Optimizing Python Code for Performance
Optimizing Python Code for Performance
Introduction
Optimizing Python code for performance is extremely important. In today's fast-paced technology world, how efficient and fast your code runs can make a big difference in the success of your project. By improving the performance of your Python code, you can achieve:
- Faster execution times
- Reduced resource usage
- Better user experience
Importance of Optimizing Python Code for Performance
Optimizing Python code for performance is crucial for several reasons:
- Enhanced User Experience: Faster and more responsive applications lead to improved user satisfaction and engagement.
- Scalability: Well-optimized code is better equipped to handle increased workloads and larger datasets, enabling scalability.
- Resource Efficiency: Optimized code consumes fewer system resources, making it more cost-effective and environmentally friendly.
- Competitive Advantage: In today's competitive market, faster and more efficient software can provide a significant edge.
Benefits of Improving Code Performance
The benefits of improving code performance extend beyond mere speed enhancements:
- Cost Savings: Optimized code often requires fewer hardware resources, resulting in cost savings.
- Improved Maintainability: Well-structured and optimized code is easier to maintain and debug.
- Reduced Downtime: Faster code leads to reduced downtime and improved reliability.
- Energy Efficiency: Optimized code contributes to overall energy efficiency by reducing resource consumption.
This article aims to provide a comprehensive guide to optimizing Python code for performance. It will explore various techniques, tools, and best practices for enhancing the speed, efficiency, and resource utilization of Python applications. From identifying performance bottlenecks to implementing parallel processing and utilizing efficient data structures, each aspect of optimizing Python code will be thoroughly examined to equip you with the knowledge and skills necessary to elevate your coding practices.
Optimizing Python Code for Performance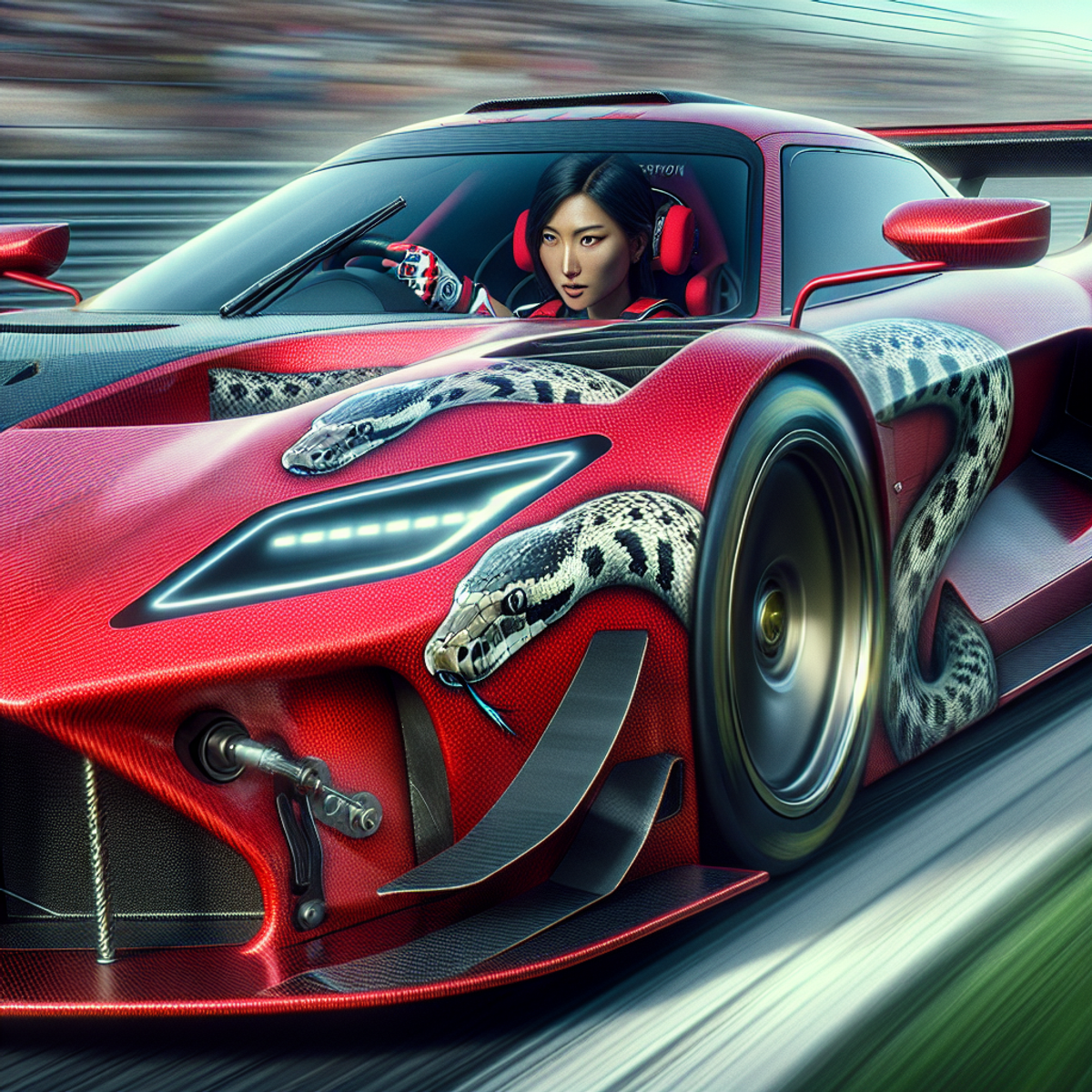
Optimizing Python code for performance is crucial in order to ensure efficient and fast execution of your programs. By improving the performance of your code, you can enhance the user experience, reduce resource consumption, and increase the scalability of your applications. In this section, we will explore various techniques and best practices for optimizing Python code.
Use Efficient Data Structures and Libraries
One of the key factors in optimizing code performance is selecting the right data structures and libraries. By utilizing efficient data structures, you can significantly improve the efficiency of your algorithms. Here are some considerations:
- Lists vs. Arrays: If you need to perform frequent insertions or deletions at arbitrary positions, consider using linked lists instead of arrays. Linked lists have constant-time insertions and deletions, while arrays have better random access time.
- Dictionaries: Take advantage of dictionaries when you need fast lookup times based on keys. Dictionaries use hash tables internally, providing constant-time average case complexity for insertion, deletion, and retrieval operations.
- Sets: Use sets when you need to efficiently check for membership or remove duplicates from a collection. Sets offer constant-time average case complexity for these operations.
In addition to utilizing efficient data structures, leveraging optimized libraries for specific tasks can also improve code performance. Some popular libraries include:
- NumPy: NumPy provides fast array processing capabilities, enabling efficient numerical computations in Python.
- Pandas: Pandas offers high-performance data manipulation and analysis tools, making it ideal for working with large datasets.
- SciPy: SciPy provides a collection of scientific computing functions and algorithms that are optimized for performance.
Optimize Algorithm Efficiency
Another crucial aspect of optimizing code performance is improving the efficiency of your algorithms. By analyzing the time and space complexity of your algorithms, you can identify potential bottlenecks and make necessary optimizations.
Consider the following techniques to optimize algorithm efficiency:
- Reduce time complexity: Aim to reduce the time complexity of your algorithms by utilizing more efficient algorithms or implementing algorithmic optimizations. For example, if you have a nested loop with quadratic time complexity, consider using a more efficient algorithm with linear or logarithmic time complexity.
- Optimize space usage: Analyze the space complexity of your algorithms and look for opportunities to reduce memory consumption. This could involve reusing variables, minimizing unnecessary data structures, or employing dynamic programming techniques.
Minimize Memory Usage and Optimize Allocation
Memory management plays a significant role in code performance. By understanding how Python manages memory and minimizing unnecessary memory usage, you can improve the efficiency of your code. Here are some tips to consider:
- Avoid excessive object creation: Creating unnecessary objects can lead to increased memory usage and decreased performance. Instead, reuse objects whenever possible.
- Use generators and iterators: Generators and iterators are memory-efficient alternatives to creating large lists or arrays. They allow you to process data one element at a time, reducing memory overhead.
- Implement lazy loading: If you're working with large datasets or files, consider implementing lazy loading techniques. This allows you to load data into memory only when it is needed, optimizing memory allocation.
