Python in Cryptocurrency Trading
Python in Cryptocurrency Trading
Introduction
Python plays a crucial role in cryptocurrency trading, offering a versatile and powerful tool for traders and developers. Its significance lies in its ability to automate processes in the fast-paced environment of cryptocurrency trading through the creation of sophisticated trading bots. These bots are designed to:
- Fetch market data
- Execute orders based on predefined strategies
- Interact with cryptocurrency exchange APIs seamlessly
The key benefits of using Python in cryptocurrency trading include:
- Automation: Python enables the automation of various trading tasks, allowing for efficient execution and management of trades.
- Flexibility: Its extensive libraries and easy syntax provide flexibility in developing and implementing diverse trading strategies.
- Efficiency: Python facilitates the swift execution of trades and real-time monitoring, contributing to overall efficiency in cryptocurrency trading operations.
Python's role in cryptocurrency trading goes beyond being just a programming language; it serves as a fundamental enabler for streamlining processes and implementing advanced strategies in the dynamic landscape of digital asset markets.
Understanding Python's Significance in Cryptocurrency Trading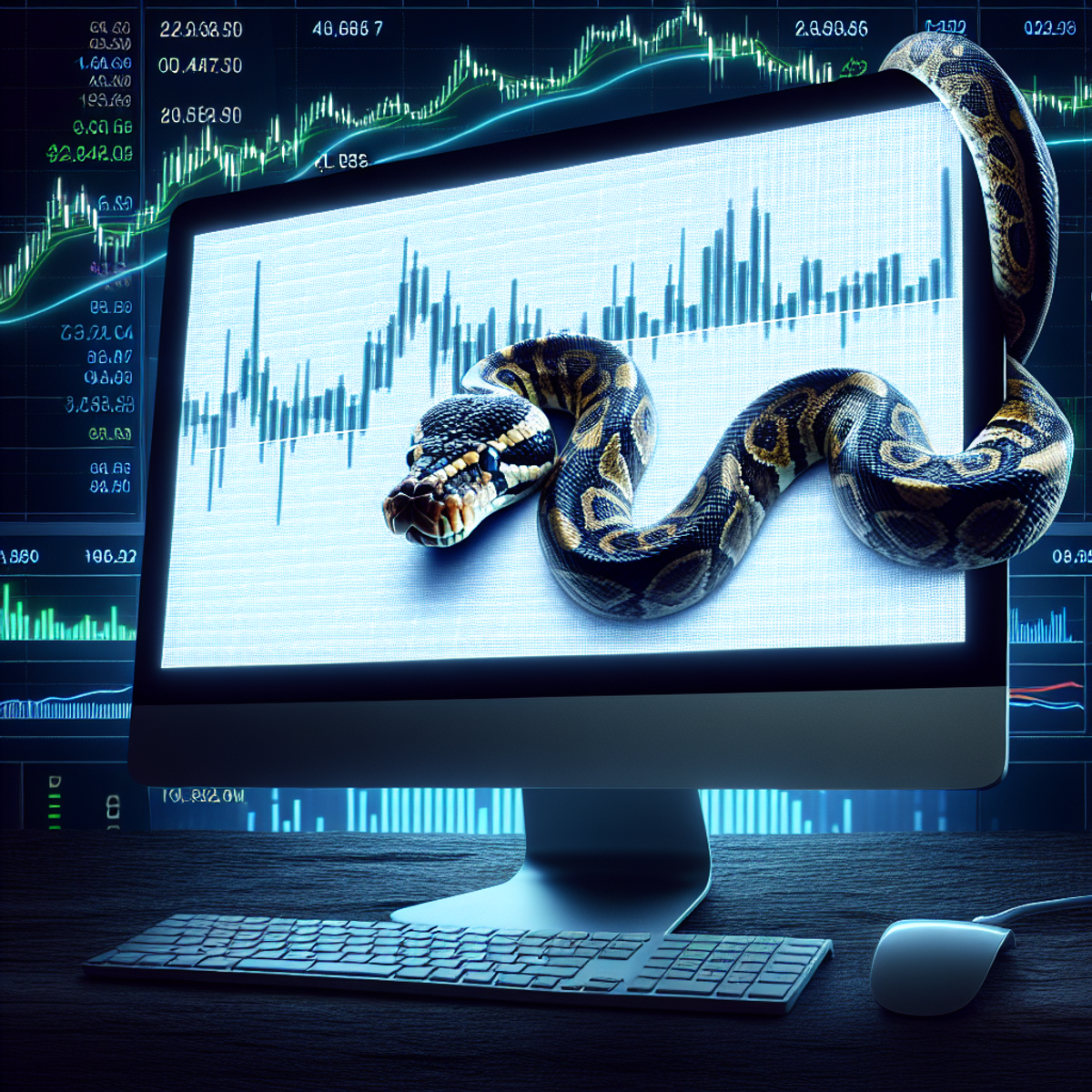
Python has become the go-to programming language for creating cryptocurrency trading bots due to its extensive libraries and easy syntax. Here's why it has emerged as a dominant force in the realm of cryptocurrency trading:
1. Extensive Libraries
Python boasts a rich ecosystem of libraries and frameworks that support the development of cryptocurrency trading bots. These libraries provide essential functionalities such as data analysis, API interaction, and strategy implementation, making Python an ideal choice for traders and developers.
2. Easy Syntax
Python's user-friendly syntax allows for rapid prototyping and seamless integration of complex trading strategies. Its readability and simplicity enable traders to focus on strategy development rather than getting bogged down in intricate syntax details.
Automated bots play a crucial role in buying and selling cryptocurrencies on exchanges. Python facilitates this process by enabling seamless interactions with exchange APIs. Through Python, traders can develop bots that fetch real-time market data, execute trades based on predefined strategies, and manage their portfolio efficiently.
By leveraging Python, traders can implement automated trading strategies that react to market conditions in real time without the need for manual intervention. This automation is particularly valuable in the fast-paced world of cryptocurrency trading, where split-second decisions can make a significant difference in profitability.
In summary, Python's significance in cryptocurrency trading stems from its robust libraries, intuitive syntax, and pivotal role in developing automated trading bots that streamline the execution of trading strategies and enhance overall efficiency.
