Exploring Python's Standard Library: Your Gateway to Efficiency
- Get link
- X
- Other Apps
Exploring Python's Standard Library: Your Gateway to Efficiency
Introduction
Python, known for its straightforward syntax and versatility, is also celebrated for its comprehensive Standard Library. The Python Standard Library is a vast collection of modules and packages, offering pre-written code to handle a wide array of programming tasks. In this blog post, we'll delve into the richness of this library, highlighting its importance and some of its most useful modules.
The Python Standard Library is a comprehensive resource that provides a wide range of functionality for developers. It consists of modules and packages that offer standardized solutions to various programming problems. With its extensive collection of tools and utilities, the Python Standard Library serves as a go-to resource for enhancing efficiency in Python development.
Here are some key talking points for this section:
1. Brief explanation of the Python Standard Library
The Python Standard Library is a collection of modules and packages that come bundled with Python. These modules cover diverse areas such as operating system interfaces, file management, error handling, mathematics, networking, data compression, internationalization, GUI development, and much more.
2. Significance in programming
The Python Standard Library plays a crucial role in programming by providing ready-to-use solutions for common tasks. It eliminates the need for developers to reinvent the wheel or rely heavily on external libraries or packages. By leveraging the power of the standard library, developers can save time, streamline their workflows, and write more efficient code.
3. Enhancing efficiency in Python development
The availability of such a comprehensive set of tools within the Python Standard Library allows developers to work more efficiently. They can leverage the library's functionality to perform complex operations without having to search for or integrate external dependencies. This not only saves time but also ensures code consistency and reduces potential compatibility issues.
In conclusion, the Python Standard Library is a valuable resource that empowers developers by providing a vast array of pre-built functionalities. Its significance in programming lies in its ability to enhance efficiency by offering standardized solutions to common programming tasks. By understanding and utilizing the capabilities of the standard library, developers can expedite their development process and deliver high-quality code.
What is the Python Standard Library?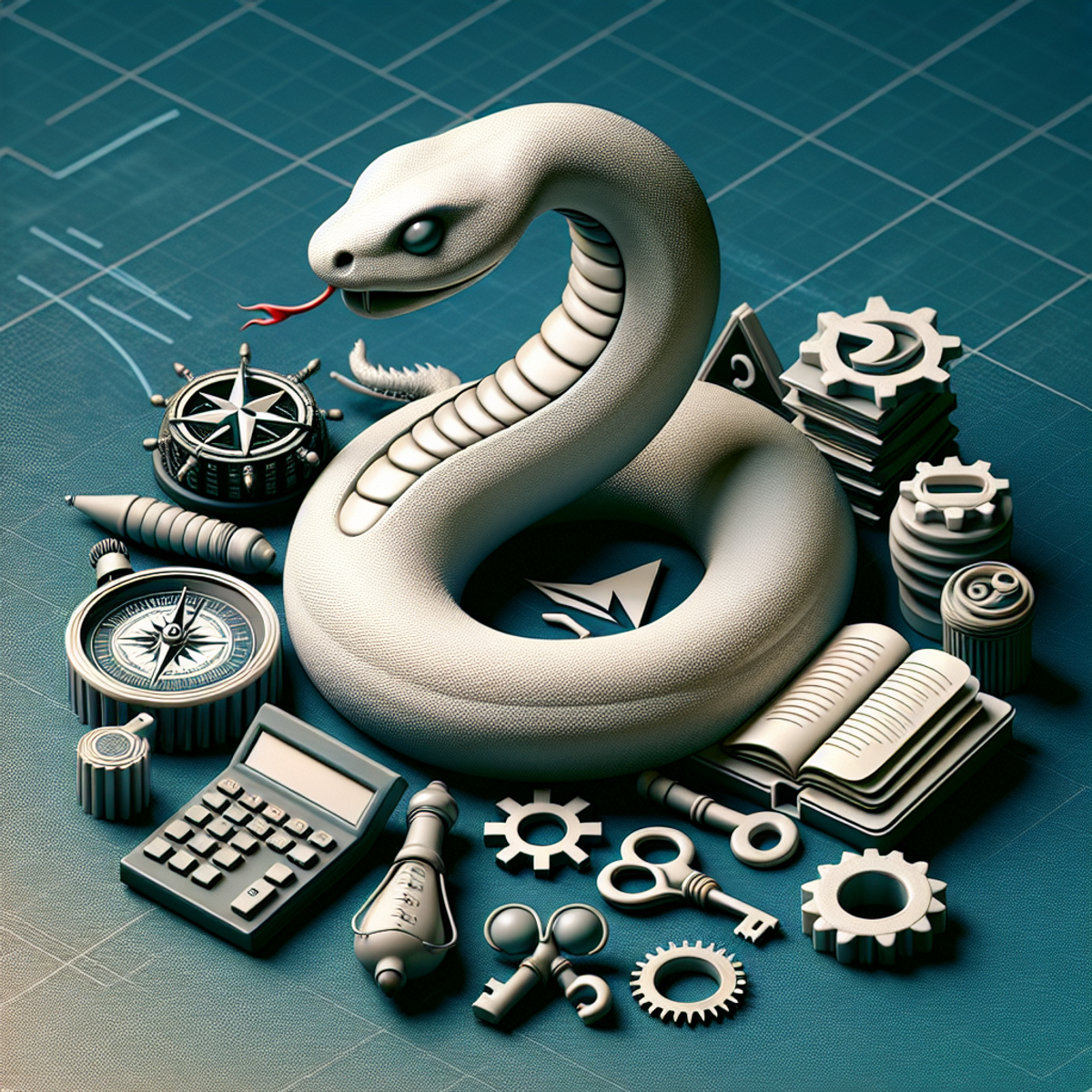
The Python Standard Library is a vast collection of modules and packages that come bundled with the Python programming language. It serves as a comprehensive resource for developers, providing ready-to-use solutions to common programming tasks without the need for external dependencies.