Optimize Loops and Iterations
Loops and iterations are common in Python code and can often be optimized for improved performance. Consider the following tips:
- Avoid unnecessary iterations: Review your code for any redundant or unnecessary iterations and eliminate them if possible. This can be achieved by refactoring your code or utilizing built-in functions that operate on entire collections.
- Use list comprehensions: List comprehensions provide a concise way to create lists based on existing collections. They are often more efficient than traditional loops as they minimize function call overhead.
- Leverage vectorized operations: When working with arrays or matrices, take advantage of vectorized operations provided by libraries like NumPy. These operations are implemented in optimized C code, resulting in faster execution times compared to traditional element-wise operations.
By implementing these optimization techniques, you can significantly improve the performance of your Python code.
Understanding Python Code Performance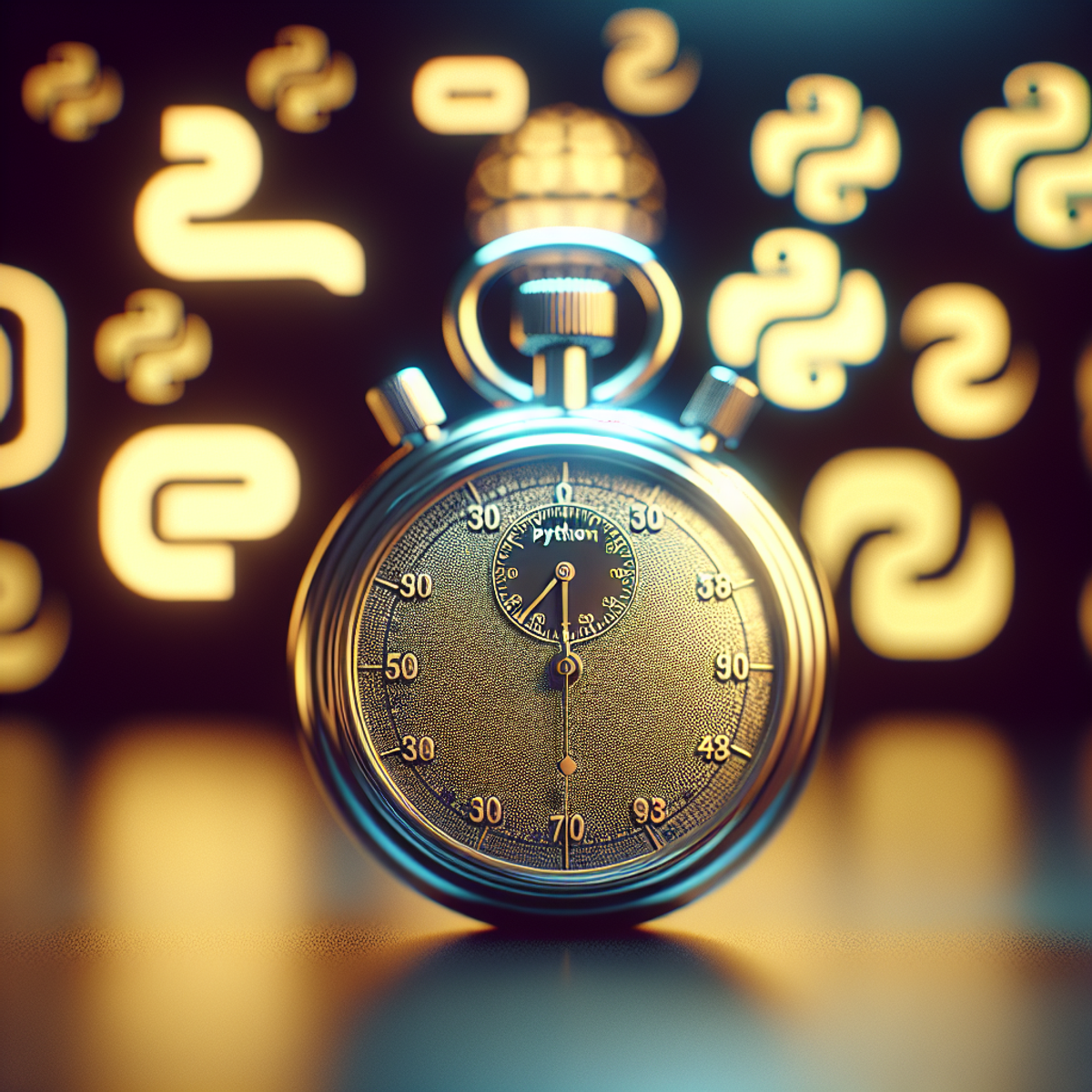
Python code performance is a critical aspect of software development, directly impacting the efficiency and responsiveness of applications. Understanding the factors that influence Python code performance, identifying common bottlenecks, and utilizing appropriate tools for measurement and analysis are essential for optimizing code performance.
Factors that Affect Python Code Performance
Several key factors can significantly impact the performance of Python code, including:
- Algorithm Efficiency: The choice and implementation of algorithms directly affect the speed and resource consumption of code execution.
- Memory Management: Inefficient memory usage and allocation can lead to performance degradation, especially in memory-intensive applications.
- Data Structures and Libraries: The selection of appropriate data structures and optimized libraries can greatly influence code performance.
- Loops and Iterations: Inefficient looping constructs and iteration techniques can introduce unnecessary overhead.
Common Performance Bottlenecks in Python Code
When developing Python applications, it's crucial to be mindful of common performance bottlenecks that can impede efficient code execution. Some prevalent issues include:
- Inefficient Algorithmic Complexity: Algorithms with high time or space complexity can lead to slow performance, particularly when dealing with large datasets.
- Excessive Memory Usage: Improper memory management and unnecessary memory allocation can result in increased memory consumption and reduced performance.
- Suboptimal Data Structures: The use of inappropriate data structures for specific tasks can hinder overall code efficiency.
- Unoptimized Loops and Iterations: Loops that are not structured efficiently or contain redundant operations can contribute to performance bottlenecks.
Tools for Measuring and Analyzing Code Performance
To accurately assess the performance of Python code, developers can utilize a range of tools designed for measuring and analyzing various aspects of code execution. Some commonly employed tools include:
- Profiling Tools: Profilers such as cProfile and line_profiler offer insights into the execution time of different functions within the code, aiding in the identification of performance bottlenecks.
- Memory Profilers: Tools like memory_profiler enable developers to track memory usage during program execution, helping identify areas where memory optimization is necessary.
- Code Linters: While not specifically designed for performance analysis, linters such as Pylint can help identify inefficient coding practices that may impact overall performance.
By understanding these factors, identifying common bottlenecks, and leveraging appropriate tools for measurement and analysis, developers can gain valuable insights into their Python code's performance characteristics, paving the way for effective optimization strategies.
