Python Best Practices for Efficient Coding
Python Best Practices for Efficient Coding
Introduction
Python best practices are essential guidelines for writing efficient code in Python programs. By adhering to these best practices, you can significantly improve the performance, maintainability, and security of your Python applications.
- Following these best practices can lead to:
- Improved performance
- Better maintainability
- Enhanced security of Python applications
In this article, we will explore specific best practices that cover various aspects of Python coding:
- Writing clean and readable code
- Organizing code for maintainability and reusability
- Optimizing performance for faster execution
- Ensuring code security
- Managing dependencies with virtual environments
- Conducting code reviews for quality assurance
These practices aim to ensure efficient coding in Python by following industry-standard guidelines and optimizing code performance and memory usage.
Python Best Practices for Efficient Coding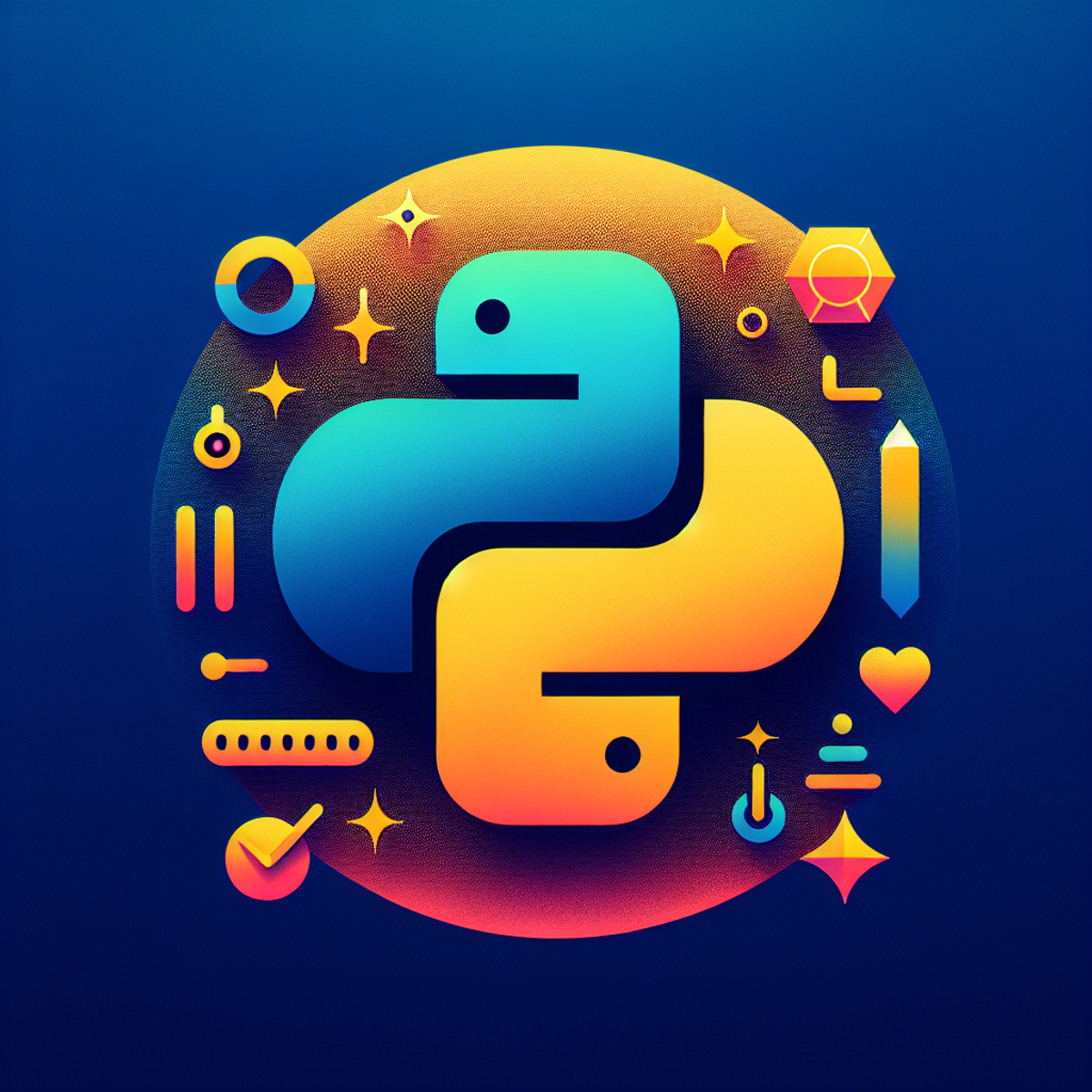
When it comes to writing efficient and maintainable code in Python, following best practices is essential. These practices not only contribute to improved performance but also play a crucial role in enhancing the security of Python applications. Here's a detailed exploration of some key best practices that can elevate your Python coding experience:
1. Writing Clean and Readable Code
Writing clean and readable code is fundamental for ensuring that your code is comprehensible and maintainable. Consider the following aspects:
- Consistent Indentation: Proper indentation enhances code readability and adherence to PEP 8 guidelines.
- Effective Comments and Docstrings: Well-written comments and docstrings provide valuable insights into the functionality and purpose of different components of your code.
- Descriptive Naming: Choosing descriptive names for variables, functions, and classes significantly improves code clarity and comprehension.
2. Organizing Code for Maintainability and Reusability
A well-organized codebase promotes easier maintenance and encourages code reuse. Key strategies include:
- Modular Architecture: Leveraging modules, packages, and classes to create a modular architecture with cohesive functionality.
- Separation of Concerns: Employing techniques like separation of concerns and encapsulation to reduce dependencies between different parts of the codebase.
3. Optimizing Performance for Faster Execution
Performance optimization is crucial, especially when dealing with large datasets or intensive computation. Consider these strategies:
- Algorithmic Improvements: Optimizing algorithms can lead to significant performance gains.
- Caching: Implementing caching mechanisms can reduce redundant computations and improve overall performance.
4. Ensuring Code Security
Security is paramount in Python applications. It's imperative to follow best practices for writing secure code:
- Input Validation: Validating input data to prevent vulnerabilities such as injection attacks.
