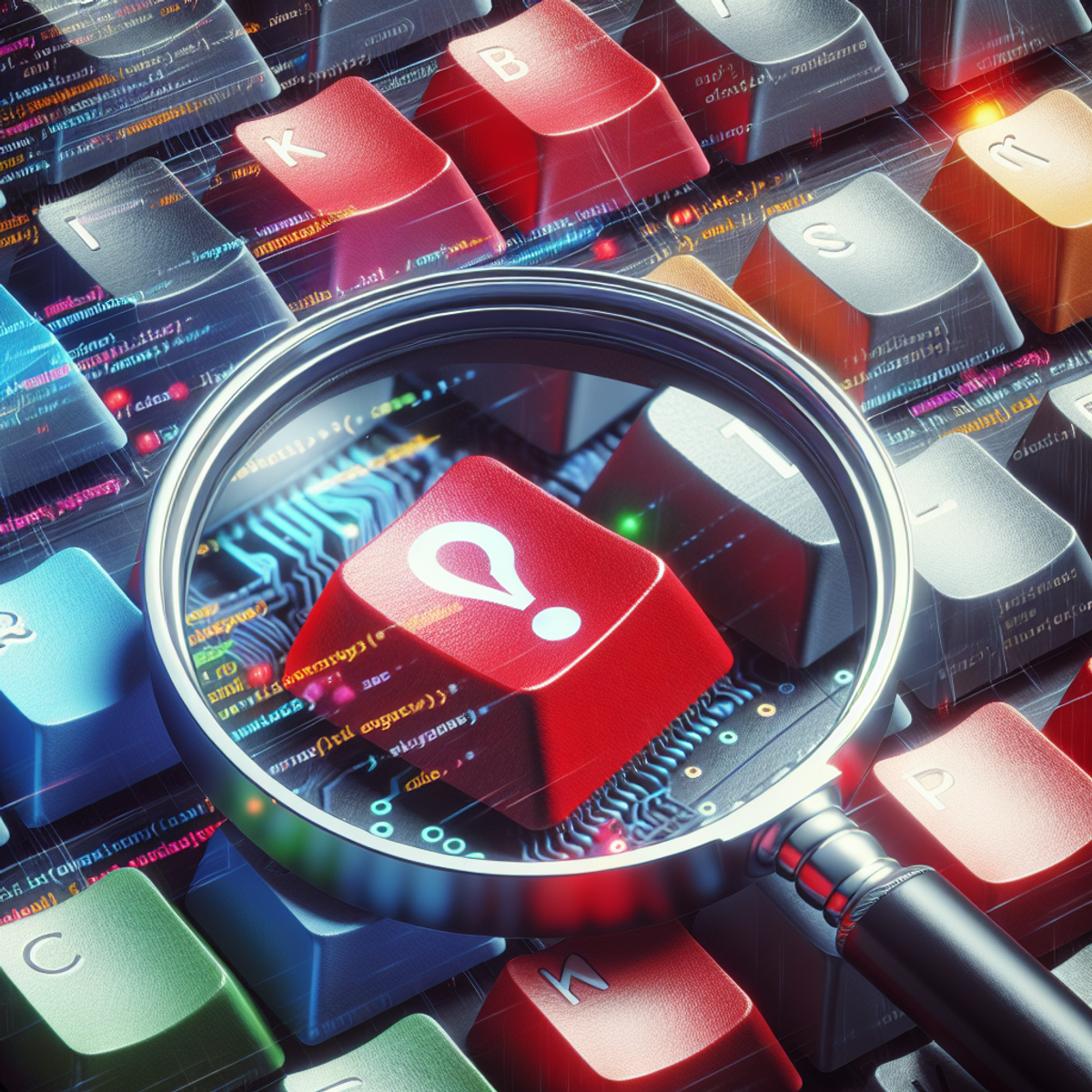
In Python, logging is crucial for capturing and tracking errors in applications. It's an essential tool for developers to understand and fix issues in their code. Here are some important things to know about logging errors:
1. Importance of Logging
- Logging records errors, warning messages, and events in an application.
- It helps understand the code's execution flow and find the causes of unexpected behavior.