Profiling Python Code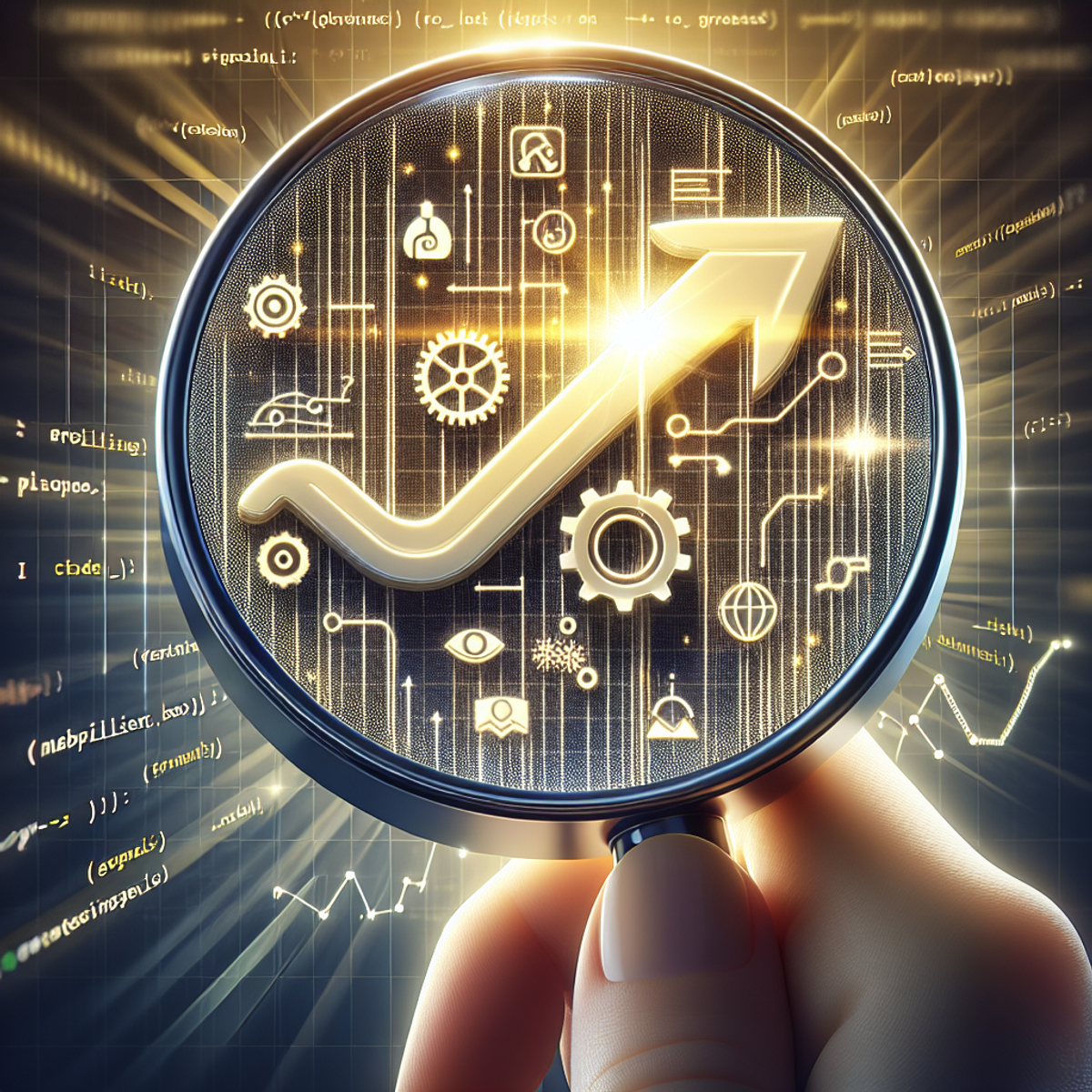
Code profiling is a crucial aspect of optimizing Python code for performance. It involves analyzing the code's behavior and performance to identify bottlenecks and areas for improvement. Here are the key points to consider when profiling Python code:
What is Code Profiling
Profiling provides insight into how much time is spent in different parts of the code, allowing you to focus optimization efforts where they will have the most impact. It helps in identifying which functions or methods consume the most time and resources during execution.
Different Types of Profilers Available for Python
Python offers various profilers to analyze code performance, such as:
- cProfile: A built-in profiler that provides deterministic profiling of Python programs.
- line_profiler: A line-by-line profiler for identifying time-consuming operations in specific lines of code.
- memory_profiler: A tool for monitoring memory usage, enabling identification of memory-intensive sections within the code.
How to Use Profilers to Identify Performance Bottlenecks
When using profilers, it's essential to run representative workloads that simulate real-world usage scenarios. The insights gained from profiling can be used to prioritize optimization efforts and make informed decisions about where to focus improvements.
Profiling can reveal opportunities for algorithmic optimizations, inefficient memory usage, or areas where parallel processing or caching techniques could be beneficial. By systematically analyzing the code's performance, you can target specific areas for enhancement, leading to significant improvements in overall efficiency.
By leveraging the capabilities of different profilers and interpreting their output effectively, you can gain a comprehensive understanding of your code's performance characteristics. This empowers you to make informed decisions about which aspects of your codebase need attention, ensuring that optimization efforts are focused on areas that will yield the greatest impact.
Optimizing Algorithm Efficiency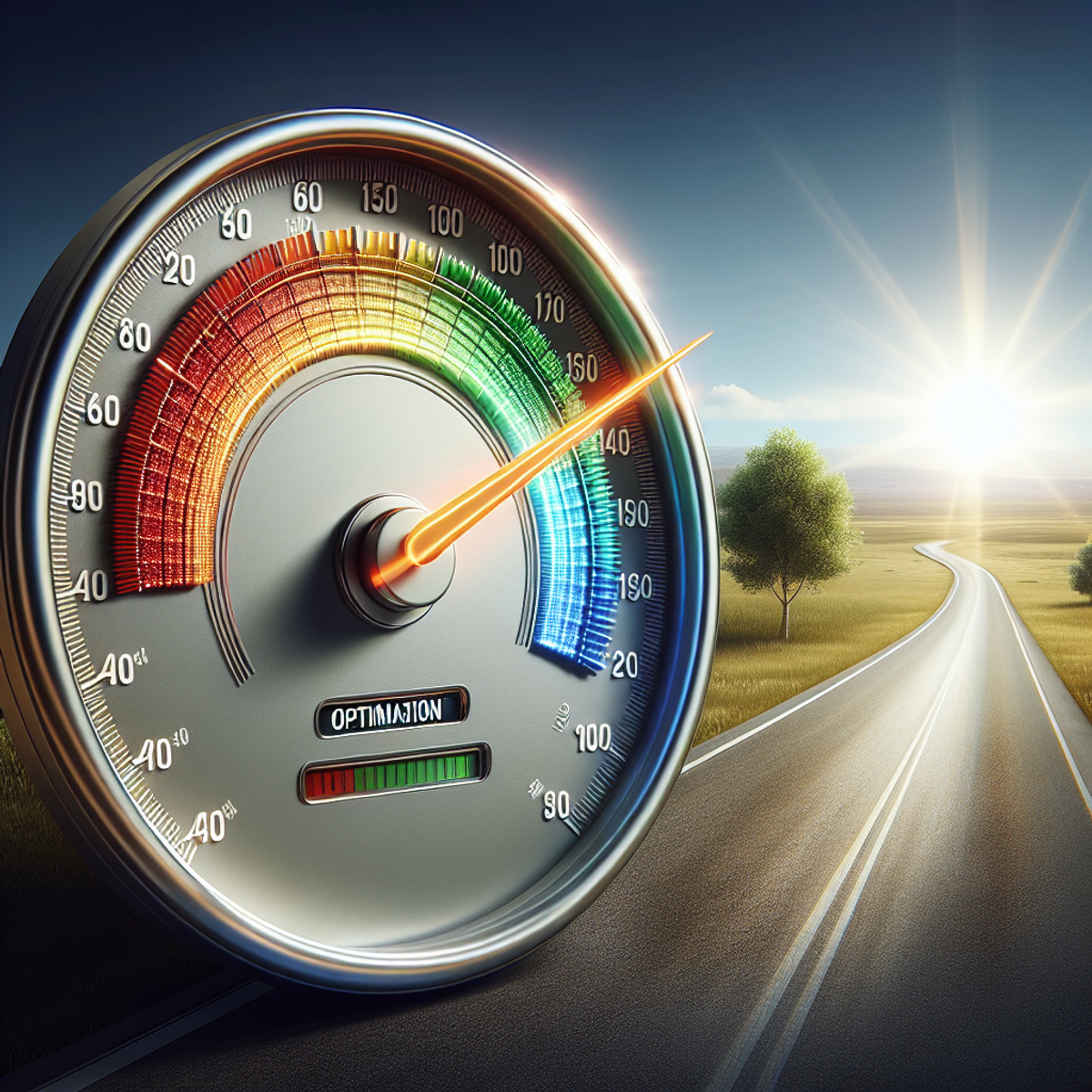
When it comes to optimizing Python code for performance, one of the key areas to focus on is algorithm efficiency. By improving the efficiency of your algorithms, you can significantly enhance the overall performance of your code. In this section, we will explore the importance of efficient algorithms, how to analyze their time and space complexity, and techniques for optimizing algorithm efficiency.
Importance of Efficient Algorithms
Efficient algorithms play a crucial role in improving code performance. They can help reduce execution time, minimize resource usage, and enhance scalability. By optimizing your algorithms, you can make your code more responsive, handle larger datasets efficiently, and improve the overall user experience.