- Handling Sensitive Data: Implementing robust mechanisms for handling sensitive data securely.
- Mitigating Common Security Threats: Protecting against common threats like SQL injection and cross-site scripting (XSS) through diligent coding practices.
5. Managing Dependencies with Virtual Environments
Managing project dependencies effectively contributes to a robust development environment. Here's what you need to consider:
- Virtual Environments: Utilizing virtual environments to isolate project dependencies, manage version requirements, and ensure consistent development environments.
- Dependency Management Tools: Exploring popular tools such as pip and conda for efficient management of package versions.
6. Conducting Code Reviews for Quality Assurance
Code reviews are indispensable for maintaining high-quality standards in your codebase:
- Constructive Feedback: Encouraging a culture of constructive feedback and knowledge sharing among team members during code reviews.
- Comprehensive Evaluation: Assessing aspects such as adherence to coding style, correctness, performance, and security implications during code reviews.
By incorporating these best practices into your Python coding workflow, you can significantly enhance the efficiency, maintainability, and security of your applications while mitigating common pitfalls.
This comprehensive approach encompasses fundamental coding principles, advanced optimization techniques, security considerations, and effective collaboration within development teams.
1. Writing Clean and Readable Code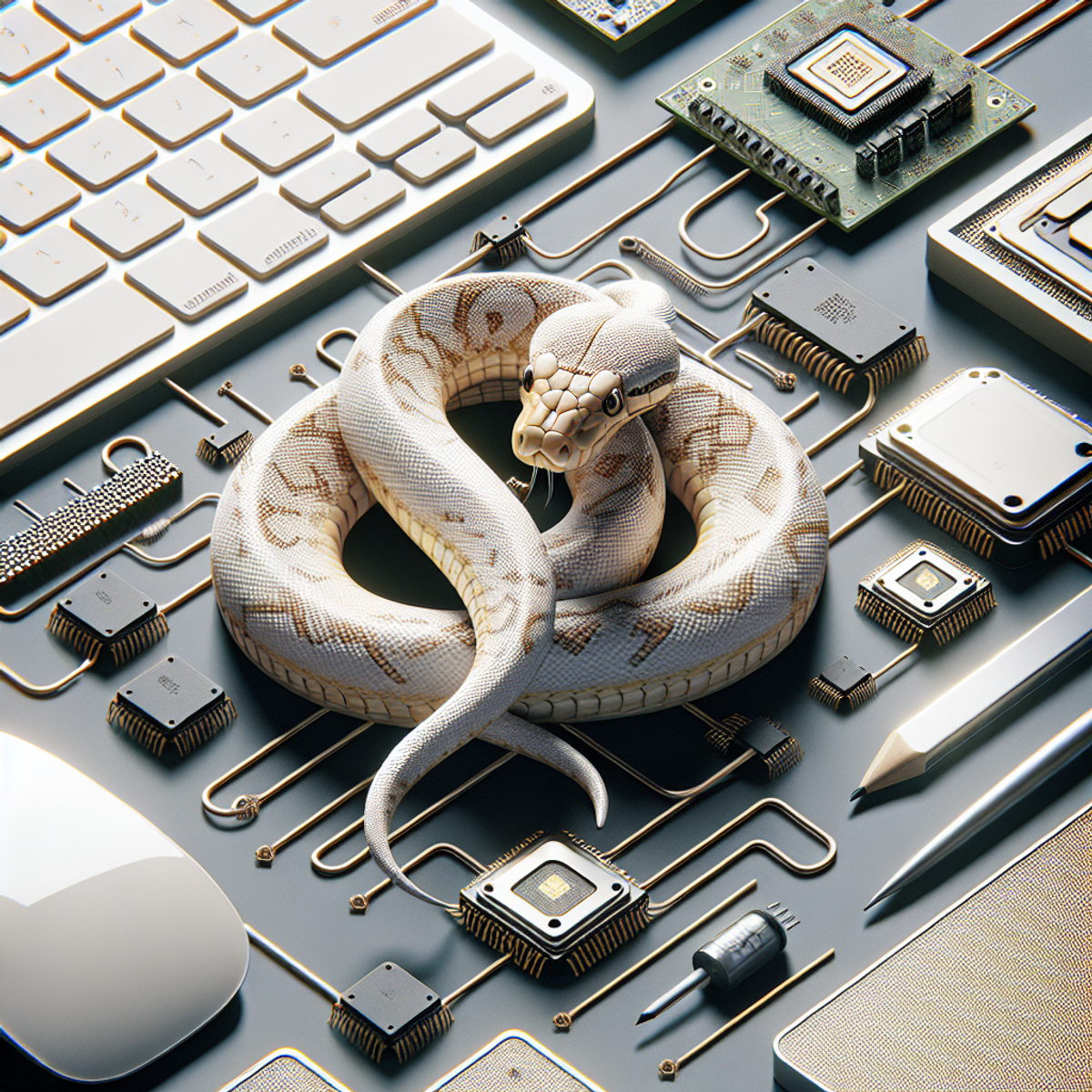
Writing clean and readable code is essential for Python developers. When code is clean and easy to understand, it becomes more maintainable, reduces the chance of introducing bugs, and improves collaboration among team members. Here are some important best practices to follow:
Consistent Formatting and Syntax
Consistency in formatting and syntax makes code more readable and understandable. It helps developers quickly grasp the structure and flow of the code. Here are some key points to keep in mind:
- Indentation: Use consistent indentation, typically four spaces per level, to indicate code blocks. This improves readability by visually separating different parts of the code.
- Whitespace: Use appropriate whitespace to enhance readability. For example, add spaces around operators (
x = 5 + 3
instead ofx=5+3
) and after commas in function arguments (func(arg1, arg2)
instead offunc(arg1,arg2)
). - Line Length: Keep lines of code within a reasonable length, typically 79 characters or less. This prevents horizontal scrolling and makes the code easier to read.
Following the PEP 8 guidelines, which are highly recommended for consistent formatting and syntax, can significantly contribute to code clarity.