Key Python Libraries for Cryptocurrency Trading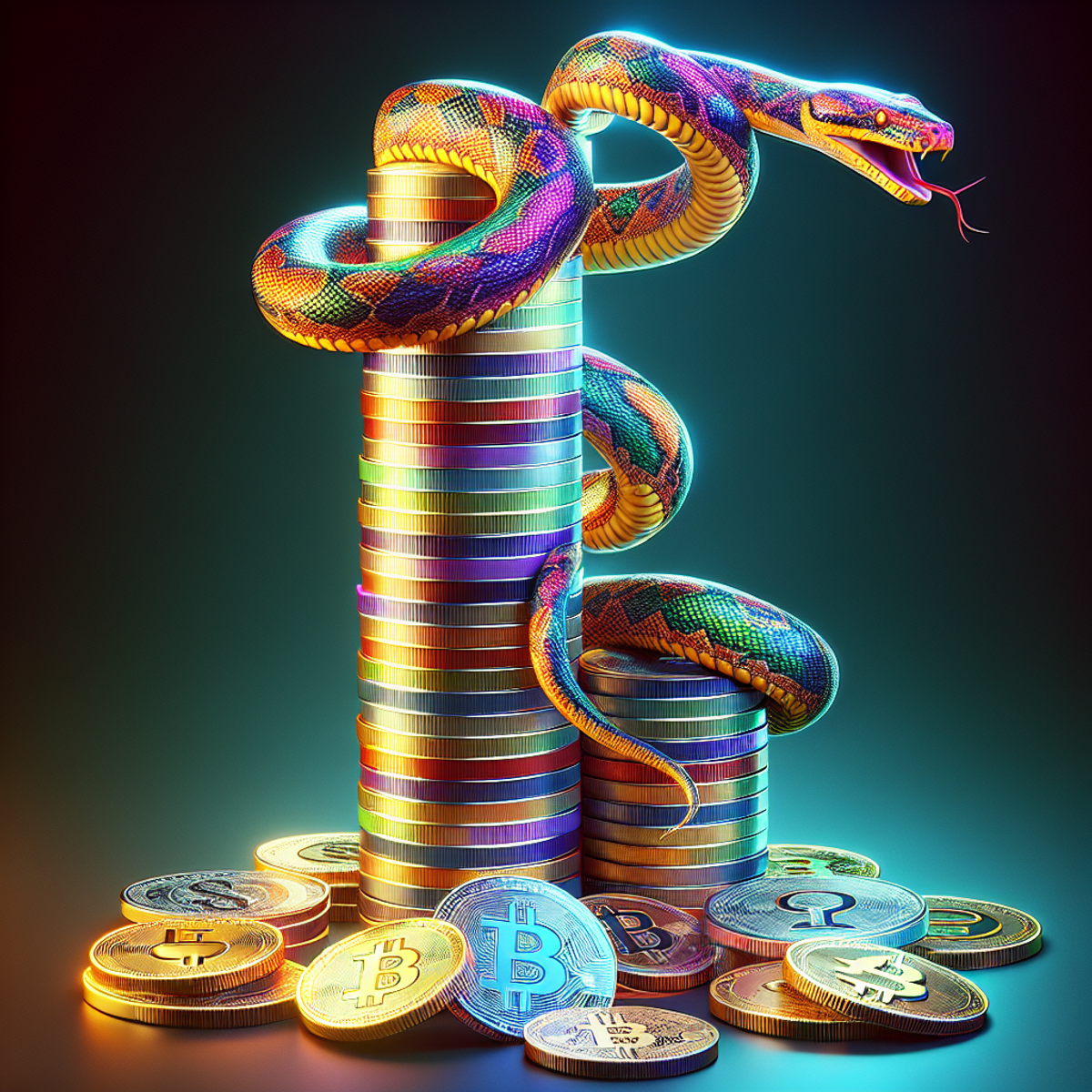
Python offers a wide range of libraries that are specifically designed for cryptocurrency trading. These libraries provide essential functionalities for interacting with cryptocurrency exchange APIs, fetching market data, analyzing data, and implementing trading strategies. In this section, we will explore two key Python libraries that are commonly used in cryptocurrency trading: ccxt and pandas.
ccxt - Your Gateway to Cryptocurrency Exchange APIs
One of the most popular and powerful libraries for cryptocurrency trading is ccxt. ccxt stands for "CryptoCurrency eXchange Trading Library" and it serves as a gateway to connect with various cryptocurrency exchange APIs. This library allows you to access a wide range of exchanges through a unified API, making it easier to fetch market data and execute trades across different platforms.
ccxt offers several notable features and advantages for cryptocurrency traders:
- Unified API: ccxt provides a consistent interface for interacting with different exchanges. This means that you can use the same code structure to fetch market data or place orders on different platforms without having to learn the intricacies of each individual API.
- Extensive Exchange Support: ccxt supports a large number of cryptocurrency exchanges, including popular ones like Binance, Coinbase Pro, Kraken, and Bitfinex. With ccxt, you can access a wide range of markets and trade various cryptocurrencies from a single codebase.
- Market Data Retrieval: Using ccxt, you can easily fetch real-time market data such as ticker prices, order book depth, trade history, and more. This allows you to stay up-to-date with the latest market trends and make informed trading decisions based on accurate information.
- Order Execution: ccxt enables you to execute trades programmatically by placing orders on supported exchanges. You can create buy or sell orders, set limit or market orders, specify order parameters like price and quantity, and monitor the status of your orders.
- Account Management: With ccxt, you can also manage your exchange accounts programmatically. This includes functionalities like fetching your account balance, retrieving transaction history, and generating deposit or withdrawal addresses.
- Easy Integration: ccxt is designed to be easy to integrate into your Python projects. It provides a simple and intuitive syntax, making it accessible for both beginner and experienced traders. Additionally, ccxt is actively maintained and frequently updated to ensure compatibility with the latest exchange APIs.
To give you a better idea of how ccxt works, here's a code snippet that demonstrates how to fetch the current Bitcoin price from the Binance exchange using ccxt:
python import ccxt
Create an instance of the Binance exchange class
binance = ccxt.binance()
Fetch the ticker price for Bitcoin
ticker = binance.fetch_ticker('BTC/USDT') btc_price = ticker['close']
Print the current Bitcoin price
print(f"The current BTC price is: {btc_price}")
Utilizing 'pandas' for Market Data Analysis in Cryptocurrency Trading
Another important Python library in cryptocurrency trading is pandas. pandas is a powerful data manipulation and analysis library that provides efficient data structures and functions for handling structured data.
When it comes to cryptocurrency trading, pandas can be used in various ways to analyze market data, perform technical analysis, and gain insights for developing trading strategies. Here are some ways in which pandas can be leveraged in cryptocurrency trading:
- Data Retrieval: pandas can be used to fetch historical market data from various sources such as CSV files, databases, or web APIs. You can import the data into pandas DataFrame objects, which provide a tabular structure for easy manipulation and analysis.
- Data Cleaning and Preprocessing: Cryptocurrency market data can be messy and inconsistent. pandas offers powerful tools for cleaning and preprocessing data, such as removing duplicate entries, handling missing values, and converting data types to ensure consistency and reliability.
- Technical Analysis: pandas provides a range of statistical functions and methods that are useful for performing technical analysis on market data. You can calculate moving averages, identify trend reversals, compute volatility measures, and more using pandas' built-in functions or by defining custom functions.
- Visualization: pandas integrates well with other Python libraries like Matplotlib and Seaborn, allowing you to visualize market data and analysis results in the form of charts, graphs, or plots. Visualizations can help you identify patterns, spot trends, and make informed trading decisions based on visual cues.
To give you a practical example of using pandas in cryptocurrency trading, consider the following code snippet that demonstrates how to calculate the 20-day simple moving average (SMA) for Bitcoin prices using historical data:
python import pandas as pd
Read historical price data from a CSV file into a DataFrame
data = pd.read_csv('btc_price.csv')
Convert the 'timestamp' column to datetime format
data['timestamp'] = pd.to_datetime(data['timestamp'])
Sort the DataFrame by timestamp in ascending order
data.sort_values(by='timestamp', inplace=True)
Calculate the 20-day SMA using the 'close' price column
data['sma_20'] = data['close'].rolling(window=20).mean()
Print the DataFrame with the SMA values
print(data)
In conclusion, Python provides powerful libraries like ccxt and pandas that are essential for cryptocurrency trading. ccxt enables seamless interactions with cryptocurrency exchange APIs, allowing you to fetch market data, execute trades, and manage your accounts across multiple exchanges. On the other hand, pandas provides efficient data manipulation and analysis capabilities for handling market data and performing technical analysis. By leveraging these libraries, you can develop effective trading strategies and gain valuable insights into the cryptocurrency market.