2. Different Log Levels
Python's logging module has different log levels to categorize the severity of logged events:
- DEBUG: Detailed information for debugging purposes
- INFO: General information about the application's operation
- WARNING: Indicates a potential issue that doesn't prevent the code from running
- ERROR: Records errors that caused a specific functionality to fail
- CRITICAL: Indicates a critical error that may lead to application failure
3. Configuring Logging
Developers can customize logging settings to suit their needs:
- Choose where log messages should go (files, console, etc.)
- Set the minimum log level to display (e.g., only show ERROR and above)
- Define log message formats
4. Integration with Error Reporting Tools
Logging can work together with error reporting tools to provide real-time monitoring and notifications. This integration ensures that development teams are alerted promptly about critical issues.
By using Python's logging features effectively, developers can easily handle errors in their applications, making them more stable and resilient.
Raising Exceptions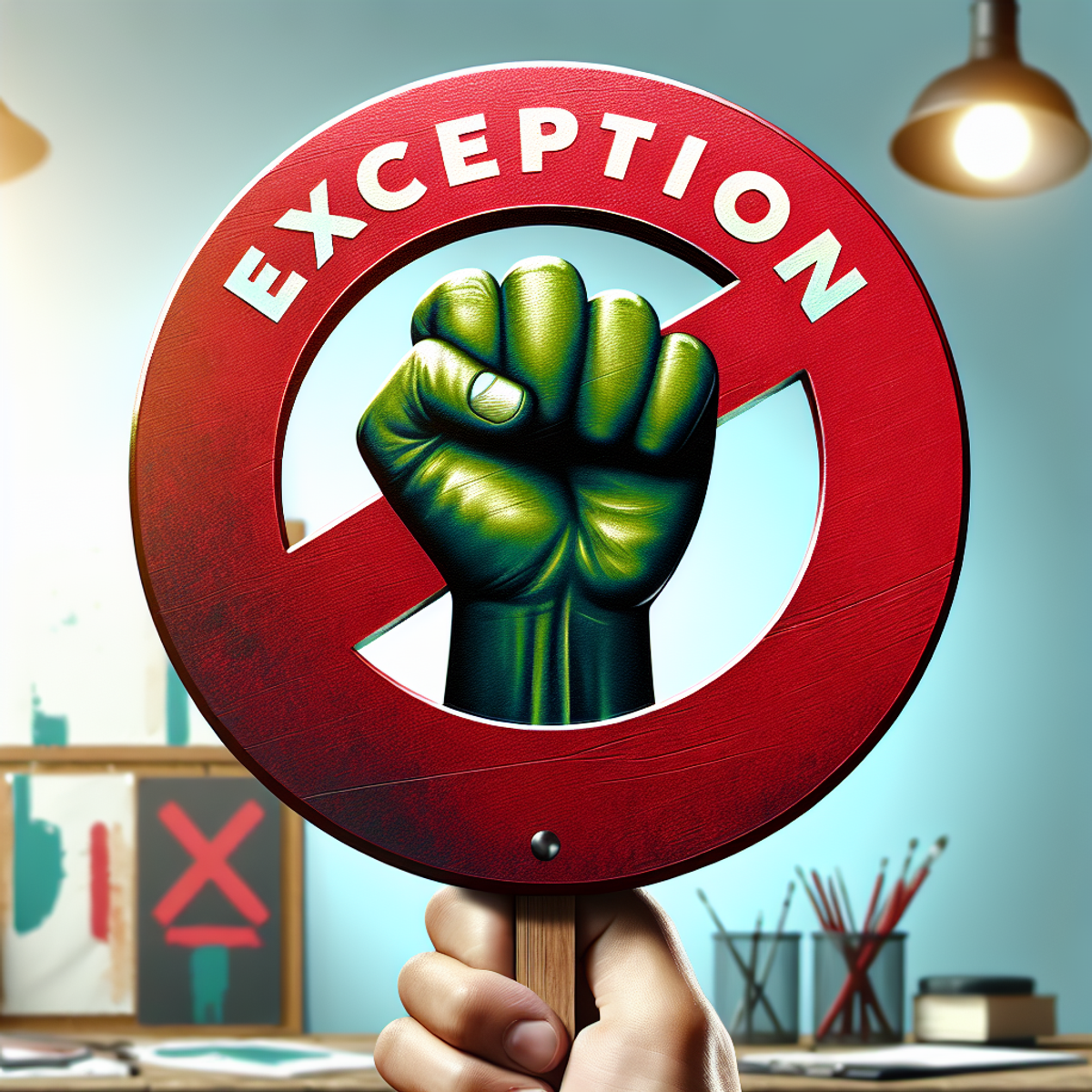
In Python, exceptions are raised when an error or unexpected situation occurs during program execution. When an exception is raised, it interrupts the normal flow of the program and jumps to a specific exception handler that can handle the error appropriately.