Effective Documentation
Good documentation plays a crucial role in making code more understandable. It provides insights into how the code works, its purpose, and any assumptions made during development. Consider the following tips for effective documentation:
- Comments: Add comments to explain complex logic or provide context for certain parts of the code. However, avoid excessive commenting on obvious or self-explanatory sections. As discussed in this Stack Exchange post, commenting every line of code may not always be necessary.
- Docstrings: Use docstrings to document functions, classes, and modules. Docstrings are multi-line strings that appear immediately after the definition of an object. They provide information about its purpose, parameters, return values, and any exceptions raised.
Descriptive Naming Conventions
Choosing descriptive and meaningful names for variables, functions, classes, and modules greatly improves code clarity. It allows others (including your future self) to understand the purpose and functionality of each element without diving into the implementation details. Consider the following strategies for choosing descriptive names:
- Avoid Single-letter Names: Instead of using single-letter variable names like
x
ori
, choose more meaningful names that reflect their purpose. For example, useindex
instead ofi
when iterating over a list. - Use Camel Case or Underscores: Follow a naming convention consistently throughout your code. Python convention favors lowercase letters with underscores for variable and function names (
my_variable
,calculate_average
), while CamelCase is commonly used for class names (MyClass
,MyModule
).
By following these best practices, you can significantly improve the readability and maintainability of your Python code. Clean and readable code reduces the time spent on debugging and makes it easier for others to collaborate on your projects.
2. Organizing Code for Maintainability and Reusability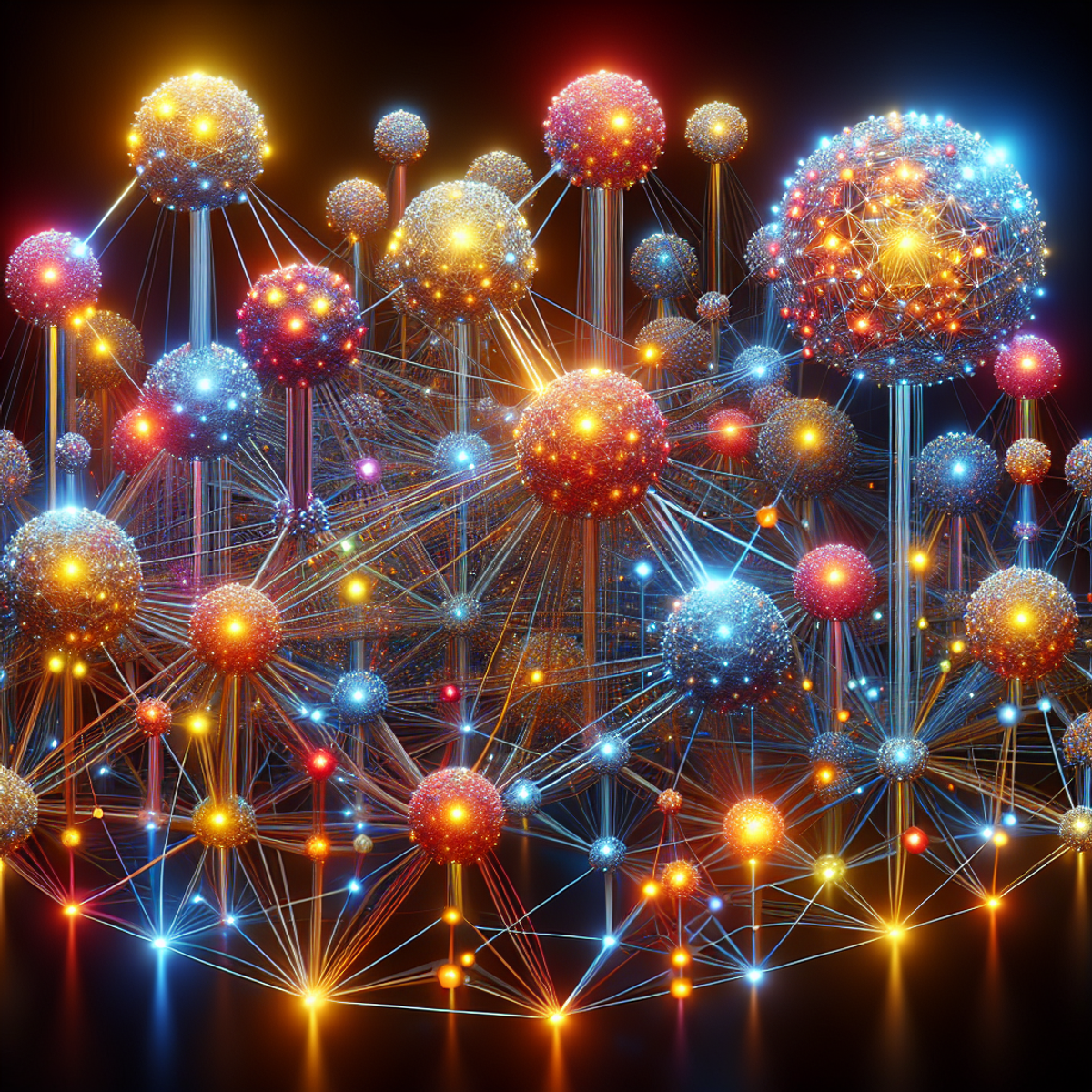
A well-organized structure is essential for easier maintenance and encouraging code reuse in Python projects. By following certain practices and principles, you can create a modular architecture that promotes maintainability and reusability. Here are some key points to consider:
Modules, Packages, and Classes
- Modularization is a fundamental aspect of organizing code. It involves breaking down the functionality of a program into separate modules, each responsible for a specific task.
- Python provides the ability to create modules by simply placing related code in separate
.py
files. These modules can then be imported and used in other parts of the project. - Packages are a way to organize related modules together. They allow you to group related functionality under a common namespace.
- Classes provide another level of organization within modules and packages. They encapsulate data and functionality together, making it easier to manage complex systems.
.py
files. These modules can then be imported and used in other parts of the project.Separation of Concerns
- Separation of concerns is a design principle that advocates dividing a program into distinct sections, each responsible for a specific aspect of functionality.
- By separating concerns, you reduce the dependencies between different parts of your codebase, making it easier to understand, modify, and maintain.
- For example, in a web application, you might separate the user interface logic from the business logic by using different modules or classes for each.