Here are some key characteristics of the Python Standard Library:
- Extensiveness: The library includes a wide range of functionality, covering areas such as file and directory management, network communication, data serialization, math operations, date and time manipulation, and more. It offers a rich set of tools and utilities that can be leveraged to enhance efficiency in Python development.
- Comprehensiveness: With hundreds of modules and packages, the Python Standard Library provides standardized solutions for various programming problems. It includes built-in functions, constants, types, exceptions, and services for text processing, binary data handling, multimedia operations, internationalization support, and GUI development.
- Batteries Included Philosophy: The library follows the "Batteries Included" philosophy of Python by providing extensive capabilities out-of-the-box. This means that developers can start working on projects immediately without having to install additional third-party libraries or packages.
- Active Maintenance: The Python Standard Library is actively maintained by the Python community. This ensures that it stays up-to-date with the latest developments in the language and continues to meet the evolving needs of developers.
By leveraging the Python Standard Library, developers can save time and effort by utilizing pre-existing functionality instead of reinventing the wheel. It promotes code reuse, improves productivity, and allows developers to focus on solving specific challenges rather than implementing basic functionalities from scratch.
Next, let's explore why relying on the standard library is beneficial in Python development.
Why Rely on the Python Standard Library?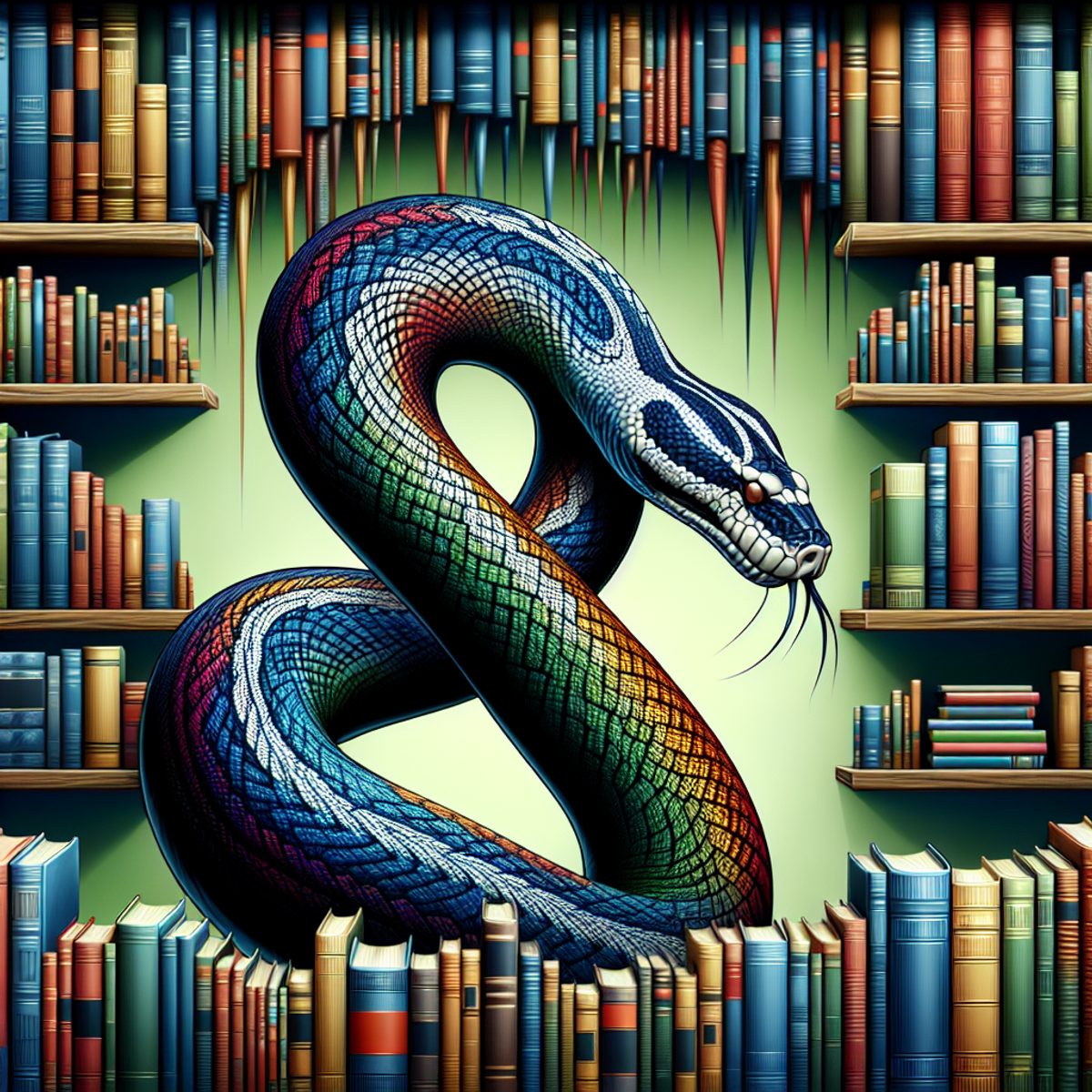
The Python programming language is widely known for its versatility and ease of use. One of its greatest strengths lies in its extensive Standard Library, which offers numerous advantages that make it a valuable resource for developers. Here are some reasons why you should rely on the Standard Library:
- Comprehensive functionality: The Standard Library provides a vast collection of modules and packages that cover a wide range of programming tasks. Whether you need to work with file systems, process data, handle network communication, or manipulate dates and times, you can find a suitable module within the library.