To raise an exception in Python, you can use the raise
keyword followed by the type of exception you want to raise. Here are some important points to consider when raising exceptions:
Choosing the Right Exception
It's important to choose the most appropriate built-in exception class or create a custom exception class that accurately represents the error condition. This helps in providing meaningful information about the error to other developers and makes debugging easier.
Providing Additional Information
You can include additional information along with the raised exception by passing arguments to the exception class constructor. For example, you might want to include a specific message or relevant data that can help in understanding and resolving the error.
Raising Exceptions Conditionally
You can use conditional statements to determine when to raise an exception. This allows you to handle specific scenarios or validate inputs before raising an exception. For example, if a function expects a positive integer as input, you can raise a ValueError
if a negative number is provided.
Here's an example of raising a custom exception with additional information:
python class CustomException(Exception): def init(self, message): self.message = message
try: age = int(input("Enter your age: ")) if age < 0: raise CustomException("Age cannot be negative") except CustomException as e: print(e.message)
In this example, if the entered age is negative, a CustomException
is raised with the specified message. The exception is caught using an except
block, and the error message is printed.
Raising exceptions allows you to handle errors gracefully and provide meaningful feedback to users or other developers. It helps in making your code more robust and reliable.
Conclusion
Exception handling is an essential skill that every Python developer should master. By embracing exception handling as a fundamental practice in Python coding, you can ensure the resilience and robustness of your applications. Effective error handling not only prevents your code from crashing but also allows you to gracefully handle unexpected situations, improving the overall user experience.
Mastering resilience in Python code requires a proactive approach to exception handling. Instead of waiting for errors to occur, it's important to anticipate potential exceptions and implement strategies to handle them. By following best practices such as creating custom exception classes, logging and error reporting, and raising and catching exceptions at appropriate levels of abstraction, you can build clean and robust code that is better equipped to handle unexpected scenarios.
Exception handling plays a crucial role in data pipeline development. The nature of data processing often involves large volumes of information, complex transformations, and interactions with external systems. Properly handling exceptions is vital for ensuring data integrity during exception recovery processes and implementing retry mechanisms for transient failures. By implementing effective exception handling strategies, you can minimize data loss or corruption and ensure the smooth operation of your data pipelines.
In conclusion, by embracing exception handling as a fundamental practice in Python coding and mastering resilience in your code, you can build more reliable and resilient applications. Whether you are developing web applications, data pipelines, or any other software solution, effective error handling will contribute to the overall stability and success of your projects.
FAQs (Frequently Asked Questions)
What is the importance of error handling in software development?
Error handling is crucial in software development as it allows for gracefully handling unexpected situations, ensuring the resilience of applications, and maintaining the integrity of data pipelines.
What is the role of exception handling in Python?
Exception handling in Python serves the purpose of gracefully managing unexpected errors that may occur during program execution. It involves the use of try-except blocks to handle potential exception scenarios.
What are some common scenarios that can lead to exceptions in Python?
Common scenarios for exceptions in Python include handling I/O operations, dealing with network connections, and working with external dependencies such as databases or APIs.
How can effective exception handling strategies be implemented in Python?
Effective exception handling strategies in Python can be implemented through techniques such as creating custom exception classes, logging and error reporting, and raising/catching exceptions at appropriate levels of abstraction within the code.
Why is testing, debugging, and monitoring important for exception handling code?
Testing, debugging, and monitoring are essential for ensuring the reliability and robustness of exception handling code. This includes unit testing for exception scenarios, proper logging, and integrating error reporting tools for real-time monitoring.
What is the significance of exception handling in data pipelines?
Exception handling plays a crucial role in data pipelines by ensuring data integrity during exception recovery processes and implementing retry mechanisms for transient failures, thus maintaining the resilience and reliability of the overall data processing system.
Comments
Post a Comment