Encapsulation
- Encapsulation is another important principle that helps in organizing code. It involves hiding internal implementation details and exposing only the necessary interfaces to interact with.
- With encapsulation, you can reduce coupling between different parts of your codebase. This makes it easier to change the internal implementation without affecting other parts of the system.
- In Python, you can achieve encapsulation by using private attributes and methods (denoted by a leading underscore) within classes.
Example: Organizing a Web Application
To illustrate these concepts, let's consider a simple web application for managing user accounts. Here's how you can organize the code:
- Create a
models
module that contains classes representing different entities likeUser
andAccount
. - Use a separate
views
module to handle the user interface logic, such as displaying forms and processing user input. - In the
views
module, import the necessary classes from themodels
module to interact with the database and perform operations on user accounts. - If required, create additional modules for handling authentication, authorization, or any other specific concerns.
By following this structure, you can easily maintain and reuse code in your web application. Each module will have a specific responsibility, making it easier to understand and modify in the future. Additionally, encapsulating related functionality within classes ensures loose coupling and better organization.
Remember that code organization is not a one-size-fits-all approach. It depends on the size and complexity of your project. However, by following these best practices, you'll be able to create well-structured and maintainable Python code.
3. Optimizing Performance for Faster Execution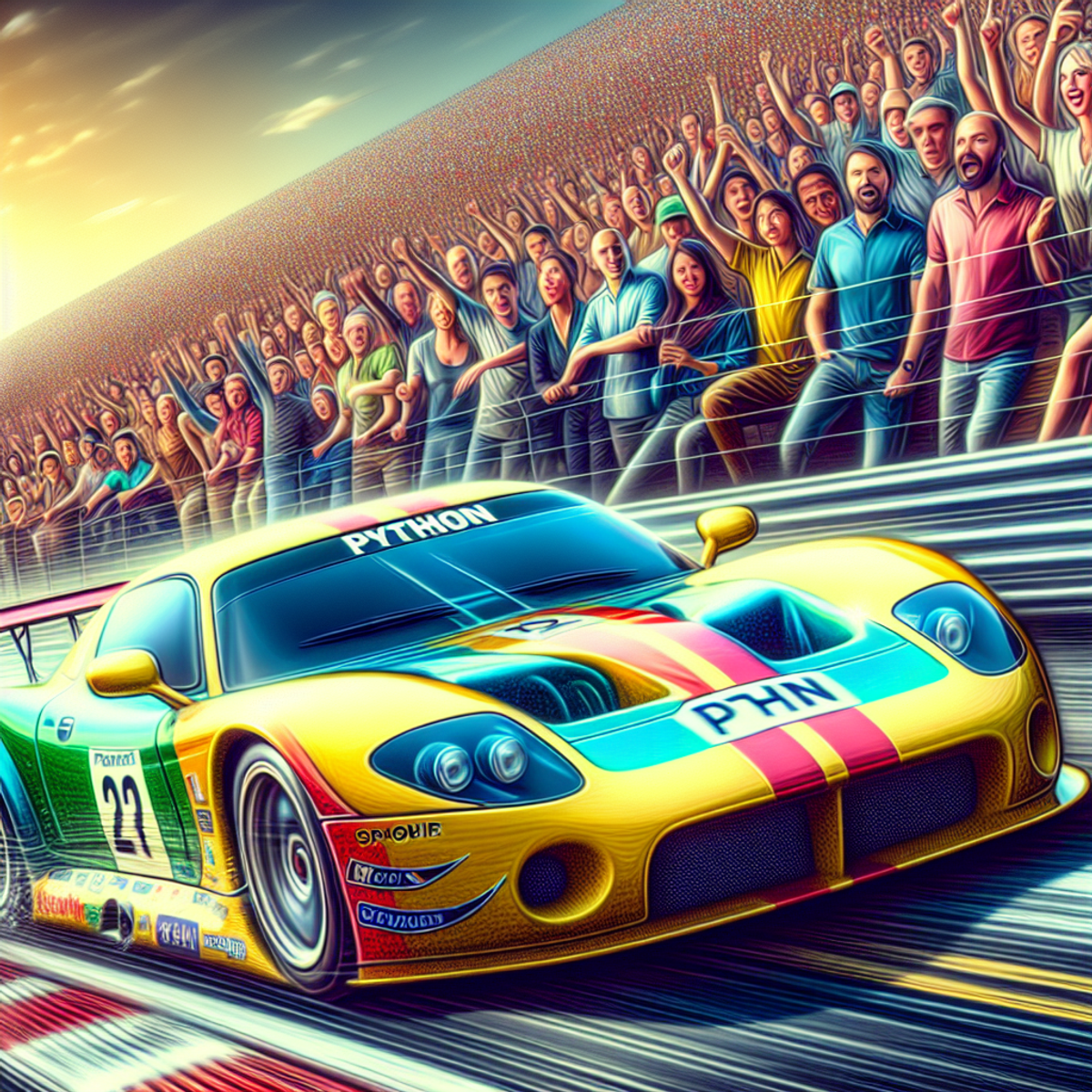
Performance optimization is crucial in Python programming, especially when working with large datasets or intensive computations. By optimizing your code's performance, you can significantly improve execution times and overall efficiency. Here are some strategies and techniques to achieve faster execution in Python:
Algorithmic Improvements
- Choose the right algorithm: The choice of algorithm can greatly affect performance. Consider different algorithms and select the one that best fits your specific problem. Sometimes, a simple algorithm change can lead to significant improvements in execution time.
- Reduce unnecessary operations: Analyze your code and identify any unnecessary operations or redundant calculations. By eliminating these operations, you can streamline your code and improve its efficiency.
Caching
- Memoization: Memoization is a technique where you store the results of expensive function calls and reuse them when needed again. This can be particularly useful when you have functions that are computationally expensive or have repetitive calculations.
- Caching data: If your code involves accessing external resources or retrieving data from databases, consider implementing caching mechanisms. Caching allows you to store frequently accessed data in memory, reducing the need for repeated retrieval from external sources.
Data Structures
- Use appropriate data structures: Choosing the right data structure for your problem can significantly impact performance. For example:
- Use dictionaries for fast lookup operations.