Analyzing Time and Space Complexity
To optimize algorithm efficiency, it is important to understand the time and space complexity of your algorithms. Time complexity refers to how the execution time of an algorithm grows as the input size increases. Space complexity, on the other hand, refers to how much memory an algorithm requires to run.
Analyzing time and space complexity allows you to identify potential bottlenecks in your code and determine areas for improvement. It helps you understand how different algorithms perform under various scenarios and choose the most suitable one for your specific use case.
Techniques for Optimizing Algorithm Efficiency
There are several techniques you can employ to optimize algorithm efficiency:
- Choose the right algorithm: Selecting the most appropriate algorithm for a given problem can have a significant impact on performance. Consider factors such as input size, expected output, and any constraints or requirements.
- Reduce unnecessary operations: Identify and eliminate any unnecessary calculations or operations within your algorithms. Simplify complex expressions and remove redundant steps wherever possible.
- Use data structures efficiently: Utilize efficient data structures such as dictionaries, sets, or arrays to store and manipulate data effectively. Choosing the right data structure can greatly improve algorithm efficiency.
- Implement memoization: Memoization is a technique that involves caching the results of expensive function calls and reusing them when the same inputs occur again. This can help avoid redundant computations and significantly speed up execution.
- Optimize loops and iterations: Minimize the number of iterations in your code by optimizing loops. Consider using techniques like loop unrolling or loop fusion to reduce overhead.
By applying these techniques, you can optimize the efficiency of your algorithms and improve the overall performance of your Python code.
Improving Memory Usage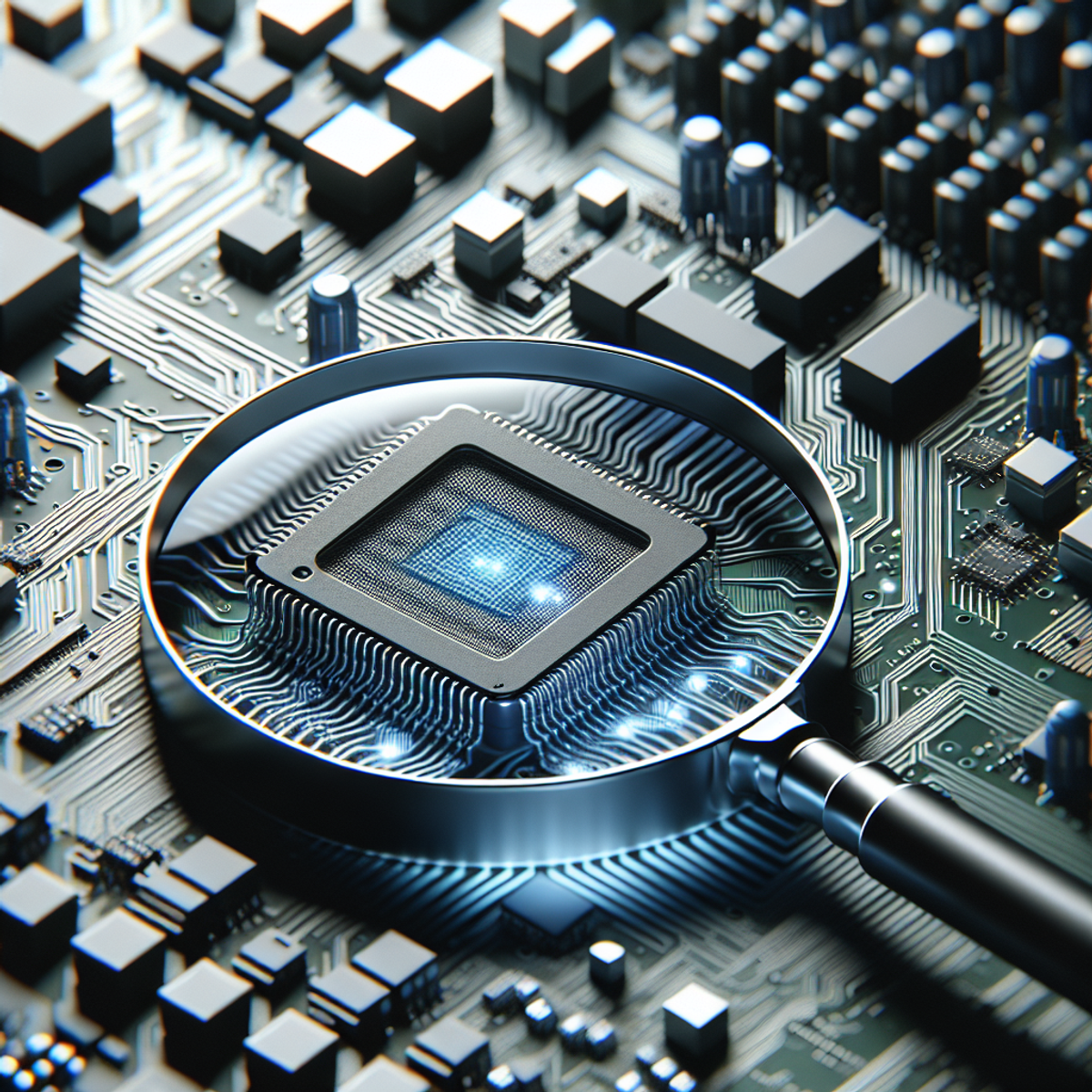
Efficient memory usage is crucial for optimizing the performance of Python code. In this section, we will explore different aspects of memory management in Python, common memory issues that affect code performance, and techniques for reducing memory usage and optimizing memory allocation.
Understanding Memory Management in Python
Python manages memory using its private heap space, where it stores all objects and data structures. The Python memory manager handles the allocation and deallocation of this heap space for Python objects. Understanding how memory works in Python is important for optimizing memory usage.
Common Memory Issues in Python Code
Several common memory issues can impact the performance of Python code:
- Memory Leaks: When objects are not deallocated after use, leading to a gradual increase in memory consumption.
- Excessive Memory Allocation: Unnecessary creation of large objects or data structures that consume more memory than necessary.
- Inefficient Data Structures: Using inefficient data structures that occupy more memory than required.
- High Memory Fragmentation: Fragmented memory allocation due to frequent object creation and deletion.
Identifying and addressing these issues can significantly improve the overall memory usage and performance of Python code.
Techniques for Reducing Memory Usage and Optimizing Memory Allocation
1. Use Generators
Generators are an efficient way to generate sequences of data without storing them in memory all at once. By using generators, you can reduce the memory footprint of your code, especially when dealing with large datasets or infinite sequences.
2. Implement Lazy Evaluation
Lazy evaluation postpones the evaluation of an expression until its value is actually needed. This technique can be particularly useful when working with complex computations or processing large amounts of data, as it helps minimize unnecessary memory allocation.
3. Optimize Data Structures
Choosing the right data structure based on the specific requirements of your code can greatly impact memory usage. For example, using sets instead of lists for unique collections or employing dictionaries for key-value mappings can optimize memory usage.
4. Avoid Unnecessary Object Instantiation
Frequent creation of unnecessary objects can lead to increased memory consumption. Reusing objects where possible and minimizing object instantiation can help reduce overall memory usage.
5. Memory Profiling and Analysis
Utilize tools for profiling and analyzing memory usage to identify areas of improvement. Tools like memory_profiler
can provide insights into the memory consumption patterns of your code, allowing you to make targeted optimizations.
By implementing these techniques and being mindful of efficient memory management practices, you can effectively reduce the memory footprint of your Python code while improving its overall performance.