Utilizing 'pandas' for Market Data Analysis in Cryptocurrency Trading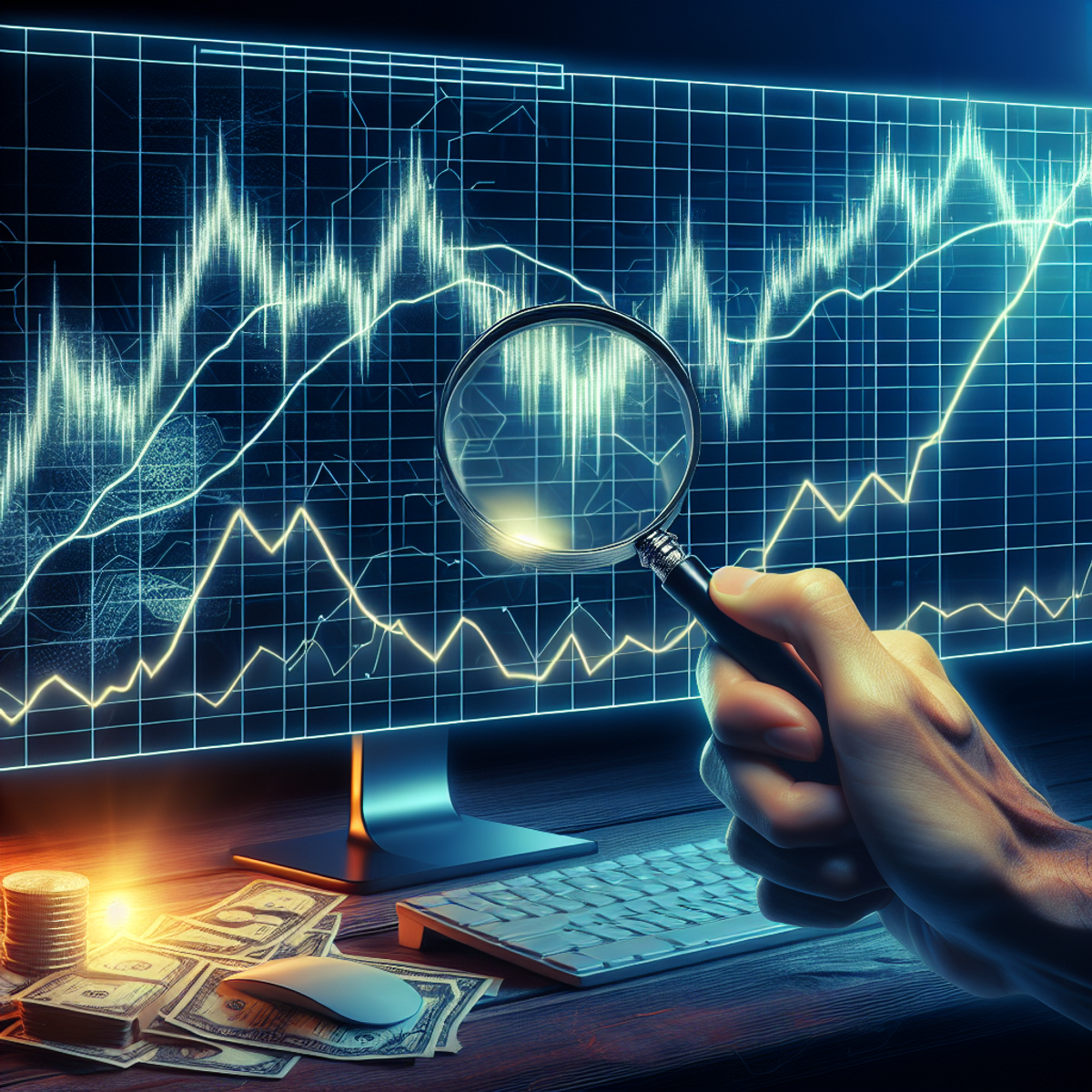
Python offers various libraries that are instrumental in analyzing and manipulating market data in cryptocurrency trading. One such library is 'pandas', which plays a vital role in developing effective trading strategies. Let's explore how 'pandas' can be leveraged for fetching market data, performing technical analysis, and more.
1. Fetching Market Data
- 'ccxt' library, as discussed earlier, provides a unified interface for interacting with multiple exchange APIs. By combining 'ccxt' with 'pandas', you can easily fetch market data from different cryptocurrency exchanges.
- Using the 'ccxt' library, you can retrieve historical price data, order book snapshots, trade history, and other relevant information.
- With the help of 'pandas', you can structure this data into a convenient format for analysis and strategy development.
2. Data Cleaning and Manipulation
- 'pandas' offers powerful tools for cleaning and manipulating data. You can remove missing values, handle outliers, and perform various transformations to ensure the data is accurate and consistent.
- The library facilitates tasks like merging multiple datasets, grouping data by specific criteria, and applying mathematical operations to different columns.
- Additionally, you can use 'pandas' to resample or aggregate time-series data at different intervals (e.g., converting minute-level data to hourly or daily intervals).
3. Technical Analysis
- Technical analysis is a crucial aspect of cryptocurrency trading. 'pandas' provides an extensive range of functionality for conducting technical analysis on market data.
- You can calculate popular technical indicators such as moving averages (simple, exponential), relative strength index (RSI), Bollinger Bands, MACD (Moving Average Convergence Divergence), and more using 'pandas'.
- By leveraging these indicators along with other statistical measures available in the library, you can gain insights into price trends, volatility, and potential trading opportunities.
4. Visualization
- 'pandas' integrates seamlessly with other Python libraries like 'Matplotlib' and 'Seaborn' to create informative visualizations of market data.
- You can generate line plots, candlestick charts, histograms, scatter plots, and various other types of charts to analyze the historical performance of cryptocurrencies.
- These visualizations aid in identifying patterns, spotting anomalies, and validating trading strategies.
By harnessing the power of 'pandas' in combination with other Python libraries such as 'ccxt', you can perform comprehensive market data analysis and derive valuable insights for cryptocurrency trading. Whether it's fetching data from exchanges, cleaning and manipulating datasets, conducting technical analysis, or visually representing trends, 'pandas' proves to be an indispensable tool for traders.
Developing Effective Trading Strategies with PythonWhen it comes to cryptocurrency trading, having a well-defined strategy is crucial for success. Python provides a powerful toolkit for developing and implementing trading strategies. In this section, we will explore some key concepts and techniques for developing effective trading strategies with Python.
Moving Average Crossover Strategy
One popular trading strategy is the moving average crossover strategy. This strategy involves using two moving averages - a shorter-term moving average and a longer-term moving average - to identify buy and sell signals. When the shorter-term moving average crosses above the longer-term moving average, it generates a buy signal, indicating that it may be a good time to enter a long position. Conversely, when the shorter-term moving average crosses below the longer-term moving average, it generates a sell signal, indicating that it may be a good time to exit a long position or enter a short position.
Implementing the moving average crossover strategy in Python is relatively straightforward. Here's an example code snippet using the popular pandas
library:
python import pandas as pd
Fetch historical price data
data = pd.read_csv('historical_data.csv')
Calculate moving averages
data['short_ma'] = data['price'].rolling(window=50).mean() data['long_ma'] = data['price'].rolling(window=200).mean()
Generate buy and sell signals
data['signal'] = 0 data.loc[data['short_ma'] > data['long_ma'], 'signal'] = 1 data.loc[data['short_ma'] < data['long_ma'], 'signal'] = -1
Plotting the strategy
import matplotlib.pyplot as plt
plt.plot(data['price']) plt.plot(data['short_ma']) plt.plot(data['long_ma']) plt.legend(['Price', 'Short MA', 'Long MA']) plt.show()
This code fetches historical price data, calculates the short-term and long-term moving averages, and generates buy and sell signals based on the crossover. The resulting plot visualizes the strategy's performance.
Backtesting Strategies
Before deploying a trading strategy in the live market, it's essential to backtest it using historical price data. Backtesting allows you to evaluate the strategy's performance under different market conditions and assess its profitability and risk.