- Use sets for membership testing or removing duplicate elements.
- Use lists for sequential access or maintaining order.
- Consider space-time trade-offs: Depending on your problem's nature, it may be beneficial to trade off memory usage for faster execution times. For instance, precomputing certain values or storing intermediate results can help reduce computational overhead.
Parallelization
- Multiprocessing: Python provides the
multiprocessing
module, which allows you to execute multiple processes concurrently. By leveraging multiple CPU cores, you can achieve parallel execution and speed up your code.
multiprocessing
module, which allows you to execute multiple processes concurrently. By leveraging multiple CPU cores, you can achieve parallel execution and speed up your code.Profiling and Optimization Tools
- Profiling: Use profiling tools to identify performance bottlenecks in your code. Profilers provide insights into which parts of your code are consuming the most time or resources, allowing you to focus your optimization efforts effectively.
- Optimization libraries: Python offers various libraries specifically designed for performance optimization. For example:
- NumPy: A powerful library for numerical computations that provides efficient array operations.
- Numba: A just-in-time compiler that can optimize certain functions for faster execution.
- Cython: A tool that allows you to write Python code with C-like performance by compiling it to C.
Remember, performance optimization is an iterative process. It's essential to measure the impact of each optimization technique and choose the ones that provide the most significant improvements based on your specific requirements. Additionally, keep in mind that premature optimization can often lead to complex and hard-to-maintain code. Focus on optimizing critical sections of your code where performance improvements will have the most significant impact.
By implementing these performance optimization strategies, as discussed in this [article](https://
4. Ensuring Code Security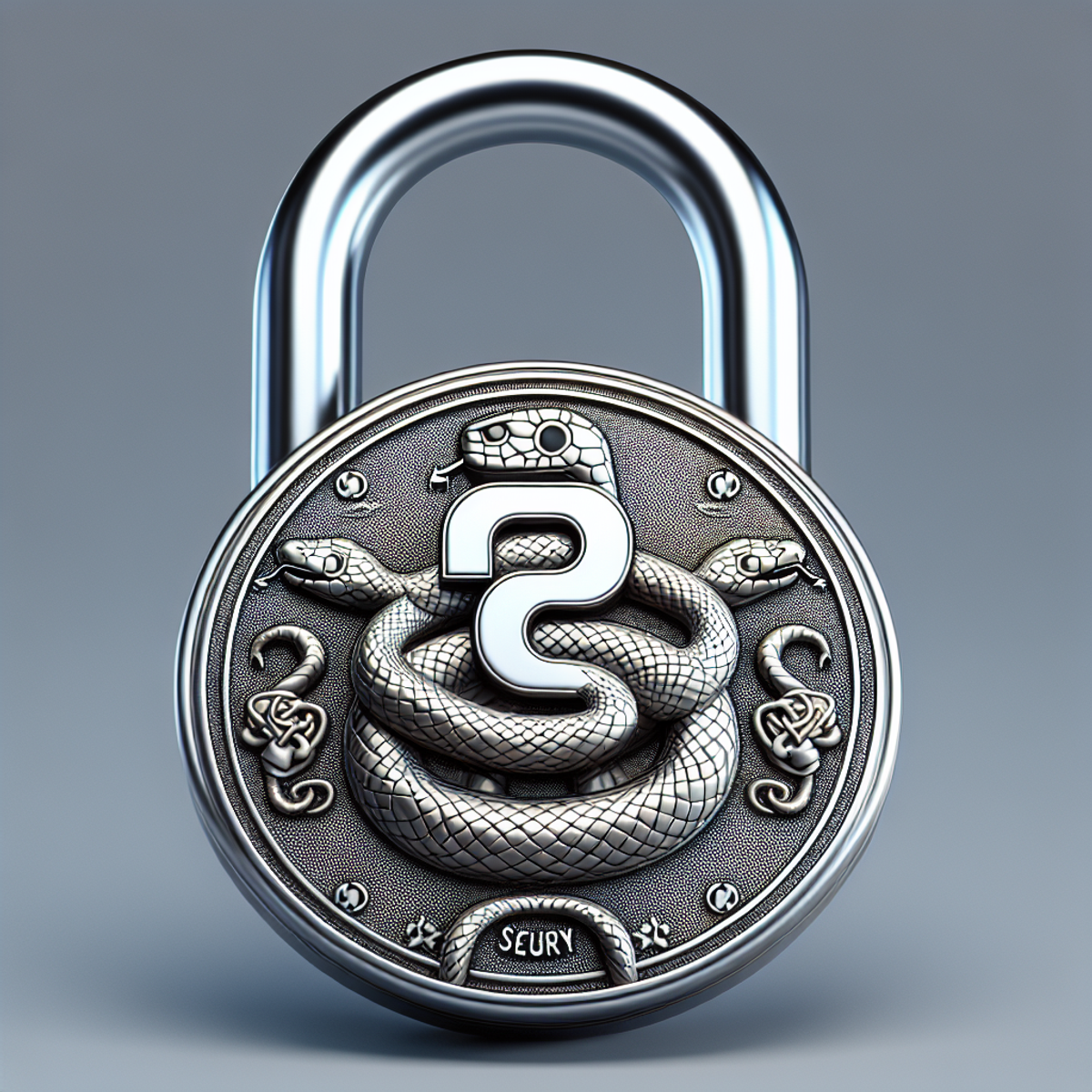
Python security is crucial for creating strong and dependable applications. Ignoring security issues can result in weaknesses that put sensitive information at risk and make the application vulnerable to attacks. Here are some important things to remember when it comes to code security in Python:
1. Importance of Secure Code
Writing secure code is vital for protecting Python applications from potential threats and weaknesses. By making security a top priority, developers can:
- Reduce the chances of unauthorized access
- Prevent data breaches
- Avoid other security incidents
2. Common Vulnerabilities and Solutions
It's essential to know about common security weaknesses in Python applications, such as:
- Problems with validating input
- Insecure handling of sensitive information
- Susceptibility to attacks like SQL injection and cross-site scripting (XSS)
By understanding these weaknesses, developers can take appropriate actions to strengthen the application's defenses.