Efficient Data Structures and Libraries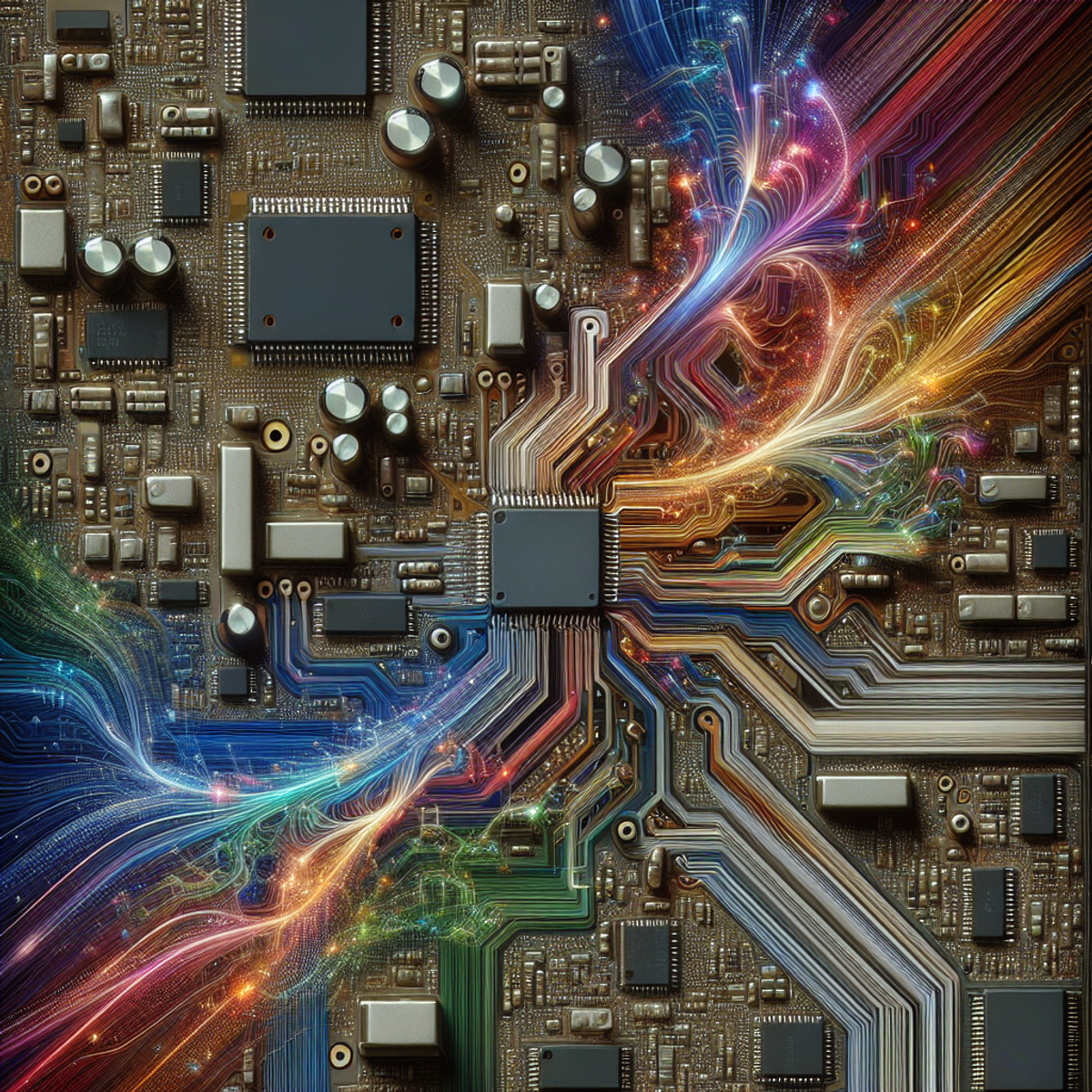
Efficient data structures and optimized libraries are essential for improving the performance of Python code. They help optimize memory usage, reduce execution time, and enhance overall program efficiency. In this section, we will explore the benefits of using efficient data structures and optimized libraries in Python.
Overview of Efficient Data Structures in Python
Python offers a variety of data structures that are designed to optimize specific operations and improve performance. Some commonly used efficient data structures in Python include:
- Lists: Python lists are dynamic arrays that allow for fast appends and pops at the end. They are commonly used when the order of elements matters.
- Tuples: Tuples are immutable sequences that provide fast access to elements. They are ideal for situations where you need to store related values together.
- Sets: Sets are unordered collections of unique elements. They offer fast membership tests and efficient set operations such as union, intersection, and difference.
- Dictionaries: Dictionaries are key-value pairs that provide fast lookup based on keys. They are particularly useful when you need to access elements by their unique identifier.
Benefits of Using Optimized Libraries for Specific Tasks
Python has a wide range of optimized libraries that are specifically designed to handle various tasks efficiently. These libraries use highly optimized algorithms and data structures to deliver superior performance. Here are some benefits of using optimized libraries in Python:
- Faster Execution: Optimized libraries often implement algorithms that have been fine-tuned for speed. Using these libraries can significantly speed up your code execution, especially for computationally intensive tasks.
- Memory Efficiency: Optimized libraries use memory-efficient data structures and techniques to minimize memory usage. This can be particularly advantageous when dealing with large datasets or limited memory environments.
- Specialized Functionality: Many optimized libraries provide specialized functions tailored for specific tasks such as numerical computation, data manipulation, machine learning, or image processing. These functions are often highly optimized and can yield substantial performance improvements over writing custom code.
Examples of Optimized Data Structures and Libraries in Python
Python has a wide range of optimized libraries that cater to various domains. Some popular examples include:
- NumPy: NumPy is a fundamental library for scientific computing in Python. It provides efficient multi-dimensional arrays, mathematical functions, and tools for working with large datasets.
- Pandas: Pandas is a powerful library for data manipulation and analysis. It offers high-performance data structures like DataFrame and Series, along with efficient methods for handling missing data, merging datasets, and performing aggregations.
- SciPy: SciPy is a library that builds upon NumPy and provides additional functionality for scientific computing. It includes modules for optimization, interpolation, signal processing, linear algebra, and more.
- Dagster: Dagster is another library that focuses on high-performance Python programming. It provides abstractions for building scalable data applications and leverages modern software engineering practices to achieve optimal performance.
By using these optimized data structures and libraries in your Python code, you can achieve significant performance gains and enhance the efficiency of your programs.
Optimizing Loops and Iterations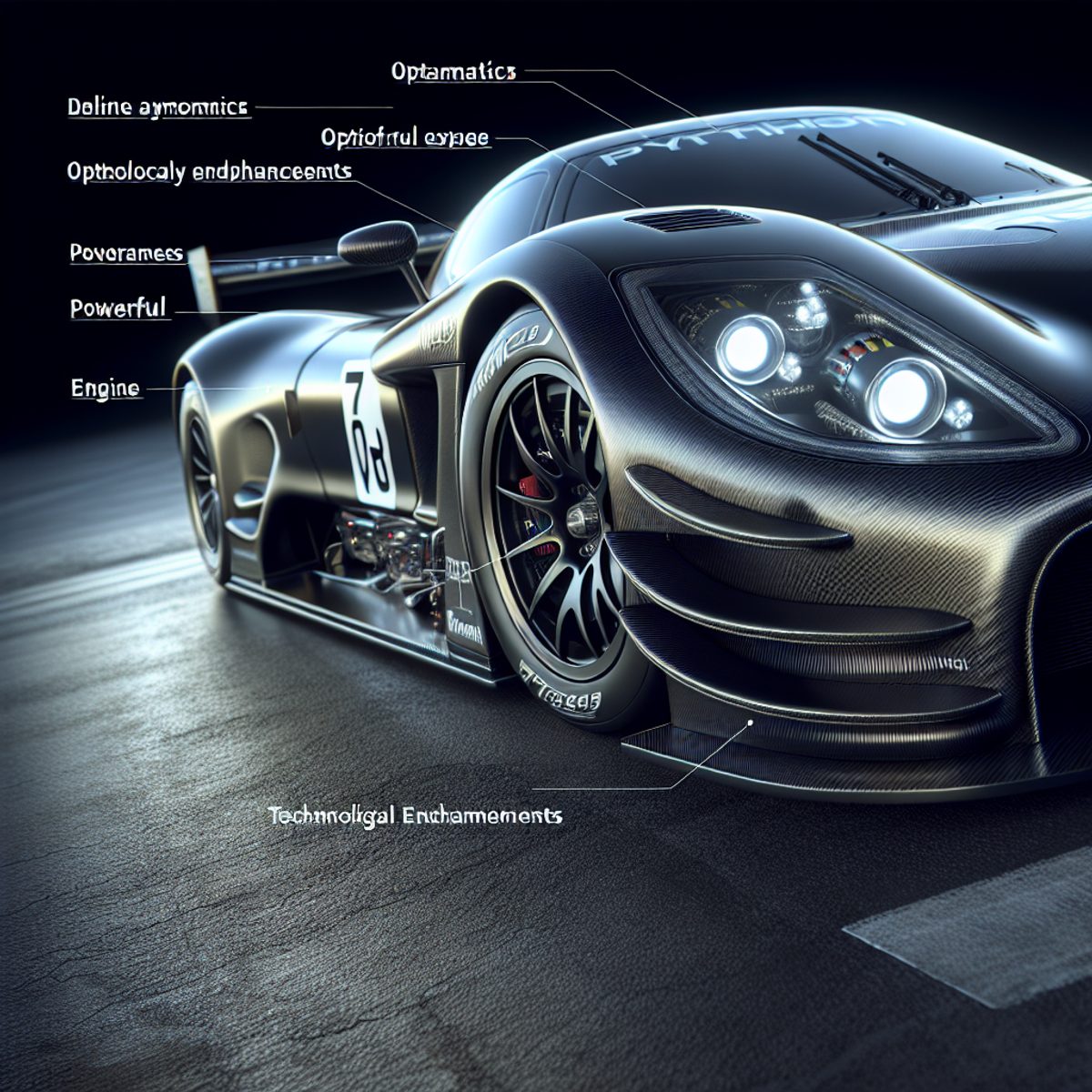
Loops and iterations are fundamental in Python programming, but they can also become a source of performance bottlenecks if not optimized properly. In this section, we will explore common pitfalls in loops and iterations that affect performance and discuss techniques for optimizing them in Python code.