- Batteries Included: Python follows the "Batteries Included" philosophy, meaning that it aims to provide a rich set of tools and functionalities out of the box. The Standard Library embodies this principle by offering ready-to-use solutions for common programming problems. By leveraging the library, you can save time and effort in implementing functionality from scratch or searching for external libraries.
- Consistent and reliable: The modules in the Standard Library are part of the official Python distribution and undergo rigorous testing and maintenance. This ensures that they are stable, well-documented, and adhere to standard coding practices. You can rely on these modules for production-grade applications without worrying about compatibility issues or frequent updates.
- Cross-platform compatibility: Python is known for its cross-platform capabilities, and the Standard Library follows suit. The modules are designed to work seamlessly across different operating systems, making it easier to develop applications that can run on multiple platforms with minimal modifications.
- Community support: The Python community is vibrant and supportive, with many developers actively using and contributing to the Standard Library. This means that you can find ample resources, tutorials, and examples online from this community to help you understand and utilize various modules effectively.
- Reduced dependencies: By utilizing the functionality provided by the Standard Library, you can minimize your dependencies on external libraries or packages. This not only simplifies your development process but also reduces potential conflicts or compatibility issues between different libraries.
Relying on the Python Standard Library empowers you to write efficient and high-quality code by leveraging the well-tested and comprehensive functionality it offers.
How to Get Started?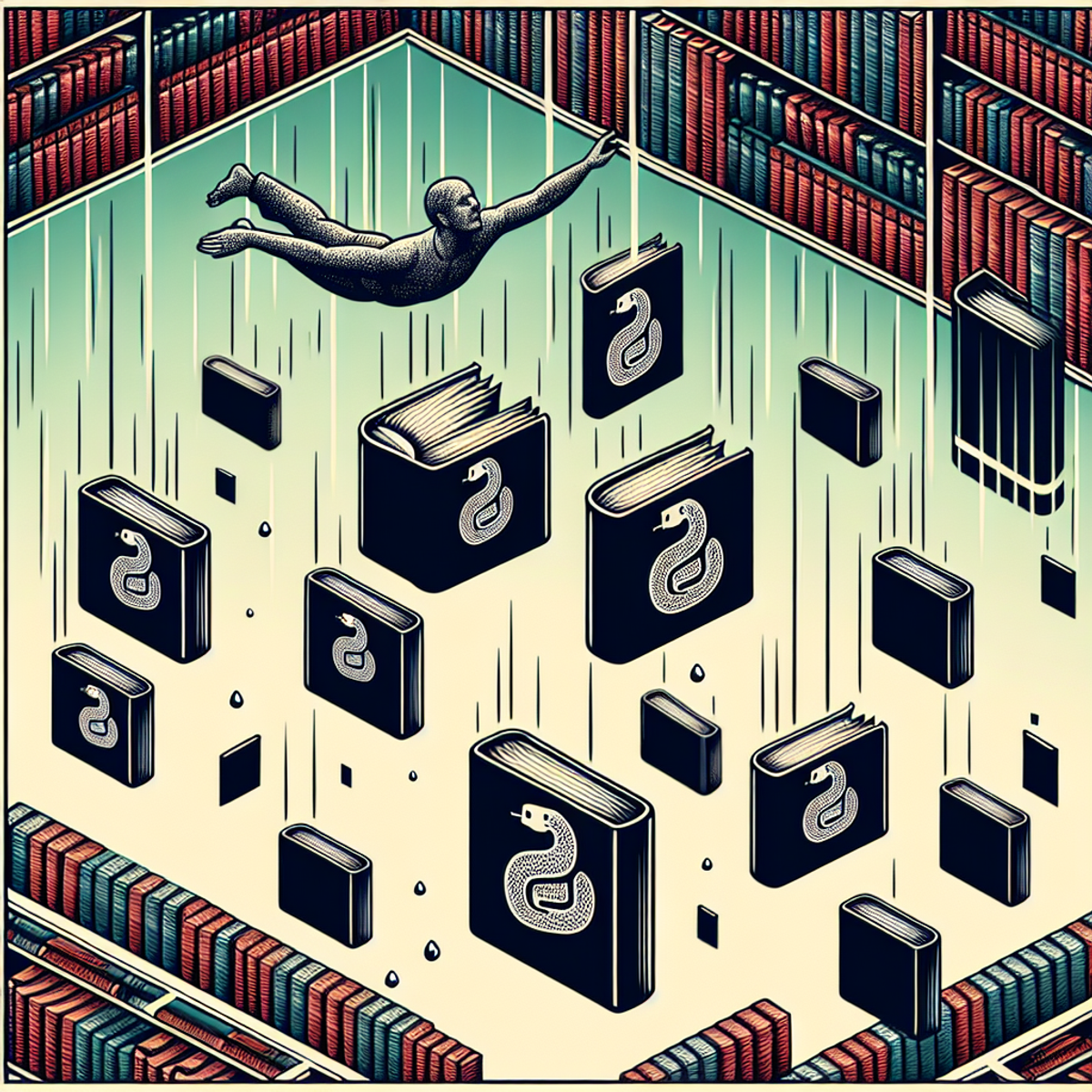
The best way to explore the Python Standard Library is by diving in and experimenting with its modules. Python's official documentation is a great starting point as it offers comprehensive guides and examples that can help you understand and utilize the library effectively.