3. Best Practices for Input Validation
Validating input is extremely important for preventing malicious input from causing issues or compromising security. Developers should validate user input at different entry points like forms, APIs, and user interfaces to:
- Minimize the risk of injection attacks
- Ensure the accuracy of processed data
4. Handling Sensitive Data
Properly managing sensitive information like user credentials, personal details, and financial data is crucial for maintaining user privacy. Developers can protect this data by:
- Using encryption methods
- Storing it securely
- Implementing access controls
5. Protection Against Common Security Threats
Python developers should know how to defend their applications against common security threats like SQL injection and cross-site scripting (XSS). They can do this by:
- Using parameterized queries for database interactions
- Encoding user-generated content correctly
These measures help make the application more resilient against attacks.
By following these best practices during the coding process, developers can improve the security of their Python applications and lower the risk of security breaches or exploits. Prioritizing code security not only protects sensitive information but also builds trust among users and stakeholders.
5. Managing Dependencies with Virtual Environment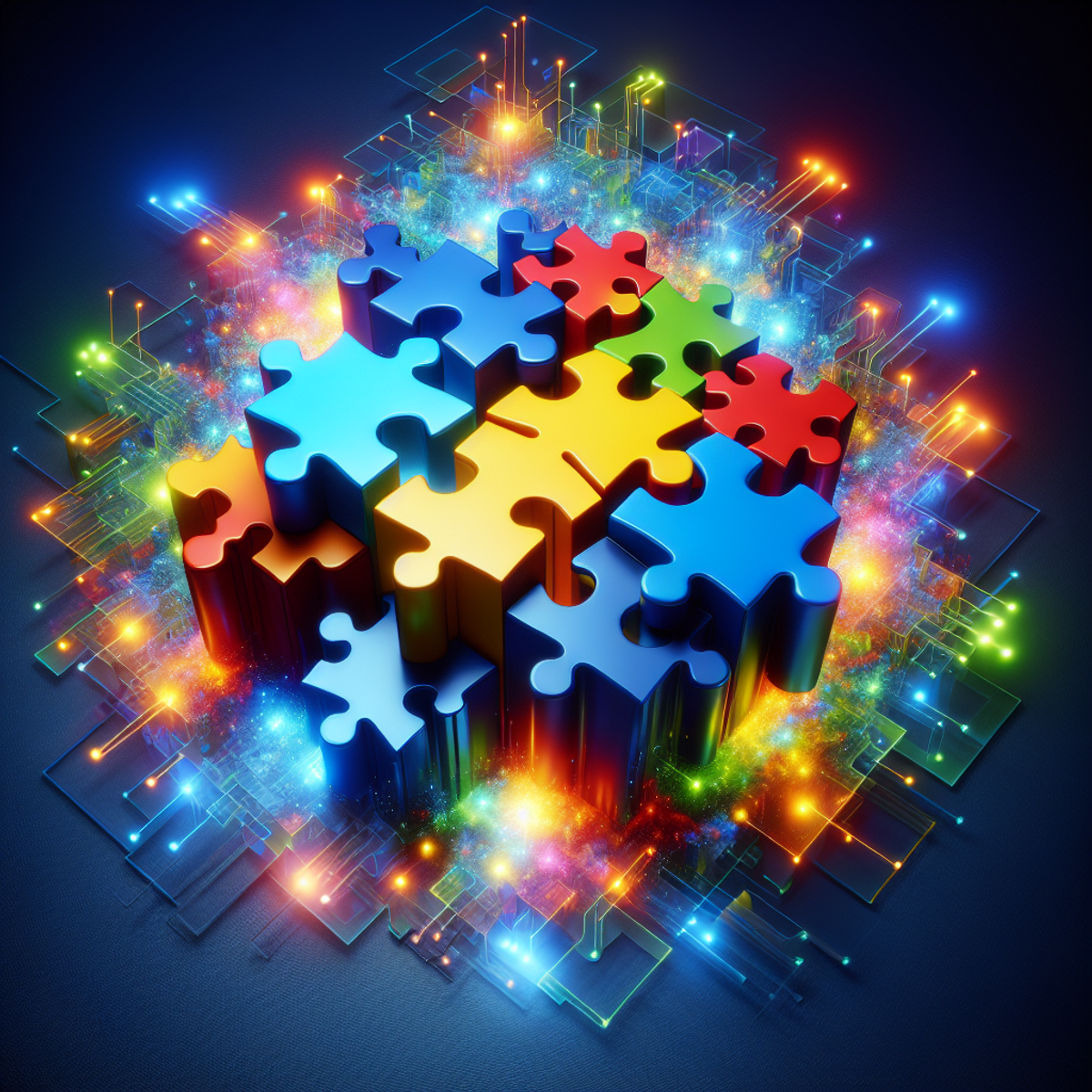
In Python development, managing project dependencies is a crucial aspect of efficient coding. Virtual environments provide a powerful tool for isolating and managing these dependencies, ensuring that your project has the necessary packages and versions without conflicts or compatibility issues. Here, we will explore what virtual environments are, how to create and activate them, and the benefits they offer in terms of dependency management. Virtual environments provide a way to create an isolated environment for a specific project, separate from the system-wide Python installation. This means that each project can have its own set of dependencies without interfering with other projects or the system itself. To create a virtual environment, you can use the built-in venv module in Python 3 or virtualenv for older versions. Once created, you can activate the virtual environment, which modifies your shell's PATH variable to use the isolated environment. This ensures that any package installations or Python commands are specific to that project. It also makes it easier to manage different versions of packages, as you can install them within the virtual environment without affecting other projects. By using virtual environments, you can ensure a consistent and reproducible development environment across different machines and collaborators. It also helps in avoiding conflicts between different versions of packages used in different projects, making it easier to maintain and update your codebase.
What are Virtual Environments?
Virtual environments are self-contained Python environments that allow you to install packages and dependencies specific to a particular project. They create an isolated space where you can have different versions of packages without interfering with each other. This isolation prevents conflicts between packages and ensures that your project runs consistently across different machines. Virtual environments are particularly useful when working on multiple projects that require different package versions. They allow you to easily switch between projects, activating the respective environment and ensuring that the correct dependencies are in place. This can be especially helpful when collaborating with others, as you can share your project's virtual environment configuration, enabling everyone to work with the same setup. Overall, virtual environments are an essential tool for any Python developer looking to create reliable, reproducible, and organized development environments.