Common Pitfalls in Loops and Iterations
- Unnecessary Computations: Performing unnecessary computations within a loop can significantly slow down the execution time. It is essential to carefully analyze the logic inside the loop and identify any calculations that can be moved outside to reduce redundant operations.
- Inefficient Data Structures: Choosing the wrong data structure for storing and accessing data in a loop can lead to poor performance. For example, using a list when a set or dictionary would be more efficient for membership checks or lookups can cause unnecessary overhead.
- Excessive Function Calls: Calling functions repeatedly within a loop can be costly, especially if the function has significant overhead. Whenever possible, try to minimize function calls or consider using inline functions or lambda expressions to avoid the overhead associated with repeated function invocations.
Techniques for Optimizing Loops and Iterations
- Loop Unrolling: Loop unrolling is a technique where multiple loop iterations are combined into a single iteration, reducing the loop overhead. This technique is particularly useful when the number of iterations is known in advance. However, it should be used with caution as unrolling too much can result in increased code size and decreased cache efficiency.
- Vectorization: Vectorization involves performing operations on entire arrays or sequences instead of individual elements, utilizing specialized libraries like NumPy or pandas. This technique leverages optimized C/C++ code under the hood, leading to significant speed improvements compared to traditional loop-based computations.
- Generator Expressions: Instead of creating intermediate lists or sequences within a loop, consider using generator expressions. Generator expressions are memory-efficient and allow for lazy evaluation, which can improve performance by reducing memory consumption and unnecessary computation.
- Caching: If the loop involves repetitive calculations or function calls with the same inputs, consider caching the results to avoid redundant computations. Caching can be implemented using techniques like memoization or utilizing libraries like functools.lru_cache.
Remember, the optimization techniques mentioned above should be applied judiciously based on the specific requirements of your code. Profiling and benchmarking can help identify critical areas for optimization and evaluate the effectiveness of different approaches.
By optimizing loops and iterations in your Python code, you can often achieve significant performance improvements and make your code more efficient overall.
Utilizing Built-in Functions and Modules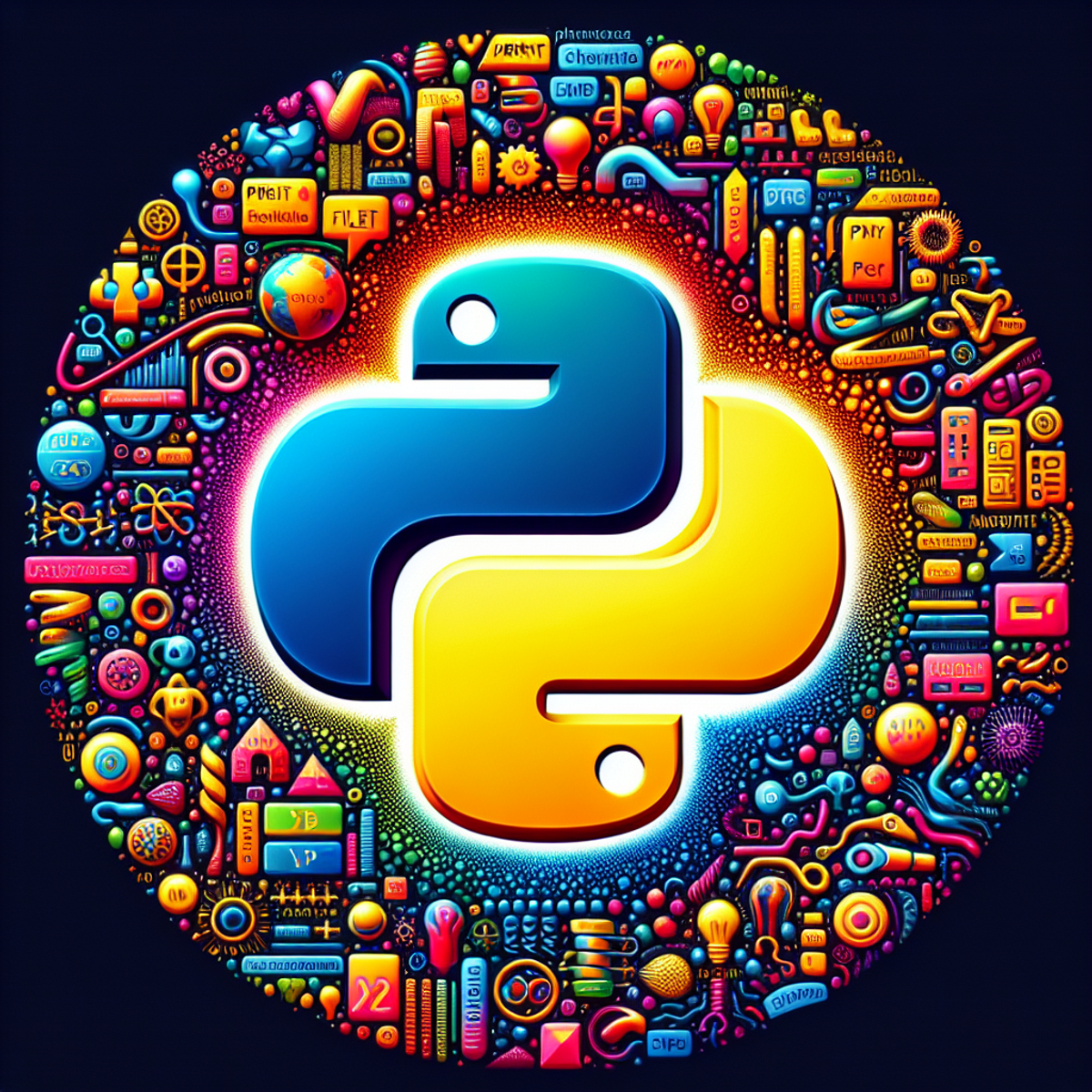
The use of built-in functions and modules in Python can greatly enhance the performance of your code. By leveraging these pre-existing resources, you can streamline your code and improve its efficiency. Let's delve into the benefits and common practices associated with utilizing built-in functions and modules for optimized performance.
Benefits of using built-in functions and modules for improved performance
- Optimized code execution: Built-in functions and modules are typically implemented in highly optimized C code, resulting in faster execution compared to equivalent Python code. This PythonSpeed/PerformanceTips guide provides more insights on optimizing Python code.
- Reduced development time: Leveraging built-in functions and modules allows you to avoid reinventing the wheel, saving time on implementing common functionalities. For example, beginners in numerical computing can refer to this absolute beginners guide for using the popular
numpy
module. - Enhanced readability: Utilizing well-known built-in functions and modules can enhance the readability of your code, making it more understandable for other developers. This is especially important for commonly used concepts such as Python function recursion, which can be better comprehended when written using built-in functions.