Python offers several libraries that facilitate backtesting, such as backtrader
and pyalgotrade
. These libraries provide a framework for simulating trades based on historical data and evaluating the strategy's outcome. They enable you to test strategies using various parameters, timeframes, and trading rules.
Parameter Optimization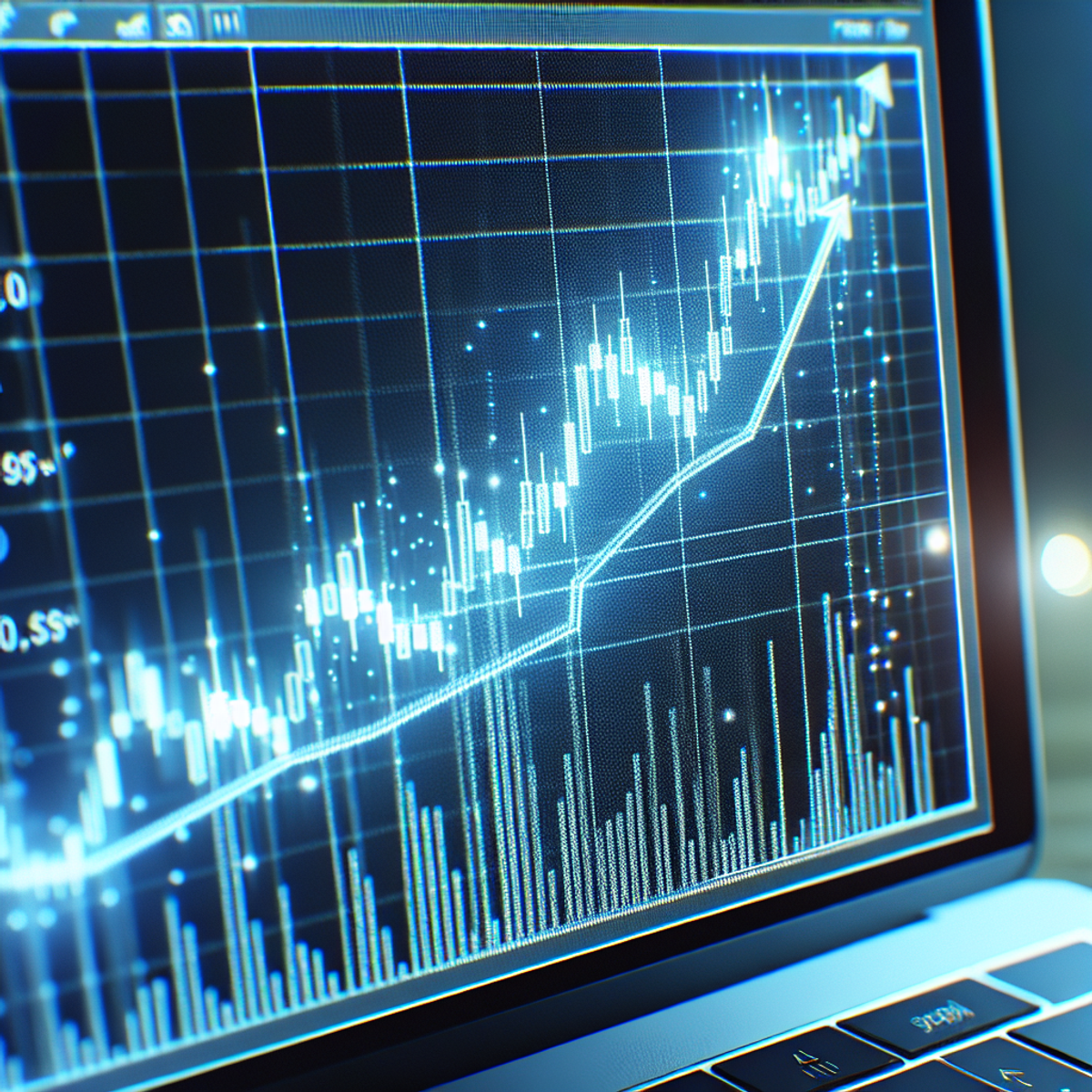
To fine-tune a trading strategy, parameter optimization plays a crucial role. Parameters are variables within a strategy that can be adjusted to achieve better results. By optimizing these parameters, traders aim to improve profitability and reduce risk.
Python provides powerful tools for parameter optimization. Libraries like scipy
and scikit-learn
offer algorithms such as grid search and random search that help find optimal parameter values. Additionally, machine learning techniques like genetic algorithms and particle swarm optimization can be used for more advanced optimization.
By systematically adjusting parameters and evaluating the strategy's performance on historical data, traders can identify the best parameter values for their trading strategies.
Developing effective trading strategies with Python involves understanding key concepts like moving average crossover strategies, performing thorough backtesting, and optimizing parameters. By leveraging Python's extensive libraries and intuitive syntax, traders can build robust strategies that adapt to changing market conditions.
Building and Deploying a Python-based Cryptocurrency Trading Bot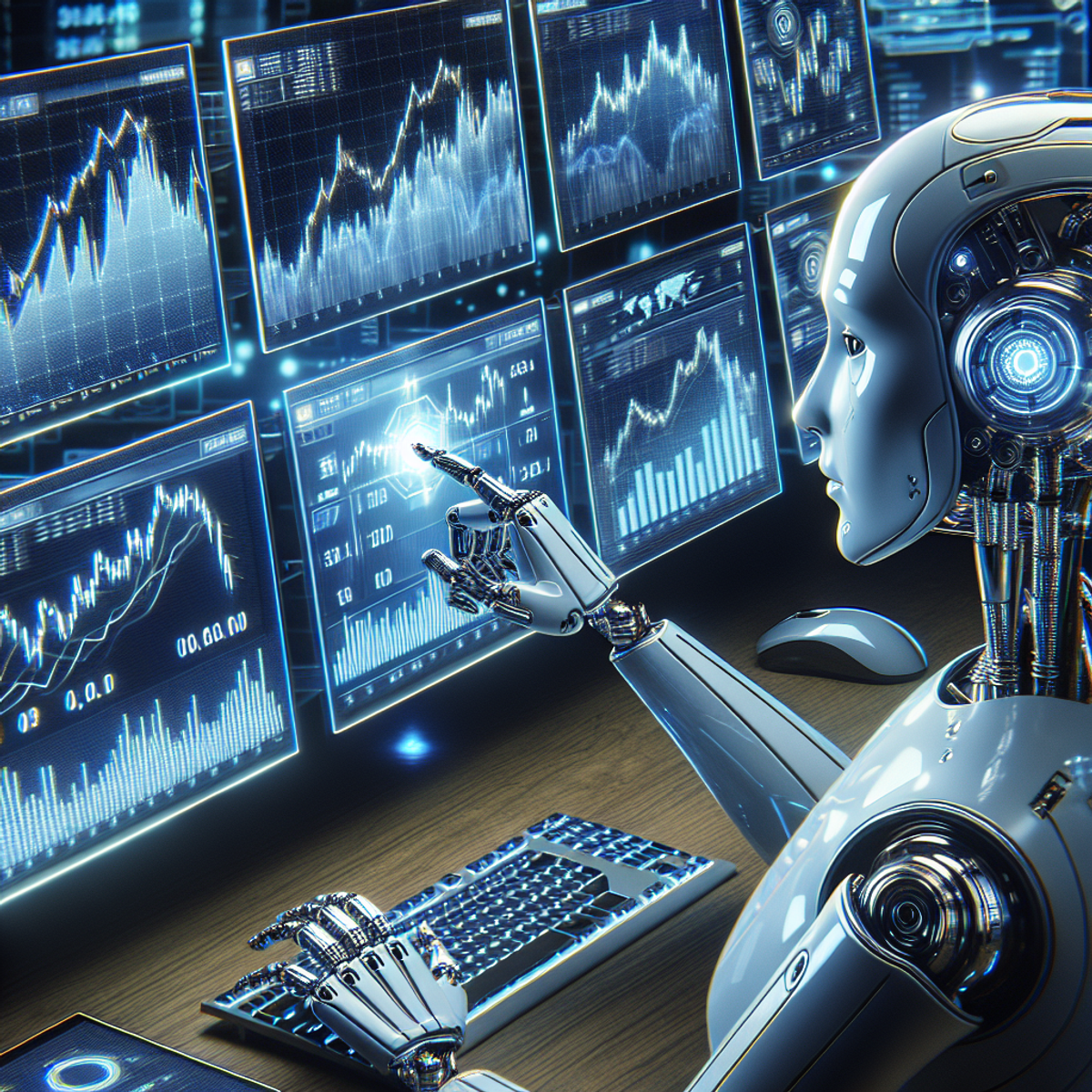
Cryptocurrency trading bots have become increasingly popular due to their ability to automate trading processes, analyze market data, and execute trades based on predefined strategies. Python's versatility and extensive libraries make it an ideal choice for developing cryptocurrency trading bots. One such platform that leverages Python for crypto trading is freqtrade.