Here are some tips on how to get started:
- Leverage the Official Documentation: The Python Standard Library documentation provides detailed information about each module, including explanations of their functionalities, examples of usage, and references to related modules. It's a valuable resource for understanding the capabilities of the library and how to use it in your projects.
- Start with Small Projects: To boost your understanding and proficiency in Python, try experimenting with the modules in small projects. By working on practical tasks, you can apply the concepts you learn from the documentation and gain hands-on experience with the library's features. This iterative approach allows you to gradually explore different modules and build your knowledge step by step.
- Focus on Modules Relevant to Your Needs: The Python Standard Library offers a wide range of modules, but not all of them may be relevant to your specific needs. To avoid overwhelming yourself with too much information, focus on modules that align with your project requirements or areas of interest. As you become more comfortable with the library, you can explore additional modules as needed.
- Refer to Community Resources: In addition to the official documentation, there are numerous community-driven resources available online that provide tutorials, articles, and code examples for working with specific modules in the library. These resources can offer different perspectives, practical tips, and real-world use cases that can enhance your understanding and help you discover new possibilities within the Standard Library.
- Document Your Code Effectively: While exploring the Python Standard Library or any other aspect of Python development, it's essential to document your code properly. This helps not only in understanding your own code but also in collaborating with other developers. You can learn more about documenting Python code to ensure your projects are well-documented and maintainable.
- Attend Workshops or Webinars: If you prefer a more interactive learning experience, consider attending workshops or webinars focused on exploring the Python Standard Library. These events often provide hands-on exercises, demonstrations, and discussions led by experienced developers who can share their insights and best practices for utilizing the library effectively.
By following these tips, you can embark on your journey of exploring the Python Standard Library and unlock its vast potential to enhance your Python development efficiency.
Pro Tip: When working with the Standard Library, it's important to keep in mind that certain modules may have dependencies on other modules. To avoid potential compatibility issues, always check the documentation for any module-specific requirements or recommendations.
Remember, the Python Standard Library is a powerful resource that can significantly streamline your development process. Take advantage of its extensive functionalities, experiment with different modules, and leverage its capabilities to write more efficient and robust Python code.
Understanding the Structure of the Python Standard Library
The Python Standard Library is organized using modules and packages, which serve as the building blocks of its organization. Here is an overview of these key components:
Modules
- Modules are individual files that contain Python code and define functions, classes, and variables.
- Each module focuses on a specific area of functionality, such as file operations (os module), mathematics (math module), or networking (socket module).
- Modules can be imported into your Python programs using the
import
statement to make their functionality accessible.
import
statement to make their functionality accessible.Packages
- Packages are directories that contain multiple modules and sub-packages.
- They provide a way to organize related modules together in a hierarchical structure.
- Packages enable you to group related functionality under a common namespace, making it easier to manage and access different modules.
- You can import modules from packages using dot notation, such as
import package.module
.
import package.module
.The standard library's organization is designed to be intuitive and logical. Modules are grouped together based on their functionality, making it easier for developers to find the tools they need. For example:
- The
os
module provides functions for interacting with the operating system, including file and directory operations. - The
datetime
module offers functions for working with dates and times. - The
json
,csv
, andxml.etree.ElementTree
modules provide support for parsing and manipulating data in various formats.
By understanding the structure of the Python Standard Library, you can navigate through its modules and packages more efficiently. This knowledge allows you to locate the desired functionality quickly, saving you time during development.
Key Features and Utility Functions
The Python Standard Library offers a diverse range of utility functions and constants, providing developers with a wide array of tools to streamline their programming tasks. Here are some key points to consider:
Built-in Functions
The library includes a rich set of built-in functions that cover various operations such as mathematical computations, string manipulation, data type conversions, and more. These functions are readily available for use without the need for additional imports or installations, making them highly convenient for everyday coding tasks.
Constants
In addition to functions, the library also provides essential constants that can be used across different applications. These constants encompass values such as mathematical constants (e.g., pi), error codes, and other predefined symbolic representations that aid in maintaining consistency and readability within code.
By leveraging the utility functions and constants offered by the Python Standard Library, developers can enhance their productivity and efficiency while minimizing the reliance on external dependencies. This comprehensive collection of tools reflects the "Batteries Included" philosophy of Python, empowering programmers with a robust foundation for their projects.
Exploration of Major Modules
The Python Standard Library has many important modules that make Python development more efficient and productive. These modules have various functions, such as working with the operating system, managing files, processing command-line arguments, handling errors, finding patterns in strings, doing math calculations, making random choices, and analyzing statistics. Let's take a closer look at some key modules and what they offer:
Module 1: Operating System Interfaces
The os module is essential for interacting with the operating system. It has many functions for working with files and directories, managing processes, and accessing environment variables. With the os module, you can easily perform tasks like manipulating files, navigating directories, and controlling processes using Python.
Module 2: File Management
The shutil module provides high-level operations for working with files and directories. It includes functions for copying files, archiving directories, and deleting files. This module simplifies complex file management tasks by offering efficient functions for different file operations. It is a valuable tool for handling files in Python applications.
Module 3: Command-Line Argument Processing
The argparse module is a powerful tool for parsing command-line arguments and creating user-friendly command-line interfaces. With this module, developers can easily define command-line options and arguments for their Python scripts. The argparse module makes it easier to interact with Python programs through the command line by handling argument parsing in a clear and consistent way.
These major modules in the Python Standard Library are crucial for performing important programming tasks related to the operating system, file management, and command-line argument processing. By using these modules effectively, developers can greatly improve the efficiency and reliability of their Python applications.