Commonly used built-in functions and modules for specific tasks
- Mathematical operations: The
math
module provides a wide range of mathematical functions, such as trigonometric, logarithmic, and exponential functions, contributing to optimized mathematical computations. - String manipulation: Built-in string methods like
split()
,join()
, andreplace()
offer efficient ways to manipulate strings without the need for custom implementations. - Data aggregation: Functions like
sum()
,max()
, andmin()
enable efficient aggregation of data without explicit looping, improving code performance. These techniques are also applicable in more advanced data processing frameworks like PySpark, where large-scale data aggregation tasks can benefit from the built-in functions provided by the framework.
By incorporating these built-in functions and modules into your Python code, you can harness their performance benefits while reducing development effort. This approach aligns with Python's philosophy of emphasizing simplicity and readability, all while optimizing code execution.
Parallel Processing and Multithreading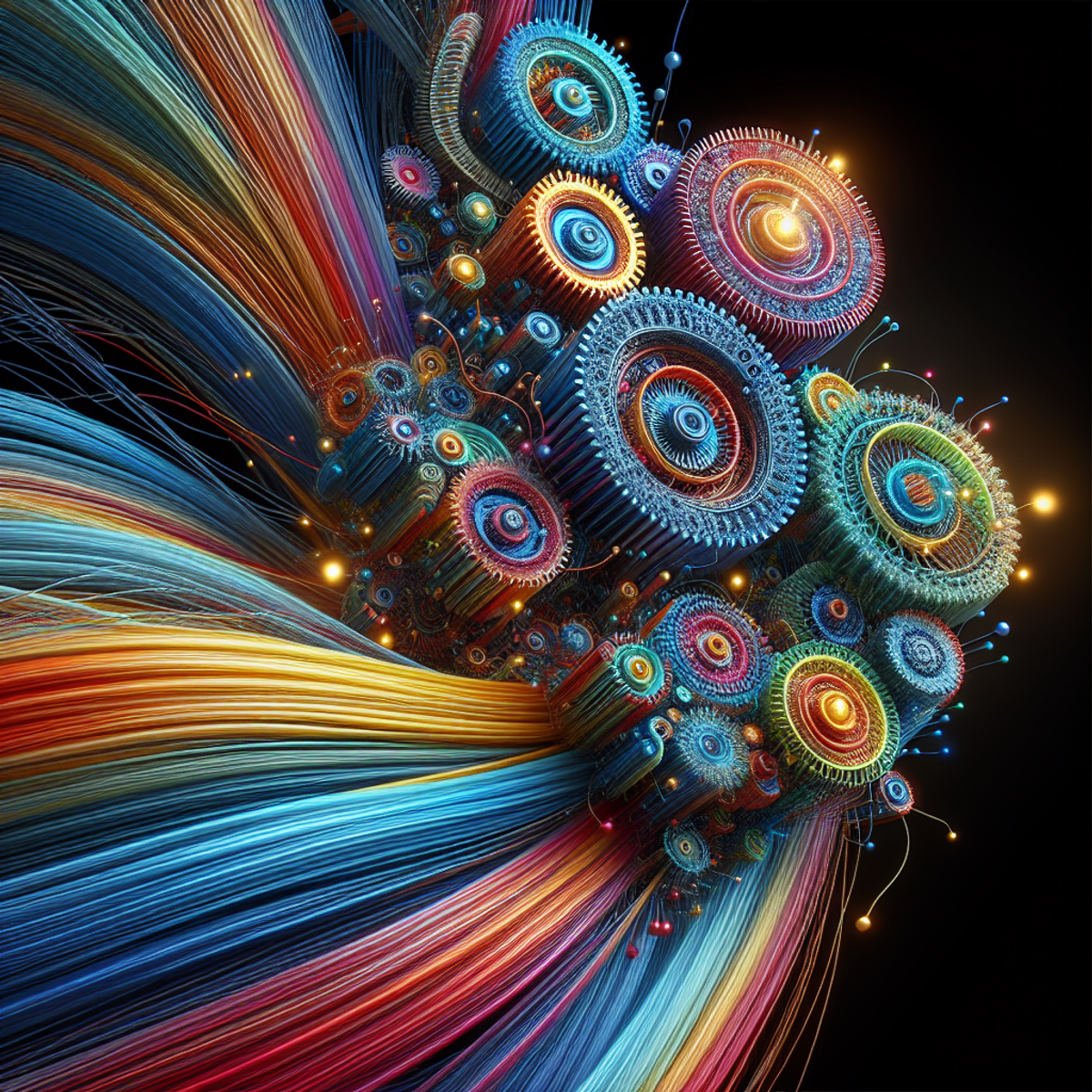
Parallel processing and multithreading are powerful techniques for optimizing the performance of Python code. They allow you to execute multiple tasks simultaneously, taking advantage of the capabilities of modern processors. In this section, we will explore the benefits and considerations of using parallel processing and multithreading in Python, as well as techniques for optimizing performance.
Overview of Parallel Processing and Multithreading in Python
- Parallel processing involves dividing a task into smaller subtasks that can be executed concurrently on different processors or cores. It enables you to take advantage of the full processing power of your machine.
- Multithreading is a technique where multiple threads within a single program execute concurrently, sharing the same memory space. Each thread performs a specific task, allowing for improved performance and responsiveness.
- Python provides several modules for parallel processing and multithreading, including
multiprocessing
,concurrent.futures
, andthreading
. These modules simplify the implementation of parallelism and concurrency in your code.
Benefits and Considerations for Using Parallel Processing
Benefits:
- Improved performance: Parallel processing allows you to distribute the workload across multiple processors or cores, reducing the overall execution time of your code.
- Increased scalability: By utilizing parallel processing, you can scale your code to handle larger datasets or more complex computations without sacrificing performance.
- Asynchronous execution: Parallel processing enables asynchronous execution, where tasks can run independently without waiting for each other to complete. This can significantly improve the efficiency of your code.
Considerations:
- Overhead: Implementing parallel processing introduces additional overhead due to communication between processes or threads. This overhead may impact performance if not managed properly.
- Shared resources: When multiple processes or threads access shared resources, such as memory or files, synchronization mechanisms must be employed to avoid conflicts and ensure data integrity.
- GIL limitations: The Global Interpreter Lock (GIL) in CPython limits the execution of Python bytecode to a single thread. This means that parallel processing is not as effective for CPU-bound tasks in CPython, but it can still provide benefits for I/O-bound tasks or when using alternative Python implementations such as Jython or IronPython.
Techniques for Optimizing Performance using Parallel Processing and Multithreading
To optimize performance when using parallel processing and multithreading in Python, consider the following techniques:
- Task granularity: Divide your task into smaller subtasks with appropriate granularity. Fine-grained tasks may incur more overhead due to communication, while coarse-grained tasks may not fully utilize the available resources.
- Load balancing: Distribute the workload evenly across processes or threads to avoid situations where some resources are idle while others are overloaded. Load balancing techniques, such as work stealing or task queues, can help achieve this balance.
- Efficient synchronization: When multiple processes or threads access shared resources, use synchronization mechanisms such as locks, semaphores, or condition variables to prevent race conditions and ensure data consistency.