Downloading Historical Price Data and Running Backtests with 'freqtrade'
Freqtrade is a powerful open-source cryptocurrency trading bot that enables users to download historical price data, perform backtests, optimize strategies, and deploy them via cryptocurrency exchange APIs. Here's a guide on how to utilize freqtrade for downloading historical price data and running backtests:
- Installation: Begin by installing freqtrade, which can be achieved through direct installation for Linux/MacOS/Windows or by using Docker for installation. This step ensures that you have the necessary environment set up to start using freqtrade.
- Downloading Historical Price Data: Once freqtrade is set up, you can use it to fetch historical price data for various cryptocurrencies. This data is crucial for backtesting trading strategies and gaining insights into potential market trends.
- Backtesting Strategies: With historical price data in hand, you can proceed to backtest your trading strategies using freqtrade. This involves simulating your strategies against past market conditions to evaluate their performance and potential profitability.
- Strategy Optimization: After running backtests, you can optimize your trading strategies based on the insights gained from the historical price data. This iterative process allows you to fine-tune your strategies for better results in live trading scenarios.
- Utilizing Crypto Exchange API: Freqtrade offers seamless integration with cryptocurrency exchange APIs, enabling users to deploy their optimized trading strategies directly on supported exchanges.
By following these steps, traders can harness the power of freqtrade to develop and test robust trading strategies tailored to the dynamic cryptocurrency market.
Taking Advantage of Advanced Features in 'freqtrade' for Strategy Optimization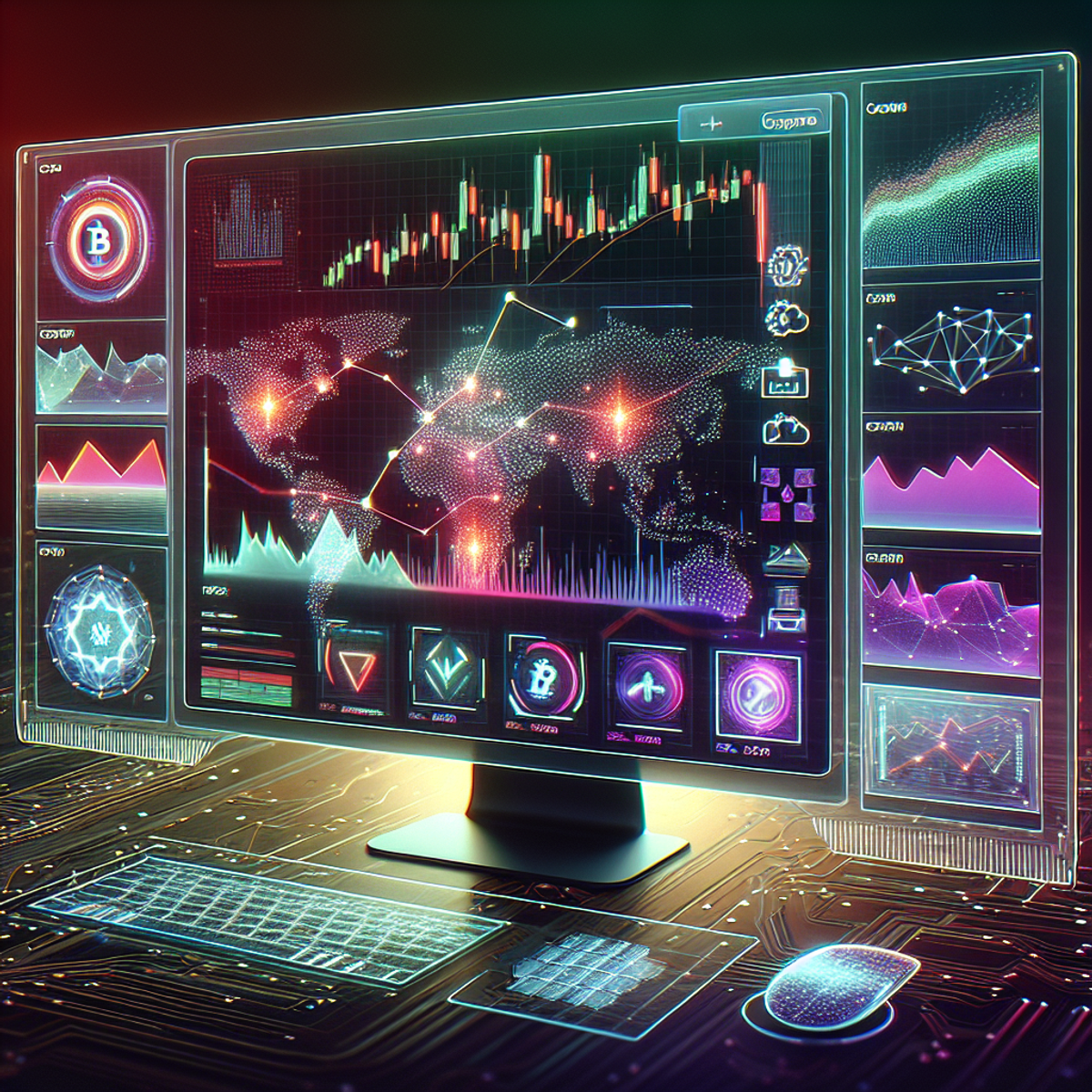
In addition to basic functionalities like historical data retrieval and backtesting, freqtrade offers advanced features for strategy optimization:
- Parameter Tuning: Freqtrade allows traders to optimize strategy parameters such as buy/sell indicators, stop-loss limits, trade size, and more, empowering them to adapt their strategies to varying market conditions.
- Time Frame Selection: Traders can explore different time frames when optimizing their strategies, considering varied intervals for analyzing price movements and identifying optimal entry/exit points.
- Coin Pair Filters: Freqtrade provides filters for selecting specific coin pairs based on predefined criteria such as volume, liquidity, or price range. This feature enables traders to focus on assets that align with their preferred trading characteristics.
By leveraging these advanced features in freqtrade, traders can refine their trading strategies with precision and adaptability, enhancing their potential for success in the volatile world of cryptocurrency trading.
Overall, freqtrade serves as a comprehensive tool for building and deploying Python-based cryptocurrency trading bots while offering extensive capabilities for strategy development and optimization.