In the next section, we will explore how built-in modules in the Python Standard Library can be used to enhance networking capabilities for better internet communication.
Enhancing Networking Capabilities with Built-in Modules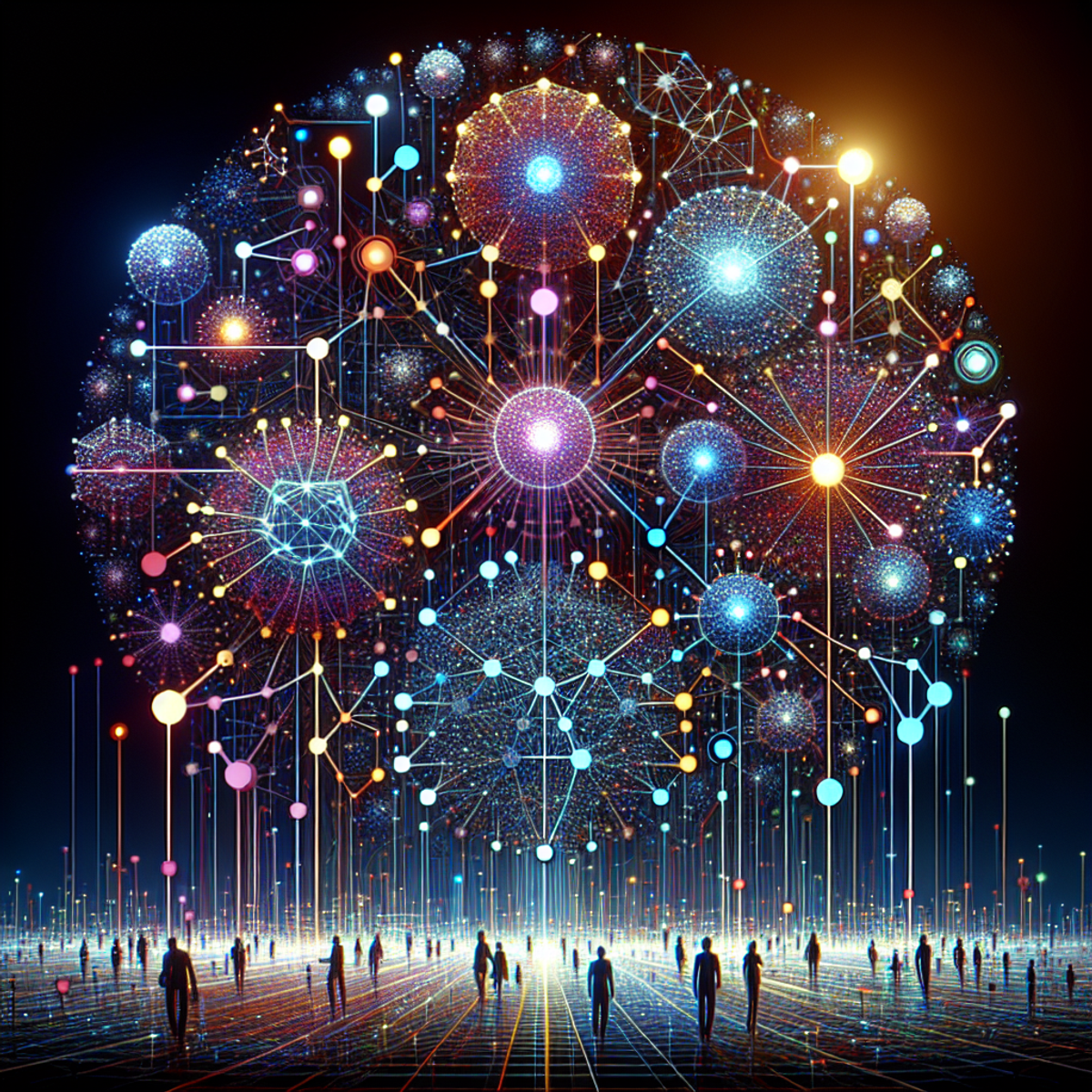
Python's Standard Library offers a range of networking modules that allow developers to enhance their internet communication abilities. By leveraging these modules, you can easily perform tasks such as handling HTTP requests, creating web clients, fetching web content, and interacting with REST APIs. Here are some key points to consider:
http module
This module provides classes and functions for handling HTTP requests and responses. It allows you to build web clients, send GET and POST requests, set headers, handle cookies, and more. With the http module, you can efficiently interact with web services and retrieve data from websites.
urllib module
The urllib module provides high-level classes and functions for URL manipulation and handling. It supports various protocols like HTTP, FTP, and others. You can use urllib to open URLs, download files, parse URLs into components, encode query parameters, and handle redirects.
socket module
The socket module enables low-level network programming by providing a way to create sockets and establish network connections. It allows you to send and receive data over TCP/IP or UDP protocols. With sockets, you can build custom network applications or implement network protocols.
socketserver module
This module simplifies the creation of network servers by providing classes for building TCP/IP servers. It handles the low-level details of accepting connections, managing threads or processes, and processing client requests. You can use socketserver to quickly develop network servers for various purposes.
imaplib module
The imaplib module allows you to interact with IMAP (Internet Message Access Protocol) servers for accessing email messages. You can fetch emails from mailboxes, search for specific messages based on criteria, manipulate message flags, and perform other email-related operations.
These networking modules in Python's Standard Library provide powerful capabilities for handling internet communication tasks. Whether you need to fetch data from APIs, build web clients, or implement network protocols, these modules can greatly enhance your networking capabilities and streamline your Python development process.