Creating and Activating a Virtual Environment
To create a virtual environment in Python, you can use tools like virtualenv
or venv
. Here's a step-by-step guide on creating and activating a virtual environment using virtualenv
:
- Install
virtualenv
if you haven't already by runningpip install virtualenv
in your terminal. - Navigate to your project directory using the
cd
command. - Create a new virtual environment by running
virtualenv env_name
, whereenv_name
is the name you want to give to your virtual environment. - Activate the virtual environment by running the appropriate command for your operating system:
- For Windows:
.\env_name\Scripts\activate
- For macOS/Linux:
source env_name/bin/activate
Once activated, your terminal prompt should change to indicate that you are now working within the virtual environment.
Benefits of Virtual Environments
Virtual environments offer several benefits when it comes to managing project dependencies:
- Isolation: Each virtual environment provides an isolated space where packages can be installed without affecting other projects or the system-wide Python installation. This isolation prevents conflicts and allows you to have different versions of packages for different projects.
- Version Control: With virtual environments, you can easily specify the exact versions of packages required for your project. This ensures that your code runs consistently across different machines and avoids potential compatibility issues.
- Reproducibility: By using virtual environments, you can recreate the exact environment your project requires at any point in time. This makes it easier to share your code with others and ensures that everyone is working with the same dependencies.
Dependency Management Tools
When working with virtual environments, there are several popular dependency management tools available in the Python ecosystem:
1. pip
Pip is the default package installer for Python and is often used in conjunction with virtual environments. It allows you to install packages from the Python Package Index (PyPI) and other sources.
Best practices for specifying package versions include using version constraints in your requirements.txt
file or setup.py
file, such as specifying a minimum and maximum version range.2. Pipenv
Pipenv is a higher-level dependency management tool that combines pip and virtualenv functionality. It automatically creates and manages virtual environments for your projects, while also managing package dependencies through a Pipfile and Pipfile.lock.
Pipenv provides a more streamlined approach to dependency management by ensuring that all team members are using the same set of packages. It also allows for easy updates and resolution of conflicting dependencies.
2. conda
Conda is a cross-platform package manager that specializes in managing dependencies across various programming languages. It provides a more comprehensive solution for managing dependencies compared to pip, as it can handle not only Python packages but also packages from other programming languages. Conda also has its own environment management system, allowing you to easily create and manage virtual environments.
3. poetry
Poetry is a relatively new tool that aims to simplify dependency management and packaging in Python projects. It provides a cohesive workflow for managing dependencies, creating virtual environments, and building distributable packages.
These are just a few examples of the tools available for managing dependencies in Python. The choice of tool depends on your specific needs and preferences. Regardless of the tool you choose, incorporating virtual environments and dependency management practices into your workflow can greatly improve the reproducibility and maintainability of your code.
6. Conducting Code Reviews for Quality Assurance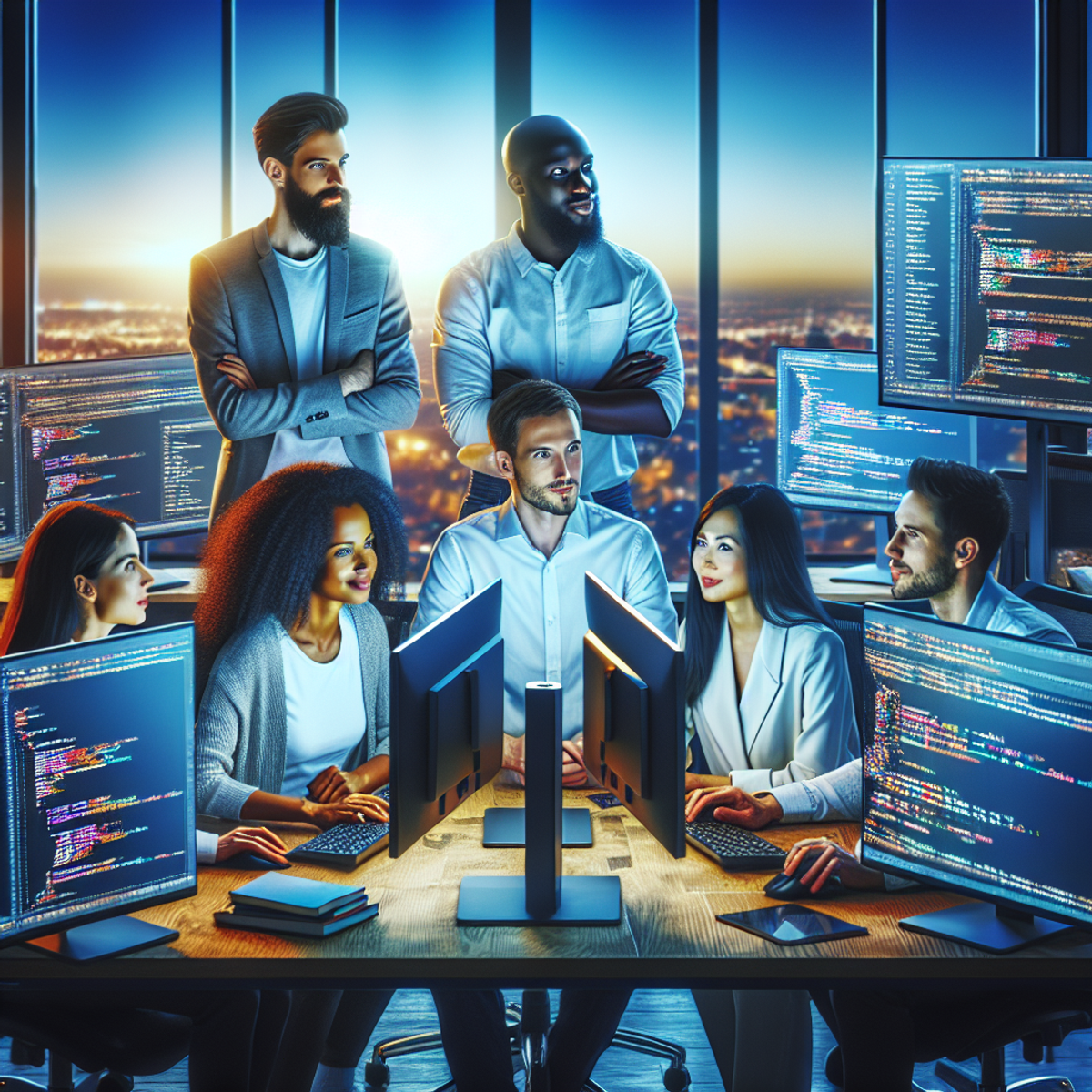
Code reviews are essential for maintaining high-quality standards and promoting collaborative coding practices within a development team. They allow team members to provide feedback, share knowledge, and ensure that the codebase meets quality expectations.