Taking Advantage of Advanced Features in 'freqtrade' for Strategy Optimization
'freqtrade' is a popular open-source framework for developing cryptocurrency trading bots in Python. It supports backtesting strategies using historical price data and offers several advanced features for strategy development and deployment.
Optimize Strategies through Parameter Tuning
One of the main benefits of using 'freqtrade' is its ability to optimize trading strategies through parameter tuning. This means traders can adjust different settings of their strategies to find the best values that generate the highest profits.
Some of the parameters that can be optimized in 'freqtrade' include:
- Stop Loss: Adjusting the stop loss level can help minimize losses and protect capital during market downturns.
- Take Profit: Optimizing the take profit level can help maximize profits by closing positions at the most advantageous price points.
- Trailing Stop: By adjusting the trailing stop parameter, traders can lock in profits as the price moves in their favor, while still allowing for potential further gains.
- Indicator Periods: Fine-tuning the indicator periods used in technical analysis can help identify optimal entry and exit points for trades.
By systematically testing different parameter values, traders can identify the ideal combination that results in the highest profitability and risk-adjusted returns. This iterative process of optimization ensures that trading strategies are tailored to specific market conditions and maximize profit potential.
Time Frame Selection
Another important feature offered by 'freqtrade' is the ability to select different time frames for backtesting and strategy development. Traders can choose from a range of time frames, such as minutes, hours, or days, depending on their preferred trading style and desired level of granularity.
Different time frames provide varying levels of detail and insights into market behavior. Shorter time frames offer more frequent trading opportunities but may be more susceptible to market noise. On the other hand, longer time frames provide a broader perspective and help identify long-term trends.
By experimenting with different time frames, traders can gain a better understanding of how their strategies perform under different market conditions and adjust their approach accordingly. This flexibility allows for adaptability in fast-changing cryptocurrency markets.
Advanced Backtesting Features
'freqtrade' also offers advanced backtesting features that enable traders to simulate trades using historical price data. This allows them to evaluate the performance of their strategies under different market scenarios before deploying them in live trading.
Some of the key features of 'freqtrade' for backtesting include:
- Realistic Simulation: 'freqtrade' simulates trades based on historical price data and accurately replicates trading conditions in real-time. This helps traders assess the viability and profitability of their strategies without risking actual capital.
- Performance Metrics: Traders can analyze various performance metrics provided by 'freqtrade', such as profit/loss ratio, win rate, risk-adjusted return, and drawdown. These metrics help in evaluating the effectiveness of trading strategies and making data-driven decisions.
- Visualization Tools: 'freqtrade' offers visualization tools that allow traders to visualize trade executions, portfolio performance, and other relevant metrics. These visual representations help in gaining insights into strategy performance and identifying areas for improvement.
By leveraging these advanced features in 'freqtrade
Challenges and Risks in Python-based Cryptocurrency Trading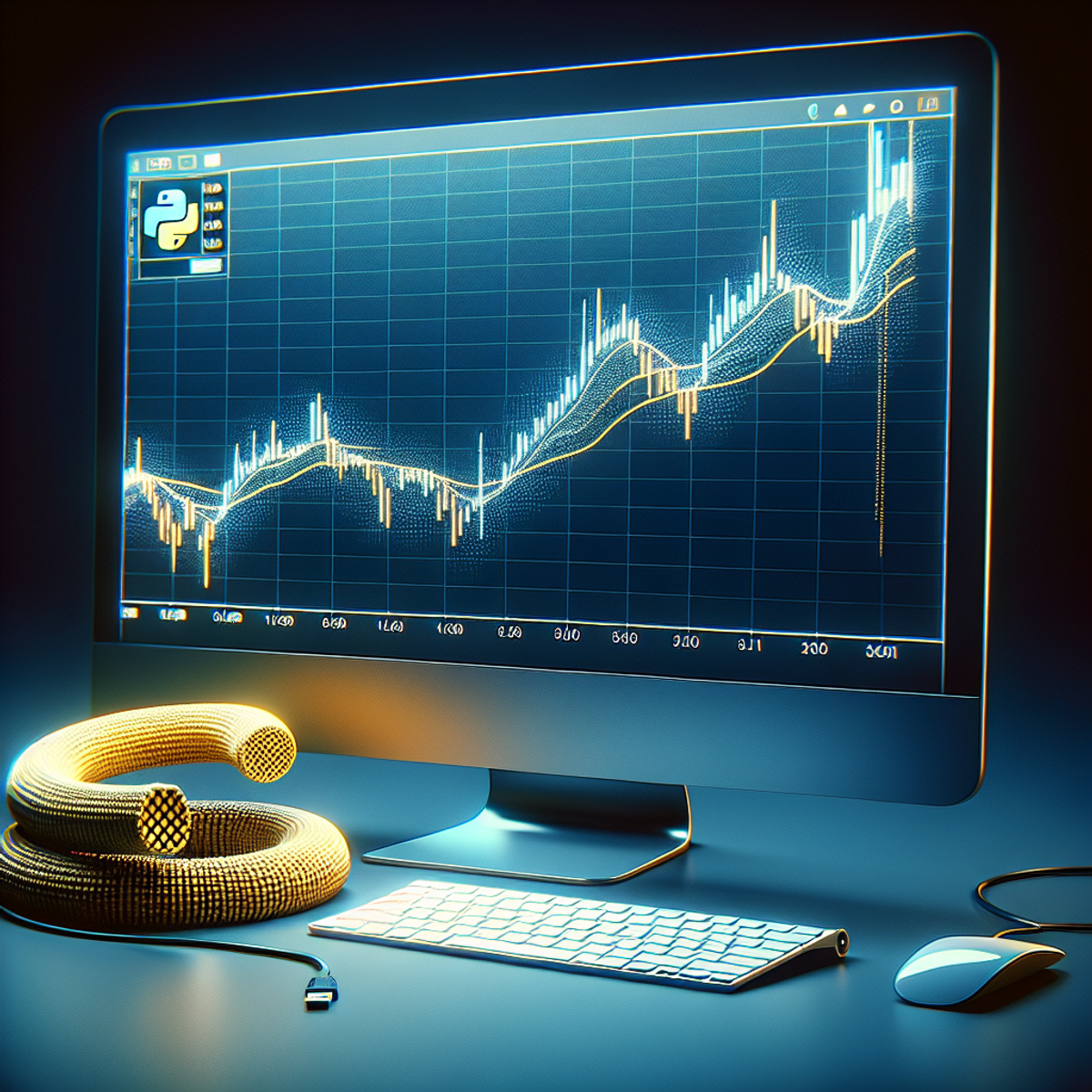
When venturing into Python-based cryptocurrency trading, it's crucial to understand the potential challenges and risks that traders may encounter. These factors can significantly impact trading results and overall success in the fast-paced and unpredictable cryptocurrency market.
1. Technological Complexity and Integration
One of the primary challenges for Python traders is effectively combining different software components. While Python offers numerous libraries and tools for trading, comprehending and integrating these resources can be daunting. Traders need a solid understanding not only of Python but also APIs, data structures, and algorithms to create robust trading strategies. This research paper on artificial intelligence and machine learning in finance could provide valuable insights.
2. Market Volatility and Risk Management
The cryptocurrency market is notorious for its extreme volatility, posing a significant risk for traders. Sudden price fluctuations and unpredictable market behavior can lead to substantial financial losses if not managed well. Python users in cryptocurrency trading must establish sound risk management plans and constantly monitor market conditions to minimize potential losses. This article on essential tips and strategies for successful cryptocurrency trading might prove beneficial.
3. Security Concerns and Cyber Threats
Like any digital asset-related activity, security is paramount in cryptocurrency trading. Developing trading bots using Python necessitates understanding cybersecurity best practices. Traders must ensure API keys are securely stored, sensitive data is encrypted, and remain updated on emerging cyber threats to safeguard their trading operations. This report on the use of AI applications in the securities industry highlights the importance of cybersecurity.