Keep in mind that the examples and use cases mentioned are just a glimpse of what you can achieve with these modules. The Standard Library's documentation offers detailed guides and examples for each module, allowing you to explore further and harness the full potential of networking in Python. So dive in and start exploring the networking modules to unleash the power of internet communication in your Python projects.
Working with Dates, Times, and Timezones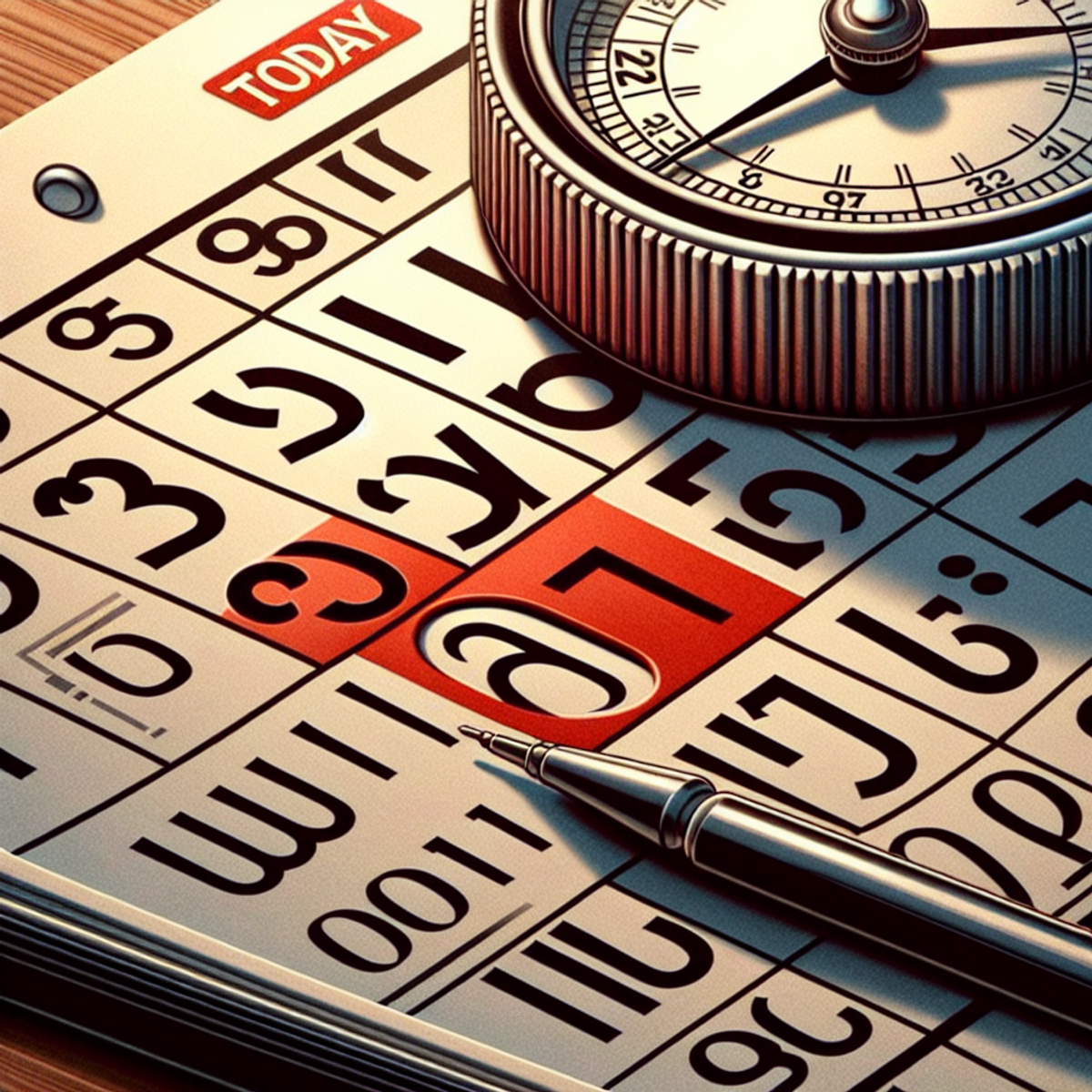
The Python Standard Library provides robust support for working with dates, times, and timezones. Understanding these features is crucial for applications that require time tracking, scheduling, or any kind of time-related operations.
The datetime
module
The datetime
module is a fundamental part of the Python Standard Library and offers classes for manipulating dates and times. It provides various functionalities such as:
- Creating date and time objects
- Performing arithmetic operations on them
- Formatting them into strings
- Extracting specific components like year, month, day, hour, minute, and second
Timezone handling with the pytz
module
Although the datetime
module provides basic timezone support, the pytz
module enhances this functionality by allowing you to work with timezones from around the world. It provides timezone localization and conversion capabilities for accurate time-related calculations.
Time intervals with the timedelta
class
The timedelta
class in the datetime
module allows you to represent a duration or difference between two dates or times. It is particularly useful for calculating intervals such as adding/subtracting days, hours, minutes, or seconds to/from a given date or time.
Parsing and formatting dates
The datetime
module includes functions like strptime()
and strftime()
that enable parsing strings into date/time objects and formatting date/time objects into strings according to specific formats.
Dealing with daylight saving time
The datetime
module takes care of daylight saving time adjustments automatically when working with local time. It ensures accurate handling of transitions between standard time and daylight saving time.
By leveraging these features in the Python Standard Library, you can easily manipulate dates, times, and timezones in your applications. Whether you need to calculate durations between events, format timestamps for display purposes, or handle different time zones seamlessly, the library provides comprehensive tools to streamline these operations.