- I/O operations: Parallel processing and multithreading can be particularly beneficial for I/O-bound tasks, where waiting for I/O operations can be overlapped with other computations. Consider using asynchronous I/O libraries like
asyncio
ortwisted
to maximize concurrency. - Utilize multiprocessing.Pool: The
multiprocessing.Pool
class provides a convenient interface for distributing tasks across multiple processes. It abstracts away many of the complexities
Caching and Memoization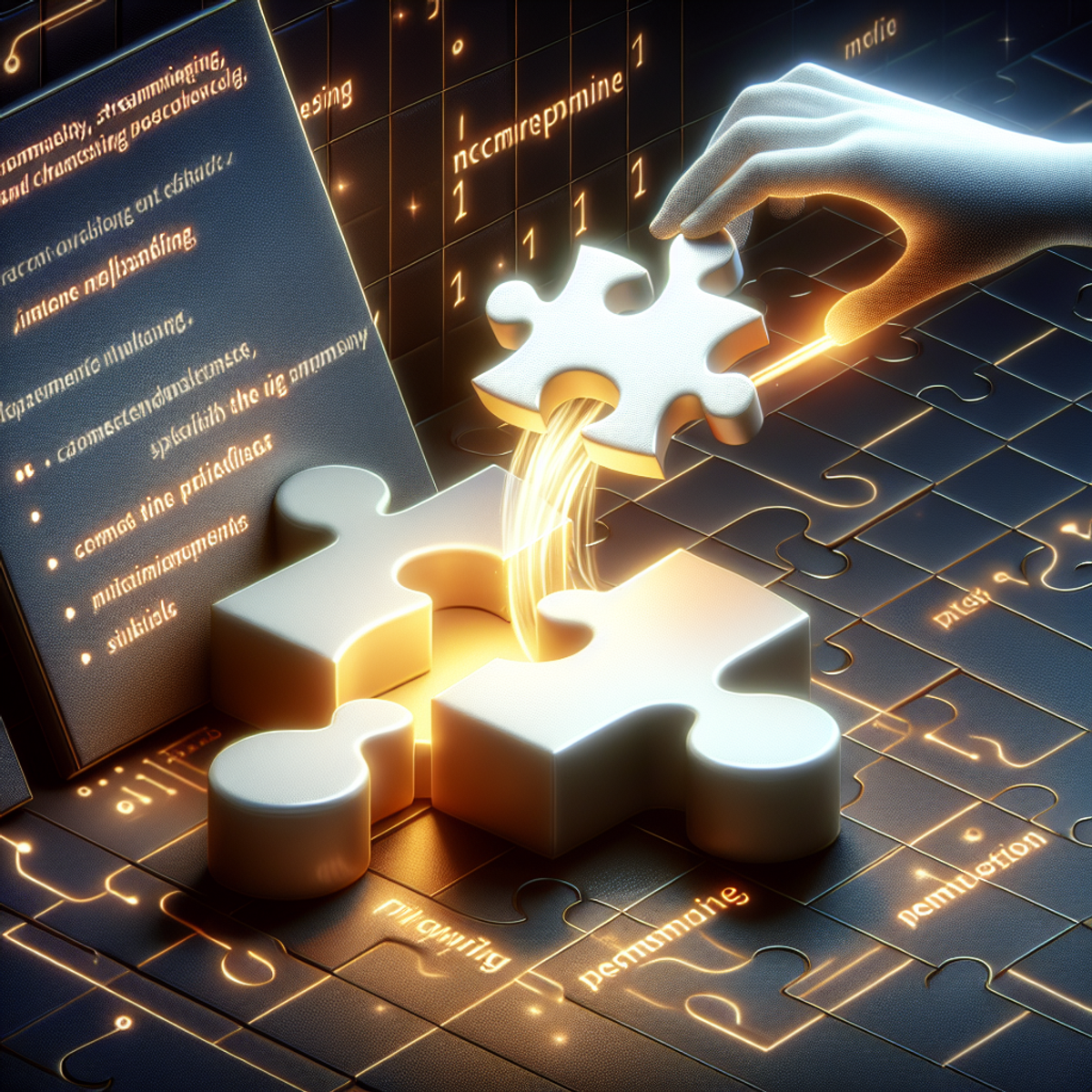
Caching and memoization are techniques used to optimize the performance of Python code by storing and reusing computed results. By avoiding unnecessary calculations, these techniques can significantly improve the efficiency of repetitive or computationally expensive operations. Let's explore what caching and memoization are, their benefits in improving performance, and how to implement them in Python code.
What is Caching and Memoization?
Caching and memoization are both strategies that involve storing previously computed results for future use. While they serve a similar purpose, there is a subtle difference between the two:
- Caching: Caching involves storing the result of a function or operation based on its inputs. The result is associated with a specific set of inputs, known as a cache key. When the function is invoked with the same inputs again, instead of recalculating the result, it is retrieved from the cache.
- Memoization: Memoization is a specific form of caching that focuses on caching the return value of a function based on its inputs. It is typically used for functions that are pure, meaning their output solely depends on their input and has no side effects. When a memoized function is called with the same arguments again, it returns the cached result instead of executing the function body.
Benefits of Caching and Memoization in Improving Performance
Implementing caching and memoization techniques in Python code can bring several benefits:
- Reduced computation time: By storing previously computed results, caching and memoization avoid redundant computations. This can lead to significant time savings, especially in scenarios where expensive calculations or complex algorithms are involved.
- Improved responsiveness: Caching and memoization can enhance program responsiveness by eliminating delays caused by repetitive calculations. With cached results readily available, subsequent invocations of functions or operations can return results more quickly.
- Optimized resource usage: By reusing computed results, caching and memoization can help conserve system resources such as CPU cycles and memory. This can lead to better overall performance and scalability, especially in resource-constrained environments.
Implementing Caching and Memoization Techniques in Python Code
Python provides several ways to implement caching and memoization techniques. Here are a few popular approaches:
Using a dictionary
A simple way to implement caching is by using a dictionary to store the computed results. The function arguments can be used as keys, and the corresponding results can be stored as values. Whenever the function is called, we first check if the result is present in the cache. If so, we return it; otherwise, we compute the result and store it in the cache for future use.
python cache = {}
def expensive_function(n): if n in cache: return cache[n]
# Perform expensive calculations result = ... cache[n] = result return result
Using the functools.lru_cache
decorator
The functools
module in Python's standard library provides the lru_cache
decorator, which stands for "Least Recently Used Cache." Applying this decorator to a function automatically adds caching behavior based on its input arguments. The decorator uses an LRU eviction policy to limit the size of the cache.
python from functools import lru_cache
@lru_cache(maxsize=None) def expensive_function(n): # Perform expensive calculations result = ...
return result
Using third-party libraries
There are also third-party libraries available that offer more advanced caching and memoization capabilities. One popular library is cachetools
, which provides additional features like cache expiration policies and support for different storage backends.
python from cachetools import cached, TTLCache
@cached(cache=TTLCache(maxsize=100, ttl=3600)) def expensive_function(n): # Perform expensive calculations result = ...
return result
By incorporating caching and memoization techniques into your Python code, you can significantly improve performance and optimize resource usage. However, it's essential to use these techniques judiciously and consider factors like cache size, cache expiration, and the trade-off between memory usage and computation time.
Conclusion
After exploring various techniques and strategies for optimizing Python code performance, it's clear that there are numerous ways to enhance the efficiency of your code:
- By focusing on algorithm efficiency, memory management, data structures, and parallel processing, you can significantly improve the performance of your Python applications.
- Leveraging built-in functions, modules, and profiling tools further enhances your ability to identify and address performance bottlenecks effectively.
- Implementing caching and memoization techniques can also play a crucial role in optimizing code performance by reducing redundant computations and enhancing data retrieval speed.
Remember that the goal of optimizing Python code for performance is not just about achieving faster execution times but also about maximizing resource utilization and minimizing unnecessary overhead.
Continuously refining your code through these optimization techniques will lead to more efficient and scalable applications, ultimately improving the overall user experience.
Embracing a mindset of ongoing improvement and staying updated with the latest advancements in Python development will enable you to adapt to evolving performance challenges effectively.
FAQs (Frequently Asked Questions)
What is the importance of optimizing Python code for performance?
Optimizing Python code for performance is crucial for improving the efficiency and speed of the code execution. It can lead to reduced resource usage, faster response times, and overall better user experience.
What are the benefits of improving code performance?
Improving code performance can result in faster execution, reduced memory usage, and better scalability. It also leads to more efficient resource utilization and cost savings in terms of infrastructure.
What are the common performance bottlenecks in Python code?
Common performance bottlenecks in Python code include inefficient algorithms, excessive memory usage, suboptimal data structures, and inefficient loop iterations.
How can one optimize algorithm efficiency in Python?
Algorithm efficiency can be optimized by analyzing time and space complexity, identifying inefficient algorithms, and implementing techniques such as dynamic programming or greedy algorithms.
What are the benefits of using parallel processing and multithreading in Python?
Parallel processing and multithreading in Python can lead to improved performance by utilizing multiple processors or cores simultaneously, resulting in faster execution of tasks and better resource utilization.
What are caching and memoization, and how do they improve performance?
Caching and memoization involve storing computed results to avoid redundant calculations. They improve performance by reducing computation time for repeated operations and enhancing overall efficiency.
Comments
Post a Comment