4. Data Quality and Reliability
Python traders heavily rely on accurate and reliable market data to make informed decisions. However, ensuring the quality of data from various sources can be a challenge. Inconsistencies or errors in data can significantly impact the performance of trading strategies, leading to suboptimal results. Hence, traders must diligently verify the accuracy of data obtained through Python libraries and APIs. This scientific article on data quality assessment could provide valuable insights.
5. Operational Risks and System Failures
The operational aspect of Python-based cryptocurrency trading comes with its own set of challenges. Traders must be prepared to handle potential system failures, downtime, or technical issues that could disrupt automated trading activities. Implementing backup plans, continuous monitoring systems, and contingency measures is crucial for effectively managing operational risks.
6. Regulatory Uncertainty
The ever-changing regulations surrounding cryptocurrencies further complicate matters for Python traders. Adapting to regulatory changes in different jurisdictions requires diligent attention and proactive compliance efforts. Traders must stay updated on legal developments affecting cryptocurrency trading practices while ensuring their Python-based strategies align with regulations.
Understanding these challenges demands a combination of technical knowledge, strategic thinking, risk management skills, and flexibility in response to market changes and regulatory shifts. By recognizing these potential obstacles, Python traders can actively work towards overcoming them and strengthen their ability to
The Future of Python in Cryptocurrency Trading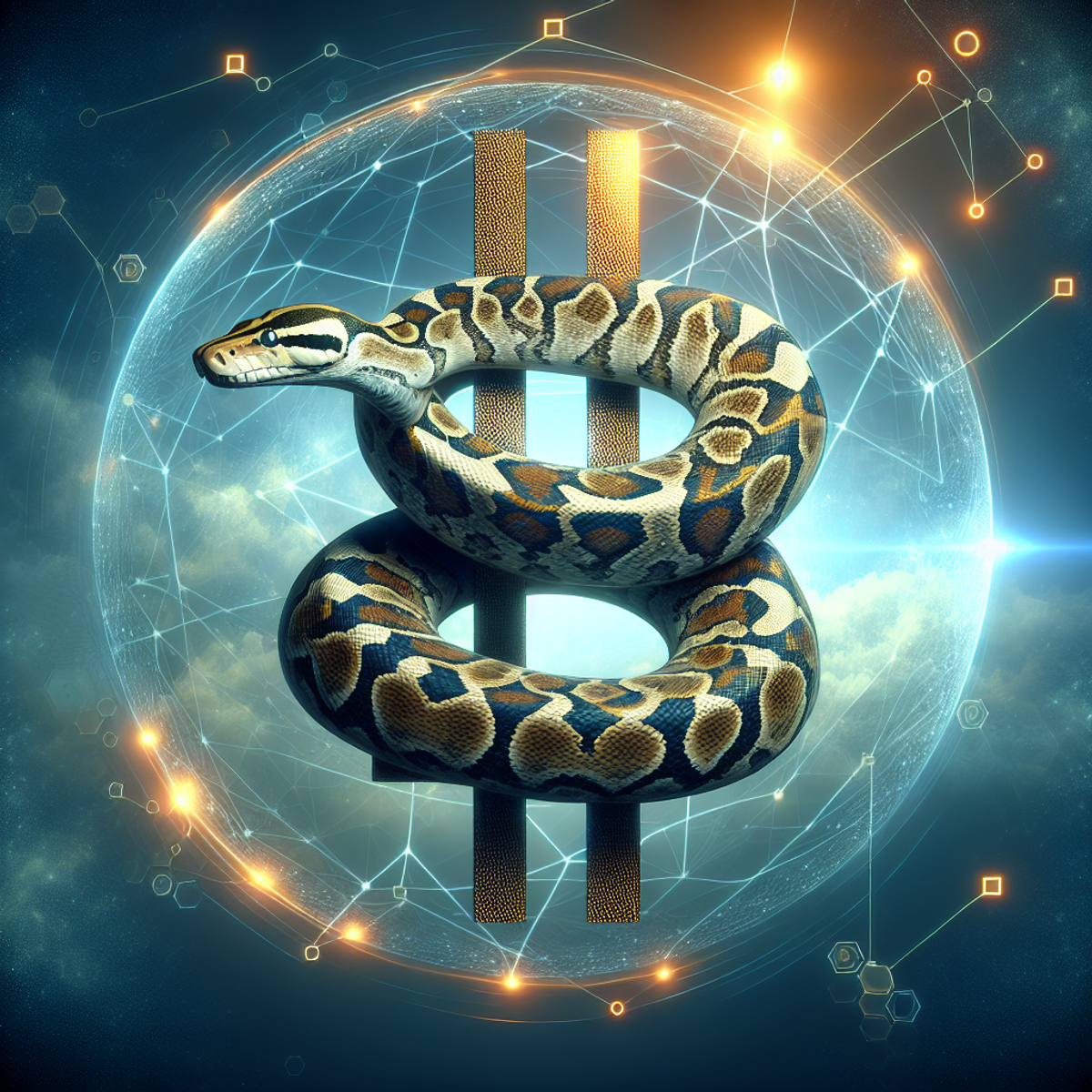
Looking ahead to the future of cryptocurrency trading, it's clear that Python will continue to play a central role in shaping the landscape. Several key factors point to the evolving significance of Python in this domain, each contributing to its continued relevance and influence:
Machine Learning/AI Advancements
The integration of machine learning and artificial intelligence (AI) technologies into cryptocurrency trading is increasing. Python's extensive libraries for machine learning, such as TensorFlow, scikit-learn, and Keras, position it as a leading language for developing AI-driven trading algorithms. These technologies enable traders to analyze large amounts of data, identify patterns, and make informed decisions in real-time. As recent publications suggest, machine learning continues to change trading strategies, and Python's ability to adjust and its strength make it an ideal choice for using these advanced techniques.
Regulatory Developments
The rules surrounding cryptocurrency trading are always changing, with governments and financial authorities trying to establish systems that ensure transparency and security within the industry. As regulatory obligations become stricter, there is a growing need for advanced tools that can help with compliance and risk management. Python's flexibility and ease of connecting with external APIs make it well-suited for meeting regulatory requirements within automated trading systems. Additionally, as described in this article on RegTech, its open-source nature allows for quick adjustments to changing regulatory standards, ensuring that traders can follow evolving legal frameworks.