Efficient Data Compression with Python Standard Library
The Python Standard Library offers powerful capabilities for working with data compression formats and algorithms. By leveraging modules like gzip and zlib, developers can efficiently compress and decompress data in various formats.
Here are the key points to explore in this section:
1. Data Compression Formats
The library provides support for popular data compression formats such as gzip, zlib, and bz2. These formats allow you to reduce the size of your data files, making them more efficient for storage and transmission.
2. Compression Algorithms
Along with supporting different compression formats, the library also includes several compression algorithms. These algorithms, such as Deflate (used by gzip) and Lempel-Ziv-Welch (used by zlib), enable effective compression of different types of data.
3. gzip Module
The gzip module in the Python Standard Library allows you to work with files that are compressed using the gzip format. You can compress or decompress files using simple functions provided by the module, making it easy to integrate compression functionality into your Python applications.
4. zlib Module
The zlib module provides functions for working with compressed data using the zlib format. It offers a wide range of features, including compressing and decompressing data in memory, calculating checksums, and working with compressed streams.
By utilizing these modules, you can significantly improve the efficiency of your data storage and transmission processes. Whether you need to compress large files for archival purposes or reduce network bandwidth usage when transferring data over the internet, the Python Standard Library has you covered.
Ensuring Code Quality and Performance Optimization
Ensuring code quality and optimizing performance are crucial aspects of software development. Python's Standard Library provides a range of tools and modules that can help you measure code performance, conduct tests, and maintain quality standards.
Here are some ways you can leverage the Python Standard Library to ensure code quality and optimize performance:
1. Profiling
The cProfile
module allows you to profile your code, identifying bottlenecks and areas where optimization is needed. It provides detailed information about the time spent in each function, helping you prioritize optimizations.
2. Testing
The unittest
module provides a framework for writing test cases and running tests. With this module, you can automate the testing process, ensuring that your code functions as expected. Additionally, the doctest
module allows you to write tests as part of your documentation, ensuring that examples in your documentation remain up-to-date.
3. Performance Measurement
The timeit
module allows you to measure the execution time of small code snippets, helping you compare different implementations and identify areas for improvement. It provides a simple interface for benchmarking and profiling.
4. Quality Control
The pylint
module is a popular tool for analyzing Python code and enforcing coding standards. It checks for potential errors, style violations, and other issues that could impact the quality of your code. By integrating pylint into your development workflow, you can catch potential problems early on and maintain a consistent coding style.
By leveraging these tools from the Python Standard Library, you can ensure that your code meets high-quality standards while optimizing its performance. Whether it's profiling your code to identify bottlenecks or automating tests to catch bugs early on, the Standard Library has you covered.
Remember to explore the official Python documentation for more information on how to use these tools effectively in your projects.
Support for Internationalization in the Library
The Python Standard Library offers robust internationalization services to support language translation and locale-specific formatting. Here are the key talking points to consider:
- gettext module: This module provides comprehensive support for message translation, allowing developers to create multilingual applications with ease. By using the
gettext
module, you can extract translatable strings from your code and manage translations for different languages efficiently. - locale module: The
locale
module enables you to format data based on specific cultural conventions, such as numbers, currency, time, and date formats. It provides essential functionalities for adapting applications to diverse linguistic and cultural environments.
By leveraging these internationalization services, developers can ensure that their Python applications are accessible and user-friendly across various regions and language preferences.
GUI Development Possibilities with Python Standard Library
Python's Standard Library offers robust capabilities for GUI development through the Tkinter module. This built-in module provides developers with a simple and efficient way to create graphical user interfaces for their applications. By leveraging Tkinter, you can design intuitive and interactive user interfaces, enhancing the overall user experience of your Python applications.
1. Exploring opportunities for GUI development using the Tkinter module
In addition to GUI development, the Standard Library also includes multimedia services that can be utilized in conjunction with GUI applications. The wave module facilitates the manipulation of WAV audio files, allowing developers to work with sound data directly within their Python programs. Similarly, the colorsys module provides functionality for converting between color representations, enabling seamless integration of color-related features within GUI applications.
2. Leveraging multimedia services from the library (e.g., wave and colorsys modules)
Overall, the Python Standard Library equips developers with the necessary tools to build powerful graphical interfaces and incorporate multimedia elements into their Python applications without relying on external libraries or dependencies.
Exploring Optional Components and External Libraries
The Python Standard Library is already packed with a wide range of modules and packages that provide comprehensive functionality for various tasks. However, there are also optional components included in Python distributions that can further enhance your programming experience. Additionally, there is a vast collection of external libraries and packages available on the Python Package Index (PyPI) that you can leverage to expand the capabilities of your Python projects.
Key Points to Consider
Here are some key points to consider when exploring optional components and external libraries/packages:
1. Optional Components
Python distributions come with optional components that provide additional functionality beyond the standard library. These components may include development tools, database connectors, scientific computing libraries, web frameworks, and more. They are designed to be optional so that developers can choose to install them based on their specific needs.
2. Python Package Index (PyPI)
PyPI is the official repository for third-party Python packages. It hosts a vast collection of external libraries and packages that you can easily install and use in your projects. PyPI provides a convenient way to search for packages based on keywords, browse package details, and manage package dependencies using package managers like pip.
3. Benefits of Using the Python Standard Library
While external libraries/packages offer additional functionality, there are several benefits to relying on the Python Standard Library alongside or in place of external dependencies:
- Efficiency: The standard library is already included with Python distributions, which means you don't need to install or manage additional dependencies. This can save time and effort when setting up your development environment.
- Compatibility: The standard library is maintained by the core Python development team and is designed to work seamlessly with different versions of Python. This ensures better compatibility and reduces the risk of version conflicts or compatibility issues between different libraries/packages.