Why Code Reviews Are Important
When doing code reviews, it's important to highlight their role in ensuring overall code quality. Code reviews not only help identify and fix potential issues but also encourage continuous improvement and learning within the team. By sharing insights and best practices during code reviews, team members can collectively improve code quality and maintain consistency throughout the project.
What to Look for During a Code Review
During a code review, there are several key areas that should be carefully evaluated to ensure a thorough examination of the code:
- Code Style: Reviewers should check if the code follows the established style guidelines, such as PEP 8, Google Python Style Guide, or any specific conventions used by the team. Consistent adherence to coding standards improves readability and maintainability.
- Accuracy: It's crucial to verify that the code logic and functionality are correct. Reviewers need to make sure that the solution effectively addresses the requirements without introducing bugs or errors.
- Performance: Assessing the performance impact of code changes is important, especially in resource-intensive applications. Reviewers can suggest optimizations or alternative approaches to enhance performance when applicable.
- Security: Code reviews are an opportunity to identify potential security vulnerabilities or weaknesses in the implementation. Reviewers should examine input validation, data sanitization, and other security-critical aspects to mitigate risks.
Giving Constructive Feedback
Encouraging constructive feedback during code reviews is key to fostering a culture of continuous improvement. Reviewers should focus on providing actionable suggestions for improvement rather than just pointing out flaws. Additionally, acknowledging well-written sections of code reinforces positive practices and boosts morale within the team.
Sharing Knowledge
Code reviews are a platform for knowledge sharing among team members with different levels of experience. Experienced developers can share valuable insights with junior members, while fresh perspectives from newer team members may lead to innovative solutions and improved approaches.
Using Tools for Code Reviews
Using specialized tools for code reviews, such as GitHub's pull request feature or dedicated code review platforms like Gerrit or Phabricator, can streamline the review process and provide a centralized platform for discussions on specific lines of code.
By promoting collaboration and continuous learning through code reviews, development teams can enhance their collective coding skills and maintain a consistently high standard of code quality across projects.
To further improve overall code quality beyond the scope of code reviews, teams can also follow additional steps such as those discussed in this article on improving code quality which covers areas like automated testing, refactoring, and documentation best practices. pecific lines of code.
By promoting collaboration and continuous learning through code reviews, development teams can enhance their collective coding skills and maintain a consistently high standard of code quality across projects.
To further improve overall code quality beyond the scope of code reviews, teams can also follow additional steps such as those discussed in this article on improving code quality which covers areas like automated testing, refactoring, and documentation best practices.These practices can help address issues beyond what code reviews alone can achieve. Automated tests, for example, can catch bugs early on and ensure that changes in code don't introduce regressions. Refactoring allows for the optimization of existing code, making it cleaner and more maintainable. Documentation best practices ensure that future developers can easily understand the codebase, reducing confusion and speeding up development time. By incorporating these steps into their workflow, development teams can foster a culture of quality and reliability in their codebase.
Conclusion
Incorporating Python best practices into your coding workflow is essential for writing efficient and maintainable code. By following industry-standard guidelines and optimizing code performance and memory usage, you can ensure that your Python applications are of high quality and adhere to best practices.
To enforce consistent code style and catch potential issues early on, consider exploring linters and automated formatting tools like Black and flake8. These tools can help you maintain a clean, readable, and standardized codebase while minimizing errors and adhering to coding standards.
By implementing these best practices, you can elevate the quality of your Python code, improve its maintainability, optimize its performance, and enhance its security. Remember that efficient coding is not just about achieving functionality; it's also about writing code that is easy to understand, maintain, and scale in the long run.
FAQs (Frequently Asked Questions)
What are some key benefits of following Python best practices for writing efficient code?
Following Python best practices can lead to improved performance, better maintainability, and enhanced security of Python applications.
Why is writing clean and readable code important in Python?
Writing clean and readable code in Python is important for code comprehension, as it makes the code more understandable. It also helps in following essential formatting and syntax best practices, such as consistent indentation, appropriate use of white space, and following PEP 8 guidelines.
How can code organization contribute to maintainability and reusability in Python projects?
A well-organized structure can facilitate easier maintenance and encourage code reuse in Python projects. This can be achieved through the use of modules, packages, classes, separation of concerns, and encapsulation for reducing dependencies between different parts of the codebase.
Why is performance optimization important in Python programming?
Performance optimization is important in Python programming, particularly for tasks that involve large datasets or intensive computation. Strategies such as algorithmic improvements, caching, and using appropriate data structures can achieve faster execution times.
What are some best practices for ensuring code security in Python applications?
Writing secure code in Python applications is important to mitigate common vulnerabilities. Best practices include input validation, handling sensitive data, and protecting against common security threats like SQL injection and cross-site scripting (XSS).
What are virtual environments and why are they used in Python development?
Virtual environments are used in Python development to manage project dependencies. They provide a way to create an isolated environment for a project with its own dependencies, allowing different projects to have different dependencies even if they require the same package but with different versions.
Why are code reviews important in maintaining high code quality standards?
Code reviews are important in maintaining high code quality standards as they allow for constructive feedback and knowledge sharing among team members. Key aspects to consider during a code review include code style adherence, correctness, performance, and security implications.
Comments
Post a Comment