Interoperability with Emerging Technologies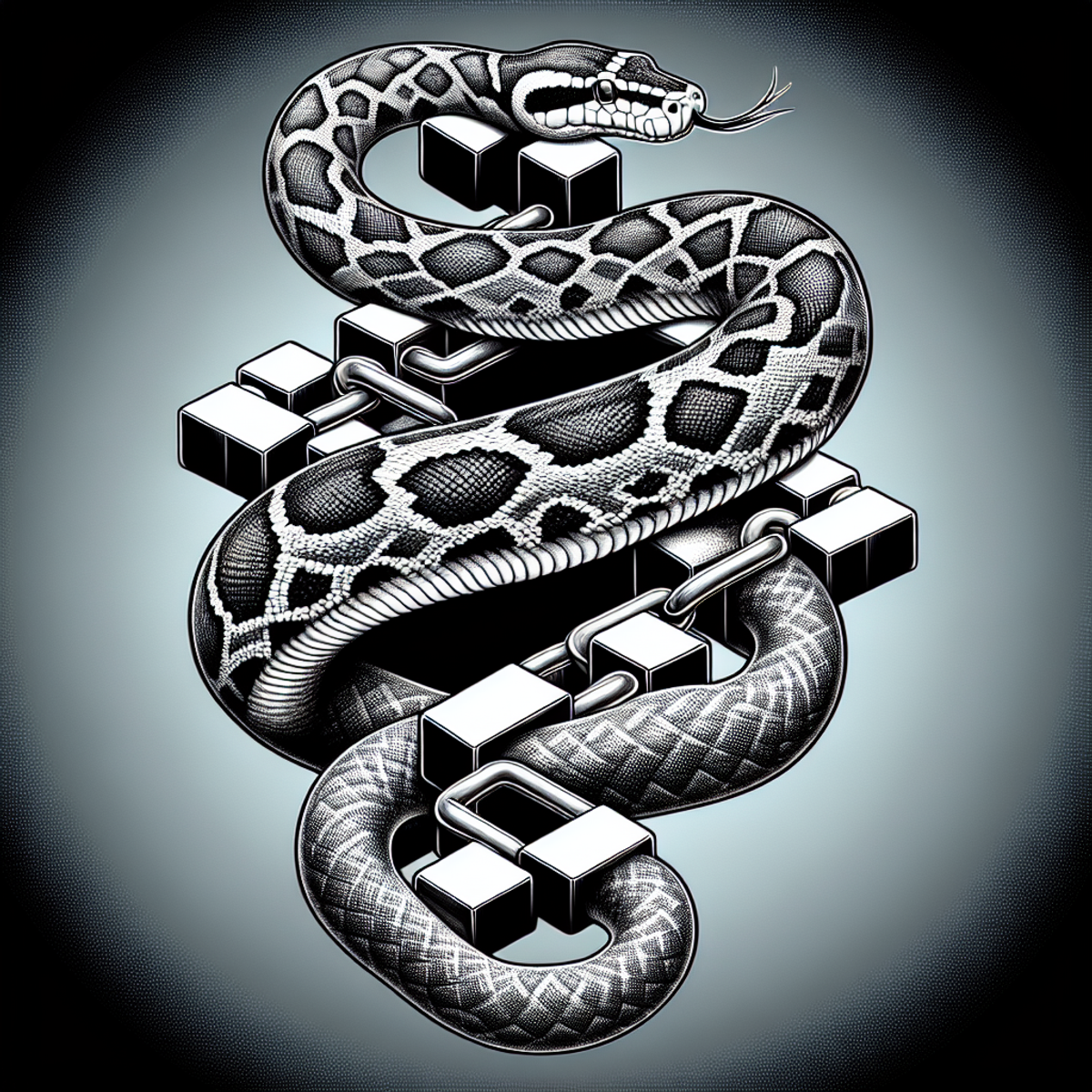
Python's ability to work well with many new technologies puts it at the forefront of innovation in cryptocurrency trading. From integrating blockchain technology to using decentralized finance (DeFi) applications, Python's adaptability allows for easy collaboration with cutting-edge developments in the crypto world. This ability empowers traders to take advantage of new opportunities and navigate the complexities of a constantly expanding system. As discussed in this scientific article, Python's interoperability with emerging technologies is crucial for driving advancements in cryptocurrency trading.
As Python continues to change alongside these developments, its role in cryptocurrency trading will become even more important. Its ability to adjust to shifting market trends, incorporate advanced technologies, and meet regulatory requirements shows its lasting importance in shaping the future of digital asset trading.
Conclusion
Throughout this article, we've explored how Python is essential in the fast-paced world of cryptocurrency trading. Its simple syntax, extensive libraries, and powerful capabilities make it the top choice for creating automated trading strategies and executing trades quickly.
Using Python, traders can develop cryptocurrency trading bots that:
- Fetch market data
- Analyze data using libraries like pandas
- Execute orders based on predefined strategies
The ccxt library acts as a bridge to cryptocurrency exchange APIs, allowing seamless communication with different exchanges.
Why Python?
Python offers several advantages for cryptocurrency trading:
- Ease of use: Python's clean and readable syntax makes it beginner-friendly and allows for faster development.
- Vast library ecosystem: The availability of libraries like pandas, NumPy, and matplotlib simplifies data analysis and visualization.
- Community support: Python has a large and active community of developers who contribute to open-source projects and provide assistance when needed.
- Integration capabilities: Python can easily integrate with other languages like C++ or JavaScript, enabling access to specialized tools or web-based platforms.
Further Resources
To deepen your knowledge of Python in cryptocurrency trading, here are some recommended resources:
- Books:
- "Python for Finance" by Yves Hilpisch
- "Mastering Bitcoin" by Andreas M. Antonopoulos
- Courses:
- "Algorithmic Trading Using Python" on Udemy
- "Cryptocurrency Trading with Python" on Coursera
- Online communities:
- Reddit's r/algotrading
- Quantopian forums
Embracing Automation with Python
In summary, Python offers immense possibilities for individuals interested in cryptocurrency trading. By leveraging its capabilities, you can:
- Automate your trading strategies
- Effectively analyze market data
- Stay ahead in this ever-changing industry
So why not start exploring Python's potential in cryptocurrency trading and embark on your own projects today?
Remember, automation is the key to success in the world of cryptocurrency trading, and Python is your indispensable tool to unlock that potential.
FAQs (Frequently Asked Questions)
What is Python's role in cryptocurrency trading?
Python plays a significant role in cryptocurrency trading by enabling the development of trading strategies, execution of trades, and creation of cryptocurrency trading bots. Its easy syntax and extensive libraries make it an ideal choice for automation in the fast-paced world of cryptocurrency trading.
How has Python emerged as the go-to programming language for creating cryptocurrency trading bots?
Python has emerged as the go-to programming language for creating cryptocurrency trading bots due to its extensive libraries and easy syntax. It enables seamless interactions with exchange APIs, making it easier to buy and sell cryptocurrencies on exchanges through automated bots.
What are some key Python libraries for cryptocurrency trading?
Some key Python libraries for cryptocurrency trading include 'ccxt' for interacting with multiple exchange APIs and 'pandas' for data analysis and manipulation in developing trading strategies.
What are the features and advantages of 'ccxt' library in detail?
'ccxt' library provides a unified interface for interacting with multiple exchange APIs, making it easier to access and trade on various cryptocurrency exchanges. It simplifies the process of connecting to different exchanges and retrieving market data.
How can 'pandas' be utilized for market data analysis in cryptocurrency trading?
'pandas' library is significant for data analysis and manipulation in developing trading strategies. It can be leveraged for fetching market data, performing technical analysis, and gaining insights into market trends.
What are some popular trading strategies that can be implemented with Python?
Popular trading strategies like the moving average crossover strategy can be implemented with Python. It is also important to backtest these strategies using historical price data to evaluate their performance, which can be facilitated by Python libraries such as 'backtrader' or 'pyalgotrade'.
How can one download historical price data and run backtests using 'freqtrade'?
'freqtrade' allows users to download historical price data for cryptocurrencies and perform backtests to evaluate the performance of their trading strategies. It provides a framework for developing and deploying cryptocurrency trading bots in Python.
What are some potential challenges faced by Python traders in cryptocurrency trading?
Python traders may face potential challenges such as technological issues, market volatility, regulatory changes, and security concerns in the fast-paced world of cryptocurrency trading.
What is the future outlook for Python in the world of cryptocurrency trading?
The future of Python in cryptocurrency trading looks promising, considering advancements in machine learning/AI, regulatory developments, and its continued relevance as a powerful tool for automation and strategy development.
How can readers further explore opportunities presented by Python in cryptocurrency trading?
Readers are encouraged to explore opportunities presented by Python in cryptocurrency trading by engaging with like-minded traders and developers through books, courses, online communities, and experimenting with their own projects.
Comments
Post a Comment