- Reliability: The standard library undergoes rigorous testing and is regularly updated to ensure stability and reliability. By relying on the standard library, you can benefit from the extensive testing and bug fixes performed by the Python community.
- Consistency: The standard library follows Python's design principles and coding conventions, ensuring a consistent and familiar programming experience. This can make it easier to understand and maintain your code over time.
By exploring optional components included in Python distributions and leveraging external libraries/packages from PyPI, you can enhance your Python projects with additional functionality while still enjoying the benefits of using the Python Standard Library.
A Glimpse at Noteworthy Modules
When exploring the Python Standard Library, there are several modules that stand out for their usefulness and versatility. Let's take a closer look at some of these noteworthy modules:
1. sys and os
- Usage: System-specific parameters and functions.
- Applications: Interacting with the Python interpreter (
sys
) and operating system-dependent functionality like file system manipulation (os
).
sys
) and operating system-dependent functionality like file system manipulation (os
).2. datetime
- Usage: Date and time manipulation.
- Applications: Handling dates, times, and time intervals – essential for applications requiring time tracking or scheduling.
3. math and random
- Usage: Mathematical functions and random number generation.
- Applications: From basic arithmetic to complex mathematical operations (
math
) and generating random numbers for simulations or games (random
).
math
) and generating random numbers for simulations or games (random
).4. json, csv, and xml.etree.ElementTree
- Usage: Data parsing and serialization.
- Applications: Parsing and manipulating data in JSON, CSV, and XML formats, essential for data exchange and API interactions.
5. http and urllib
- Usage: Handling HTTP requests and URL manipulation.
- Applications: Creating web clients, fetching web content, and interacting with REST APIs.
6. sqlite3
- Usage: Lightweight disk-based database management.
- Applications: Local data storage and retrieval without the need for a separate database server.
7. re
- Usage: Regular expressions.
- Applications: String searching, manipulation, and pattern matching – essential for text processing.
8. unittest
- Usage: Testing framework.
- Applications: Writing and running tests to ensure code reliability and avoid regressions.
These modules offer a wide range of functionality that can significantly enhance your Python development experience. By leveraging these modules from the Python Standard Library, you can save time and effort by utilizing pre-existing solutions for common programming tasks.
Examples of Module Usage
For example, the sys
module provides access to system-specific parameters and functions. You can use it to interact with the Python interpreter, retrieve command-line arguments, manipulate the import path, and more. Similarly, the os
module allows you to perform various operating system-dependent operations such as file and directory manipulation.
The datetime
module is invaluable when working with dates, times, and time intervals. It provides a rich set of classes and functions for handling time-related operations, making it easier to manage tasks like parsing dates from strings, calculating time differences, or formatting date output.
If you need to perform mathematical calculations or generate random numbers, the math
and random
modules come in handy. The math
module offers a wide range of mathematical functions, from basic arithmetic to trigonometry and logarithms. On the other hand, the random
module allows you to generate random numbers for simulations or games.
When it comes to data parsing and serialization, modules like json
, csv
, and xml.etree.ElementTree
provide powerful tools. These modules enable you to read and write data in popular formats such as JSON, CSV, and XML. For example, you can easily parse JSON responses from APIs or export data to CSV files using these modules.
The http
and urllib
modules are essential for handling HTTP requests and manipulating URLs. With these modules, you can create web clients, fetch web content from URLs, send GET/POST requests, handle cookies, and interact with REST APIs.
For lightweight database management without the need for a separate database server, the sqlite3
module is a great choice. It allows you to create SQLite databases on disk, execute SQL queries, manage transactions, and retrieve data efficiently.
Regular expressions are powerful tools for string manipulation and pattern matching. The re
module provides a robust set of functions for working with regular expressions in Python. You can use it to search for specific patterns in strings, replace text, or extract substrings based on a pattern.
Lastly, the unittest
module offers a built-in testing framework for writing and running tests. It provides a convenient way to ensure code reliability, catch bugs early, and avoid regressions when making changes to your codebase.
These are just a few examples of the many modules available in the Python Standard Library. By exploring and understanding these modules, you can unlock the full potential of the library and streamline your Python development process.
Conclusion
The Python Standard Library is essential for efficient Python programming, providing a wide range of tools for various tasks. By learning about the different modules and using their features, developers can work more effectively in different programming situations.
FAQs (Frequently Asked Questions)
What is the Python Standard Library?
The Python Standard Library is a comprehensive resource that provides a wide range of functionalities for Python programming. It includes built-in modules and packages that offer support for various tasks such as data manipulation, networking, GUI development, and more.
Why Rely on the Standard Library?
Relying on the Python Standard Library allows developers to access a rich set of pre-built tools and functionalities without the need for external dependencies. This can significantly enhance efficiency in Python development by reducing the time and effort required to implement common programming tasks.
How to Get Started?
The best way to explore the Standard Library is by diving into Python’s official documentation, which provides comprehensive guides and examples. Experimenting with modules in small projects can boost understanding and proficiency in Python.
Understanding the Structure of the Python Standard Library
The Python Standard Library is organized using modules and packages as building blocks. Modules contain related functions, classes, and constants, while packages are collections of related modules.
Key Features and Utility Functions
The library offers a diverse range of utility functions and constants that provide essential tools for tasks such as data manipulation, mathematical operations, file management, and more.
Exploration of Major Modules
In-depth discussion on key modules showcasing their functionalities and how they contribute to efficiency: - Module 1 - Module 2 - Module 3
- Get link
- X
- Other Apps
Comments
Post a Comment